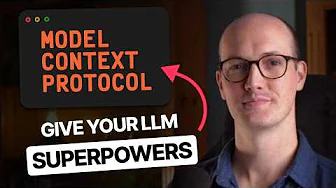
The Model Context Protocol (mCP) opens up powerful possibilities for extending AI capabilities through custom tools. In this tutorial, we'll explore how to create a minimal mCP server that connects directly to Claude, allowing you to build custom functionality that your AI assistant can leverage—all with a single TypeScript file and no build process.
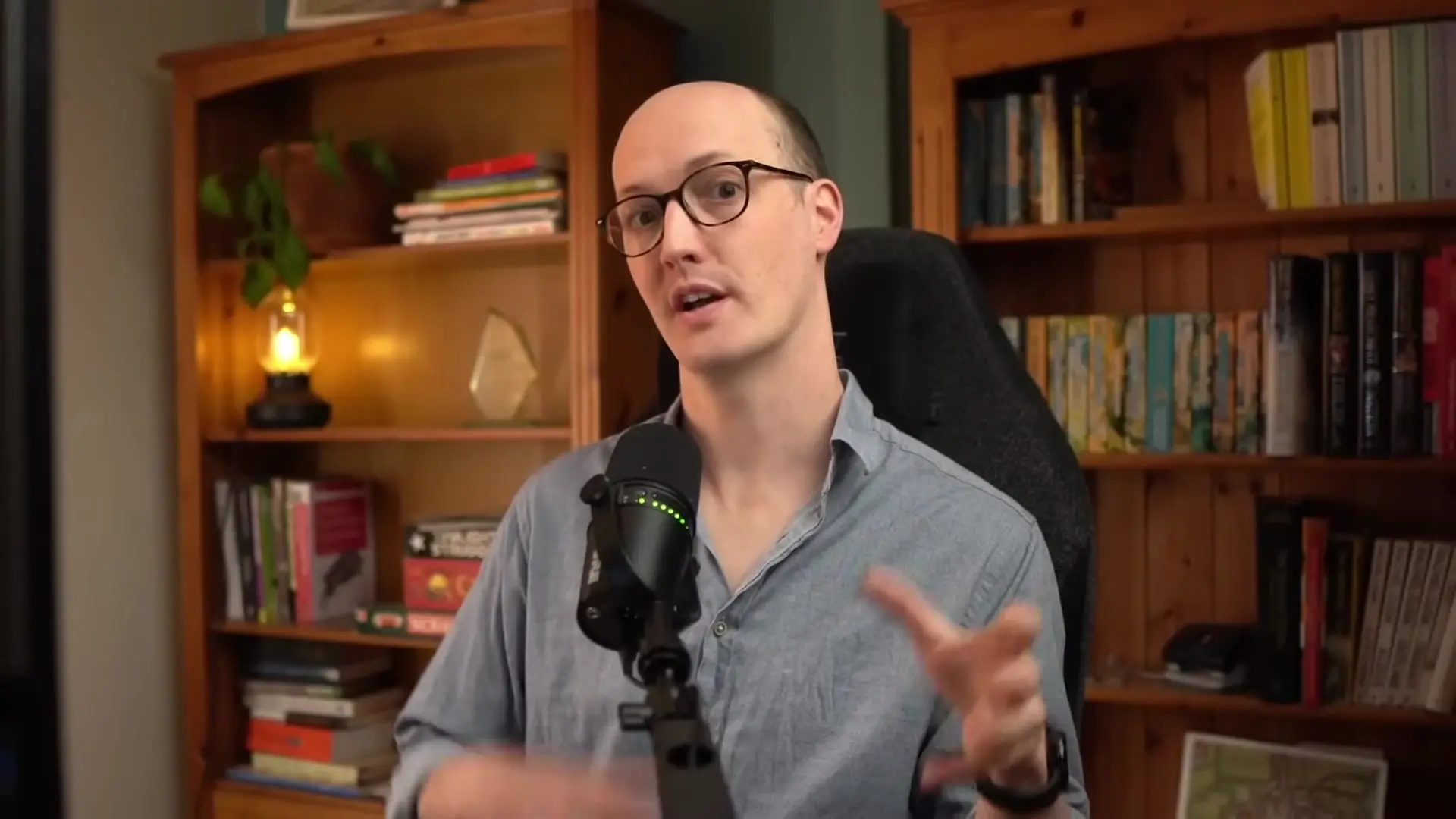
Understanding the Model Context Protocol
The Model Context Protocol (mCP) provides a standardized way for AI models to interact with external tools and services. By implementing this protocol, we can create context models that extend Claude's capabilities, allowing it to perform actions it couldn't do natively, such as fetching real-time data or interacting with local systems.
Creating Your First mCP Server
Let's start by creating a simple mCP server that provides a weather tool. This example demonstrates the protocol model in action with minimal setup.
Step 1: Set Up Your Project
First, create a new file named `main.ts`. This will contain our entire server implementation without requiring any build steps, thanks to TSX which can run TypeScript files directly.
Step 2: Initialize the mCP Server
import { MCPServer } from '@anthropic/model-context-protocol-sdk';
import { StandardIOServerTransport } from '@anthropic/model-context-protocol-sdk';
import { z } from 'zod';
// Create a new MCP server
const server = new MCPServer({
name: 'weather-server'
});
We've imported the necessary modules and initialized our server with a name. The Model Context Protocol SDK provides the foundation for our implementation, while Zod will help us define the schema for our tool's arguments.
Step 3: Add a Tool to Your Server
// Add a weather tool to our server
server.addTool({
name: 'getWeather',
description: 'Get the current weather for a city',
inputSchema: z.object({
city: z.string().describe('The name of the city to get weather for')
}),
handler: async ({ city }) => {
// In a real implementation, this would call a weather API
return [{ type: 'text', text: `The weather in ${city} is sunny` }];
}
});
Here we've defined a tool called `getWeather` that accepts a city name as input and returns the weather condition. In a production context model example, you would replace the hardcoded response with an actual API call to a weather service.
Step 4: Set Up Communication with Claude
To enable communication between our server and Claude, we need to set up a transport layer. The Model Context Protocol uses standard I/O for this communication.
// Initialize the transport layer
const transport = new StandardIOServerTransport();
// Connect the server to the transport
server.connect(transport);
// Start the server
transport.start();
This code establishes the communication channel between our mCP server and Claude. The protocol tutorial demonstrates how Standard I/O provides a simple yet effective way for tools to exchange information with the AI model.
Registering Your Server with Claude
Now that our server is complete, we need to register it with Claude so it can access our custom tool. This is done using the Claude CLI.
claude mcp add --name weather-example --global --command "npx tsx /path/to/your/main.ts"
This command tells Claude about our mCP server. The `--global` flag makes it available in all Claude sessions, and the command specifies how to run our server using TSX to execute the TypeScript file directly.
Testing the Integration
With everything set up, you can now launch Claude and test your custom tool. Simply ask Claude about the weather in a specific city:
User: Get the weather in Oxford
Claude: I'll check the weather in Oxford for you.
[weather-example getWeather] The weather in Oxford is sunny
Claude automatically detects that it needs to use your custom tool, calls the `getWeather` function with "Oxford" as the city parameter, and returns the result. This demonstrates how the context model seamlessly integrates with the AI's capabilities.
Expanding Your mCP Server
This simple example is just the beginning of what you can do with the Model Context Protocol. You can add multiple tools to your server to create a comprehensive context model that extends Claude's capabilities in various ways:
- Running local Docker commands
- Executing Git operations
- Managing database migrations
- Fetching data from private APIs
- Interacting with local files and systems
Benefits of the Model Context Protocol Approach
Using mCP offers several advantages when implementing context models for AI assistants:
- Simplicity: A single TypeScript file with no build process
- Flexibility: Add any functionality you need through custom tools
- Security: Tools run locally under your control
- Extensibility: Easily add new tools as your needs evolve
- Standardization: Follow a consistent protocol for all tool interactions
Conclusion
The Model Context Protocol offers a powerful way to extend AI capabilities through custom tools. With just a simple TypeScript file, you can create a server that connects to Claude and enables it to perform custom actions. This approach to protocols and models opens up endless possibilities for AI integration in your workflows.
As you become more familiar with mCP, you can create more sophisticated context models that leverage the full power of your local environment and external services, making Claude an even more versatile assistant for your specific needs.
Let's Watch!
Build an mCP Server for Claude in 2 Minutes: The Simplest TypeScript Setup
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence