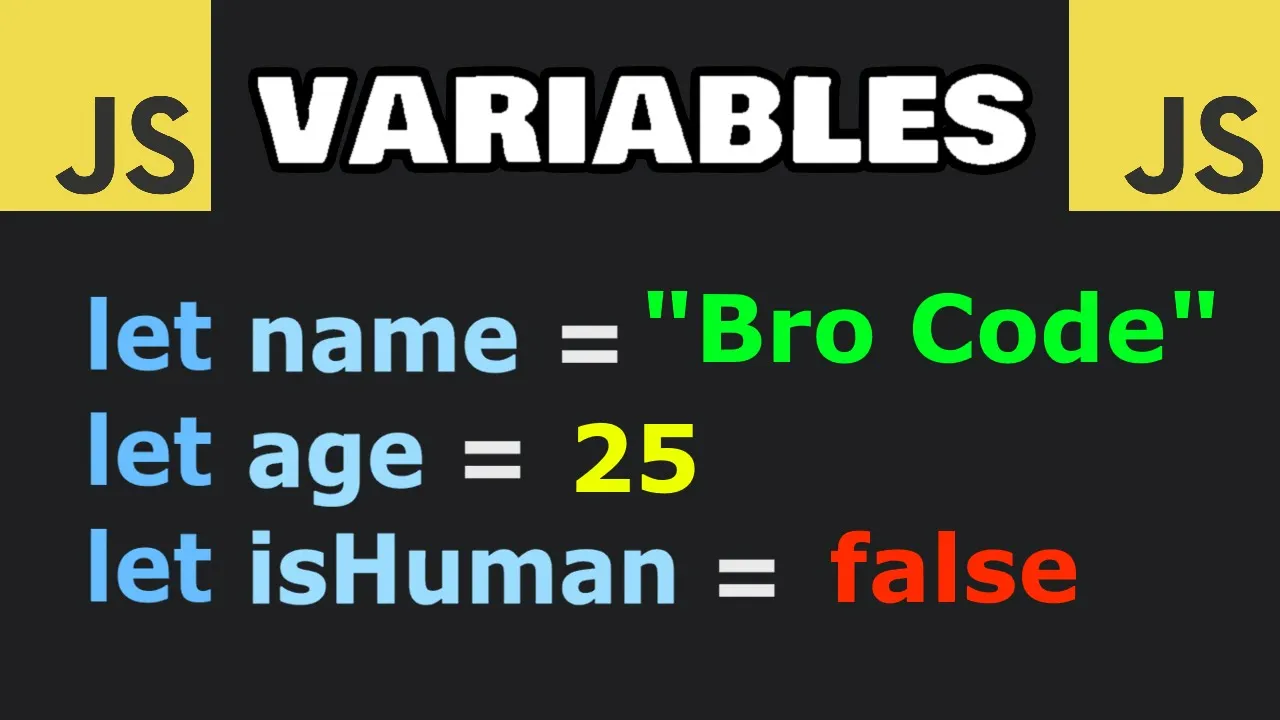
Variables are the foundation of any programming language, and JavaScript is no exception. If you're just starting your coding journey or need a refresher on the basics, understanding variables is essential. Think of variables as containers that store values - much like the algebraic 'x' you learned about in school. In this comprehensive guide, we'll explore how variables work in JavaScript, how to create them, and the different types of data they can hold.
What Are JavaScript Variables?
In JavaScript, a variable is a named container that stores a value. The beauty of variables is that they behave as if they were the value they contain. This allows you to reference and manipulate data throughout your code without repeating the actual values.
Creating a variable in JavaScript involves two key steps: declaration and assignment. Let's break down each step.
Step 1: Declaration
To declare a variable, you use the 'let' keyword followed by a unique variable name and a semicolon:
let x;
This tells JavaScript to reserve memory for a variable named 'x'. It's important to note that each variable name must be unique within its scope. If you try to declare another variable with the same name, you'll encounter a syntax error: 'Identifier x has already been declared.'
Step 2: Assignment
After declaration, you can assign a value to your variable using the assignment operator (=):
x = 100;
You can also combine declaration and assignment in a single line, which is common practice when you know the initial value:
let x = 100;
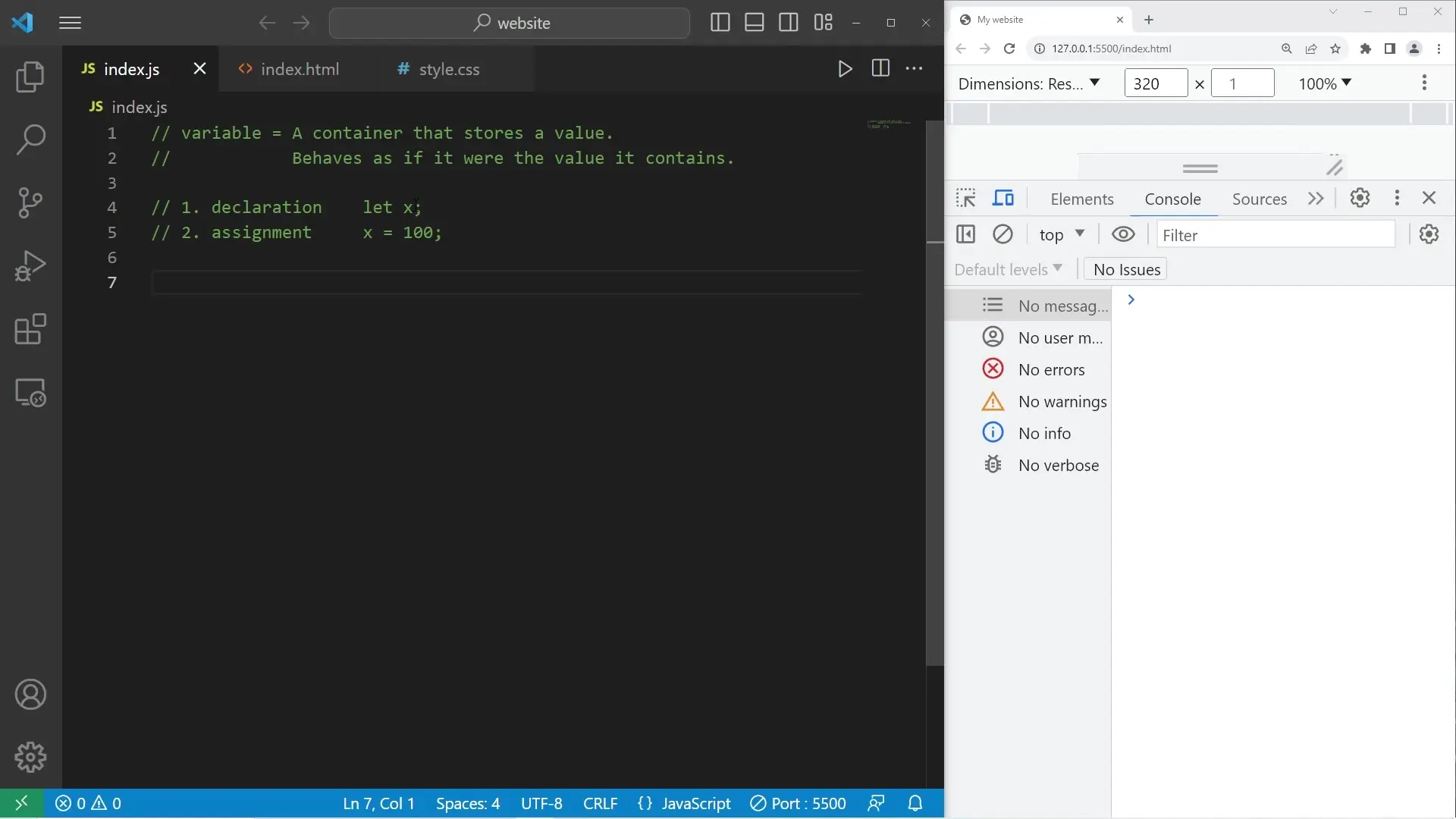
JavaScript Data Types: Numbers, Strings, and Booleans
JavaScript has several data types, but we'll focus on the three most basic ones: numbers, strings, and booleans.
Working with Numbers
Numbers in JavaScript can be integers or decimals (floating-point numbers). Let's look at some examples:
let age = 25;
let price = 10.99;
let gpa = 2.1;
console.log(typeof age); // Output: number
console.log(`You are ${age} years old`);
console.log(`The price is $${price}`);
console.log(`Your GPA is ${gpa}`);
As shown above, we can use the 'typeof' operator to check the data type of a variable. All three examples (age, price, and gpa) are of the 'number' type, despite some having decimal points.
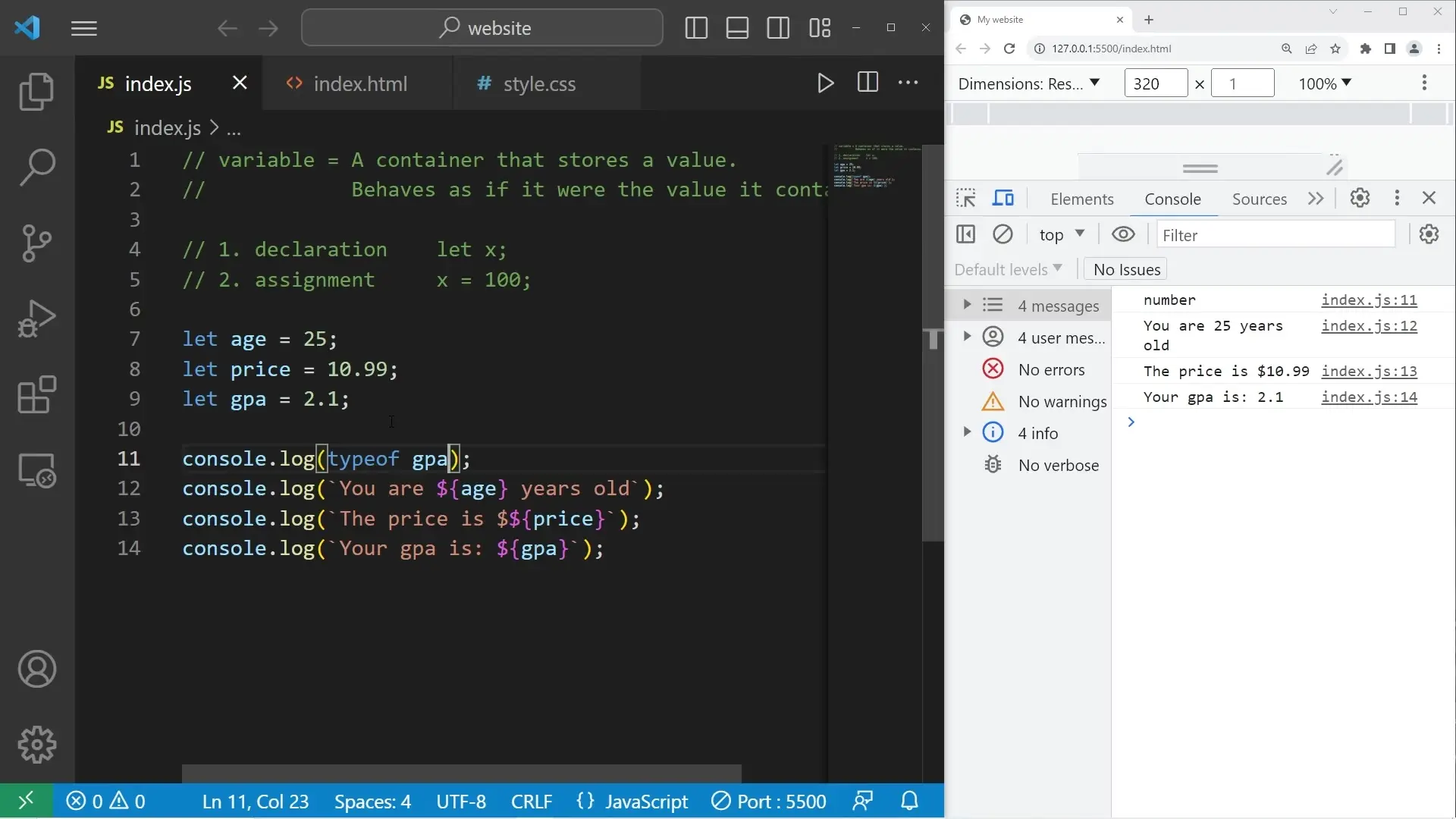
Working with Strings
Strings are sequences of characters enclosed in either single quotes (') or double quotes ("). They're used to represent text data:
let firstName = "John";
let favoriteFood = 'pizza';
let email = "[email protected]";
console.log(typeof firstName); // Output: string
console.log(`Your name is ${firstName}`);
console.log(`You like ${favoriteFood}`);
console.log(`Your email is ${email}`);
An important distinction: strings can contain numbers (like '123'), but these numbers can't be used in mathematical operations because they're treated as text, not numerical values.
Working with Booleans
Boolean values are either true or false. They're often used as flags or conditions in programming:
let online = true;
let forSale = true;
let isStudent = false;
console.log(typeof online); // Output: boolean
console.log(`John is online: ${online}`);
console.log(`Is this car for sale: ${forSale}`);
console.log(`Enrolled: ${isStudent}`);
Booleans are essential for control flow in your programs, particularly with conditional statements (if/else). They allow your code to make decisions based on whether conditions are true or false.
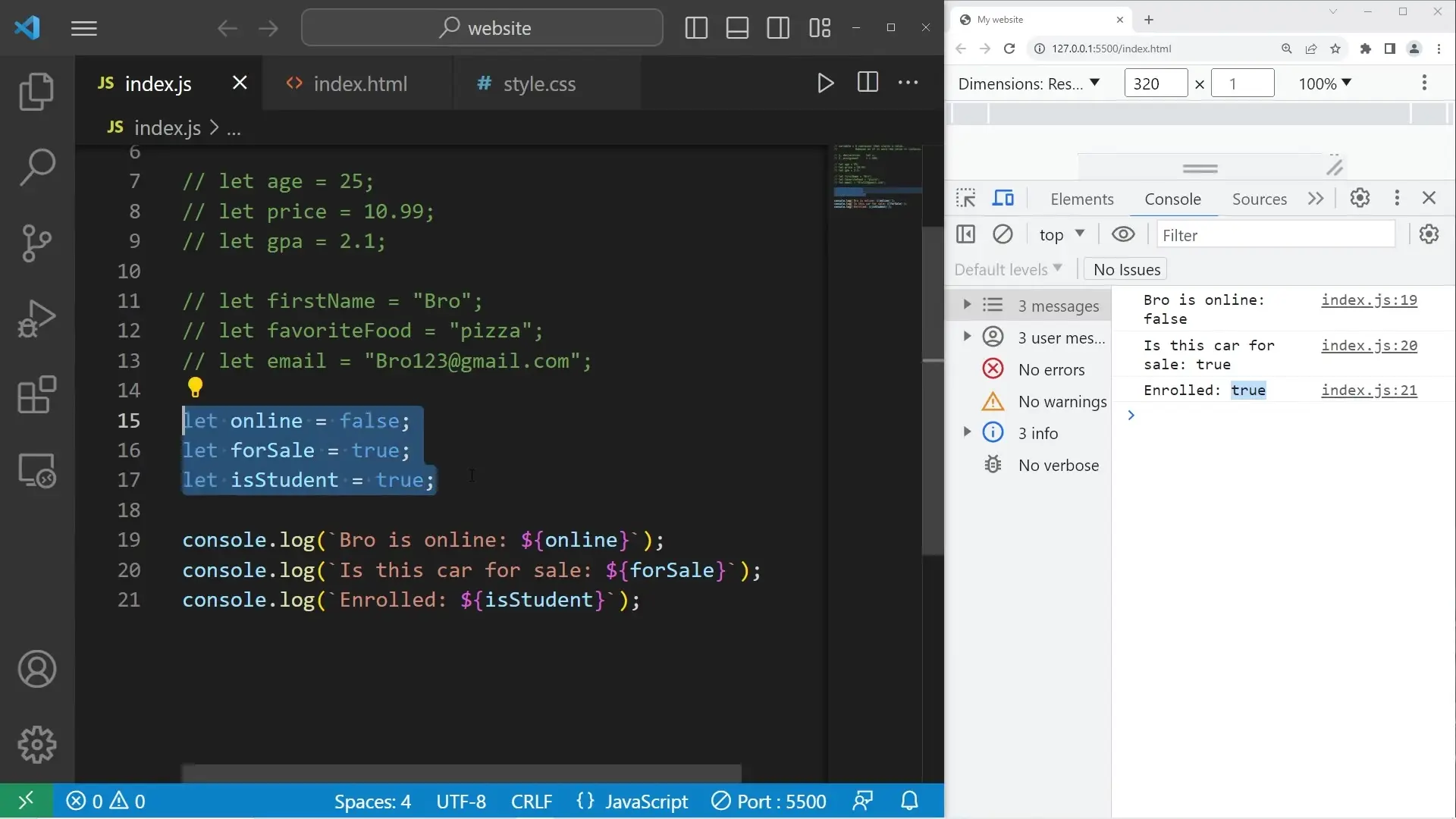
Template Literals: Embedding Variables in Strings
JavaScript offers a powerful way to include variables within strings using template literals. These are created using backticks (`) instead of quotes, with variables embedded using ${variable} syntax:
let fullName = "Jane Doe";
let age = 28;
let isStudent = true;
console.log(`Your name is ${fullName}`);
console.log(`You are ${age} years old`);
console.log(`Enrolled: ${isStudent}`);
Template literals make your code more readable when you need to combine text with variable values, eliminating the need for string concatenation with the + operator.
Displaying Variables in HTML
One of the most common uses of JavaScript variables is to dynamically update content on a webpage. Here's how you can modify HTML elements using JavaScript variables:
// Define your variables
let fullName = "Jane Doe";
let age = 28;
let isStudent = true;
// Select HTML elements and update their content
document.getElementById("p1").textContent = `Your name is ${fullName}`;
document.getElementById("p2").textContent = `You are ${age} years old`;
document.getElementById("p3").textContent = `Enrolled: ${isStudent}`;
This code assumes you have three paragraph elements in your HTML with IDs of "p1", "p2", and "p3". The textContent property changes the text inside these elements to include our variable values.
Best Practices for Working with Variables
- Use descriptive variable names that indicate what data they contain (e.g., 'firstName' instead of 'x')
- Declare variables with 'let' (or 'const' for values that won't change)
- Combine declaration and assignment when you know the initial value
- Use template literals for string interpolation rather than concatenation
- Check variable types with the 'typeof' operator when debugging
- Be consistent with your naming conventions (camelCase is common in JavaScript)
Conclusion
Variables are the fundamental building blocks of any JavaScript program. They allow you to store, retrieve, and manipulate data throughout your code. By understanding how to declare and assign variables, and how to work with different data types like numbers, strings, and booleans, you've taken an important step in your JavaScript journey.
As you continue learning, you'll discover more complex data types and ways to work with variables, but mastering these basics will serve as a solid foundation for all your future programming endeavors.
Let's Watch!
JavaScript Variables Explained: Master the Core Building Blocks of Programming
Neural Knowledge Pathways
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence