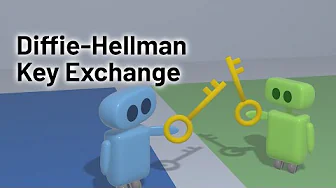
In today's interconnected digital world, secure communication between computers is essential. But how do two computers that have never communicated before establish a secure connection? This is where the Diffie-Hellman key exchange protocol comes in—a fundamental cryptographic method that allows computers to agree on a shared secret key while communicating over public channels.
The Challenge of Secure Communication
For two computers to communicate securely, they typically need a shared secret key. With this key, one computer can encrypt a message, send it publicly, and only the intended recipient with the same key can decrypt it. This approach, known as symmetric key cryptography, is effective but presents a critical challenge: how do the computers initially agree on a secret key without exposing it to potential eavesdroppers?
The obvious solution—one computer generating a key and sending it to the other—creates a vulnerability. If an attacker intercepts this key during transmission, they can decrypt all subsequent messages. The Diffie-Hellman key exchange method elegantly solves this problem by allowing two parties to generate a shared secret key through public communication without ever directly transmitting the key itself.
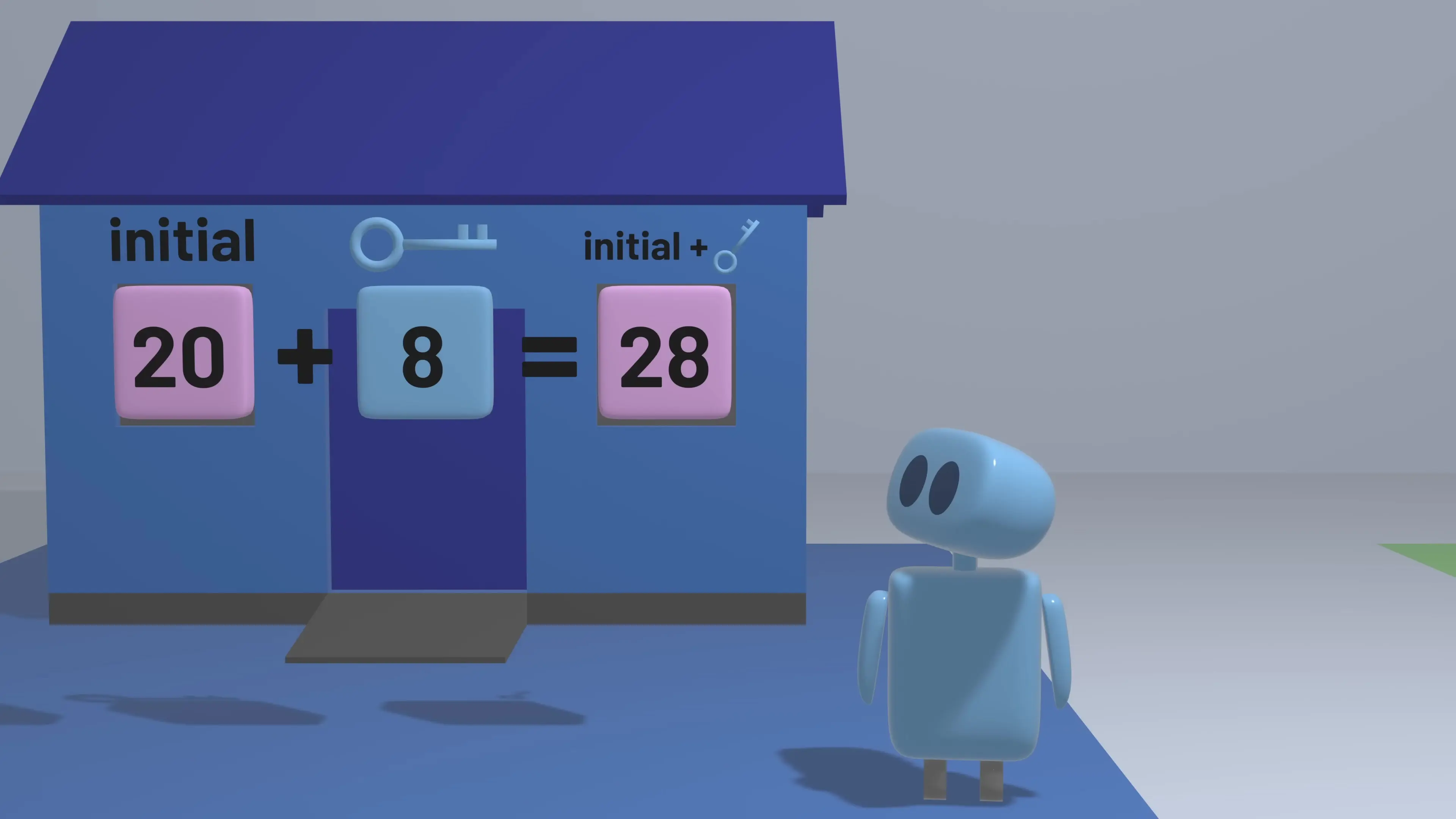
Why Simple Addition Doesn't Work for Key Exchange
To understand why the Diffie-Hellman key exchange protocol is necessary, let's first look at why a simpler approach fails. Imagine two people, Alice and Bob, who each have a secret number. They want to combine these numbers to create a shared secret, but they can only communicate over a public channel where Eve is eavesdropping.
If they start with a public number (say 5) and Alice adds her secret number (say 3) to get 8, then sends 8 to Bob, who adds his secret number (say 4) to get 12, they haven't achieved security. Eve can easily determine Alice's secret by subtracting the initial public number from what Alice sent (8 - 5 = 3). Simple addition fails because it's easily reversible.
Modular Exponentiation: The Mathematical Foundation of Diffie-Hellman
The Diffie-Hellman key exchange method uses modular exponentiation instead of addition because it's computationally difficult to reverse. Modular exponentiation involves raising a base number to a power and then taking the remainder when divided by a modulus.
For example, calculating 3^4 mod 19: First, 3^4 = 81, then 81 ÷ 19 = 4 with a remainder of 5. So 3^4 mod 19 = 5.
While calculating modular exponentiation is straightforward, the reverse operation—determining the exponent when you know the base, modulus, and result—is extremely difficult. This problem, known as the discrete logarithm problem, has no known efficient general solution, making it perfect for cryptographic applications.
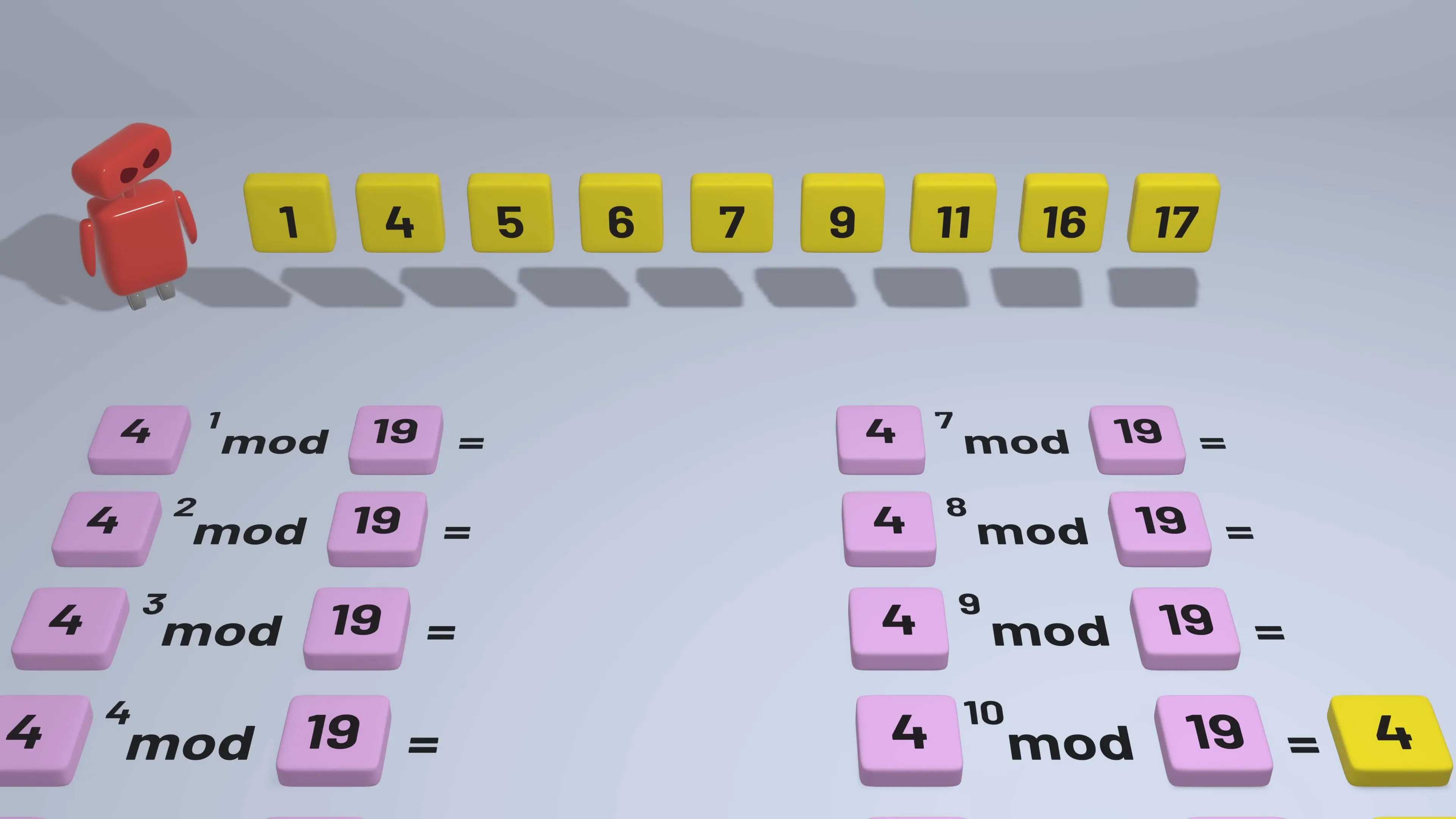
How the Diffie-Hellman Key Exchange Protocol Works: Step by Step
The Diffie-Hellman key exchange method follows these steps to establish a shared secret between two parties:
- Alice and Bob publicly agree on two numbers: a base (g) and a modulus (p). These don't need to be secret.
- Alice chooses a secret number (a) and calculates A = g^a mod p. She sends A to Bob.
- Bob chooses his own secret number (b) and calculates B = g^b mod p. He sends B to Alice.
- Alice computes the shared secret key using Bob's value: K = B^a mod p.
- Bob computes the same shared secret key using Alice's value: K = A^b mod p.
Both Alice and Bob now have the same secret key K = g^(a*b) mod p, which equals g^(b*a) mod p because multiplication is commutative. An eavesdropper who sees g, p, A, and B cannot feasibly calculate K without knowing either a or b.
# Simple Diffie-Hellman key exchange example in Python
# Publicly agreed parameters
g = 3 # base
p = 19 # modulus (should be a large prime in practice)
# Alice's side
alice_secret = 6 # Alice's private key
A = pow(g, alice_secret, p) # Alice's public key
# Bob's side
bob_secret = 15 # Bob's private key
B = pow(g, bob_secret, p) # Bob's public key
# Exchange public keys and compute shared secret
alice_shared_secret = pow(B, alice_secret, p)
bob_shared_secret = pow(A, bob_secret, p)
print(f"Alice's shared secret: {alice_shared_secret}")
print(f"Bob's shared secret: {bob_shared_secret}")
# Both should be identical
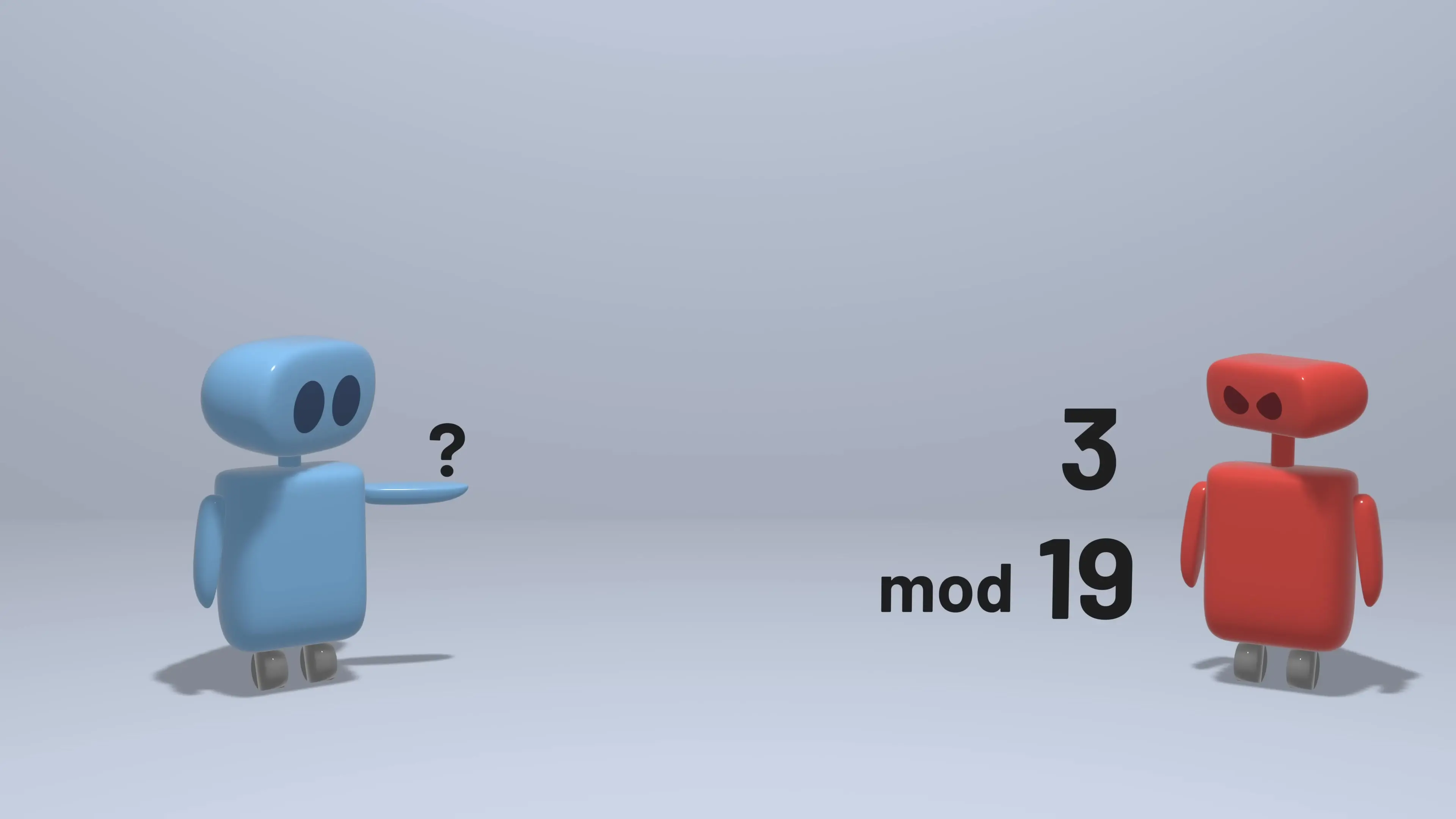
Security Requirements for Effective Diffie-Hellman Key Exchange
For the Diffie-Hellman key exchange method to be secure in practice, several requirements must be met:
- The modulus (p) should be a large prime number, ideally thousands of bits long, to prevent brute-force attacks.
- The base (g) should be chosen carefully to ensure it can generate many different possible values for the shared secret. This property is achieved when g is a primitive root modulo p.
- The private exponents should be randomly generated and kept secret.
- Additional authentication mechanisms should be implemented to prevent man-in-the-middle attacks.
Poor choices for the base can significantly weaken security. For example, if g = 1, then all modular exponentiations would result in 1, making the shared secret obvious. Similarly, if g doesn't generate all possible remainders when raised to different powers, the attacker has fewer possibilities to check when attempting to determine the shared secret.
Real-World Applications of Diffie-Hellman Key Exchange
The Diffie-Hellman key exchange protocol has become a cornerstone of modern secure communications. It's widely implemented in various security protocols and applications:
- HTTPS: When you visit secure websites, Diffie-Hellman is often used to establish the secure connection.
- Virtual Private Networks (VPNs): Many VPN solutions use Diffie-Hellman for key exchange during connection establishment.
- Secure messaging apps: Applications like Signal use Diffie-Hellman as part of their end-to-end encryption protocols.
- SSH (Secure Shell): Used for secure remote server access and management.
- TLS/SSL: The security protocols that protect most internet traffic.
Diffie-Hellman Variants and Enhancements
Since its introduction, several variants of the Diffie-Hellman key exchange method have been developed to address specific needs or improve security:
- Elliptic Curve Diffie-Hellman (ECDH): Uses elliptic curve cryptography to achieve the same security with smaller keys, making it more efficient.
- Authenticated Diffie-Hellman: Adds authentication mechanisms to prevent man-in-the-middle attacks.
- Perfect Forward Secrecy (PFS): Implementations that generate new key pairs for each session to ensure that compromising one session key doesn't compromise past or future communications.
Conclusion: The Lasting Impact of Diffie-Hellman Key Exchange
The Diffie-Hellman key exchange protocol represents one of the most important breakthroughs in modern cryptography. By solving the fundamental problem of establishing a shared secret over an insecure channel, it has enabled secure communications across the internet and countless other applications.
Despite being developed in the 1970s, the mathematical principles behind the Diffie-Hellman key exchange method remain sound, and when implemented correctly with appropriate parameters, it continues to provide strong security for digital communications. Understanding how this protocol works gives insight into the elegant mathematical foundations that make our digital world secure.
Let's Watch!
How Diffie-Hellman Key Exchange Creates Secure Communication Between Computers
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence