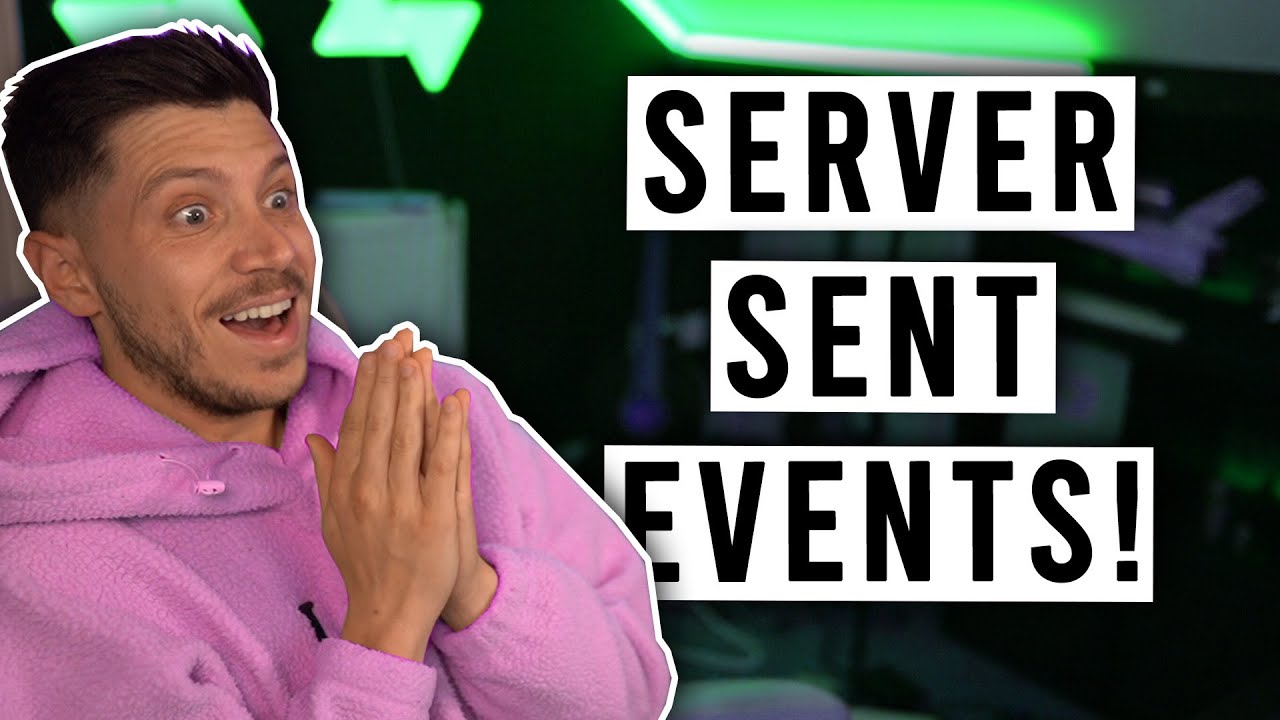
One of the most exciting features coming to .NET 10 is native support for Server-Sent Events (SSE), a game-changing capability for developers building real-time applications. This powerful feature allows for efficient unidirectional streaming of data from server to client without the complexity of SignalR or WebSockets. If you're developing APIs or web applications that need to push real-time updates like stock tickers, live orders, or notifications, this feature will revolutionize your approach.
What Are Server-Sent Events in .NET 10?
Server-Sent Events (SSE) is a standard that enables a server to push updates to a client over a single HTTP connection. Unlike WebSockets or SignalR, SSE is unidirectional—data flows only from server to client. This makes it perfect for scenarios where you don't need bidirectional communication but still want real-time updates without polling.
The beauty of SSE in .NET 10 is its simplicity. It works with standard HTTP GET requests and is natively supported by modern browsers. No special protocols, no complex setup—just straightforward, efficient data streaming.
Implementing SSE in a .NET 10 Minimal API
Let's see how to implement Server-Sent Events in a .NET 10 Minimal API. We'll create a simple endpoint that streams live food orders to clients.
app.MapGet("/live-orders", async (CancellationToken cancellationToken) => {
// Return type is Results<ServerSentEvents>
return Results.Extensions.ServerSentEvents(
GetOrdersStream(cancellationToken),
cancellationToken);
});
The key component here is the `ServerSentEvents` result type, which accepts an async enumerable of items. This is what enables the streaming functionality. Let's look at how to implement the order stream generator:
private static async IAsyncEnumerable<ServerSentEventsItem<FoodOrder>> GetOrdersStream(
[EnumeratorCancellation] CancellationToken cancellationToken)
{
while (!cancellationToken.IsCancellationRequested)
{
// Simulate delay between order generation
await Task.Delay(1000, cancellationToken);
// Create and yield a new order event
yield return new ServerSentEventsItem<FoodOrder>
{
Data = OrderGenerator.CreateOrder(),
Event = "order", // Event type identifier
ReconnectionInterval = TimeSpan.FromMinutes(1)
};
}
}
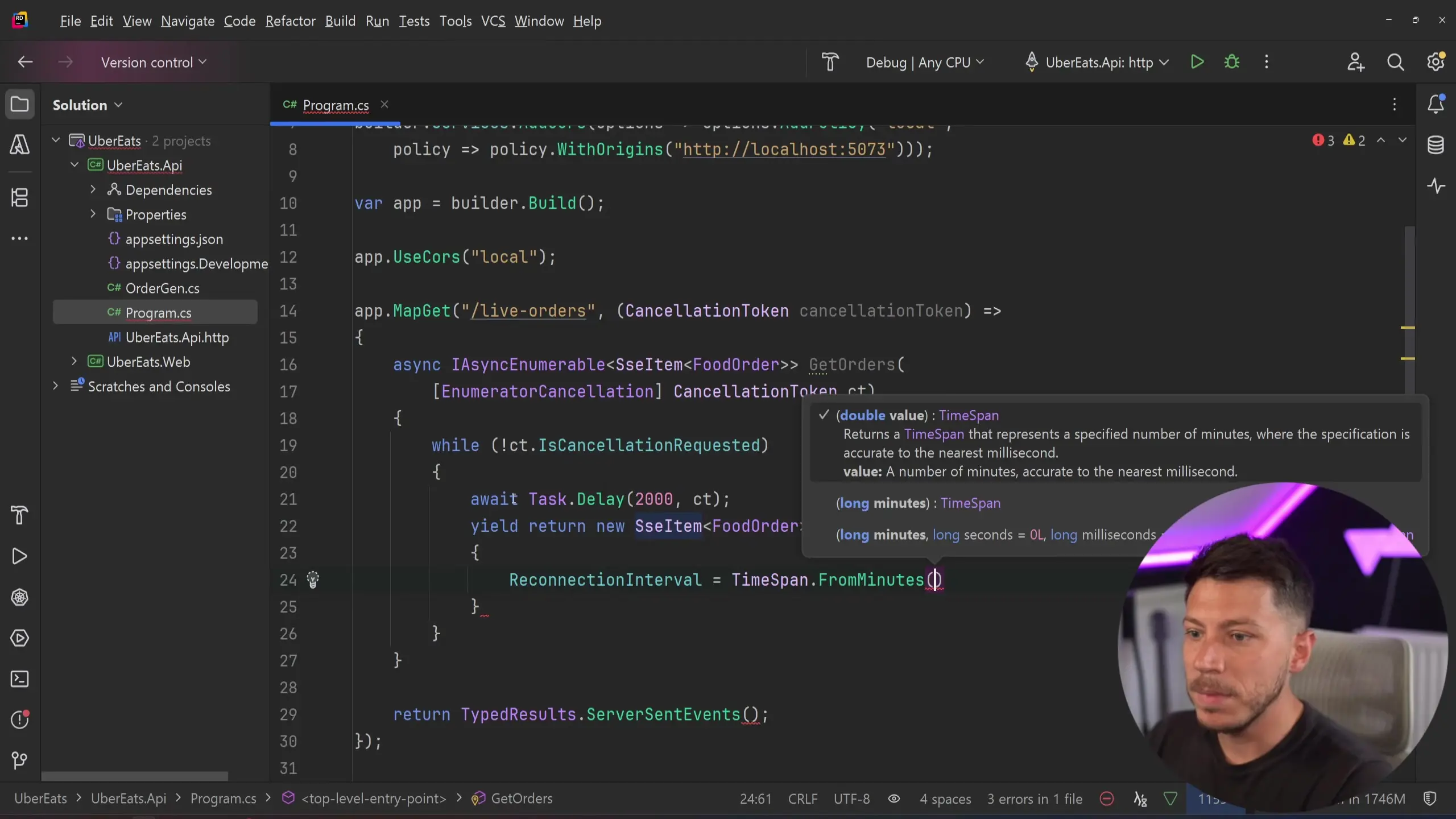
This implementation uses an async enumerable to continuously generate food order events. Each event is wrapped in a `ServerSentEventsItem
- The data object (a FoodOrder in this case)
- An event type identifier ("order")
- Optional reconnection interval (1 minute)
- Optional event ID (not shown in this example)
The cancellation token is crucial here as it allows the connection to be properly terminated when needed, preventing resource leaks.
Consuming SSE in a Web Client
One of the major advantages of Server-Sent Events is that they're natively supported by modern browsers through the EventSource API. Here's how to consume our SSE endpoint in a web application:
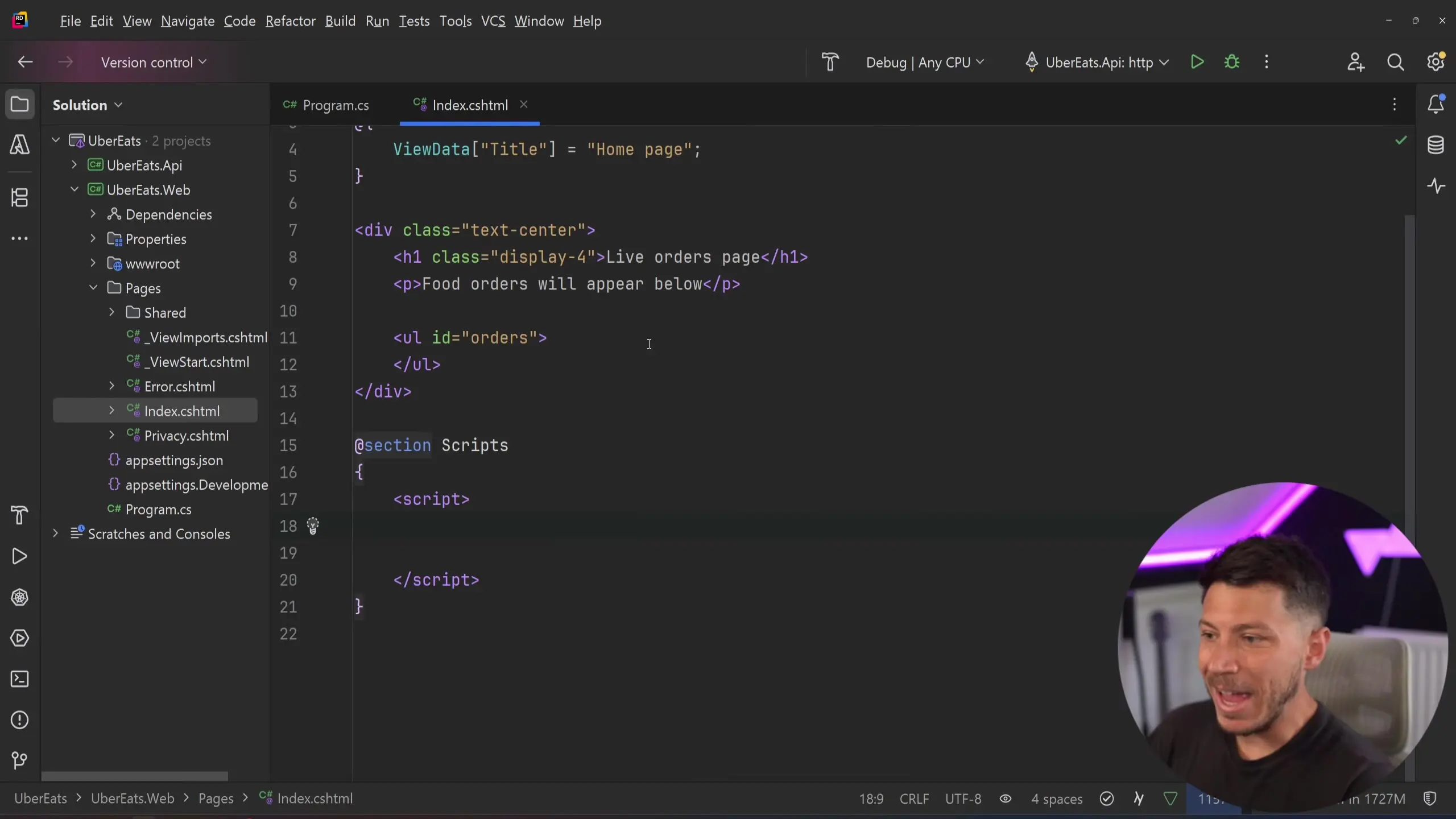
// Create an EventSource connected to our endpoint
const eventSource = new EventSource('/live-orders');
// Listen for events of type "order"
eventSource.addEventListener('order', function(event) {
const order = JSON.parse(event.data);
// Create a new list item for the order
const listItem = document.createElement('li');
listItem.textContent = `New order: ${order.name} - $${order.price}`;
// Add it to our orders list
document.getElementById('ordersList').appendChild(listItem);
});
// Handle connection errors
eventSource.onerror = function(error) {
console.error('EventSource failed:', error);
};
The JavaScript code establishes a connection to our SSE endpoint and listens specifically for events of type "order". When an order event is received, it parses the JSON data and adds the order to a list in the UI. The browser handles reconnection automatically based on the reconnection interval we specified.
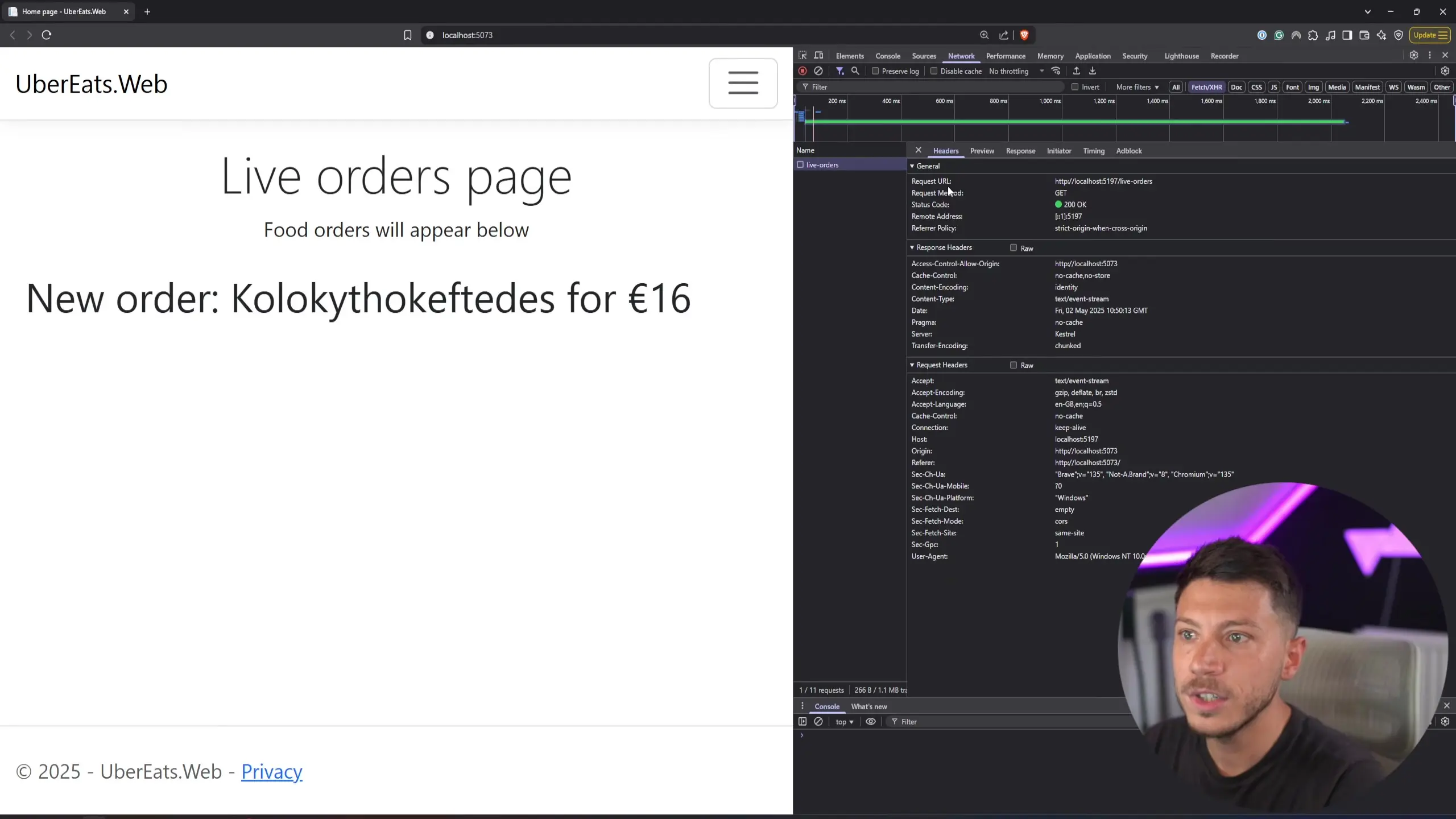
Advantages of Server-Sent Events Over Alternatives
- Simpler implementation compared to SignalR or WebSockets for unidirectional scenarios
- Works over standard HTTP with no special protocols required
- Native browser support with automatic reconnection handling
- Lower overhead than polling approaches
- Compatible with existing HTTP infrastructure (proxies, load balancers, etc.)
- Easy to implement with .NET 10's new ServerSentEvents result type
Use Cases for Server-Sent Events
SSE is ideal for many real-time scenarios where updates flow from server to client:
- Live dashboards displaying metrics or KPIs
- Stock tickers and financial data feeds
- Social media feeds and notifications
- Order tracking systems
- Live sports scores and updates
- IoT device monitoring
- Log streaming and system monitoring
Technical Considerations When Using SSE
While Server-Sent Events offer many advantages, there are some technical considerations to keep in mind:
- Browser connection limits: Most browsers limit the number of concurrent connections to a domain (typically 6-8), so use SSE connections judiciously.
- Reconnection strategy: While browsers handle basic reconnection, you may need custom logic for more complex scenarios.
- Error handling: Implement proper error handling both server-side and client-side.
- Data format: JSON is commonly used, but you can transmit any text-based format.
- Event filtering: Use event types to allow clients to filter which events they process.
SSE vs. SignalR: When to Use Each
Server-Sent Events and SignalR serve different purposes and have different strengths:
- Use SSE when: You need simple server-to-client updates, want browser native support, or prefer HTTP-based solutions with minimal overhead.
- Use SignalR when: You need bidirectional communication, client-to-server messages, or advanced features like groups and connection management.
Conclusion
Server-Sent Events in .NET 10 provide a powerful yet simple way to implement real-time updates in your web applications. With native support through the new ServerSentEvents result type, implementing streaming APIs has never been easier. This feature fills an important gap between traditional request/response patterns and more complex real-time solutions like SignalR.
As .NET 10 continues to evolve, this feature demonstrates Microsoft's commitment to providing developers with modern, efficient tools for building real-time applications. Whether you're creating dashboards, monitoring systems, or any application that benefits from live updates, Server-Sent Events should be in your toolkit.
Let's Watch!
The Coolest .NET 10 Feature: Server-Sent Events for Real-Time Data Streaming
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence