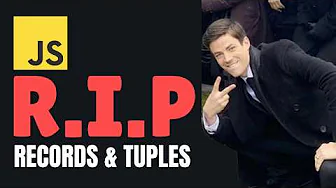
The JavaScript development community has been buzzing with news that the long-anticipated Records and Tuples proposal has been discontinued after reaching stage two in the TC39 process. According to the committee, the proposal "was unable to gain further consensus" despite significant progress in the standardization pipeline. However, this setback comes with a silver lining: a new proposal called Composites that may offer even better solutions to the same problems.
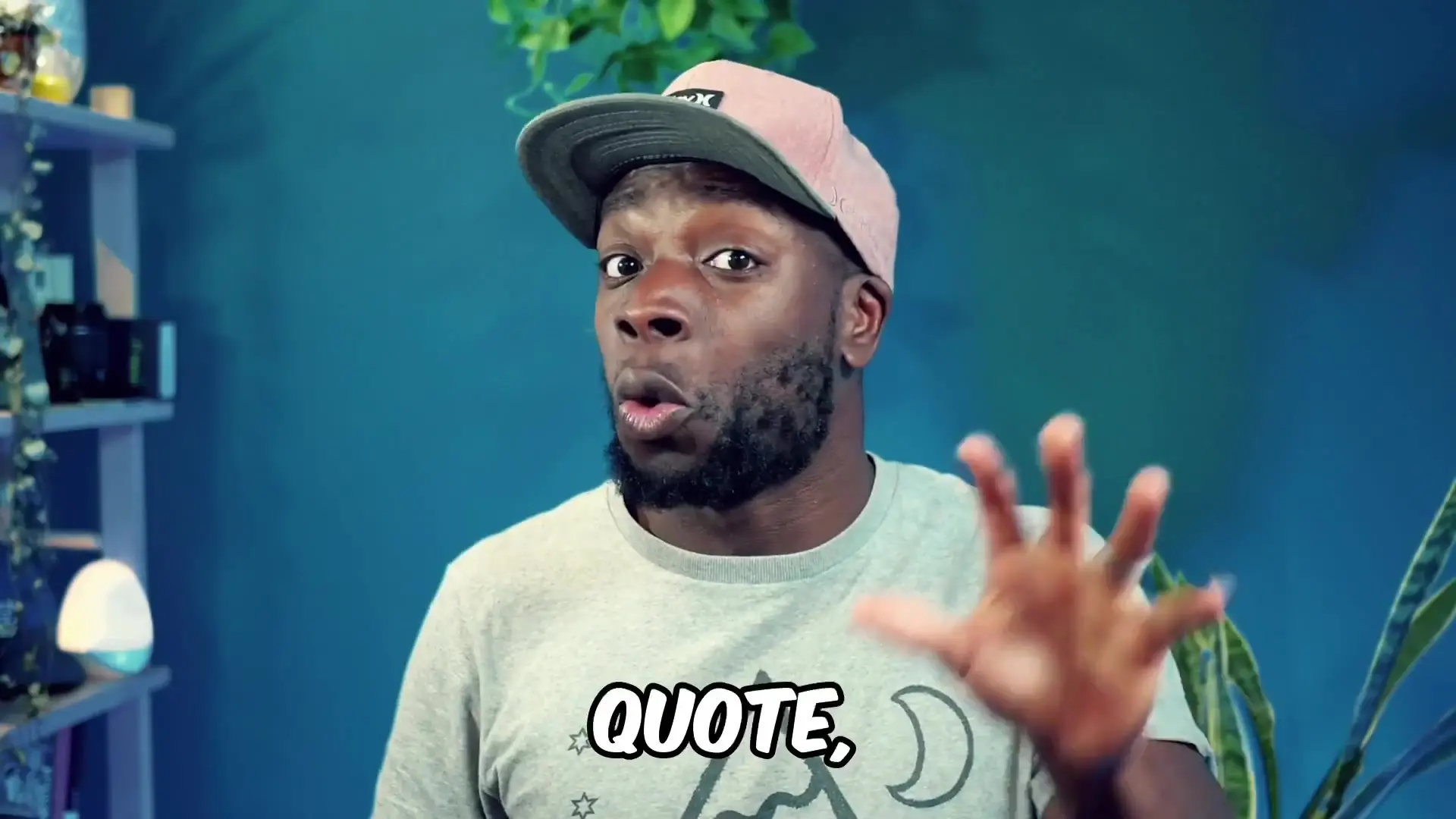
The Problem with JavaScript Object Mutability
To understand why Records and Tuples (and now Composites) are important, we need to examine a fundamental issue with JavaScript objects. Despite using the `const` keyword, objects in JavaScript remain mutable, which can lead to unexpected behavior and bugs in complex applications.
While developers can use `Object.freeze()` to create immutable objects, this approach has two significant limitations:
- Two frozen objects with identical properties will never equal each other because they are different object instances
- `Object.freeze()` only works at the top level (shallow immutability) and doesn't protect nested objects without custom solutions
These limitations create challenges when working with complex data structures, particularly in applications where immutability and predictable equality comparisons are crucial for performance and reliability.
What Records and Tuples Would Have Offered
Records and Tuples were designed as new JavaScript primitives that would have provided two key benefits:
- Deep immutability by default
- Value-based equality (rather than reference-based equality)
The syntax would have been simple and elegant - just adding a hash symbol (#) before an object or array literal would transform it into a record or tuple, respectively. This would have aligned JavaScript with features available in other programming languages like Python.
// Record example (immutable object)
const user = #{ name: "John", age: 30 };
// Tuple example (immutable array)
const coordinates = #[10, 20];
// Value-based equality
#{ a: 1, b: 2 } === #{ a: 1, b: 2 }; // true
#[1, 2, 3] === #[1, 2, 3]; // true
Introducing Composites: The New Alternative
With Records and Tuples no longer moving forward, the TC39 committee has introduced Composites as an alternative approach. Composites can be thought of as a more pragmatic version of the Records and Tuples proposal, designed to achieve similar goals without requiring entirely new primitive types in the language.
The Composites proposal (currently at stage one) offers a solution that uses existing JavaScript mechanisms while providing the key benefits developers were seeking:
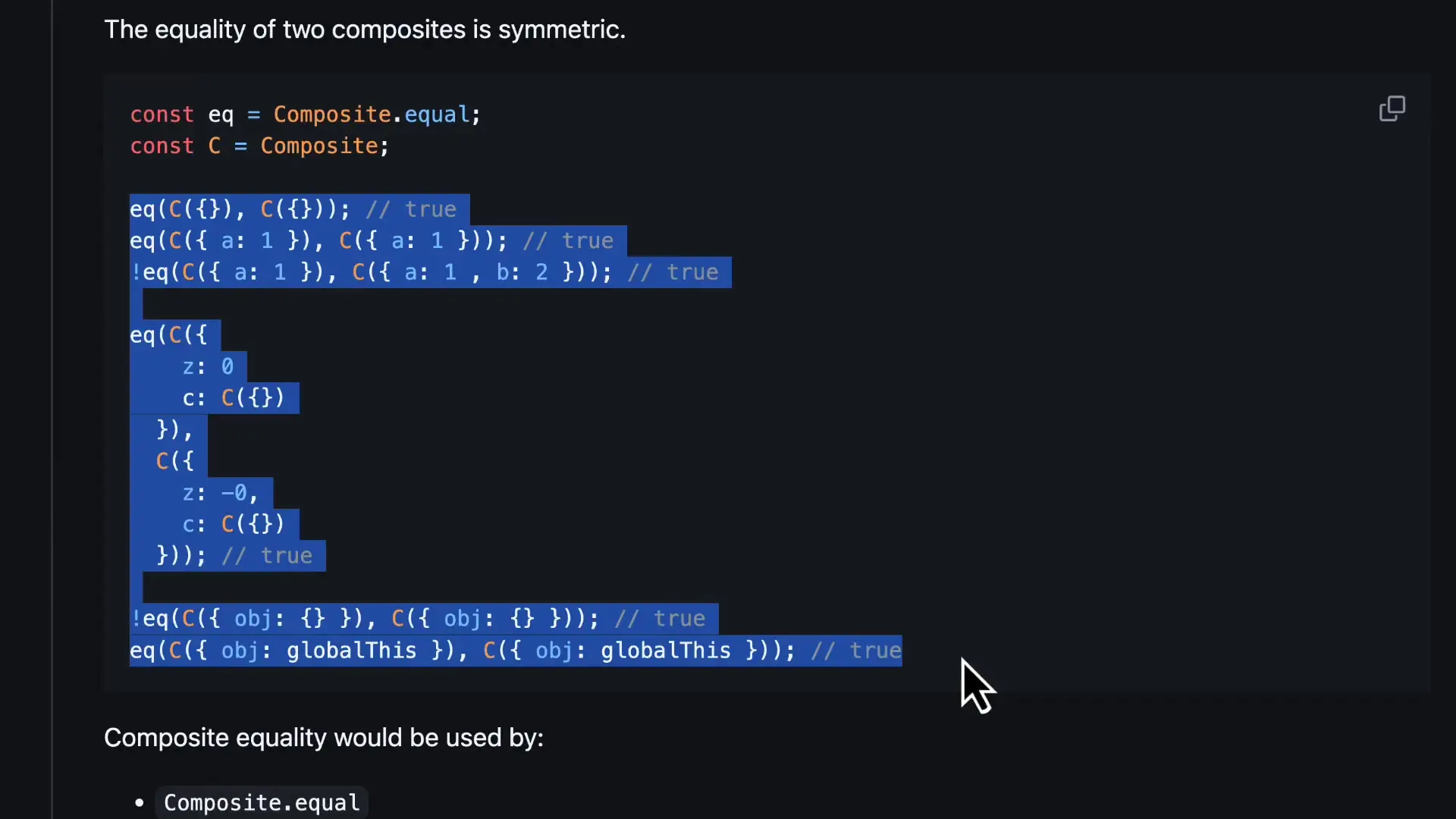
How Composites Work
Composites utilize `Object.freeze()` under the hood but add an important enhancement: a dedicated `.equal()` method for checking value-based equality. This approach provides shallow immutability out of the box without requiring a completely new primitive type in the language.
// Creating a composite object
const person = Composite({ name: "Alice", role: "Developer" });
// Creating a composite array
const numbers = Composite.array([1, 2, 3]);
// Using the .equal() method for value-based comparison
Composite({ a: 1, b: 2 }).equal(Composite({ a: 1, b: 2 })); // true
Composite.array([1, 2, 3]).equal(Composite.array([1, 2, 3])); // true
While the syntax is different from the hash-based approach of Records and Tuples, the Composites proposal includes options to potentially support the original Records and Tuples syntax as an alternative notation.
Advantages of the Composites Approach
- Implementation simplicity: Uses existing JavaScript mechanisms rather than introducing entirely new primitives
- Compatibility: Works with existing JavaScript code and patterns
- Faster path to adoption: May face fewer implementation hurdles than Records and Tuples
- Flexibility: Can potentially support multiple syntax options including the Records and Tuples notation
Practical Use Cases for Composites in JavaScript Components
When building javascript components, Composites can be particularly valuable for several common scenarios:
- State management: Immutable data structures simplify state tracking and change detection in complex applications
- Configuration objects: Creating guaranteed immutable configuration objects prevents accidental modifications
- API responses: Converting API data to Composites provides predictable equality checking and prevents mutation
- Component props: Using Composites for component properties can improve performance by enabling more reliable memoization
Comparing Records vs Map in JavaScript
When considering record vs map javascript structures, it's important to understand their different characteristics and use cases. Maps already provide some benefits similar to what Records would have offered, but with different tradeoffs:
- Maps allow any value as keys (including objects), while Records (and now Composites) use standard object property keys
- Maps maintain insertion order, similar to modern JavaScript objects
- Maps are mutable by default, unlike the immutable Records and Composites
- Maps require method calls for access (.get(), .set()), while Records and Composites use standard property access notation
Composites offer a middle ground that provides immutability with familiar object syntax, making them potentially more appealing for many use cases than Maps when immutability is a requirement.
Current Status and How to Start Using Composites
The Composites proposal is currently at stage one in the TC39 process, which means it's still in the exploratory phase. However, developers interested in experimenting with this functionality don't need to wait for official implementation.
A polyfill for Composites is already available and reportedly works quite well. This allows developers to start experimenting with the concept in their projects today, though caution is advised for production use given the proposal's early stage.
// Using the Composites polyfill
// First, install via npm
// npm install @bloomberg/record-tuple-polyfill
import { Composite } from '@bloomberg/record-tuple-polyfill';
const userSettings = Composite({
theme: "dark",
notifications: true,
language: "en-US"
});
// Immutable - this will throw an error
// userSettings.theme = "light";
// Create a new composite with changes instead
const updatedSettings = Composite({
...userSettings,
theme: "light"
});
The Future of JavaScript Immutability
While the discontinuation of Records and Tuples was disappointing for many in the JavaScript community, the introduction of Composites represents an evolution in thinking about how to bring immutable data structures to the language.
The TC39 process is deliberately cautious, ensuring that new features are thoroughly vetted before becoming part of the language specification. This sometimes means promising proposals don't make it to the finish line, but it also ensures that the features that do get implemented are robust and well-designed.
For developers working with javascript components who need immutability today, several options exist beyond waiting for Composites to progress through the standardization process:
- Libraries like Immutable.js and Immer provide comprehensive solutions for immutable data structures
- Object.freeze() with careful handling of nested objects can work for simpler cases
- TypeScript's readonly modifiers can provide compile-time immutability guarantees
- The Composites polyfill offers a forward-looking approach that may align with future standards
Conclusion
While the JavaScript community may have lost Records and Tuples, the emergence of Composites offers a promising path forward for developers seeking immutable data structures with value-based equality. The more pragmatic approach taken by Composites may ultimately lead to faster adoption and implementation, bringing these important capabilities to JavaScript sooner rather than later.
As with any stage one proposal, the final form of Composites may evolve significantly before reaching standardization. Developers interested in these features should follow the proposal's progress through the TC39 process while exploring the available polyfill to gain hands-on experience with this potentially game-changing addition to JavaScript.
Let's Watch!
JavaScript Composites: The New Alternative to Records and Tuples
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence