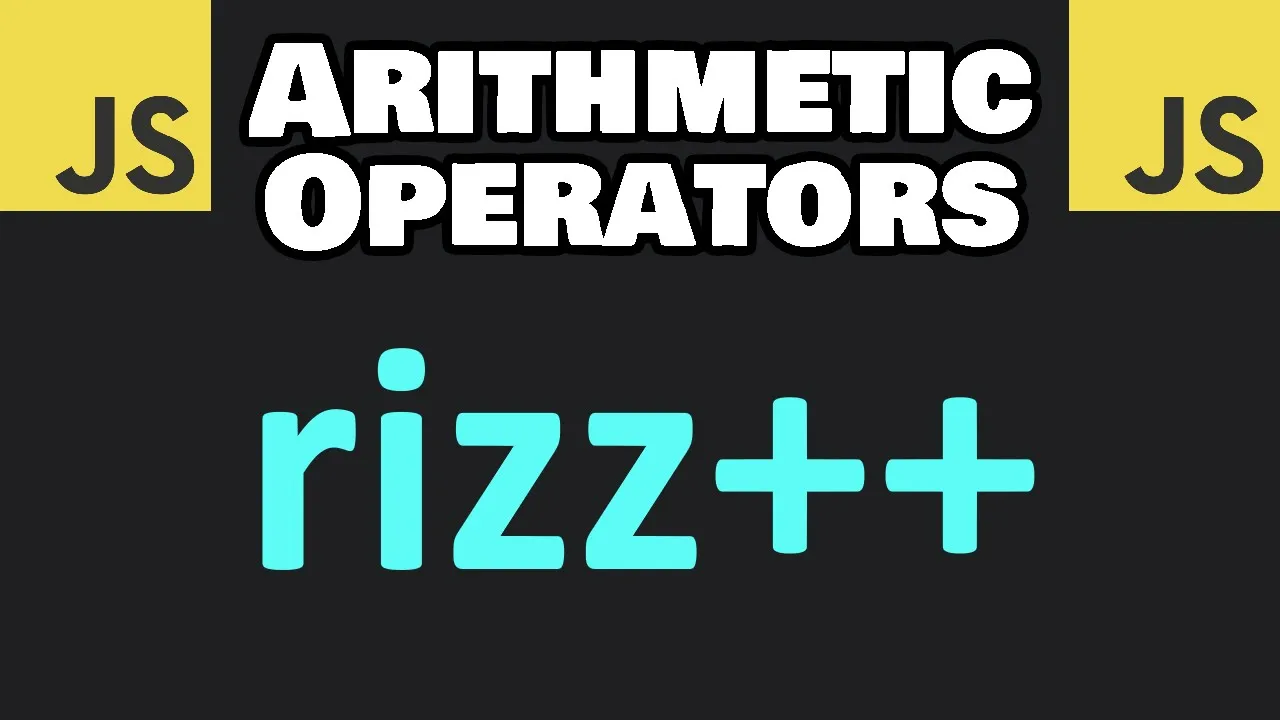
Arithmetic operators form the foundation of mathematical operations in JavaScript. Whether you're calculating totals in an e-commerce site, manipulating data, or creating interactive web applications, understanding these operators is essential for any developer.
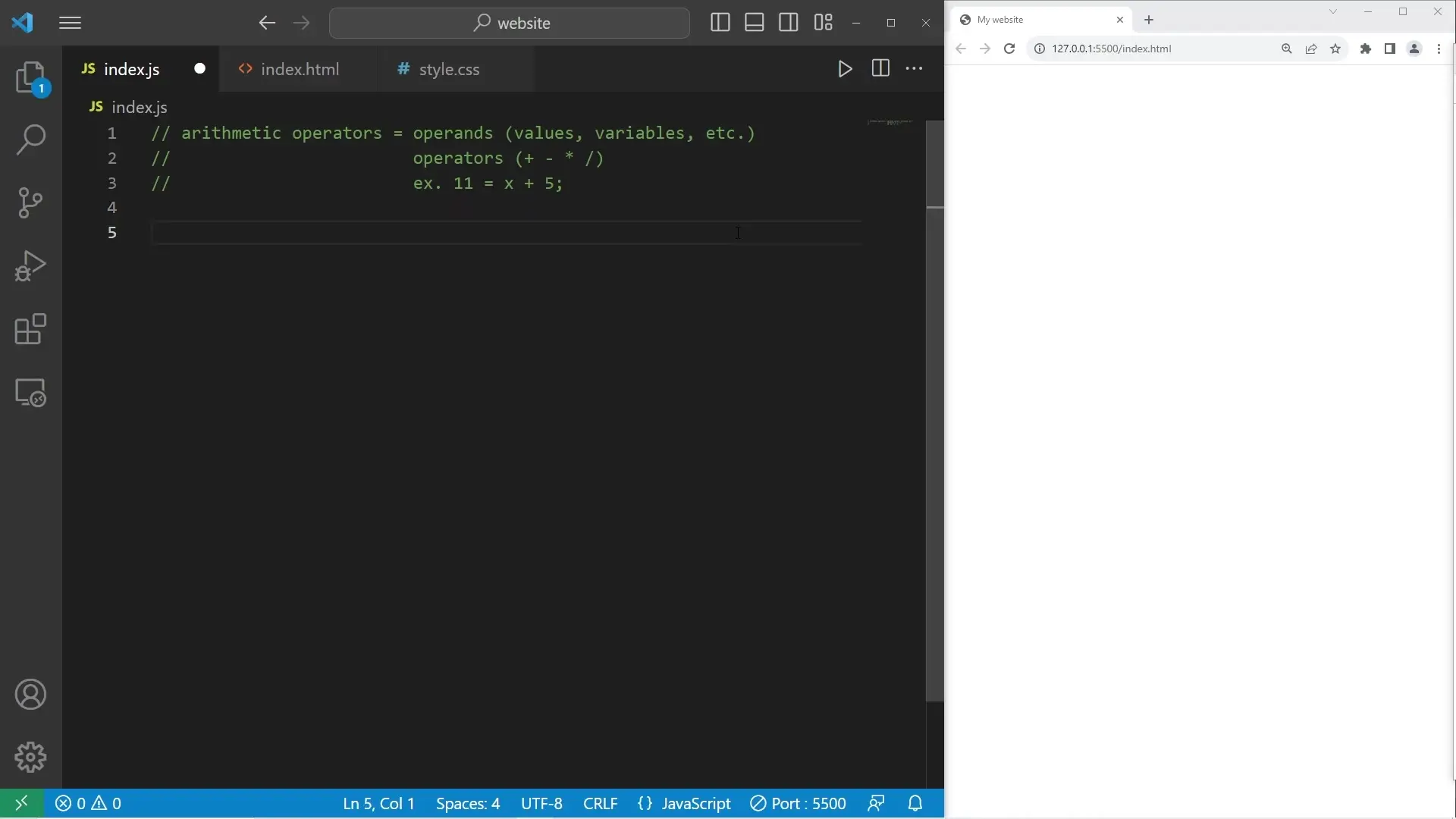
Understanding Operands and Operators
Before diving into specific operators, it's important to understand the terminology. In an arithmetic expression, operands are the values or variables being operated upon, while operators are the symbols that perform the action.
For example, in the expression `11 + 5`, the numbers 11 and 5 are operands, and the plus sign is the operator. Let's explore the different arithmetic operators available in JavaScript.
Basic Arithmetic Operators
Let's use a practical classroom scenario to illustrate these operators. Imagine we're tracking the number of students in a class.
// Initialize student count
let students = 30;
console.log(students); // Output: 30
Addition (+)
The addition operator adds values together. If a new student joins the class:
students = students + 1;
console.log(students); // Output: 31
Subtraction (-)
The subtraction operator subtracts one value from another. If a student leaves the class:
students = students - 1;
console.log(students); // Output: 30
Multiplication (*)
The multiplication operator multiplies values. If we need to double the class size:
students = students * 2;
console.log(students); // Output: 60
Division (/)
The division operator divides one value by another. If we split the class in half:
students = students / 2;
console.log(students); // Output: 30
Exponentiation (**)
The exponentiation operator raises the first operand to the power of the second operand:
students = students ** 2;
console.log(students); // Output: 900 (30²)
Modulus (%)
The modulus operator returns the remainder after division. This is particularly useful for determining if a number is even or odd, or for cycling through a range of values.
// Check if we can divide students evenly into groups of 2
let extraStudents = students % 2;
console.log(extraStudents); // Output: 0 (30 divides evenly by 2)
// Check if we can divide students evenly into groups of 3
extraStudents = students % 3;
console.log(extraStudents); // Output: 0 (30 divides evenly by 3)
// With 31 students, check remainder when dividing by 3
students = 31;
extraStudents = students % 3;
console.log(extraStudents); // Output: 1 (31 ÷ 3 = 10 with remainder 1)
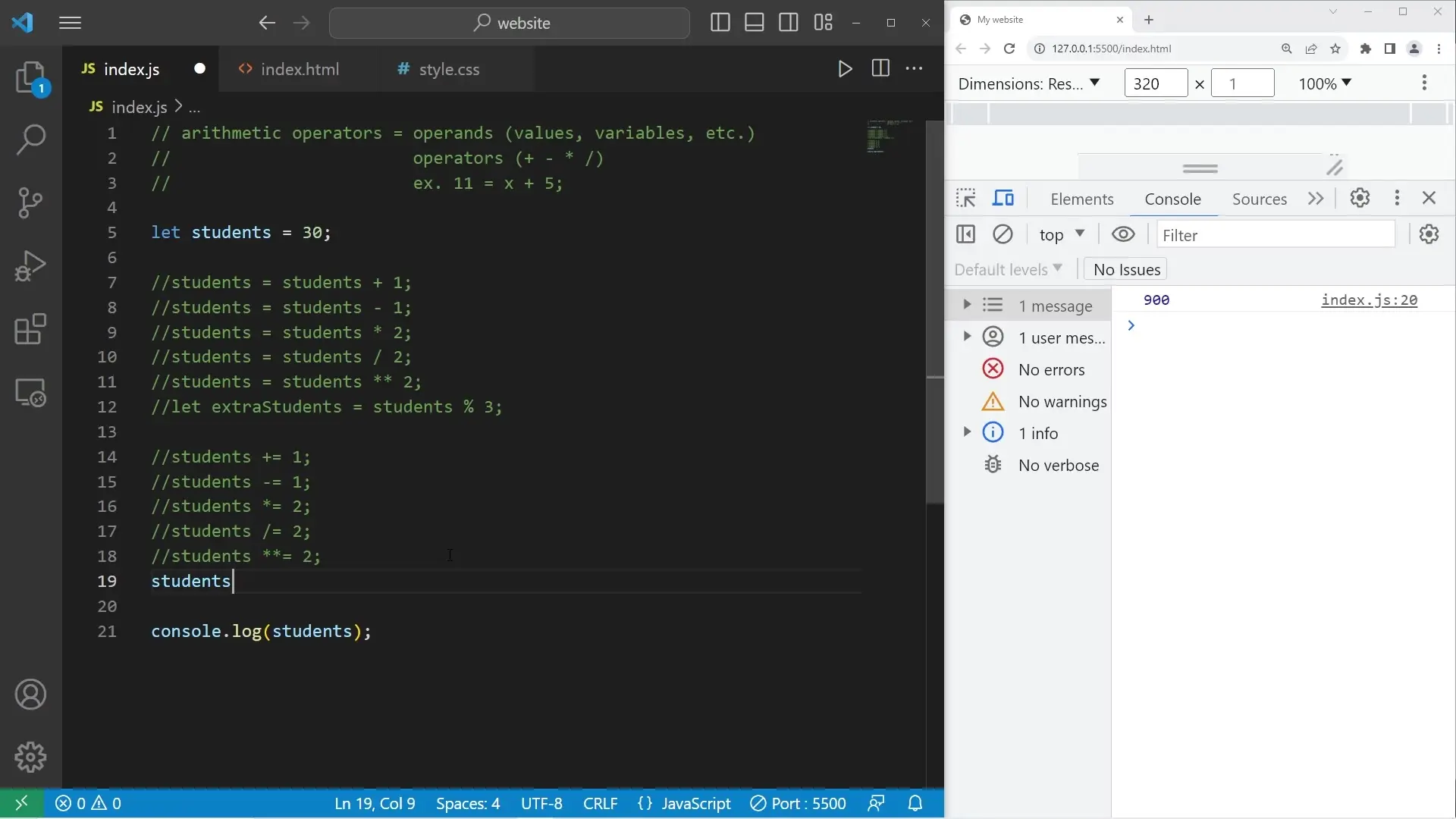
Augmented Assignment Operators
JavaScript provides shorthand operators that combine an arithmetic operation with assignment. These are more concise and often more readable than writing out the full expression.
- Addition assignment (+=): students += 1 is equivalent to students = students + 1
- Subtraction assignment (-=): students -= 1 is equivalent to students = students - 1
- Multiplication assignment (*=): students *= 2 is equivalent to students = students * 2
- Division assignment (/=): students /= 2 is equivalent to students = students / 2
- Exponentiation assignment (**=): students **= 2 is equivalent to students = students ** 2
- Modulus assignment (%=): students %= 2 is equivalent to students = students % 2
// Reset student count
let students = 30;
// Add 2 students
students += 2;
console.log(students); // Output: 32
// Remove 1 student
students -= 1;
console.log(students); // Output: 31
// Double the class size
students *= 2;
console.log(students); // Output: 62
// Divide the class in half
students /= 2;
console.log(students); // Output: 31
Increment and Decrement Operators
For the common case of adding or subtracting exactly 1, JavaScript provides even more concise operators:
- Increment (++): Increases a value by 1
- Decrement (--): Decreases a value by 1
// Reset student count
let students = 30;
// Add one student
students++;
console.log(students); // Output: 31
// Remove one student
students--;
console.log(students); // Output: 30
These operators can be used in prefix (++students) or postfix (students++) form, which affects their behavior when used in expressions. The prefix form returns the value after incrementing, while the postfix form returns the original value before incrementing.
Operator Precedence
When an expression contains multiple operators, the order in which they are evaluated is determined by operator precedence. Understanding this precedence is crucial for writing correct mathematical expressions.
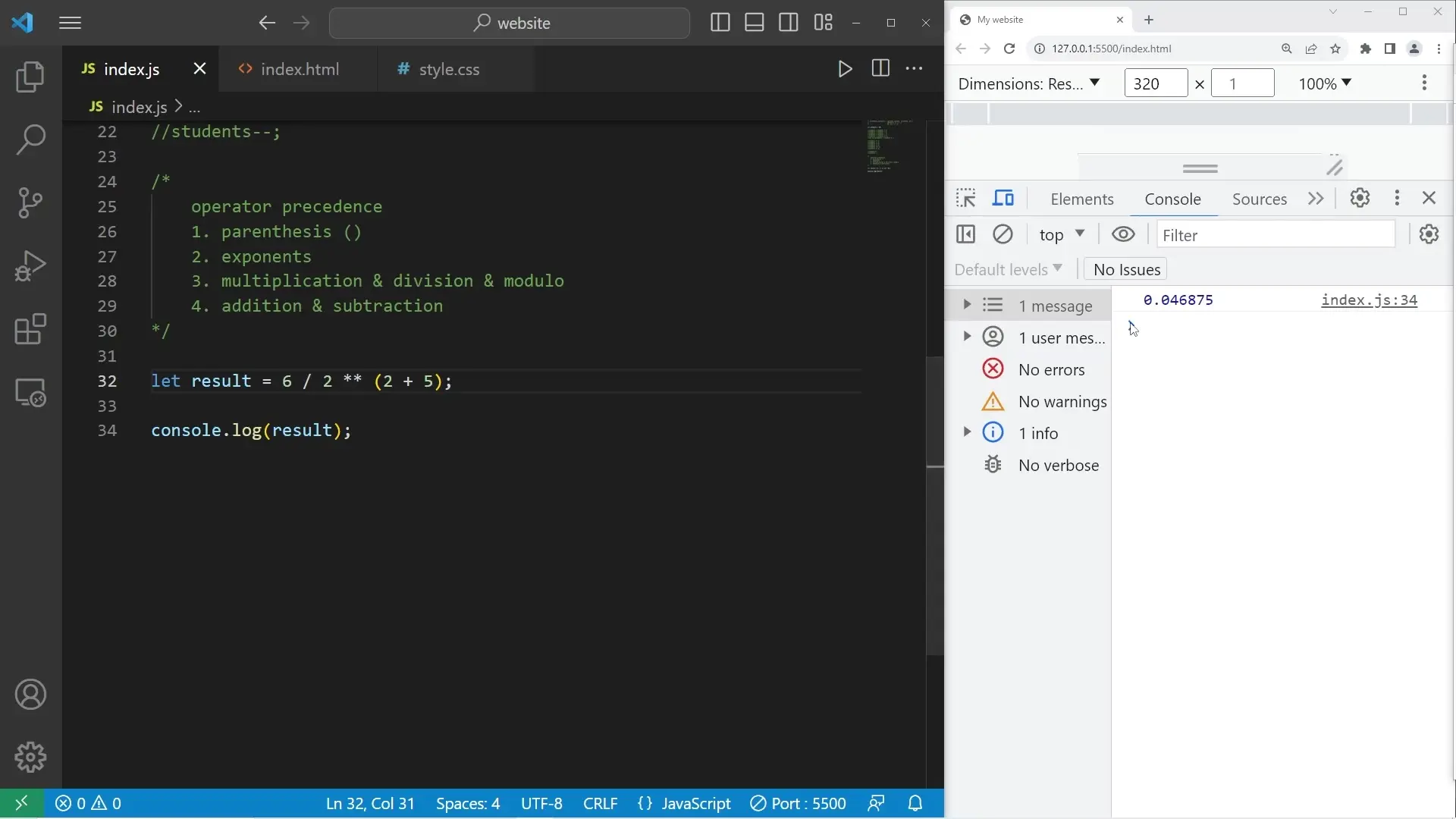
JavaScript follows the standard mathematical order of operations, which can be remembered with the acronym PEMDAS:
- Parentheses: Expressions inside parentheses are evaluated first
- Exponents: Exponentiation operations are evaluated next
- Multiplication, Division, and Modulus: These operations are evaluated from left to right
- Addition and Subtraction: These operations are evaluated last, from left to right
Let's analyze some examples to understand operator precedence:
// Example 1
let result = 1 + 2 * 3 + 4 ** 2;
console.log(result); // Output: 23
// Step by step:
// 1. 4 ** 2 = 16 (exponentiation first)
// 2. 2 * 3 = 6 (multiplication next)
// 3. 1 + 6 + 16 = 23 (addition last)
// Example 2
result = 8 / 2 + 12 % 5;
console.log(result); // Output: 6
// Step by step:
// 1. 8 / 2 = 4 (division)
// 2. 12 % 5 = 2 (modulus, remainder of 12 ÷ 5)
// 3. 4 + 2 = 6 (addition last)
// Example 3
result = 6 / (2 + 5) ** 2;
console.log(result); // Output: 0.0468
// Step by step:
// 1. (2 + 5) = 7 (parentheses first)
// 2. 7 ** 2 = 49 (exponentiation next)
// 3. 6 / 49 = 0.0468 (division last)
Practical Applications
Understanding arithmetic operators is essential for many common programming tasks:
- E-commerce: Calculating totals, taxes, and discounts
- Data visualization: Scaling and normalizing data
- Game development: Calculating scores, positions, and physics
- Form validation: Verifying numerical inputs and ranges
- Responsive design: Calculating dimensions and positions
Best Practices
- Use parentheses to make complex expressions more readable, even when not strictly necessary
- Be aware of potential issues with floating-point arithmetic in JavaScript
- Consider using the modulus operator for tasks like alternating behaviors or cycling through arrays
- Choose augmented assignment operators for better readability when reassigning variables
- Remember that operator precedence can affect the result of expressions
Conclusion
Arithmetic operators are fundamental building blocks in JavaScript programming. By understanding how to use these operators effectively, you'll be better equipped to write clean, efficient code for a wide range of applications. Whether you're performing simple calculations or building complex mathematical models, these operators provide the tools you need to manipulate numerical data in your programs.
As you continue your JavaScript journey, you'll find these operators appearing in virtually every program you write. Mastering them now will provide a solid foundation for more advanced programming concepts.
Let's Watch!
Master JavaScript Arithmetic Operators: Essential Guide for Beginners
Neural Knowledge Pathways
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence