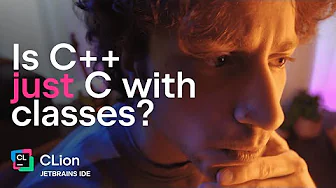
The programming world offers a variety of languages, each with their own strengths and purposes. Among the most commonly confused are C, C++, and C#. While their names suggest a close relationship, these languages have significant differences in design philosophy, features, and use cases. This comprehensive guide explores the key distinctions between these languages to help you choose the right tool for your development needs.
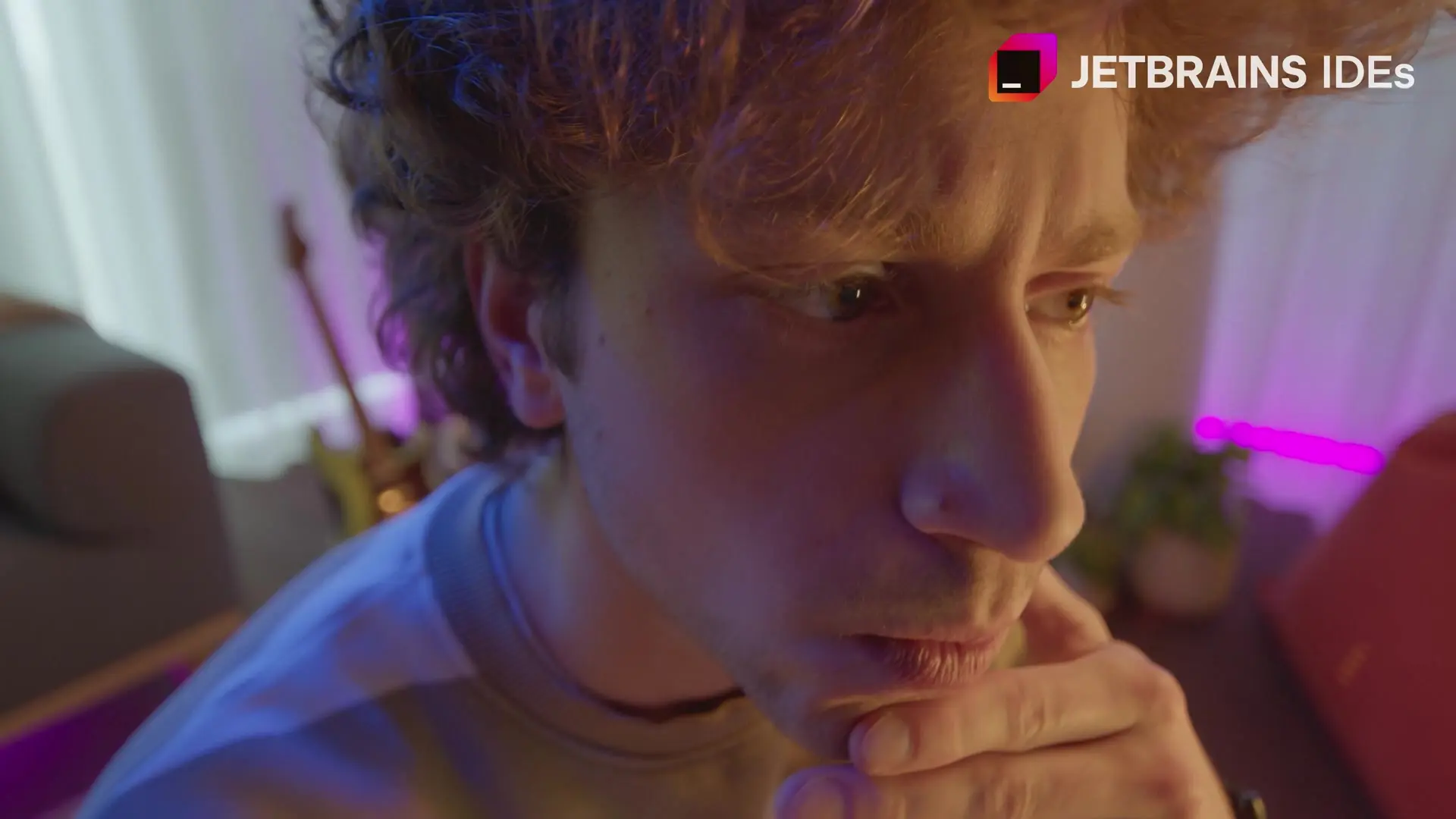
Is C++ Just "C with Classes"?
One of the most common misconceptions is that C++ is simply "C with classes." While C++ did evolve from C and maintains backward compatibility with most C code, it has grown into a much more sophisticated language with numerous features that go far beyond its predecessor.
C++ introduced object-oriented programming to the C language family, but it also implements functional programming paradigms, generic programming through templates, and numerous modern features that make it a multi-paradigm language. CLion, JetBrains' IDE for C and C++ development, helps developers navigate these advanced features with intelligent code assistance.
Core Differences Between C and C++
- C is a procedural language focused on functions, while C++ supports multiple programming paradigms including procedural, object-oriented, and generic programming
- C++ supports classes and objects, enabling encapsulation, inheritance, and polymorphism
- C++ includes exception handling mechanisms not available in C
- C++ offers Standard Template Library (STL) with reusable containers, algorithms, and iterators
- C++ has stronger type checking and function overloading capabilities
- C++ supports references in addition to pointers, providing safer alternatives in many cases
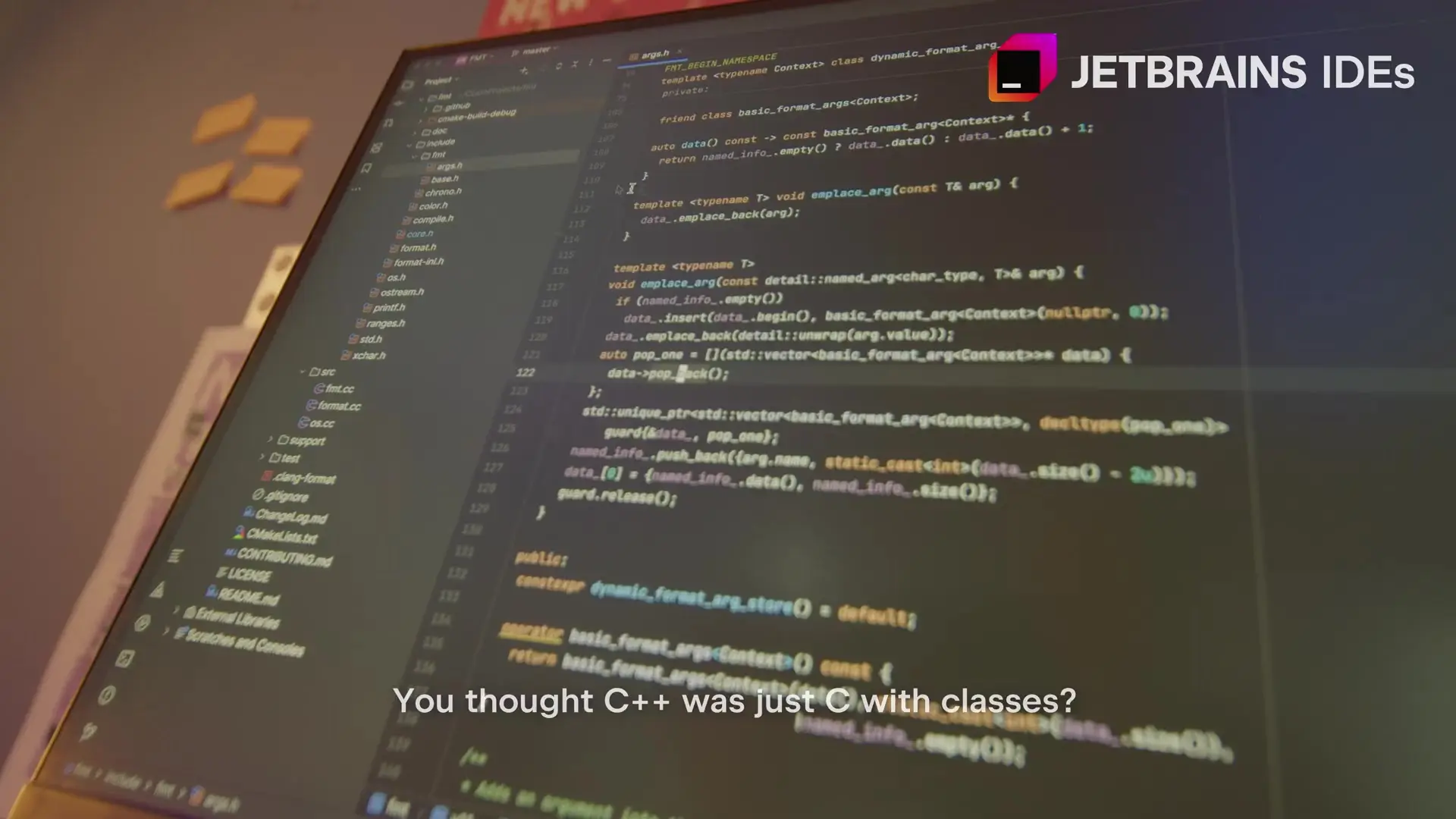
// C++ template example - not possible in C
template <typename T>
class Vector {
private:
T* elements;
size_t capacity;
size_t size;
public:
Vector() : elements(nullptr), capacity(0), size(0) {}
void push_back(const T& element) {
// Implementation details
}
T& operator[](size_t index) {
return elements[index];
}
};
Understanding C#: Not Just Another C Variant
C# (C-sharp) is often grouped with C and C++ due to naming similarity, but it's actually quite different. Developed by Microsoft as part of the .NET initiative, C# is more closely related to Java in its design philosophy than to C or C++.
- C# is a high-level, managed language that runs on the .NET framework
- Memory management in C# is handled through automatic garbage collection, unlike C/C++
- C# is primarily object-oriented, with more modern language constructs like properties, events, and delegates
- C# offers simplified syntax compared to C++ while maintaining power and flexibility
- C# includes built-in support for asynchronous programming and LINQ (Language Integrated Query)
- C# has strong platform integration with Windows and cross-platform capabilities through .NET Core
// C# example with features not found in C/C++
public class Program
{
public static async Task Main()
{
var numbers = new List<int> { 1, 2, 3, 4, 5 };
// LINQ query syntax
var evenNumbers = from n in numbers
where n % 2 == 0
select n;
// Async/await pattern
await ProcessDataAsync(evenNumbers);
}
private static async Task ProcessDataAsync(IEnumerable<int> data)
{
// Async implementation
}
}
Performance Comparison: C vs C++ vs C#
When considering which language to use for your project, performance characteristics are often a crucial factor:
- C typically offers the highest raw performance and lowest memory overhead, making it ideal for embedded systems and performance-critical applications
- C++ performance is comparable to C for procedural code, with minimal overhead for OOP features when used judiciously, suitable for game engines and high-performance applications
- C# trades some performance for developer productivity and safety, with its garbage collection and runtime environment adding overhead, but modern optimizations have significantly narrowed the gap
Syntax Differences and Code Complexity
The syntax differences between these languages reflect their design philosophies and evolution over time.
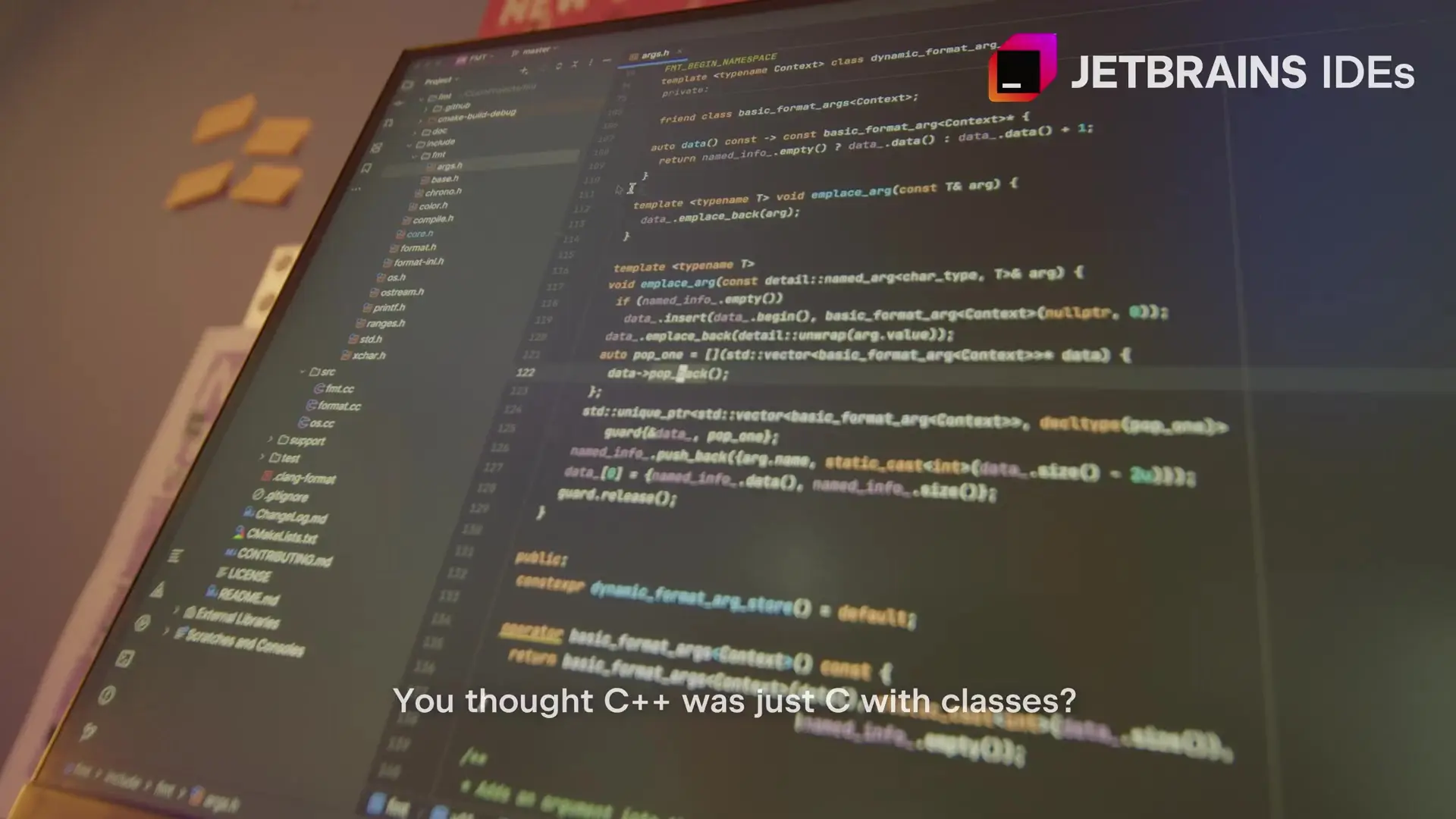
C syntax is relatively simple and straightforward, focused on procedural programming with functions operating on data. C++ extends this syntax to support classes, templates, and other advanced features, which can lead to more complex code structures. C# offers a cleaner, more modern syntax with features designed to reduce common programming errors and boilerplate code.
When to Use Each Language
- Choose C when: You need maximum performance with minimal overhead, are working with limited resources (embedded systems), or need direct hardware manipulation
- Choose C++ when: You need high performance with the benefits of OOP, are developing resource-intensive applications like game engines or simulations, or require fine-grained memory control with modern language features
- Choose C# when: Developer productivity is prioritized over absolute performance, you're building Windows applications or web services, or need rapid application development with a comprehensive standard library
Development Tools and Environment
The development experience differs significantly between these languages. C and C++ development traditionally required more manual configuration and build setup, though modern IDEs like CLion have simplified this process considerably. CLion provides intelligent code assistance, powerful refactoring tools, and integrated debugging capabilities that make C and C++ development more productive.
C# development is typically done in Visual Studio or Visual Studio Code with the .NET SDK, offering a more integrated and streamlined experience out of the box. The tooling differences reflect the different design goals of the languages - C and C++ prioritizing performance and control, while C# emphasizes developer productivity and ease of use.
Conclusion: Choosing the Right Tool for Your Project
Understanding the differences between C, C++, and C# is essential for choosing the right language for your specific needs. Each language has its strengths and appropriate use cases. C excels in low-level system programming, C++ offers a balance of performance and modern features, and C# prioritizes developer productivity and platform integration.
For those working with C or C++, tools like CLion (now free for non-commercial use) can significantly improve the development experience, helping navigate the complexity of these powerful languages. The right combination of language and tools can make all the difference in your development journey.
Let's Watch!
Beyond Basics: Understanding the Key Differences Between C, C++, and C#
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence