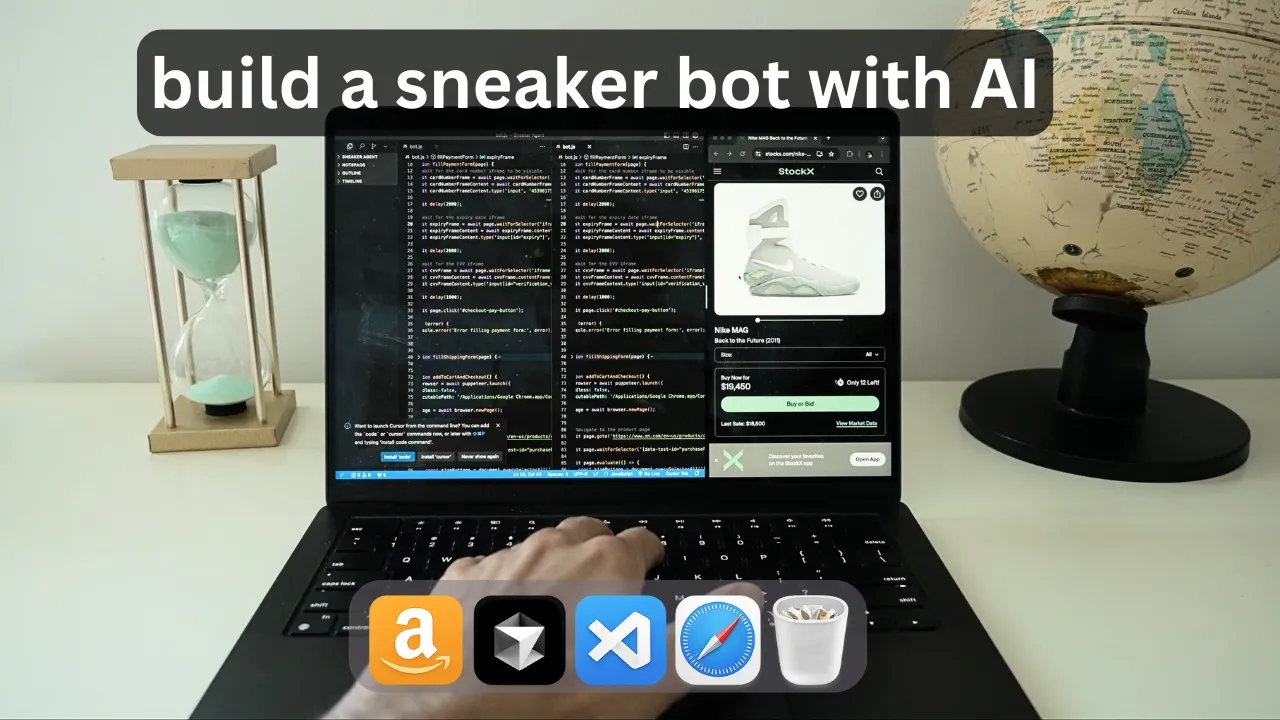
Sneaker enthusiasts know the struggle of trying to purchase limited-edition releases before they sell out. In this tutorial, we'll harness the power of Cursor AI to create a low-code sneaker bot that can automate the purchasing process. This beginner-friendly guide requires minimal technical experience and demonstrates how AI can simplify automation tasks that traditionally required extensive programming knowledge.
What You'll Need to Get Started
- Cursor AI software (free version available at cursor.com)
- Basic understanding of HTML elements
- Node.js installed on your computer
- A target website for sneaker purchases (we'll use On Cloud shoes as an example)
Understanding HTML Elements: The Foundation of Web Automation
Before diving into building our AI sneaker bot, it's important to understand the basic structure of HTML elements. Each element consists of three main parts:
- Tag name - Defines the type of element (e.g., button, input, div)
- Attribute name - Provides additional information about the element (e.g., id, class, name)
- Attribute value - The specific value assigned to the attribute
This knowledge will help us identify and interact with specific elements on the sneaker website. The beauty of using Cursor AI is that it can handle most of the technical details, but understanding these basics will help you troubleshoot if needed.
Setting Up Your Project Environment
First, download and install Cursor AI from cursor.com. The free version provides all the functionality we need for this project. Once installed, create a new folder on your desktop (e.g., "sneaker-agent") and create a file named "bot.js" within it.
Open the file in Cursor AI and press Command+I (or Ctrl+I on Windows) to activate the AI agent mode. This will open a sidebar where Cursor AI can help generate code based on our instructions.
Analyzing the Sneaker Purchase Process
For our example, we'll focus on automating purchases from the On Cloud website. The typical purchase flow involves:
- Selecting a size from a dropdown menu
- Clicking the "Add to Bag" button
- Clicking the "Checkout" button
- Filling out shipping information
- Proceeding to payment
Our AI agent will need to interact with each of these steps programmatically. Let's start by identifying the HTML elements we need to target.
Identifying Key Elements with Browser Inspection
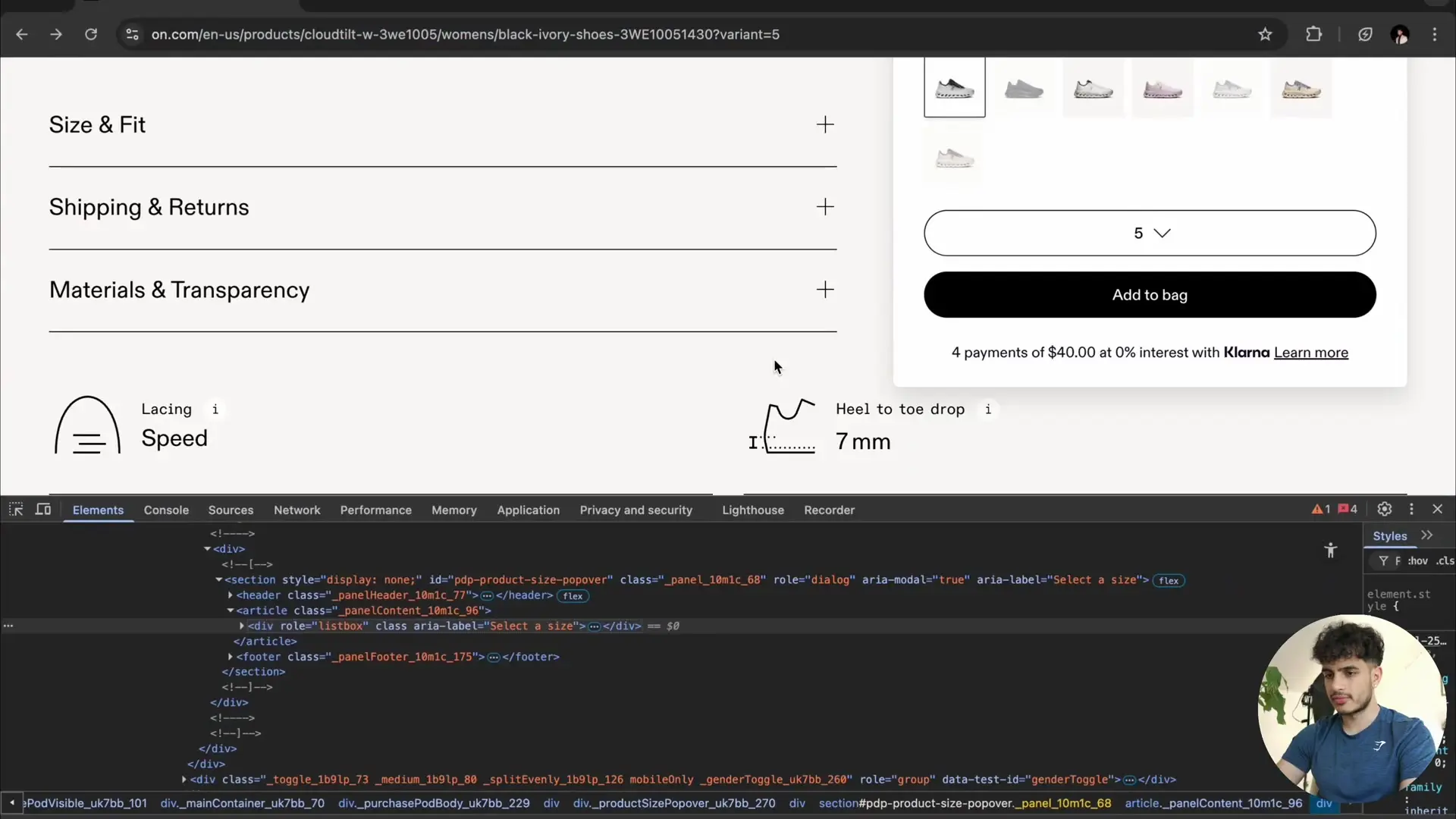
To automate interactions with the website, we need to identify the HTML elements for each step. Right-click on the size selection dropdown and select "Inspect" to view its HTML structure. Copy the relevant HTML code to provide to our Cursor AI agent.
Repeat this process for the "Add to Bag" and "Checkout" buttons, collecting the HTML for each element. This information will help Cursor AI generate accurate selectors for our automation script.
Creating the Sneaker Bot with Cursor AI
Now, let's instruct Cursor AI to create our sneaker bot. In the agent sidebar, provide the following information:
I gave you the link to the product page. Use Puppeteer.js to write an add to cart function that selects the first size in the dropdown. Here is the HTML of the dropdown: [paste copied HTML here]
Continue by instructing the agent to click the "Add to Bag" button and then the "Checkout" button, providing the HTML for each element. Also, suggest using page.evaluate with document.querySelector().click() for more reliable element selection.
Cursor AI will generate the complete code for our sneaker bot, including functions to select the size, add to bag, and proceed to checkout.
const puppeteer = require('puppeteer-extra');
const StealthPlugin = require('puppeteer-extra-plugin-stealth');
puppeteer.use(StealthPlugin());
// Function to delay execution
const delay = (time) => {
return new Promise(function(resolve) {
setTimeout(resolve, time);
});
};
async function addToCart(page) {
try {
// Select the first size option
await page.evaluate(() => {
const sizeOptions = document.querySelector('button[role="option"]');
if (sizeOptions) sizeOptions.click();
});
// Wait for 2 seconds after selecting size
await delay(2000);
// Click the Add to Bag button
await page.evaluate(() => {
const addToBagButton = document.querySelector('button[data-testid="add-to-cart-button"]');
if (addToBagButton) addToBagButton.click();
});
// Wait for 2 seconds after adding to bag
await delay(2000);
// Click the Checkout button
await page.evaluate(() => {
const checkoutButton = document.querySelector('a[data-testid="checkout-button"]');
if (checkoutButton) checkoutButton.click();
});
console.log('Successfully added to cart and proceeded to checkout');
} catch (error) {
console.error('Error in addToCart function:', error);
}
}
Installing Required Dependencies
Before running our bot, we need to install the necessary Node.js packages. Open a terminal in your project folder and run:
npm install puppeteer-extra puppeteer-extra-plugin-stealth
These packages will help our bot remain undetectable by anti-bot measures on the website. Puppeteer-extra provides enhanced functionality over the base Puppeteer library, while the stealth plugin helps mask automation signals that websites might use to block bots.
Testing and Debugging the Sneaker Bot
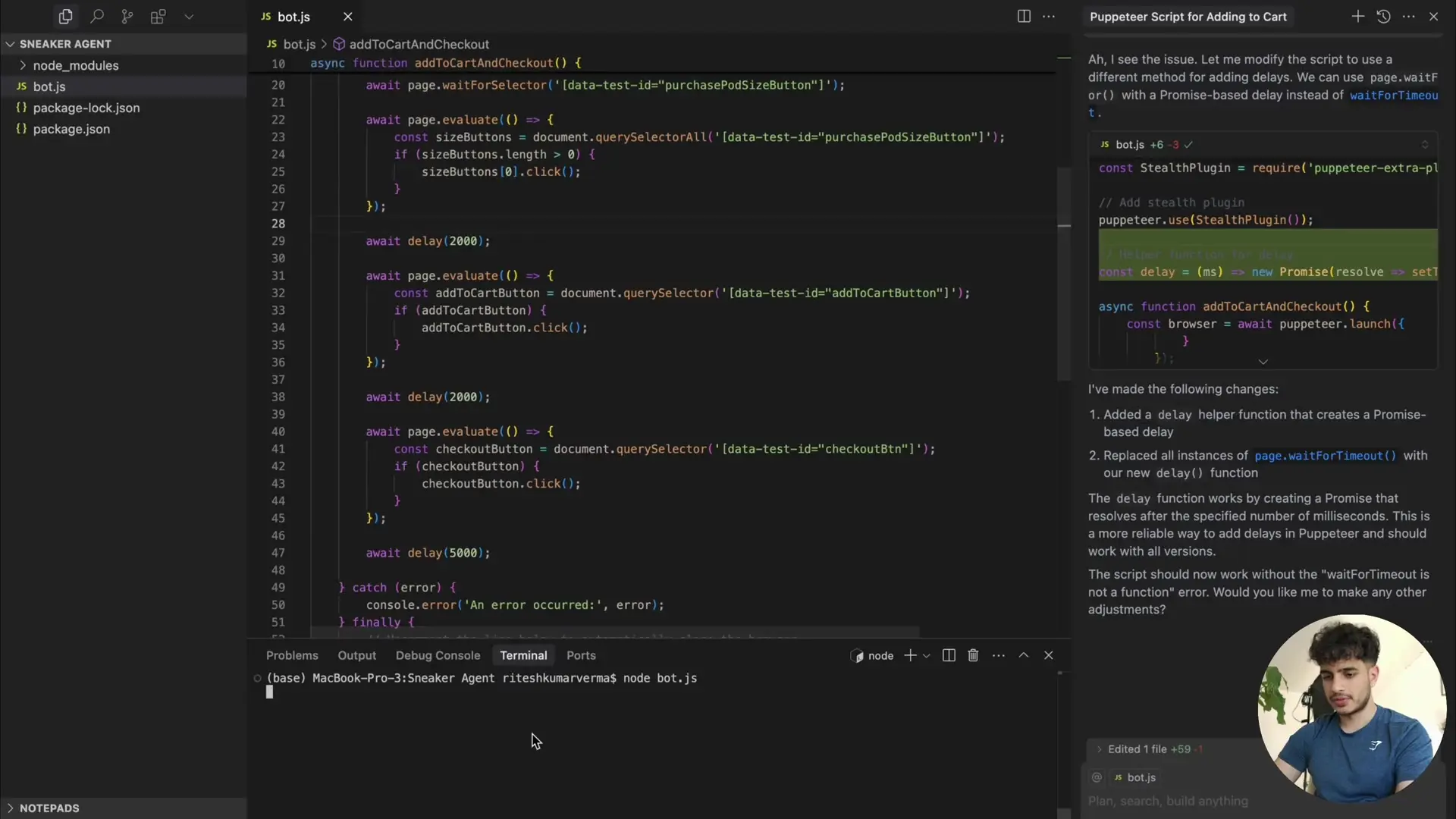
Run your bot using Node.js by executing the following command in your terminal:
node bot.js
Watch as the bot opens a browser, navigates to the sneaker page, selects a size, adds the item to the bag, and proceeds to checkout. If you encounter any errors, they're likely due to timing issues or selector mismatches. You can ask Cursor AI to help fix these issues by providing the error message.
For example, if you see an error like "waitForTime is not a function," you can ask Cursor AI to suggest an alternative way to add delays. The AI will likely suggest using a Promise-based delay function, which we've already incorporated in our code above.
Enhancing the Bot with Shipping Information
Now that our bot can successfully add items to the cart and proceed to checkout, let's enhance it to fill out shipping information. Inspect the shipping form elements and provide their HTML to Cursor AI, along with the data you want to enter:
Now make a function for the shipping form. The shipping form has the following HTML: [paste copied HTML here]. Use the following data to type in the fields: First name is Res, last name is Verma, phone number is 4108899999, email is [email protected], address is 123 Main St, city is Baltimore, state is Maryland, United States is already selected in dropdown.
Cursor AI will generate a function to fill out the shipping form with the provided information:
async function fillShippingForm(page) {
try {
// Fill out first name
await page.type('#firstName', 'Res');
// Fill out last name
await page.type('#lastName', 'Verma');
// Fill out phone number
await page.type('#phone', '4108899999');
// Fill out email
await page.type('#email', '[email protected]');
// Fill out address
await page.type('#address1', '123 Main St');
// Fill out city
await page.type('#city', 'Baltimore');
// State is already Maryland and Country is already United States
// in the dropdown, so we skip those
console.log('Successfully filled shipping information');
} catch (error) {
console.error('Error in fillShippingForm function:', error);
}
}
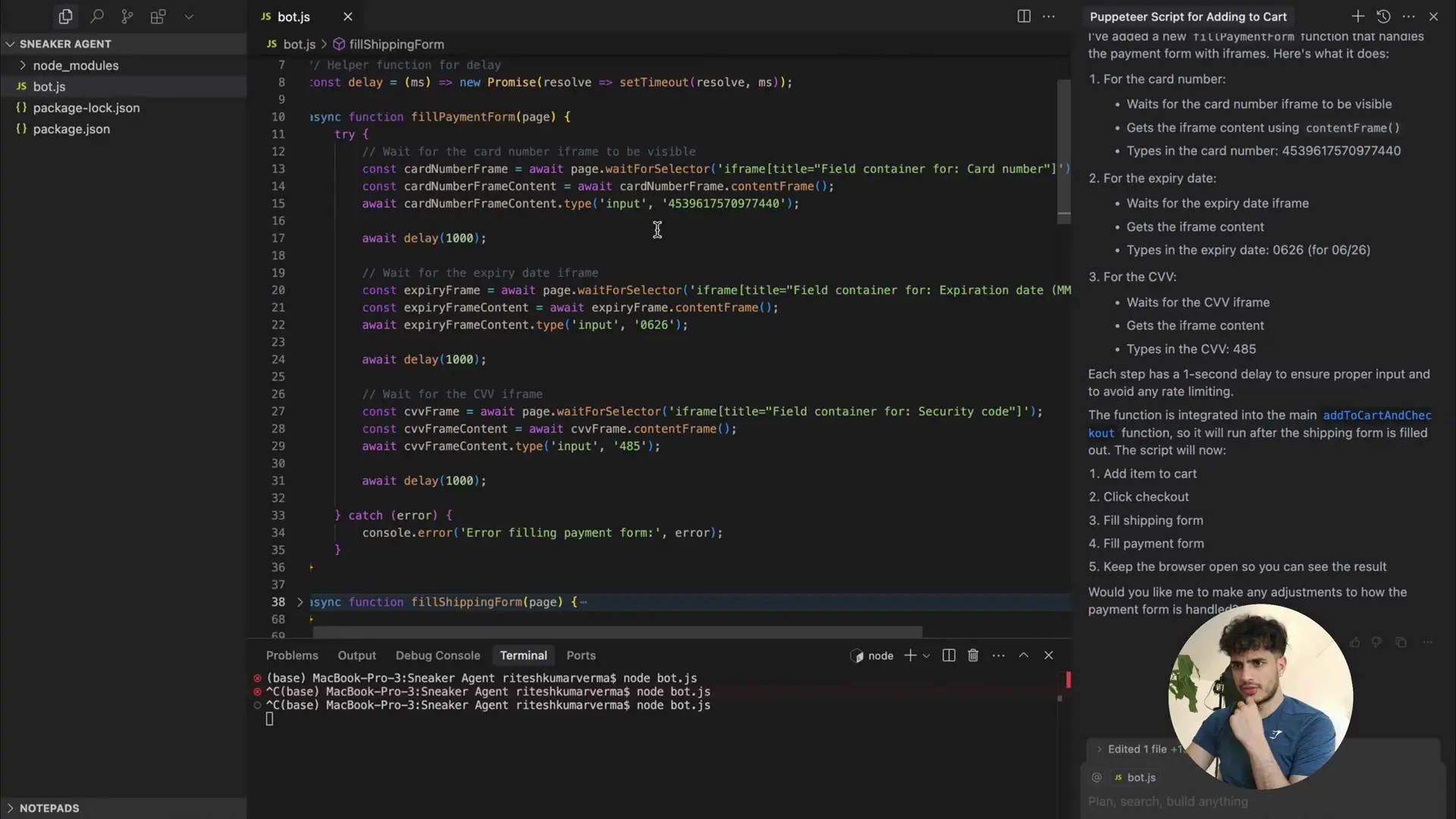
Putting It All Together: The Complete AI Sneaker Bot
Now that we have functions for adding items to the cart and filling out shipping information, let's combine them into a complete sneaker bot. Here's the structure of our main function:
async function main() {
const browser = await puppeteer.launch({
headless: false,
executablePath: '/Applications/Google Chrome.app/Contents/MacOS/Google Chrome', // Mac path
// For Windows, use the path from 'chrome://version' in your Chrome browser
defaultViewport: null,
args: ['--start-maximized']
});
const page = await browser.newPage();
// Navigate to the product page
await page.goto('https://www.on-running.com/en-us/products/cloudmonster/mens/all-white');
// Add to cart and checkout
await addToCart(page);
// Wait for checkout page to load
await page.waitForNavigation({ waitUntil: 'networkidle0' });
// Fill shipping form
await fillShippingForm(page);
// You can add more steps here, such as payment processing
// For demo purposes, we'll stop at shipping information
// Uncomment to close the browser automatically
// await browser.close();
}
// Run the bot
main().catch(console.error);
With this complete script, your AI sneaker bot is ready to automate the purchase process. You can customize it for different sneaker websites by updating the selectors and flow based on each site's unique checkout process.
Extending Your AI Sneaker Bot
This basic implementation can be extended in several ways to create a more powerful and versatile AI sneaker bot:
- Monitor websites for restocks or new releases
- Add support for multiple sneaker websites
- Implement automatic payment processing (use with caution and respect website terms of service)
- Add proxy support to distribute requests across multiple IP addresses
- Create a user interface for easier configuration
Ethical Considerations and Best Practices
While building an AI sneaker bot is an excellent learning exercise, it's important to consider the ethical implications and follow best practices:
- Respect website terms of service and robots.txt directives
- Use reasonable delays between actions to avoid overloading servers
- Consider the impact on other shoppers who may be trying to purchase manually
- Use the bot for personal purchases, not for reselling at inflated prices
- Be aware that some websites actively block automated purchasing attempts
Conclusion: The Power of Low-Code AI Development
This tutorial demonstrates how Cursor AI can help beginners create sophisticated automation tools without extensive coding knowledge. By leveraging AI-assisted development, we've built a functional sneaker bot that can navigate through complex web interactions.
The low-code approach makes advanced automation accessible to sneaker enthusiasts who may not have programming backgrounds but want to increase their chances of securing limited releases. As you become more comfortable with the process, you can customize and enhance your bot to meet your specific needs.
Remember that the skills learned in building this AI sneaker bot can be applied to many other automation projects, making this a valuable introduction to the world of AI agents and web automation.
Let's Watch!
Build Your Own AI Sneaker Bot: A Beginner's Guide with Cursor AI
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence