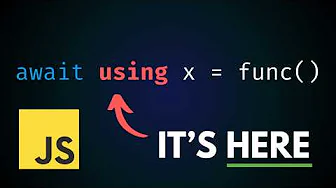
With the recent Node.js v24 update, JavaScript developers have gained access to an exciting new language feature: the 'using' keyword. This addition completely transforms how we handle resource cleanup in our code, potentially eliminating the need for try-finally blocks in many common scenarios. In this article, we'll explore how this new syntax works, examine practical applications, and consider its impact on JavaScript development.
Understanding the Problem: Resource Cleanup in JavaScript
Before diving into the new syntax, let's understand the problem it solves. Consider a common scenario: creating a temporary file, performing operations with it, then closing and deleting it when done. Traditionally, we might write code like this:
// Traditional approach
const tempFile = createTempFile();
// Use the file for something
tempFile.close();
fs.unlinkSync(tempFile.path);
This approach works fine in simple cases, but what if we need to close the file early due to a specific condition? We'd need to duplicate our cleanup code:
const tempFile = createTempFile();
if (someCondition) {
// Early cleanup
tempFile.close();
fs.unlinkSync(tempFile.path);
return;
}
// Normal flow
// Use the file
tempFile.close();
fs.unlinkSync(tempFile.path);
The standard solution to this code duplication is using try-finally blocks:
const tempFile = createTempFile();
try {
// Use the file
if (someCondition) {
return; // Early exit is safe now
}
// More operations
} finally {
// Cleanup always happens
tempFile.close();
fs.unlinkSync(tempFile.path);
}
While this works, it can become messy with multiple resources, especially when they depend on each other. This is where the new 'using' keyword comes in to simplify everything.
The 'using' Keyword: A Cleaner Approach
With the new 'using' keyword, we can rewrite our file handling example much more elegantly:
{
using tempFile = createTempFile();
// Use the file
if (someCondition) {
return; // File will be automatically closed and deleted
}
// More operations
} // File automatically closed and deleted here
The 'using' keyword in JavaScript variables can be declared to automatically handle resource cleanup at the end of the scope or before an early return. This is significantly cleaner and less error-prone than manual cleanup or try-finally blocks.
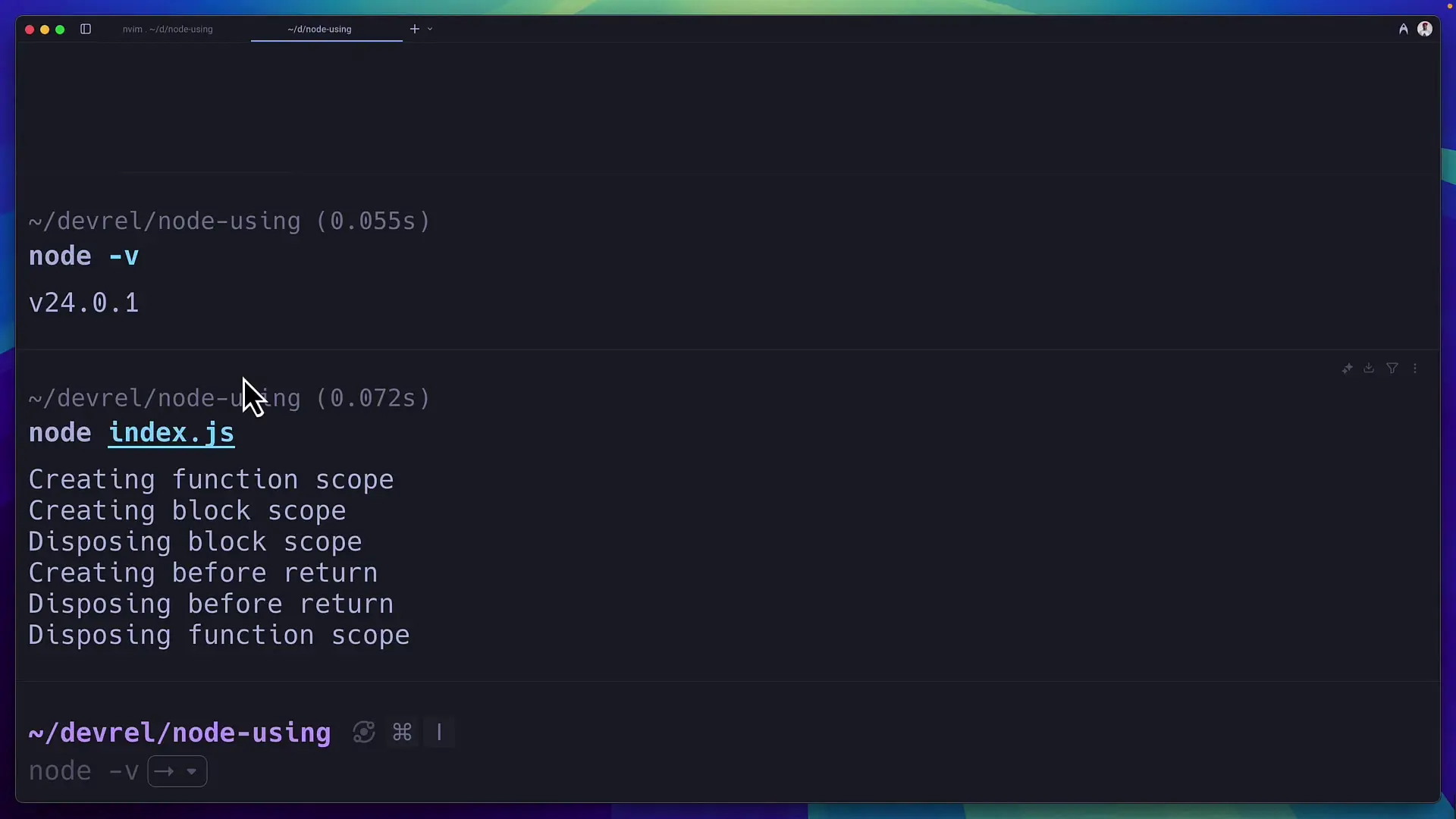
How the 'using' Keyword Works Behind the Scenes
For a variable to work with the 'using' keyword, the object it references must implement a special method: Symbol.dispose. When we create an object using new keyword in JavaScript that implements this method, the JavaScript runtime will automatically call this method when the variable goes out of scope.
// A simple disposable resource
class Resource {
constructor(name) {
this.name = name;
console.log(`Creating ${name}`);
}
[Symbol.dispose]() {
console.log(`Disposing ${this.name}`);
}
}
function demo() {
using functionResource = new Resource('function scope');
{
using blockResource = new Resource('block scope');
console.log('Inside block');
} // blockResource disposed here
console.log('Before return');
return 'done';
} // functionResource disposed here
demo();
When this code runs, we see the following output:
Creating function scope
Creating block scope
Inside block
Disposing block scope
Before return
Disposing function scope
This demonstrates how resources are created and disposed in the reverse order of their creation, following proper resource management principles.
The 'await using' Pattern for Asynchronous Resources
JavaScript also introduces a variant for asynchronous resources with the 'await using' syntax. When using the await keyword in JavaScript with 'using', the object must implement Symbol.asyncDispose instead:
class AsyncResource {
constructor(name) {
this.name = name;
console.log(`Creating ${name}`);
}
async [Symbol.asyncDispose]() {
console.log(`Starting disposal of ${this.name}`);
await new Promise(resolve => setTimeout(resolve, 100));
console.log(`Finished disposal of ${this.name}`);
}
}
async function demoAsync() {
await using resource = new AsyncResource('async resource');
console.log('Using async resource');
} // resource.asyncDispose() will be awaited here
demoAsync();
This is particularly useful for resources that need asynchronous cleanup, such as database connections or network resources.
Managing Multiple Resources with DisposableStack
For cases where you need to manage multiple disposable resources, JavaScript provides the DisposableStack and AsyncDisposableStack classes:
function multipleResources() {
using stack = new DisposableStack();
const resource1 = new Resource('first');
stack.adopt(resource1); // Will be disposed when stack is disposed
const resource2 = new Resource('second');
stack.adopt(resource2);
console.log('Using multiple resources');
} // All resources disposed when stack goes out of scope
The stack.adopt() method adds resources to be disposed when the stack itself is disposed, giving you flexible control over multiple resources.
Practical Applications of the 'using' Keyword
The 'using' keyword has several practical applications in JavaScript development:
- File handling (opening, using, and automatically closing files)
- Database connections (ensuring connections are properly closed)
- Network resources (sockets, HTTP connections)
- Locks and semaphores (automatically releasing when done)
- Unit testing (setting up and tearing down test environments)
For unit testing, the 'using' keyword is particularly valuable. Instead of relying on beforeEach and afterEach helper functions, you can write more portable tests:
test('should process data correctly', () => {
using testDb = createTestDatabase();
using mockServer = createMockServer();
// Test code here
const result = processData(testDb, mockServer);
expect(result).toBe(expectedValue);
// No need for cleanup - happens automatically
});
Browser Compatibility and Node.js Version Requirements
To use this feature, you'll need Node.js version 24 or later. As of writing, browser support is limited, so it's primarily a server-side JavaScript feature for now. When using the 'using' keyword in JavaScript, be aware of compatibility concerns for production code.
Comparison with Other Languages
The 'using' keyword isn't unique to JavaScript. Similar constructs exist in other programming languages:
- C# has the 'using' statement for IDisposable objects
- Python has 'with' context managers
- Java has try-with-resources
- Kotlin has 'use' extension function
JavaScript's implementation is most similar to C#'s approach, continuing the trend of JavaScript adopting features from statically-typed languages.
Conclusion: Is the 'using' Keyword Worth Adopting?
The 'using' keyword is a welcome addition to JavaScript that can significantly clean up resource management code. It's particularly valuable for scenarios involving file operations, database connections, and testing environments.
However, it's worth considering whether JavaScript is accumulating too many features too quickly. Along with other recent and proposed features like composits, the pipe operator, and structs, JavaScript continues to evolve at a rapid pace.
For everyday JavaScript development, the 'using' keyword may not be something you reach for frequently, but when you do need it for resource management, it provides a cleaner, more maintainable alternative to try-finally blocks. In JavaScript, a function is defined using the function keyword, but with 'using', we now have an elegant way to handle cleanup code that makes our programs more robust and readable.
Let's Watch!
The New JavaScript 'using' Keyword: Write Cleaner Code for Resource Management
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence