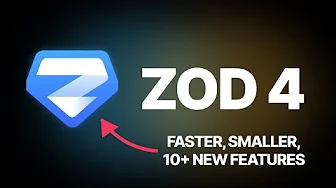
The popular schema validation library Zod has just announced its version 4 beta, bringing significant improvements and new features after three years of development since version 3.0's release in May 2021. With 25 million weekly downloads, Zod has become a cornerstone for developers ensuring API data integrity and is now gaining traction in AI applications for structured outputs.
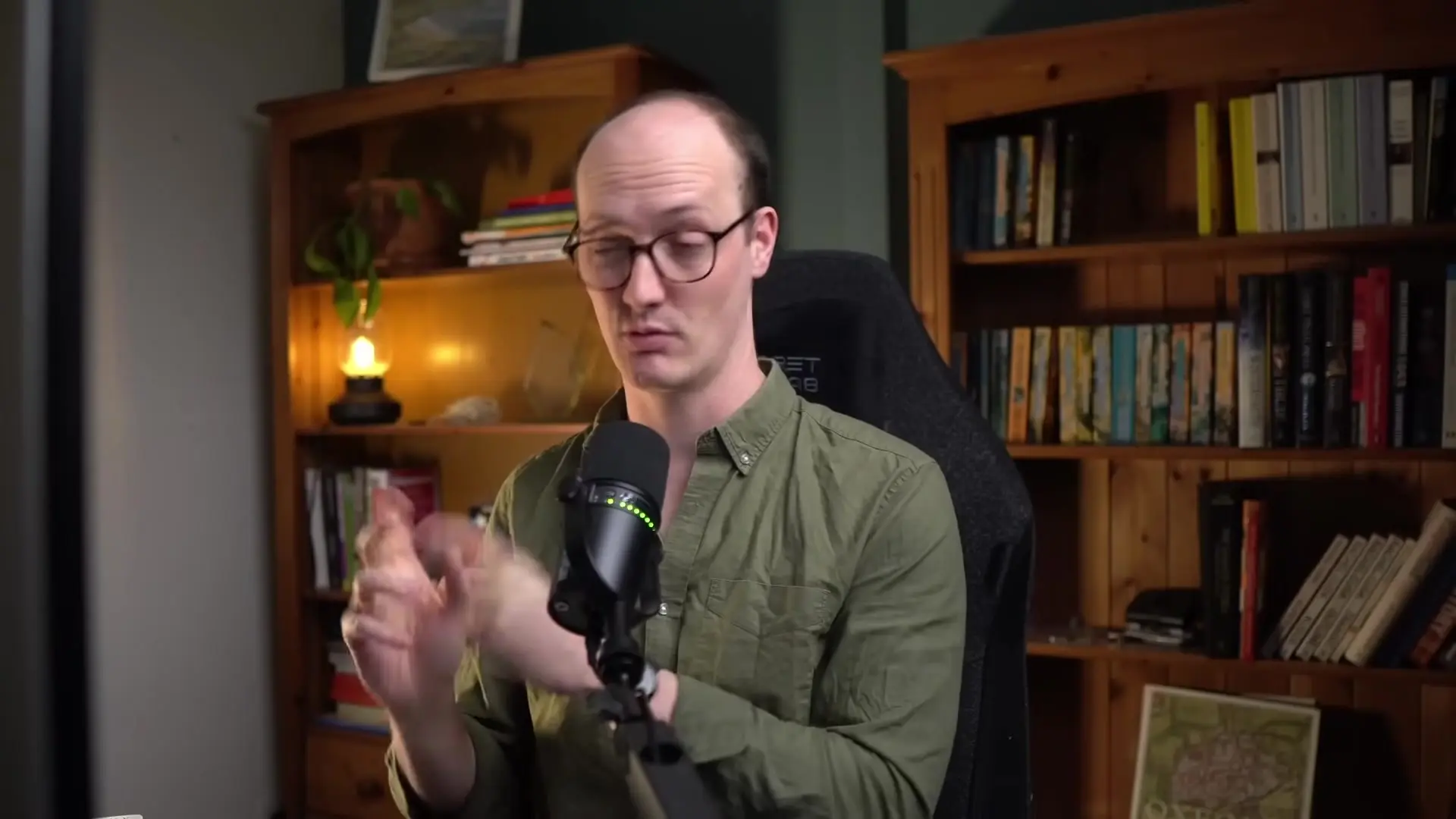
Dramatic Performance Improvements
Performance is at the forefront of Zod 4's improvements. The new version delivers impressive speed enhancements across various validation operations:
- String parsing: 2.6x faster
- Array parsing: 3x faster
- Object parsing: 7x faster
Even more significant is the leap in TypeScript performance. Previous versions of Zod and similar libraries like tRPC faced criticism for slowing down TypeScript in large codebases. Zod 4 addresses this by dramatically reducing type instantiations - from 25,000 down to about 1,000 when using features like 'extend'. This translates to faster editor responsiveness, quicker type checking, and better scalability with large schemas.
When combined with the upcoming TSGO compiler, Zod 4's editor performance will scale to significantly larger schemas and codebases, making it viable for enterprise-level applications.
Bundle Size Reduction and Zod Mini
Zod 4 brings a substantial reduction in bundle size - a 57% decrease from 12.5KB to 5.36KB gzipped for common validation scenarios. This improvement makes Zod more viable for frontend applications where bundle size is critical.
For developers requiring even smaller bundles, Zod 4 introduces Zod Mini, a sister library with a functional, tree-shakable API. While regular Zod uses methods that are harder to tree-shake, Zod Mini employs wrapper functions that allow for extreme bundle optimization.
// Regular Zod approach
z.string().min(5)
// Zod Mini approach
z.string(z.check(z.minLength(5)))
This approach feels similar to Valibot's API, which makes sense as both teams have been collaborating on standards like Standard Schema. The payoff is impressive - using Zod Mini can reduce bundle size from 12.5KB to under 2KB, making it suitable for highly bundle-constrained environments.
JSON Schema Conversion for AI Applications
One of the most exciting additions is built-in JSON schema conversion. This feature is particularly valuable for AI applications that use structured outputs and tool calling, where JSON schema is commonly used.
const mySchema = z.object({
name: z.string(),
points: z.number()
});
const jsonSchema = z.toJsonSchema(mySchema);
// Produces proper JSON schema with required fields, types, etc.
While third-party libraries previously offered this functionality, having it as a first-class feature in Zod 4 streamlines development workflows for AI-integrated applications.
New Interface API for Advanced Type Definitions
Zod 4 introduces `z.interface()`, a new API for defining object types that addresses limitations in how Zod 3 handled optionality. This new approach distinguishes between two types of optional properties:
- Key optional: The key itself is optional (may be omitted entirely)
- Value optional: The key must be present, but its value can be undefined
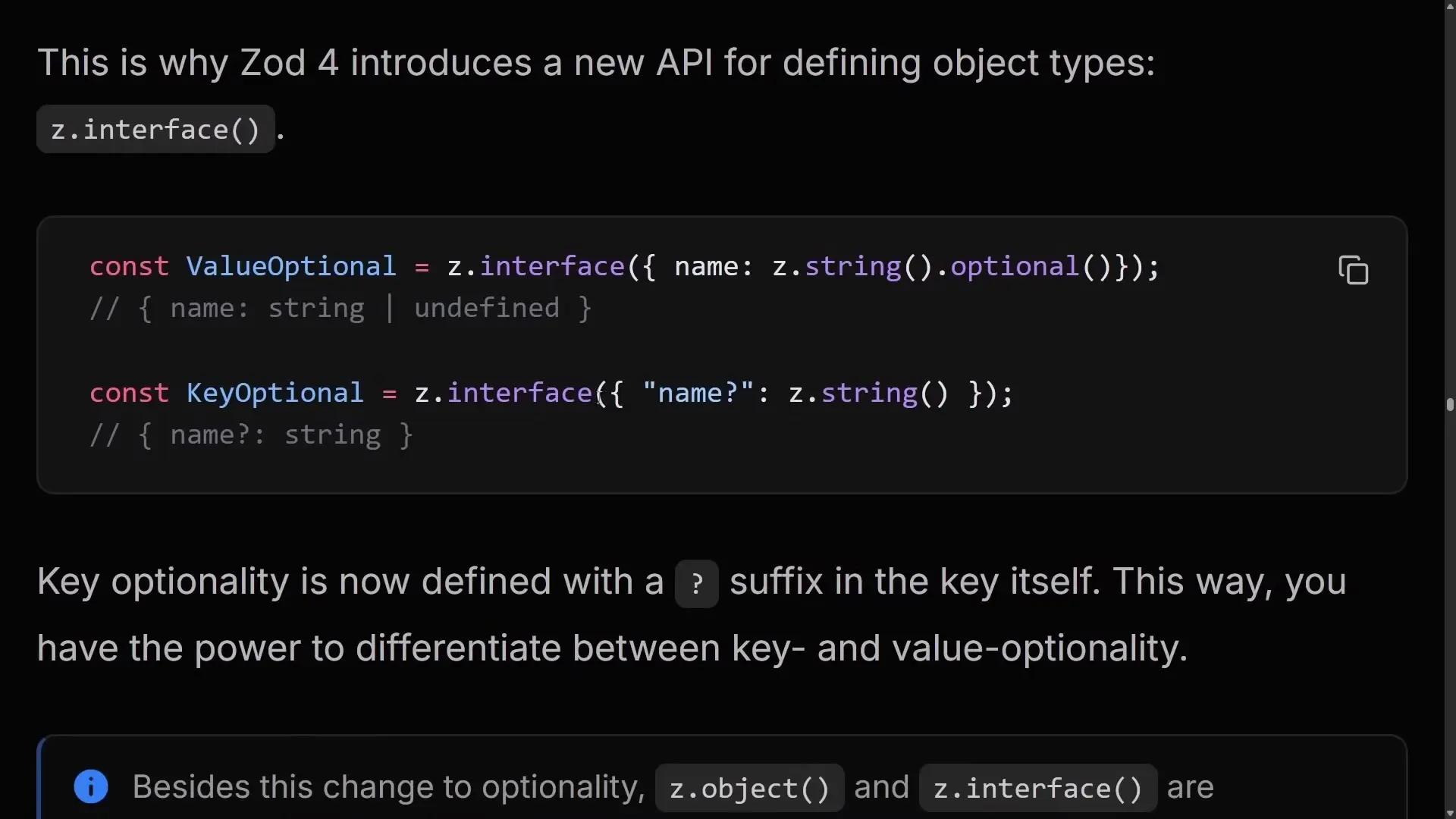
The interface API also enables true recursive types with a cleaner syntax. In Zod 3, recursive types required a complex combination of manual type annotations and `z.lazy()`. Zod 4 simplifies this with a getter-based approach:
// Zod 4 recursive type definition
const categorySchema = z.interface({
name: z.string(),
get subcategories() {
return z.array(categorySchema);
}
});
This approach eliminates the need for separate type declarations, making recursive schemas more intuitive and maintainable for complex data structures.
File Validation and Template Literals
Zod 4 adds native support for validating File instances with `z.File()`, allowing validation of file size and MIME types. This is particularly useful for form data processing and file upload scenarios.
The new version also introduces powerful template literal validations that mirror TypeScript's template literal types. This enables more precise string format validation:
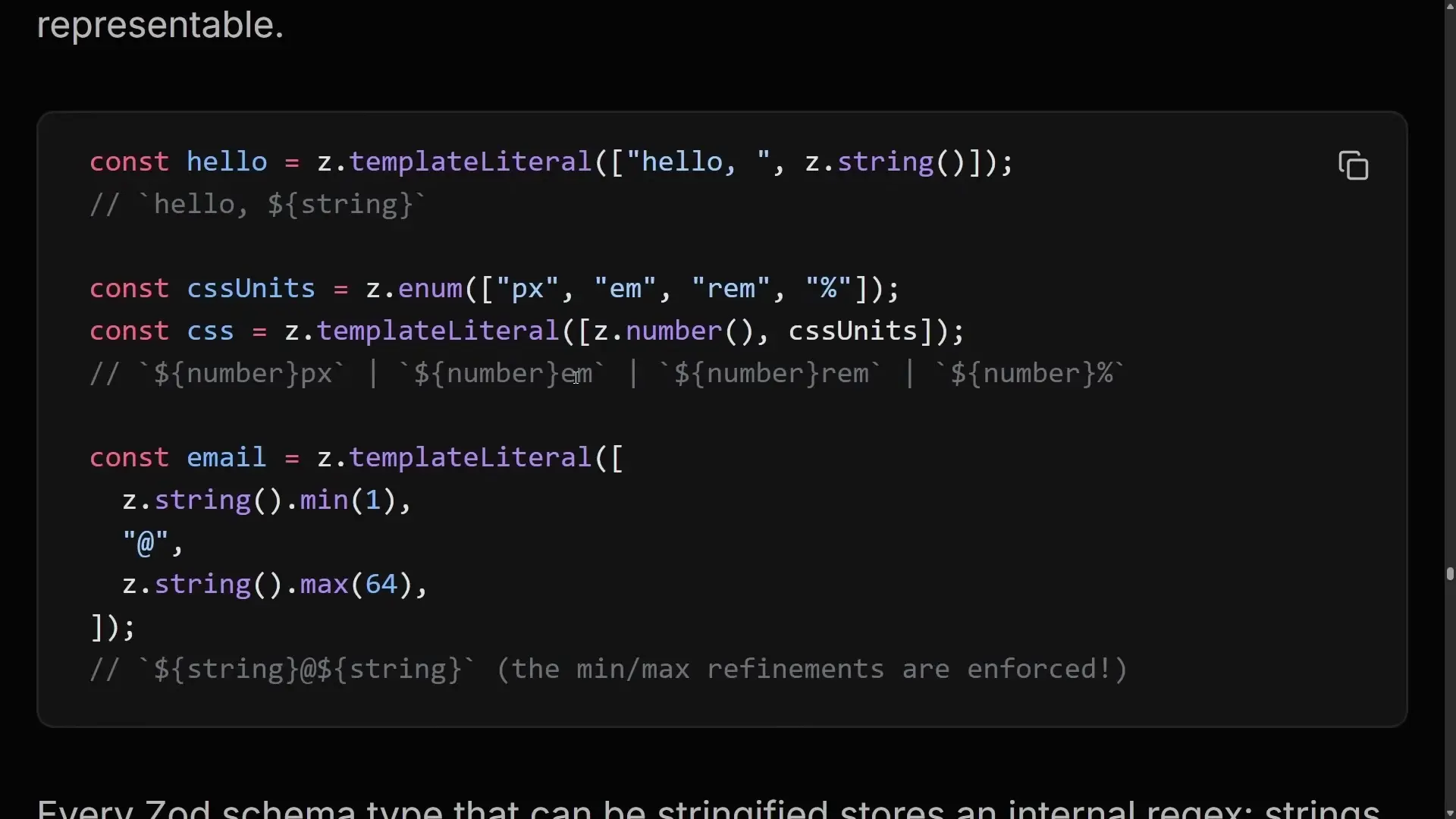
// Validate CSS units
const cssUnit = z.templateLiteral(
z.number(),
z.union([z.literal('px'), z.literal('em'), z.literal('rem')])
);
// Valid: '10px', '2.5rem'
// Invalid: '10vw', 'px10'
Improved Error Handling and Internationalization
Zod 4 introduces several quality-of-life improvements for error handling. The new `z.prettifyError()` function transforms complex validation errors into readable, multi-line strings - useful for both API responses and frontend form validations.
For internationalization, Zod 4 adds a new locale API for translating error messages. Currently, only English is available, but this foundation will likely expand to support multiple languages in future releases.
API Reorganization for Better Tree-Shaking
To further optimize bundle size, Zod 4 moves string formats (email, IP, URL, JWT, etc.) to the top level of the module, making them more tree-shakable. While method equivalents like `z.string().email()` remain available, they're deprecated and planned for removal in Zod 5.
// Deprecated approach
z.string().email()
// New approach
z.string(z.email())
This reorganization paves the way for even smaller bundle sizes in future versions while maintaining the library's powerful validation capabilities.
Practical Applications for Zod 4
Zod 4's improvements make it even more versatile for a wide range of validation scenarios:
- Backend API validation with improved performance for high-traffic services
- Frontend form validation with smaller bundle sizes through Zod Mini
- AI application development with first-class JSON schema support
- Complex data modeling with recursive types and enhanced interface definitions
- File upload validation with the new File validator
- Internationalized applications with the new locale system
Conclusion: Should You Upgrade to Zod 4?
Zod 4 represents a significant step forward for TypeScript validation, addressing key pain points around performance, bundle size, and advanced type definitions. For most projects, the performance improvements alone make upgrading worthwhile, especially for larger codebases that have experienced TypeScript slowdowns.
Frontend developers will appreciate Zod Mini's minimal footprint, while backend developers will benefit from faster validation processing. Teams working with AI applications gain valuable JSON schema tools that streamline integration with large language models.
While still in beta, Zod 4's comprehensive improvements make it a compelling upgrade for both existing Zod users and developers considering schema validation solutions for TypeScript projects.
Let's Watch!
Zod 4 Beta: Revolutionary Updates for TypeScript Validation Performance
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence