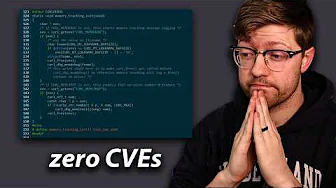
In the realm of software development, creating secure applications in C can seem like a daunting challenge. C, while powerful and versatile, is notorious for its lack of memory safety guarantees, leading to vulnerabilities that plague many applications. However, curl—the ubiquitous command-line utility for making server requests used in billions of installations worldwide—stands as a remarkable exception with its impressive security track record.
Curl's codebase, comprising nearly 180,000 lines of C89 code, has achieved something extraordinary: over the past five years, it has received no critical vulnerability reports and only two vulnerabilities rated as high severity. This achievement is particularly notable considering the tool's widespread deployment and the inherent challenges of C programming.
The Memory Safety Challenge in C
Before diving into curl's secure coding principles, it's important to understand the fundamental challenge: C is not memory safe. Unlike languages such as Rust that provide memory safety guarantees, C allows programmers to allocate memory buffers and potentially access memory outside those buffers, leading to security vulnerabilities.
Industry data suggests that 60-70% of security vulnerabilities across major software projects stem from memory safety issues. Remarkably, curl has managed to reduce this figure to approximately 40% in its own codebase—still significant but substantially better than the industry average.
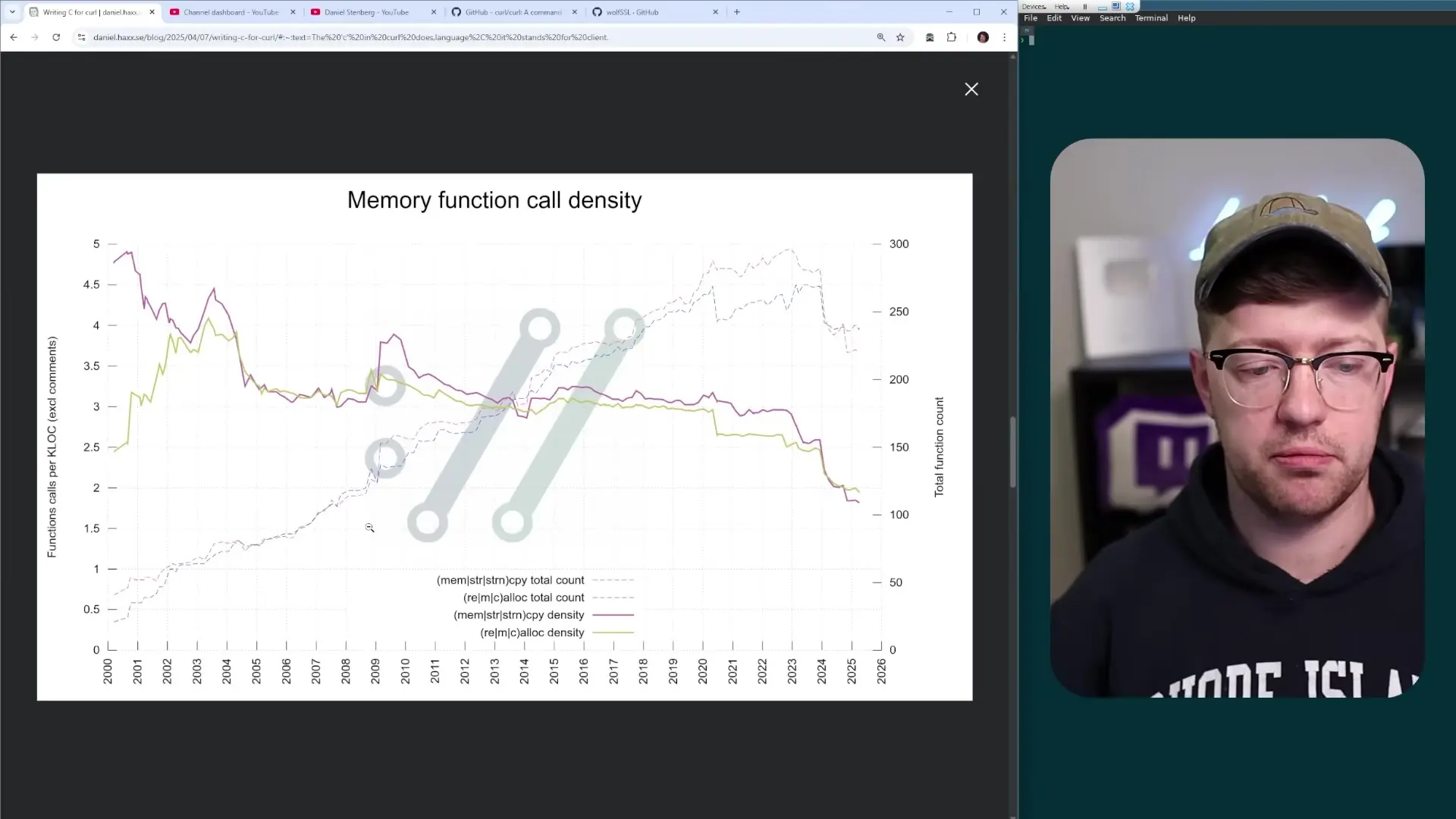
This achievement isn't accidental. It stems from a deliberate set of secure coding principles that guide curl's development. Let's explore these principles that have made curl exceptionally reliable despite using a language prone to security issues.
Principle 1: Code Readability Above All
The first and perhaps most fundamental principle in curl's approach to secure C programming is prioritizing readability. Code that's easy to read is easier to audit for security issues and less likely to contain subtle bugs that lead to vulnerabilities.
Curl's codebase avoids clever constructs, fancy macros, and overloading that might obfuscate the code's intent. The emphasis is on clear, straightforward code where function names accurately reflect their purpose, and the contract of each function is immediately apparent from its declaration and documentation.
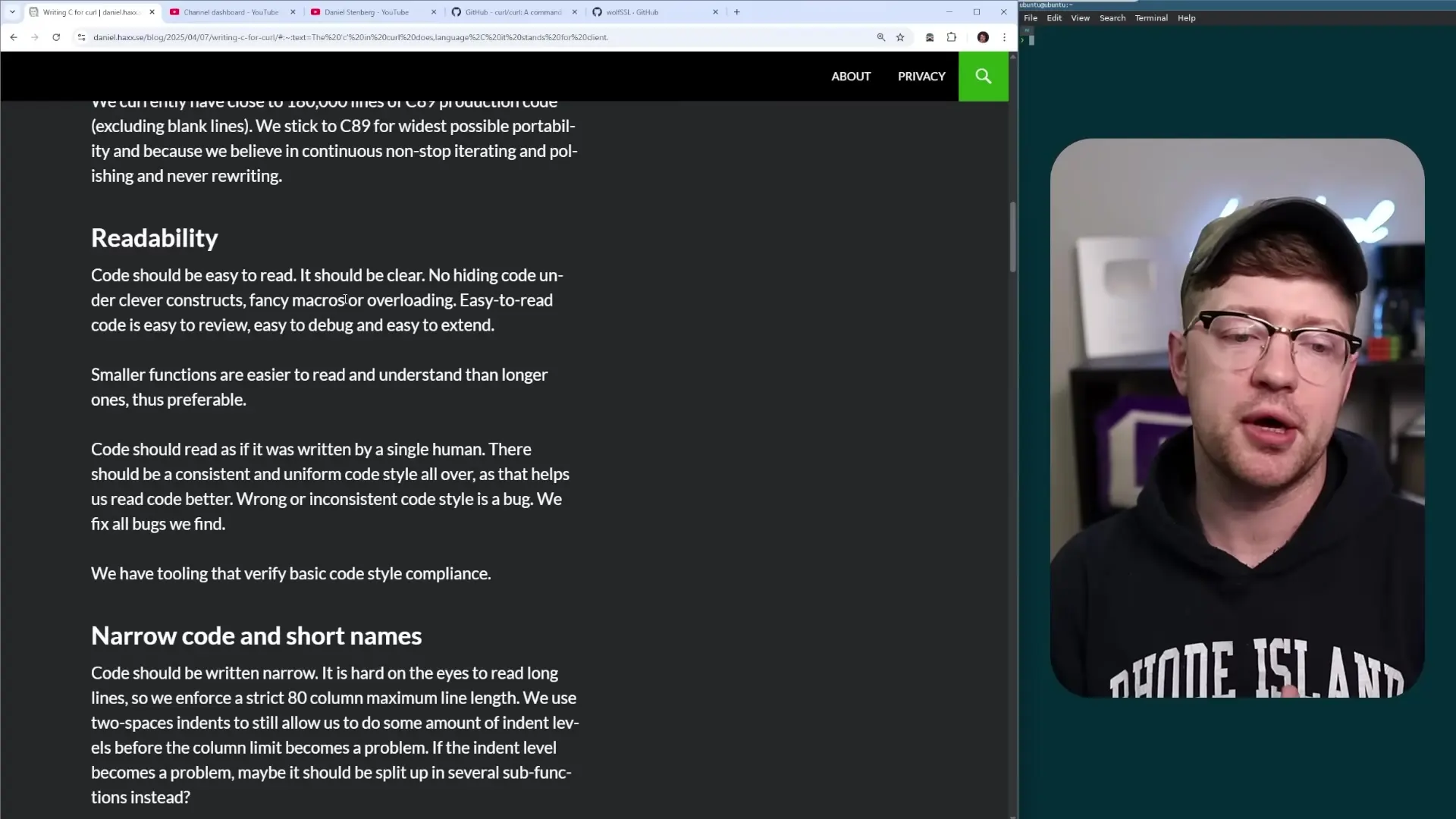
When security auditors or new contributors examine the code, this readability principle ensures they can quickly understand what each piece of code does without making assumptions about its behavior—a critical factor in identifying potential security issues.
Principle 2: Consistent, Uniform Code Style
Curl treats inconsistent code style as a bug that must be fixed. The codebase should read as if written by a single person, with uniform formatting and conventions throughout. This consistency makes it easier to spot anomalies that might indicate security issues.
To enforce this principle, curl employs automated tooling that verifies code style compliance. This isn't mere pedantry—it's a security measure that ensures the codebase remains understandable to all contributors and auditors, reducing the risk of misunderstandings that could lead to vulnerabilities.
/* Example of curl's consistent style */
CURLcode function_name(struct Curl_easy *data, void *ptr)
{
/* Two-space indentation */
if (!data)
return CURLE_BAD_FUNCTION_ARGUMENT;
/* Clear variable names */
int result = do_something(ptr);
return result ? CURLE_OK : CURLE_FAILED_INIT;
}
Principle 3: Small Functions with Clear Purpose
In secure C programming, smaller functions are generally safer than larger ones. They're easier to understand, test, and verify for correctness. Curl embraces this principle wholeheartedly, preferring numerous small functions over fewer large ones.
Each function should do one thing and do it well. If a function starts to grow complex or handle multiple responsibilities, it's a sign that it should be broken down into smaller, more focused functions. This approach not only improves readability but also makes it easier to reason about the code's behavior and identify potential security issues.
- Functions should have a single responsibility
- Function names should clearly indicate what they do
- Complex operations should be broken down into simpler sub-functions
- Deep nesting of control structures is a sign that a function should be refactored
Principle 4: Narrow Code with Short Names
Curl enforces a strict 80-column maximum line length throughout its codebase. This might seem like an archaic limitation dating back to terminal width constraints, but it serves several important security purposes.
First, it naturally limits the complexity of code that can fit on a single line. Second, it discourages deeply nested control structures, which can be difficult to reason about. Third, it encourages breaking complex operations into smaller, more manageable pieces.
To accommodate this narrow code style while maintaining readability, curl uses two-space indentation and favors short, descriptive variable names. If indentation levels become a problem due to the column limit, it's taken as a sign that the function should be split into smaller sub-functions.
Principle 5: Warning-Free Compilation
Another cornerstone of curl's secure coding approach is ensuring the codebase compiles without warnings when using strict compiler settings. Compiler warnings often indicate potential issues that could lead to security vulnerabilities, so curl treats them as errors that must be addressed.
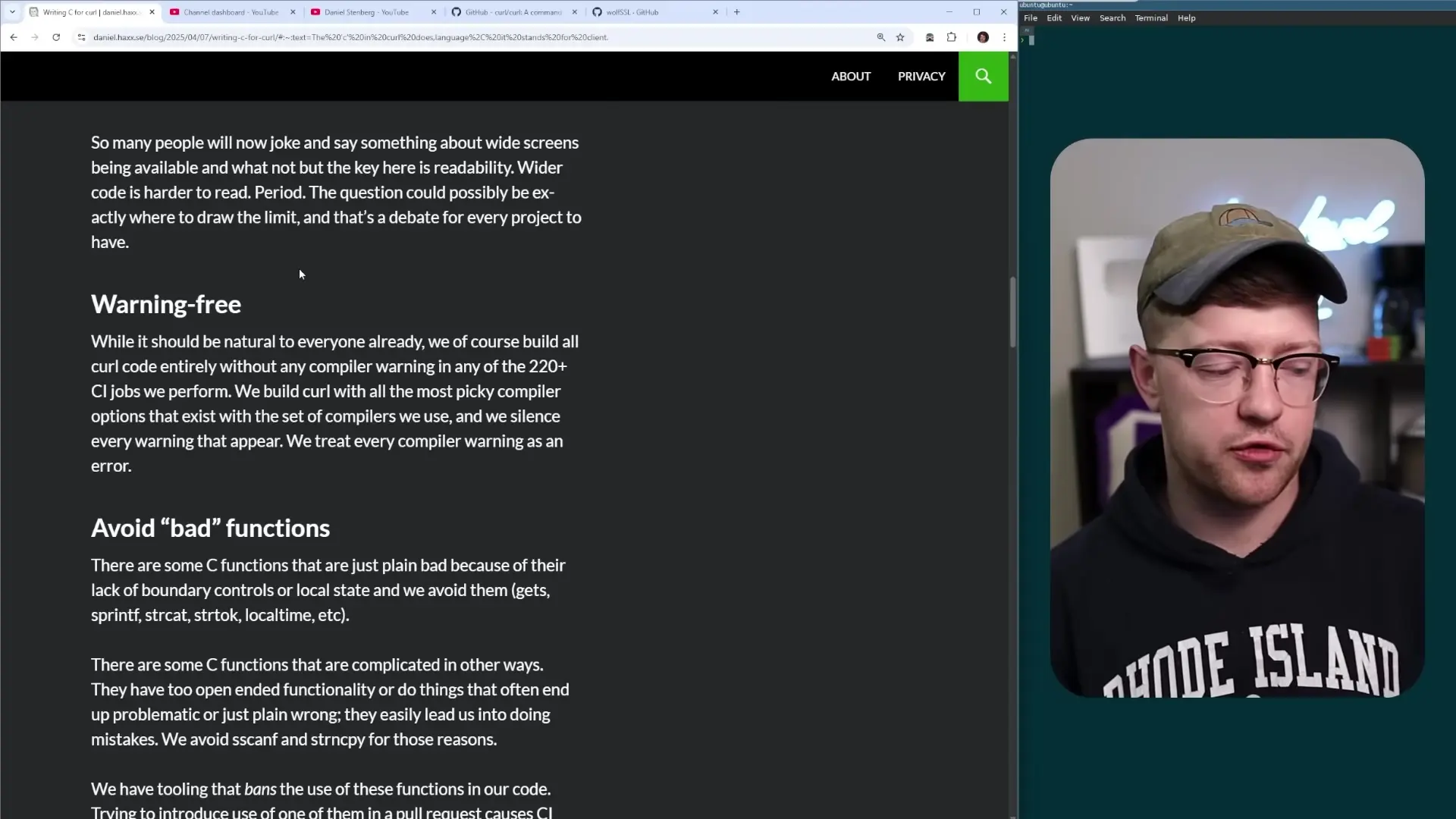
This principle extends beyond just fixing warnings—it involves proactively using compiler features and static analysis tools to identify potential issues before they become problems. By leveraging these tools and treating their output seriously, curl catches many potential security issues during development rather than after deployment.
Principle 6: Avoiding Unsafe Functions
C's standard library includes several notoriously unsafe functions that are common sources of security vulnerabilities. Functions like strcpy(), sprintf(), and gets() can easily lead to buffer overflows if not used with extreme caution.
Curl systematically avoids these unsafe functions, preferring safer alternatives like strncpy(), snprintf(), and fgets() that include buffer size parameters. Moreover, curl often implements its own wrapper functions that provide additional safety checks beyond what the standard library offers.
/* Avoid unsafe functions like: */
strcpy(dest, src); /* No size checking */
/* Instead use safer alternatives: */
strncpy(dest, src, dest_size);
/* Or even better, use curl's custom safe functions: */
curl_msnprintf(dest, dest_size, "%s", src);
Principle 7: Continuous Iteration Without Rewrites
Rather than pursuing complete rewrites in safer languages, curl follows a philosophy of continuous improvement and iteration. The codebase evolves gradually, with ongoing refinements and polishing rather than revolutionary changes.
This approach recognizes that rewrites often introduce new bugs and security issues, while incremental improvements can steadily enhance security without disrupting stability. It's a pragmatic acknowledgment that perfect security is unattainable, but continuous vigilance and improvement can achieve remarkable results.
- Identify and fix issues promptly when discovered
- Continuously refine code quality through small, targeted improvements
- Learn from past vulnerabilities to prevent similar issues
- Maintain backward compatibility while enhancing security
- Leverage automated testing to prevent regressions
The Results Speak for Themselves
The effectiveness of these secure C programming principles is evident in curl's impressive security record. Despite being written in a language prone to memory safety issues and deployed on billions of systems worldwide, curl has maintained a remarkably clean security record.
Over the past five years, curl has received no reports of critical vulnerabilities and only two vulnerabilities rated as high severity. The remaining 60+ vulnerabilities were classified as low or medium severity—an exceptional achievement for software with such widespread deployment.
While curl's maintainers acknowledge that they occasionally merge security bugs—they're human, after all—their coding principles have demonstrably reduced both the frequency and severity of such issues compared to other C projects of similar scale and complexity.
Conclusion: Secure C Programming Is Challenging But Possible
Curl's experience demonstrates that while C lacks the memory safety guarantees of languages like Rust, disciplined coding practices can significantly reduce security vulnerabilities. The principles outlined above—prioritizing readability, maintaining consistent style, using small functions, enforcing narrow code, eliminating compiler warnings, avoiding unsafe functions, and continuously iterating—provide a roadmap for creating more secure C code.
For developers working with C codebases, these principles offer valuable guidance for enhancing security without undertaking risky rewrites. While memory-safe languages are preferable for new projects, curl shows that existing C codebases can achieve impressive security records through disciplined development practices.
By applying these secure coding principles consistently, C developers can create more robust, secure software that stands the test of time—even when deployed billions of times across the global internet.
Let's Watch!
7 Secure C Programming Principles Behind Curl's Remarkable Safety Record
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence