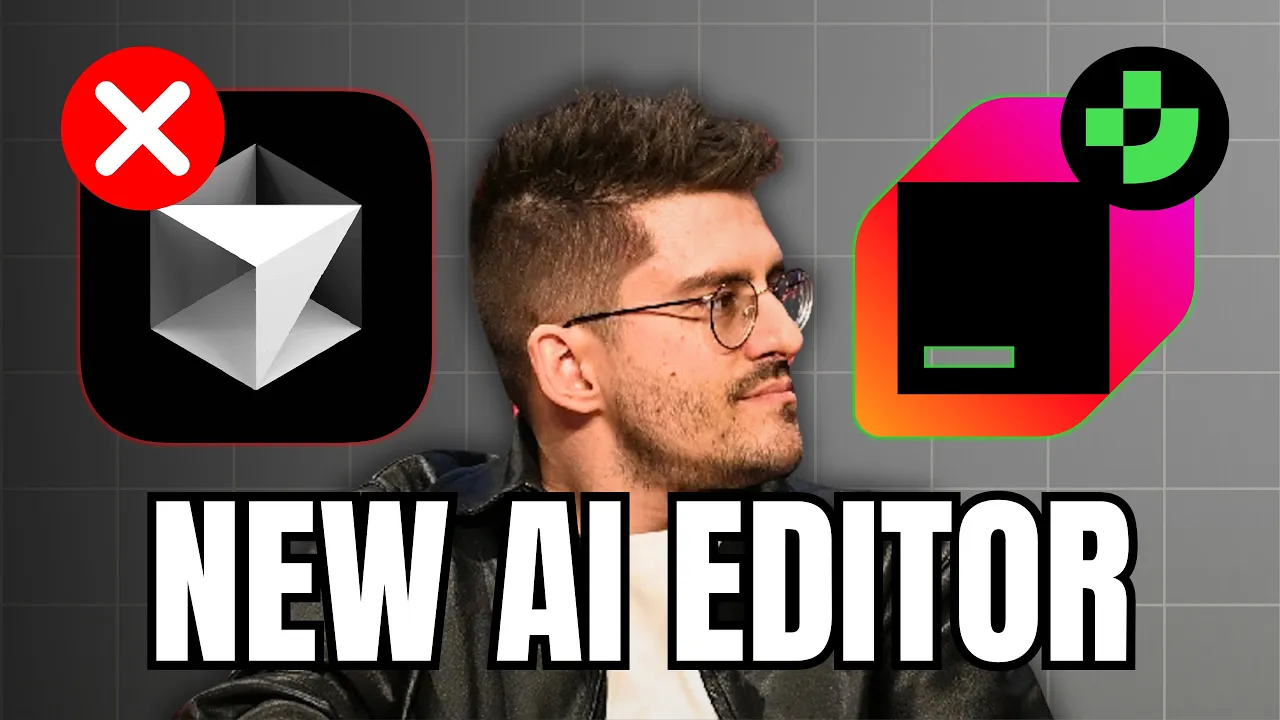
The landscape of AI-assisted coding is evolving rapidly, with JetBrains' Junie emerging as a powerful contender in the PHP development space. This new AI coding agent for PHP Storm demonstrates remarkable capabilities that could significantly enhance developer productivity and code quality. Let's explore what makes Junie special and how it performs when tasked with creating a Laravel todo application from scratch.
What is JetBrains Junie?
Junie is JetBrains' latest AI coding assistant integrated directly into PHP Storm. Unlike traditional code completion tools, Junie can understand project context, generate entire applications, execute terminal commands, and adhere to predefined coding standards. It represents a significant advancement in AI-assisted development tools, potentially positioning itself as a strong alternative to tools like Cursor AI and GitHub Copilot.
One of Junie's most impressive features is its "Brave Mode," which allows the AI to execute terminal commands without requiring confirmation from the developer. While this feature should be used with caution, it dramatically speeds up the development process by automating repetitive tasks.
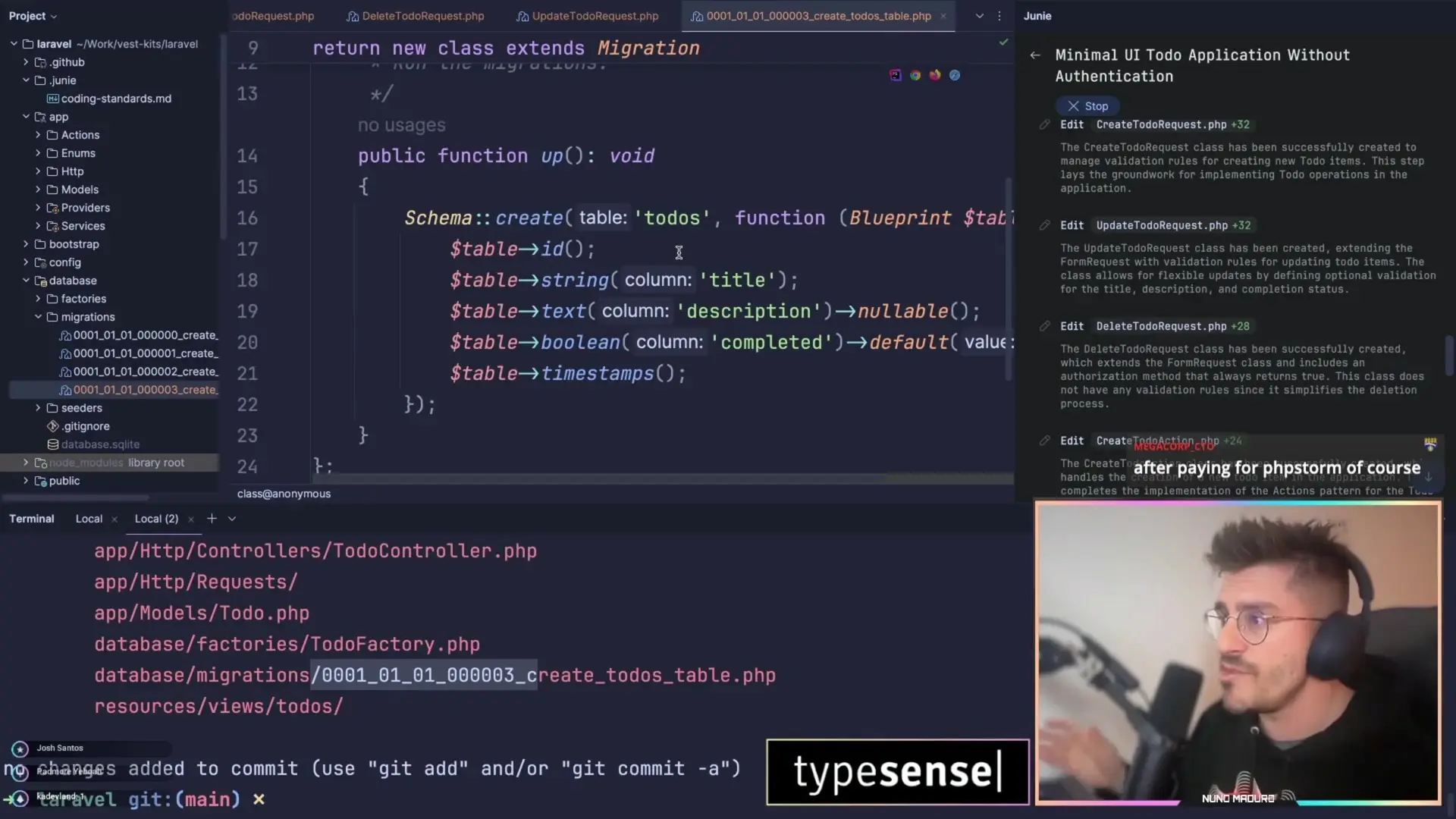
Building a Laravel Todo Application with Junie
To test Junie's capabilities, we challenged it to develop a simple todo application with minimal UI using Laravel. The requirements were straightforward: create a todo application without any overhead that adheres to specific coding standards, including avoiding fillable properties in models.
Database Migration Generation
Junie's first step was creating a migration file for the todos table. The AI correctly structured the migration with appropriate fields: a title (string), description (text), and a completed boolean field. While it included a default value for the completed field (which might not align with some coding preferences), the overall structure was sound and demonstrated Junie's understanding of database design principles.
public function up(): void
{
Schema::create('todos', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('description')->nullable();
$table->boolean('completed')->default(false);
$table->timestamps();
});
}
Model Creation with Property Attributes
Perhaps the most impressive aspect of Junie's implementation was its model creation. The AI generated a Todo model that followed modern PHP practices, including proper property attributes documentation. Notably, Junie respected the coding standard of avoiding fillable properties, instead using property attributes to document the model's structure.
final class Todo extends Model
{
/**
* @property int $id
* @property string $title
* @property string|null $description
* @property bool $completed
* @property Carbon $created_at
* @property Carbon $updated_at
*/
protected $casts = [
'completed' => 'boolean',
];
}
This implementation demonstrates Junie's ability to understand and apply modern PHP coding practices, including the use of final classes and properly documented property attributes.
Factory Generation
Junie also generated a factory for the Todo model, correctly implementing PHP generics and using appropriate faker methods for each field type: sentence for the title, paragraph for the description, and boolean for the completed field.
class TodoFactory extends Factory
{
/**
* @return array<string, mixed>
*/
public function definition(): array
{
return [
'title' => $this->faker->sentence(),
'description' => $this->faker->paragraph(),
'completed' => $this->faker->boolean(),
];
}
}
Action Classes Implementation
Following modern Laravel development practices, Junie created dedicated action classes for creating and deleting todos. While the implementation was solid, there was room for improvement in terms of array shapes and data transfer objects (DTOs).
final class CreateTodoAction
{
/**
* Create a new todo
*
* @param array<string, mixed> $data
* @return Todo
*/
public function handle(array $data): Todo
{
return Todo::query()->create([
'title' => $data['title'],
'description' => $data['description'] ?? null,
'completed' => $data['completed'] ?? false,
]);
}
}
Controller Implementation
The TodoController generated by Junie followed RESTful principles and properly utilized the action classes. The controller included methods for index, create, store, show, edit, update, and destroy operations, all properly implemented with appropriate response types.
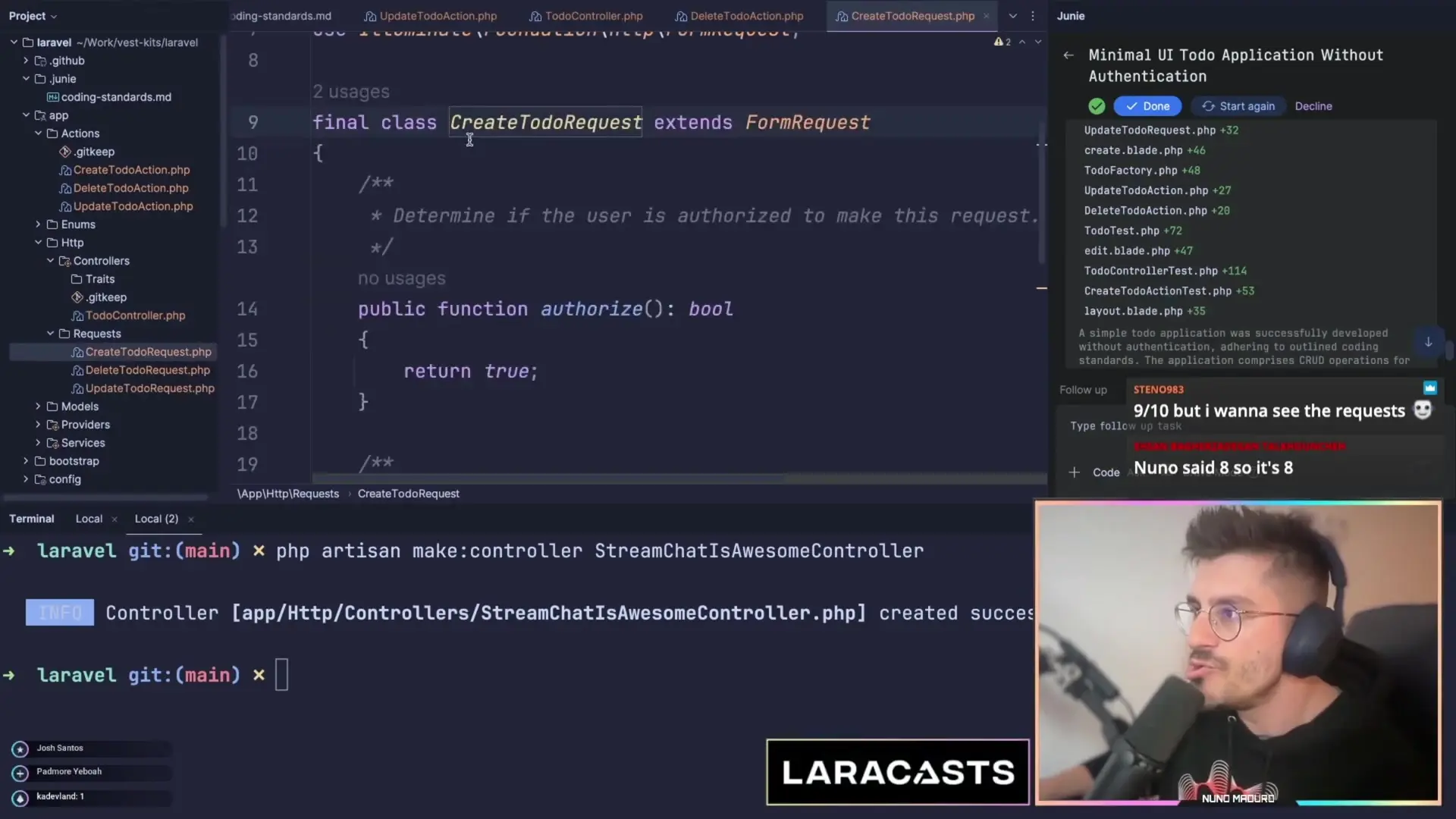
public function store(CreateTodoRequest $request, CreateTodoAction $action): RedirectResponse
{
$action->handle($request->validated());
return to_route('todos.index')->with('success', 'Todo created successfully.');
}
One minor issue was the use of the compact() function in some methods, which introduces a level of magic that some developers prefer to avoid for clarity. A more explicit approach would be preferred for maximum code readability.
Form Request Validation
Junie also created form request classes for validation, correctly implementing rules such as requiring the title field and setting a maximum length of 255 characters, which aligns with the database schema.
final class CreateTodoRequest extends FormRequest
{
public function authorize(): bool
{
return true;
}
/**
* @return array<string, \Illuminate\Contracts\Validation\ValidationRule|array|string>
*/
public function rules(): array
{
return [
'title' => ['required', 'string', 'max:255'],
'description' => ['nullable', 'string'],
'completed' => ['nullable', 'boolean'],
];
}
}
Junie vs. Other AI Coding Assistants
What sets Junie apart from other AI coding assistants like the standard JetBrains AI assistant or GitHub Copilot is its ability to not just suggest code but to actually execute terminal commands, create files, and implement entire features. This makes it significantly more powerful for rapid application development.
- Junie can execute terminal commands autonomously in Brave Mode
- It understands project context and coding standards
- It generates not just snippets but complete, working features
- It follows modern PHP practices like property attributes documentation
- It integrates seamlessly with PHP Storm's development environment
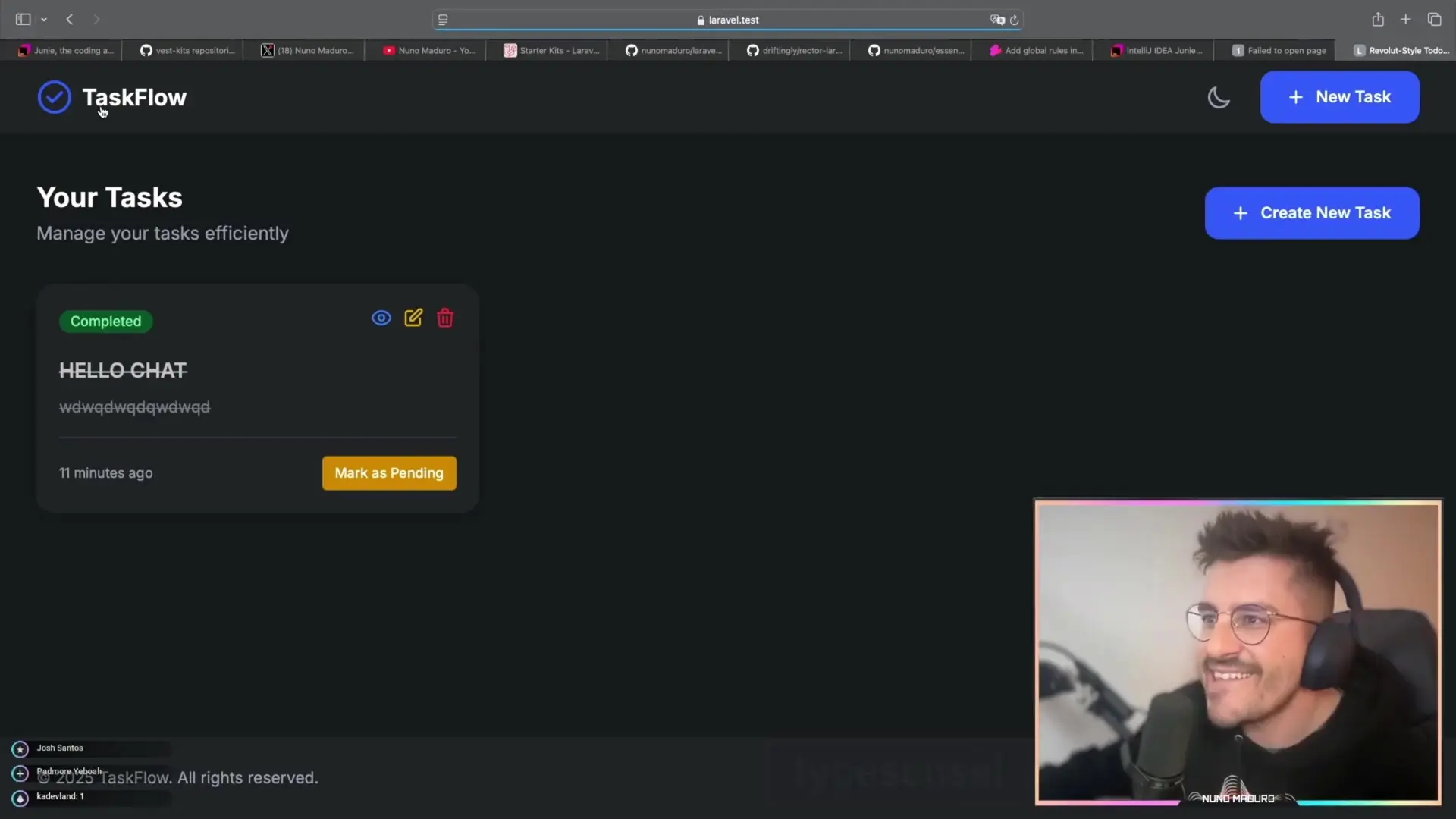
Strengths and Areas for Improvement
Strengths
- Excellent understanding of Laravel architecture and conventions
- Proper implementation of modern PHP features and documentation practices
- Ability to generate complete, working features from simple prompts
- Adherence to specified coding standards
- Terminal command execution capabilities
Areas for Improvement
- Use of compact() function in controllers reduces code clarity
- Could better implement array shapes or DTOs for data validation
- Default values in migrations might be better placed in actions
- Some minor inconsistencies in coding style
Conclusion: Is Junie the Future of Laravel Development?
JetBrains Junie represents a significant advancement in AI-assisted PHP development. Its ability to understand project context, adhere to coding standards, and generate complete features makes it a powerful tool for Laravel developers. While there are still areas for improvement, the overall quality of the code generated is impressive.
For PHP developers looking to increase productivity without sacrificing code quality, Junie offers a compelling alternative to existing AI coding assistants. Its deep integration with PHP Storm and understanding of Laravel conventions make it particularly valuable for rapid application development in this ecosystem.
As AI coding tools continue to evolve, Junie sets a new standard for what developers can expect from their development environments. Whether it will completely replace traditional coding practices remains to be seen, but it certainly offers a glimpse into a future where AI and human developers work together to create better software more efficiently.
Let's Watch!
Hands-On with JetBrains Junie: The PHP Storm AI Assistant That's Changing Laravel Development
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence