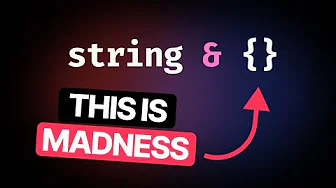
TypeScript has become an essential tool for modern web development, especially for React developers seeking type safety and improved developer experience. However, many developers only scratch the surface of TypeScript's capabilities. In this comprehensive guide, we'll explore six advanced TypeScript tips that will significantly enhance your coding skills and transform you into a true TypeScript wizard.
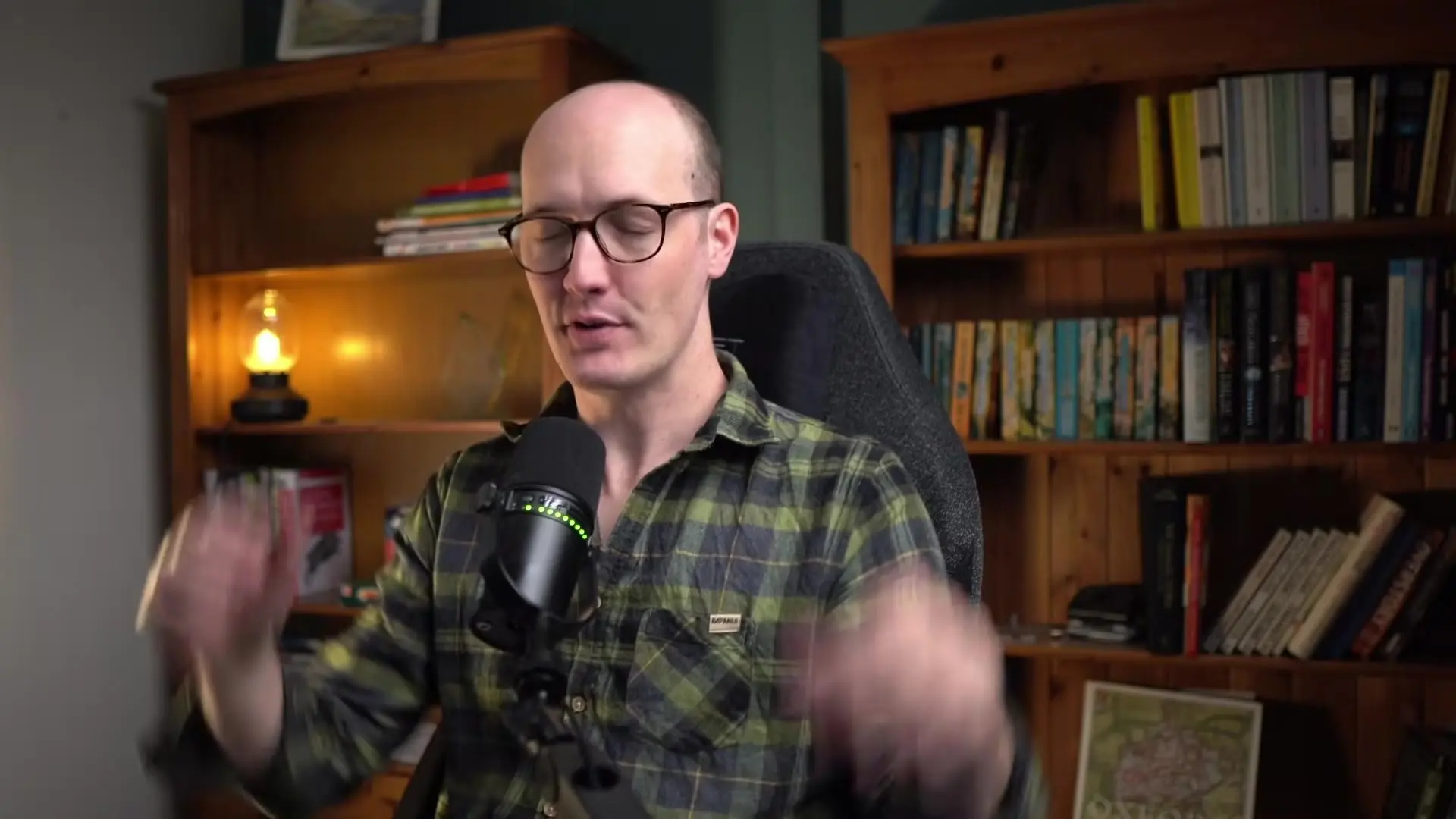
Tip 1: Understanding Key Optional vs Value Optional
One of the most crucial distinctions in TypeScript, especially when working with modern development tools, is understanding the difference between making a key optional versus making a value optional. This subtle difference can significantly impact how your code functions and how data flows through your application.
Key Optional: The Question Mark Approach
When you use the question mark after a property name in an interface or type definition, you're making the key itself optional. This means the property doesn't need to be provided at all when creating an object of that type.
interface FunctionParams {
traceId?: string; // Key optional - the property can be omitted entirely
}
function doThing(params: FunctionParams) {
// Implementation
}
// This is valid - traceId key is omitted
doThing({});
Value Optional: The Union with Undefined Approach
Alternatively, you can make a value optional by using a union type with undefined. This approach requires the property to be explicitly provided, even if its value is undefined.
interface FunctionParams {
traceId: string | undefined; // Value optional - the property must be provided, but can be undefined
}
function doThing(params: FunctionParams) {
// Implementation
}
// This is valid - traceId is explicitly provided as undefined
doThing({ traceId: undefined });
// This is NOT valid - traceId must be provided
// doThing({});
This distinction becomes particularly important when you're building functions that pass data through a chain of other function calls. Value optional properties ensure that even if a value might be undefined, it must be explicitly passed along, which can prevent accidental data loss in telemetry, logging, or other critical systems.
Tip 2: Mastering TypeScript's Essential Utility Types
TypeScript provides several built-in utility types that can dramatically simplify your type manipulations. Let's explore four of the most useful ones: Pick, Omit, Exclude, and Extract.
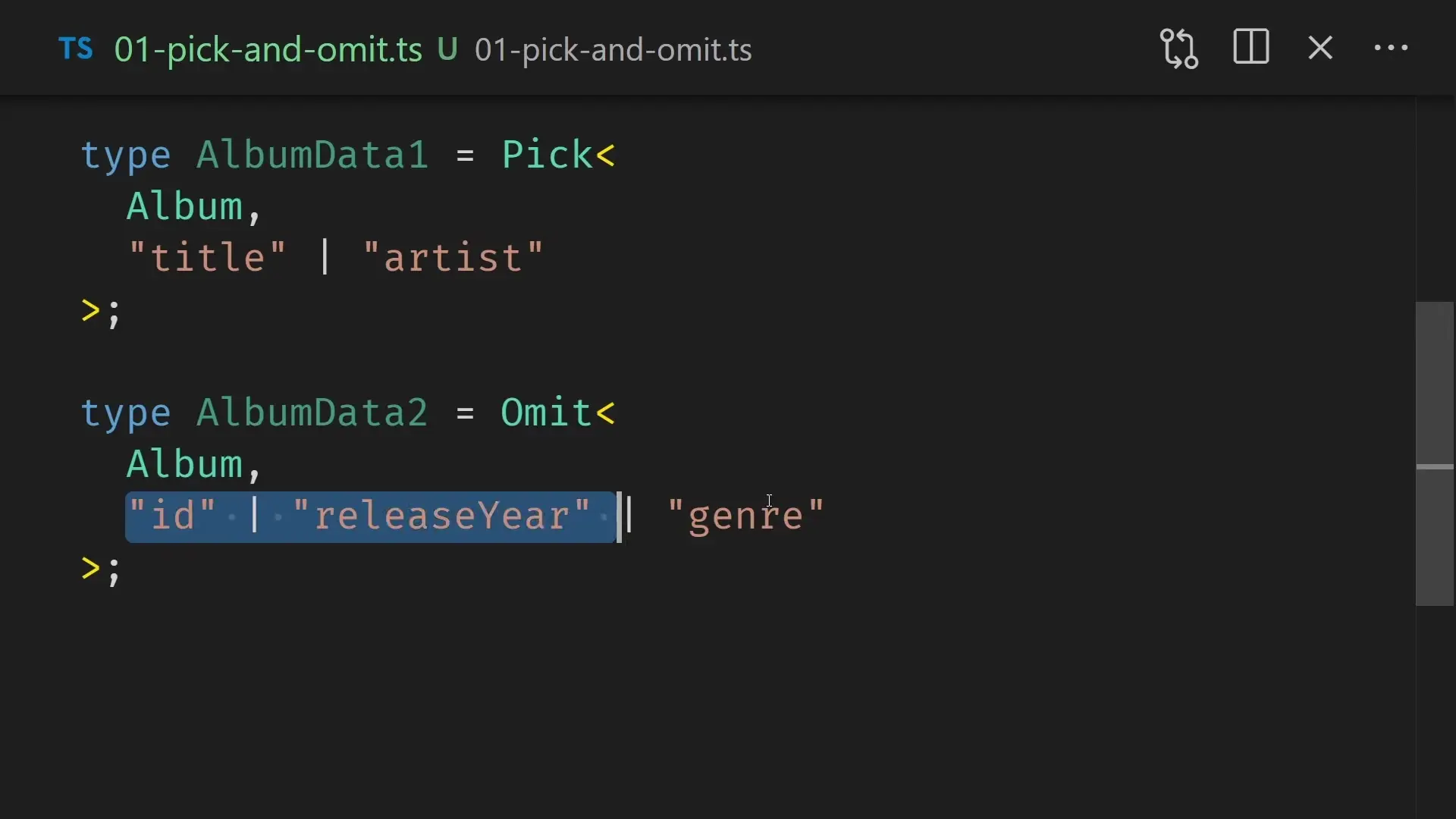
Pick and Omit: Working with Object Types
Pick and Omit are utility types that work with object shapes, allowing you to create new types by either including or excluding specific properties from an existing type.
interface Album {
id: number;
title: string;
artist: string;
releaseYear: number;
genre: string;
}
// Using Pick to create a type with only title and artist
type AlbumBasics = Pick<Album, 'title' | 'artist'>;
// Result: { title: string; artist: string; }
// Using Omit to create a type without id, releaseYear, and genre
type AlbumDisplayData = Omit<Album, 'id' | 'releaseYear' | 'genre'>;
// Result: { title: string; artist: string; }
Exclude and Extract: Working with Union Types
While Pick and Omit work with object properties, Exclude and Extract operate on union types, allowing you to filter union members based on certain criteria.
// Define a discriminated union for album states
type AlbumState =
| { state: 'released'; releaseDate: string }
| { state: 'recording'; studio: string }
| { state: 'mixing'; engineer: string };
// Using Exclude to remove the 'released' state
type NotReleasedState = Exclude<AlbumState, { state: 'released' }>;
// Result: { state: 'recording'; studio: string } | { state: 'mixing'; engineer: string }
// Working with simple unions
type MixedTypes = 'A' | 'B' | 'C' | 1 | 2;
// Extract only string types
type StringTypes = Extract<MixedTypes, string>;
// Result: 'A' | 'B' | 'C'
// Exclude string types
type NumberTypes = Exclude<MixedTypes, string>;
// Result: 1 | 2
Understanding these utility types and when to use each one will significantly improve your ability to manipulate and transform types in TypeScript, leading to more concise and maintainable code.
Tip 3: Using the Prettify Type Helper for Complex Types
When working with complex types that involve multiple intersections, type helpers, or utility types, the resulting type can become difficult to read and understand. This is where the Prettify type helper comes in handy.
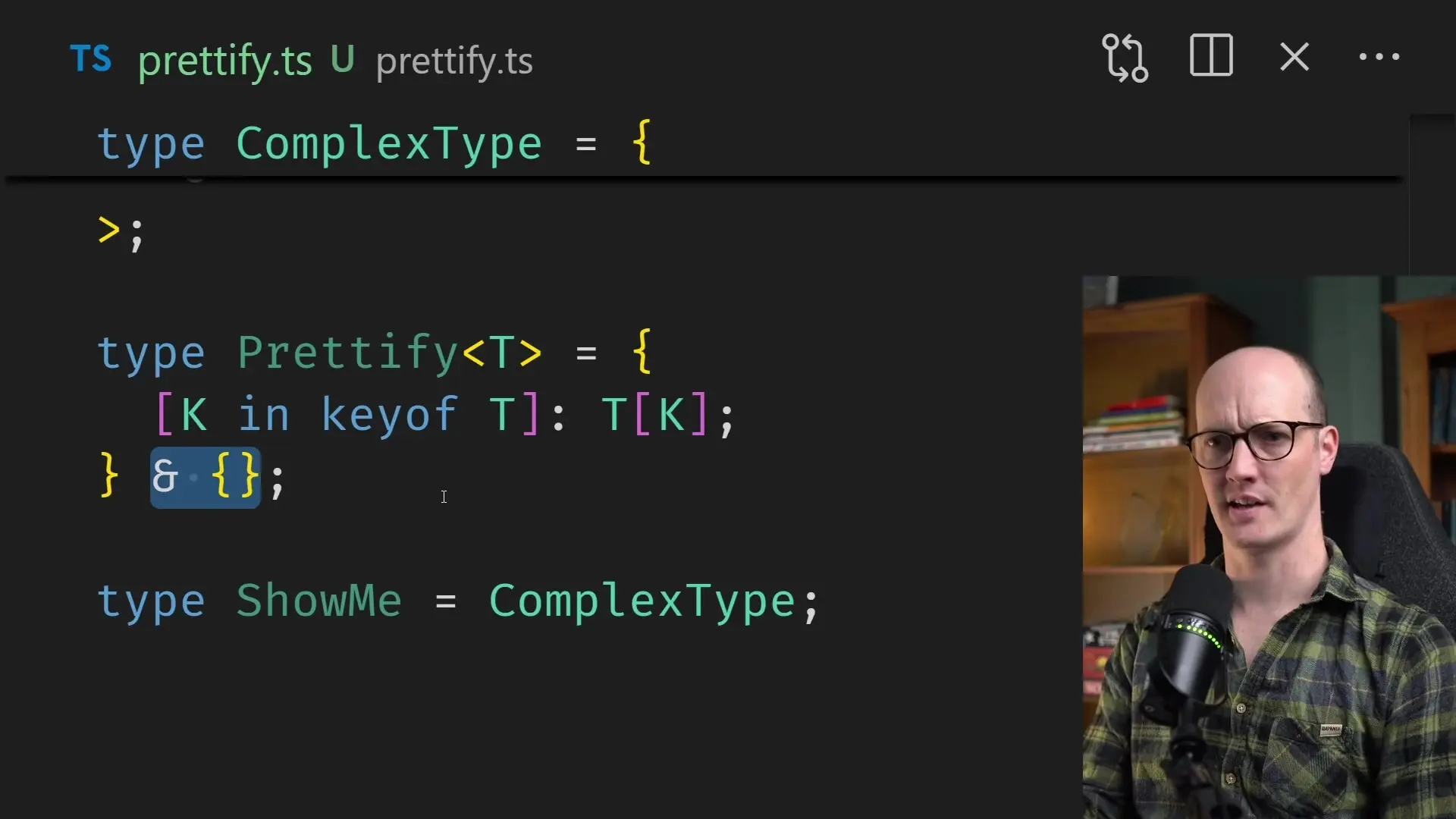
The Prettify type helper is not built into TypeScript, but it's a powerful utility you can add to your projects. It takes a complex type and transforms it into a more readable, simplified representation.
// Define the Prettify type helper
type Prettify<T> = {
[K in keyof T]: T[K];
} & {};
// Example of a complex type
type ComplexType = {
name: string;
age: number;
} & {
address: string;
city: string;
} & Omit<{ id: number; createdAt: Date }, 'createdAt'>;
// Without Prettify, hovering over this type shows the complex intersection
type UglyType = ComplexType;
// With Prettify, the type is simplified to a clean object type
type PrettyType = Prettify<ComplexType>;
// Result: {
// name: string;
// age: number;
// address: string;
// city: string;
// id: number;
// }
The Prettify type helper is especially useful in library development, where you want to provide clean, readable types to your users instead of exposing the complex type machinery that powers your library internally.
Tip 4: Implementing Precise Type Guards
Type guards are functions that help TypeScript narrow down the type of a variable within a conditional block. While basic type guards are straightforward, implementing precise type guards for complex types can significantly improve type safety in your applications.
// Define a discriminated union type
type Result<T> =
| { status: 'success'; data: T }
| { status: 'error'; error: Error };
// Implement a precise type guard
function isSuccess<T>(result: Result<T>): result is { status: 'success'; data: T } {
return result.status === 'success';
}
// Usage in a function
function handleResult<T>(result: Result<T>) {
if (isSuccess(result)) {
// TypeScript knows that result.data exists here
console.log('Success:', result.data);
} else {
// TypeScript knows that result.error exists here
console.error('Error:', result.error.message);
}
}
By creating precise type guards, you can write more type-safe code that handles different cases correctly, with full TypeScript support to prevent errors.
Tip 5: Leveraging Mapped Types for Dynamic Type Creation
Mapped types allow you to create new types by transforming the properties of existing types. This is particularly useful when you need to create variations of existing types systematically.
// Original type
interface User {
id: number;
name: string;
email: string;
password: string;
}
// Make all properties optional
type PartialUser = Partial<User>;
// Make all properties readonly
type ReadonlyUser = Readonly<User>;
// Create a custom mapped type that makes all properties nullable
type Nullable<T> = { [K in keyof T]: T[K] | null };
type NullableUser = Nullable<User>;
// Create a type with only the public fields (excluding password)
type PublicUser = Omit<User, 'password'>;
// Create a type for updating a user (all fields optional except id)
type UpdateUser = Pick<User, 'id'> & Partial<Omit<User, 'id'>>;
Mapped types are incredibly powerful for creating consistent type transformations across your codebase, reducing duplication and ensuring type safety.
Tip 6: Using Template Literal Types for String Manipulation
Template literal types, introduced in TypeScript 4.1, allow you to manipulate string types in ways that were previously impossible. They're particularly useful for creating types based on string patterns.
// Define basic types
type HttpMethod = 'GET' | 'POST' | 'PUT' | 'DELETE';
type Resource = 'users' | 'posts' | 'comments';
// Create endpoint types using template literals
type Endpoint = `/${Resource}`;
// Result: '/users' | '/posts' | '/comments'
// Create more complex endpoint patterns
type ResourceEndpoint = `/${Resource}/:id`;
// Result: '/users/:id' | '/posts/:id' | '/comments/:id'
// Create HTTP method + endpoint combinations
type HttpEndpoint = `${HttpMethod} ${Endpoint}`;
// Result: 'GET /users' | 'GET /posts' | 'GET /comments' | 'POST /users' | ... etc.
// Practical example: API function with strongly typed endpoints
function fetchApi<T extends HttpEndpoint>(endpoint: T, options?: RequestInit): Promise<unknown> {
const [method, path] = endpoint.split(' ');
return fetch(path, { method, ...options }).then(res => res.json());
}
// Usage with complete type safety
fetchApi('GET /users'); // Valid
fetchApi('PUT /posts/:id'); // Valid
// fetchApi('PATCH /users'); // Error: 'PATCH /users' is not assignable to parameter of type HttpEndpoint
Template literal types enable you to create powerful, flexible type systems that capture string patterns and relationships, which is particularly valuable for API definitions, route handling, and event systems.
Conclusion: Becoming a TypeScript Wizard
Mastering these advanced TypeScript tips will significantly elevate your development skills, especially if you work with React or other modern frameworks. By understanding key vs. value optionality, leveraging utility types, using the Prettify helper, implementing precise type guards, creating mapped types, and utilizing template literal types, you'll be able to create more robust, maintainable, and type-safe applications.
Remember that becoming a TypeScript wizard isn't just about knowing these techniques—it's about understanding when and how to apply them to solve real-world problems. Start incorporating these advanced patterns into your codebase gradually, and you'll soon see the benefits in code quality, developer experience, and reduced runtime errors.
- Use key optional (?) when you want to make your API cleaner and more flexible
- Use value optional (| undefined) when you need to ensure values are explicitly passed through a chain of functions
- Leverage utility types like Pick, Omit, Exclude, and Extract to transform types without duplication
- Apply the Prettify type helper to make complex types more readable
- Create precise type guards to enable TypeScript to narrow types correctly in conditional blocks
- Use mapped types and template literal types for advanced type transformations
By applying these advanced TypeScript techniques in your React projects and other TypeScript codebases, you'll write more robust code with fewer bugs and better developer experience. Happy coding!
Let's Watch!
6 Advanced TypeScript Tips to Transform You Into a Code Wizard
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence