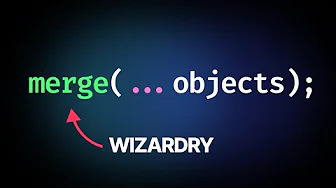
TypeScript developers often struggle with maintaining proper type safety when merging objects. While Object.assign is a powerful JavaScript utility, its TypeScript implementation doesn't preserve the specific property types during merges. In this tutorial, we'll build a truly type-safe alternative to Object.assign that correctly handles property overrides and maintains precise type information.
The Challenge with Object.assign Type Safety
Let's start by examining the problem. When using Object.assign to merge objects in TypeScript, we often lose precise type information. Consider a scenario where we're merging objects with overlapping properties that have different types:
// Objects with overlapping properties of different types
const obj1 = { foo: "string value", bar: "bar value" };
const obj2 = { foo: 42 };
const obj3 = { foo: true };
// Standard Object.assign loses precise type information
const result = Object.assign({}, obj1, obj2, obj3);
// result.foo has type 'string | number | boolean' at best, or 'any' at worst
The challenge is that we want the final type of 'foo' to be just 'boolean' since that's the last value that overrides previous ones. Standard TypeScript typings don't capture this sequential override behavior correctly.
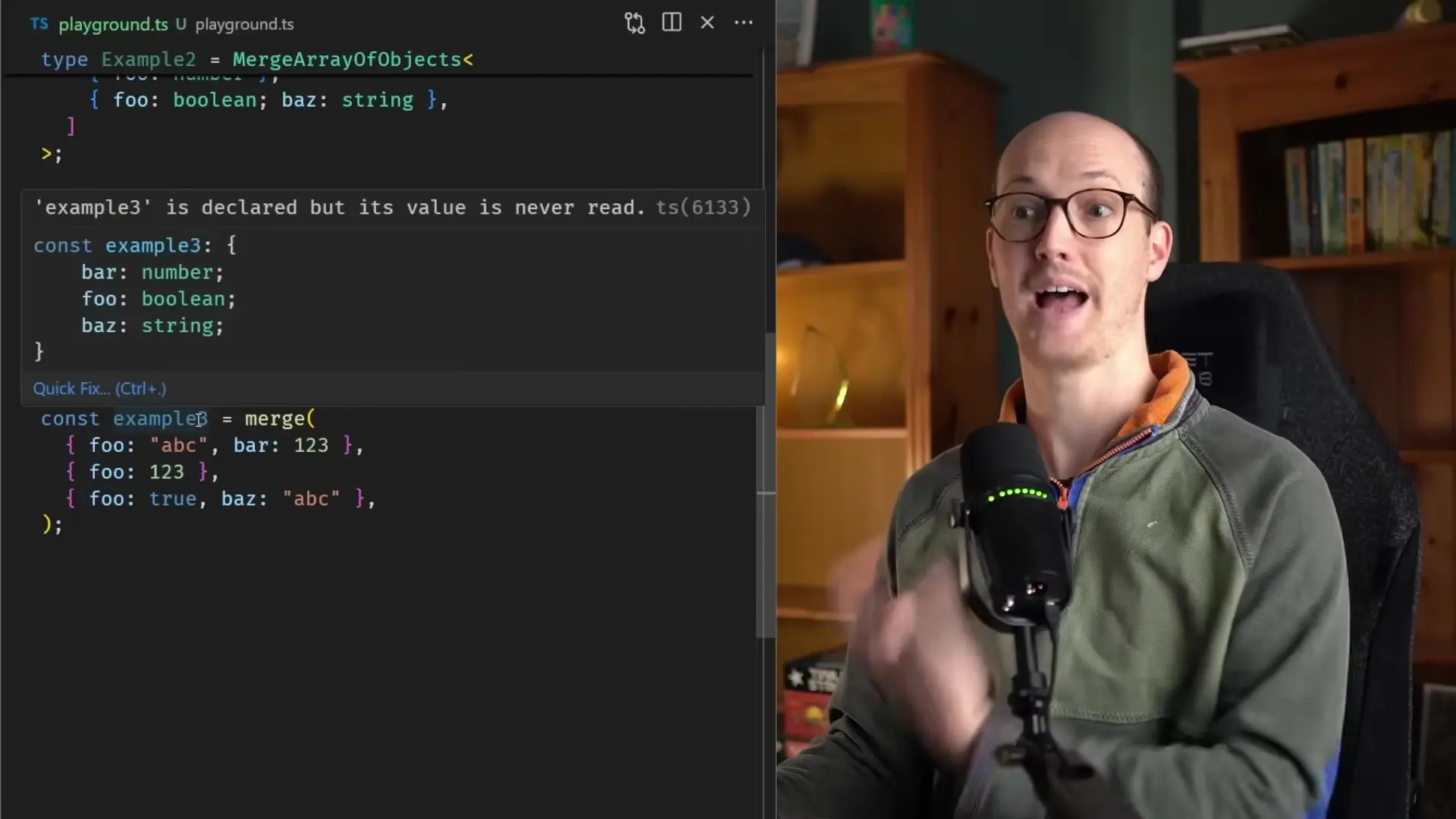
Building a Type-Safe Merge Helper
To solve this problem, we'll create a custom merge type helper that correctly captures the type transformations that happen during object merging. The key insight is using TypeScript's utility types to properly model how properties get overridden.
// Our core merge type helper
type Merge<T1, T2> = Omit<T1, keyof T2> & T2;
// Helper to make the resulting type more readable
type Prettify<T> = {
[K in keyof T]: T[K];
};
// Function that uses our type helpers
function merge<T1 extends object, T2 extends object>(obj1: T1, obj2: T2): Prettify<Merge<T1, T2>> {
return Object.assign({}, obj1, obj2) as Prettify<Merge<T1, T2>>;
}
The `Merge
The `Prettify
Extending to Array of Objects with Recursive Types
While merging two objects is useful, we often need to merge an array of objects. This requires a more sophisticated type helper that can handle an arbitrary number of objects while maintaining type safety throughout the merging process.
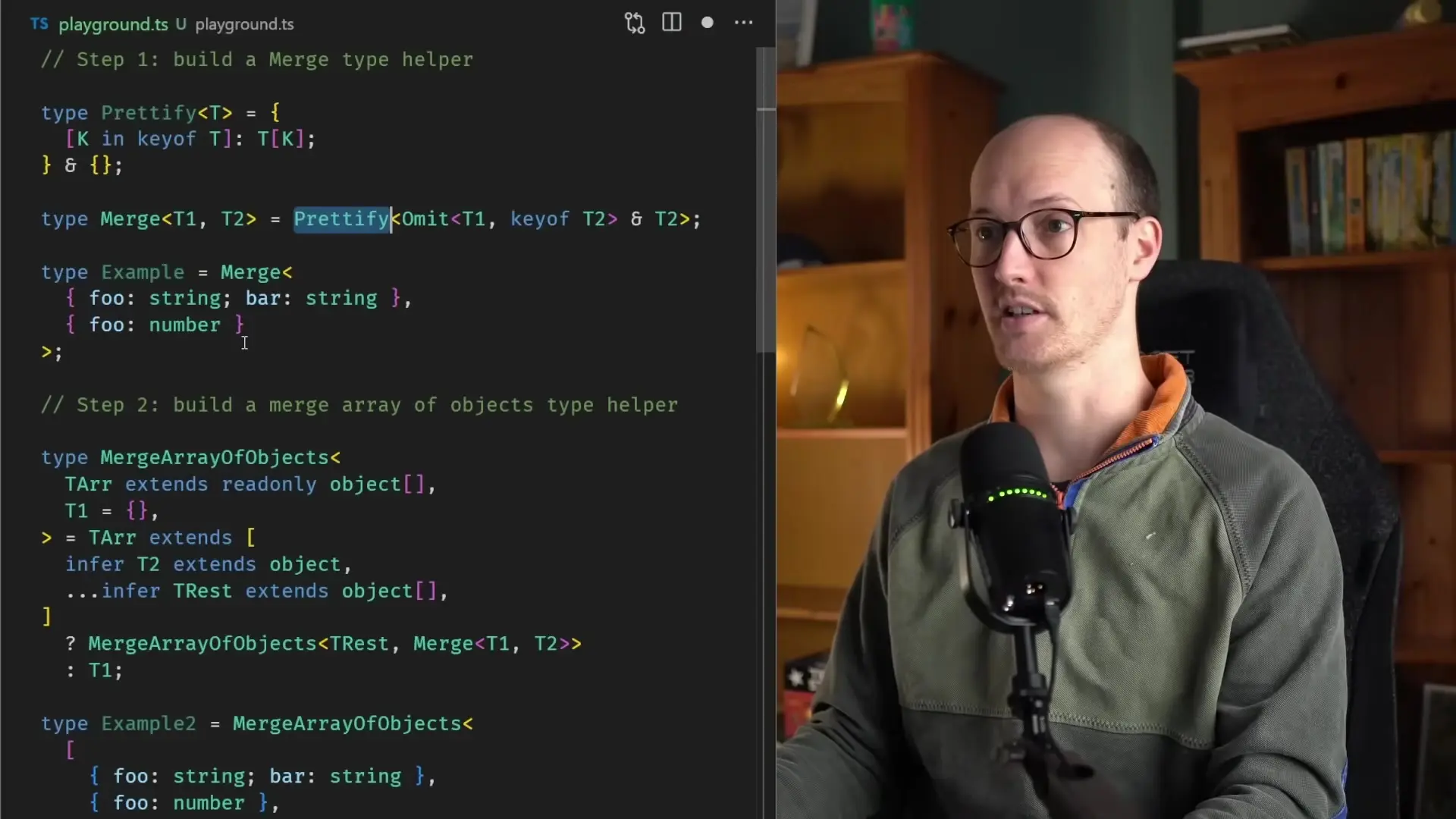
// Recursive type for merging an array of objects
type MergeArrayOfObjects<
T1 extends object,
TR extends readonly object[]
> = TR extends [infer T2 extends object, ...infer Rest extends object[]]
? MergeArrayOfObjects<Merge<T1, T2>, Rest>
: T1;
// Function implementation using our recursive type
function mergeObjects<const T extends readonly object[]>(
...objects: T
): Prettify<MergeArrayOfObjects<{}, T>> {
return Object.assign({}, ...objects) as Prettify<MergeArrayOfObjects<{}, T>>;
}
This recursive type solution is particularly powerful. The `MergeArrayOfObjects` type uses conditional types and inference to process each object in the array sequentially, correctly modeling how Object.assign merges properties from left to right.
The type checks if there are any objects left in the array. If so, it merges the current accumulated object (T1) with the next object in the array (T2), then recursively processes the rest of the array. If there are no objects left, it returns the accumulated object.
Testing Our Type-Safe Object.assign Alternative
Let's test our implementation with the example we started with to verify that it correctly maintains type safety:
const obj1 = { foo: "string value", bar: "bar value" };
const obj2 = { foo: 42 };
const obj3 = { foo: true };
// Using our type-safe merge function
const result = mergeObjects(obj1, obj2, obj3);
// TypeScript correctly infers result.foo as boolean
// result.bar is still string
// If we change the order, the types change accordingly
const result2 = mergeObjects(obj1, obj3, obj2);
// Now result2.foo is correctly inferred as number
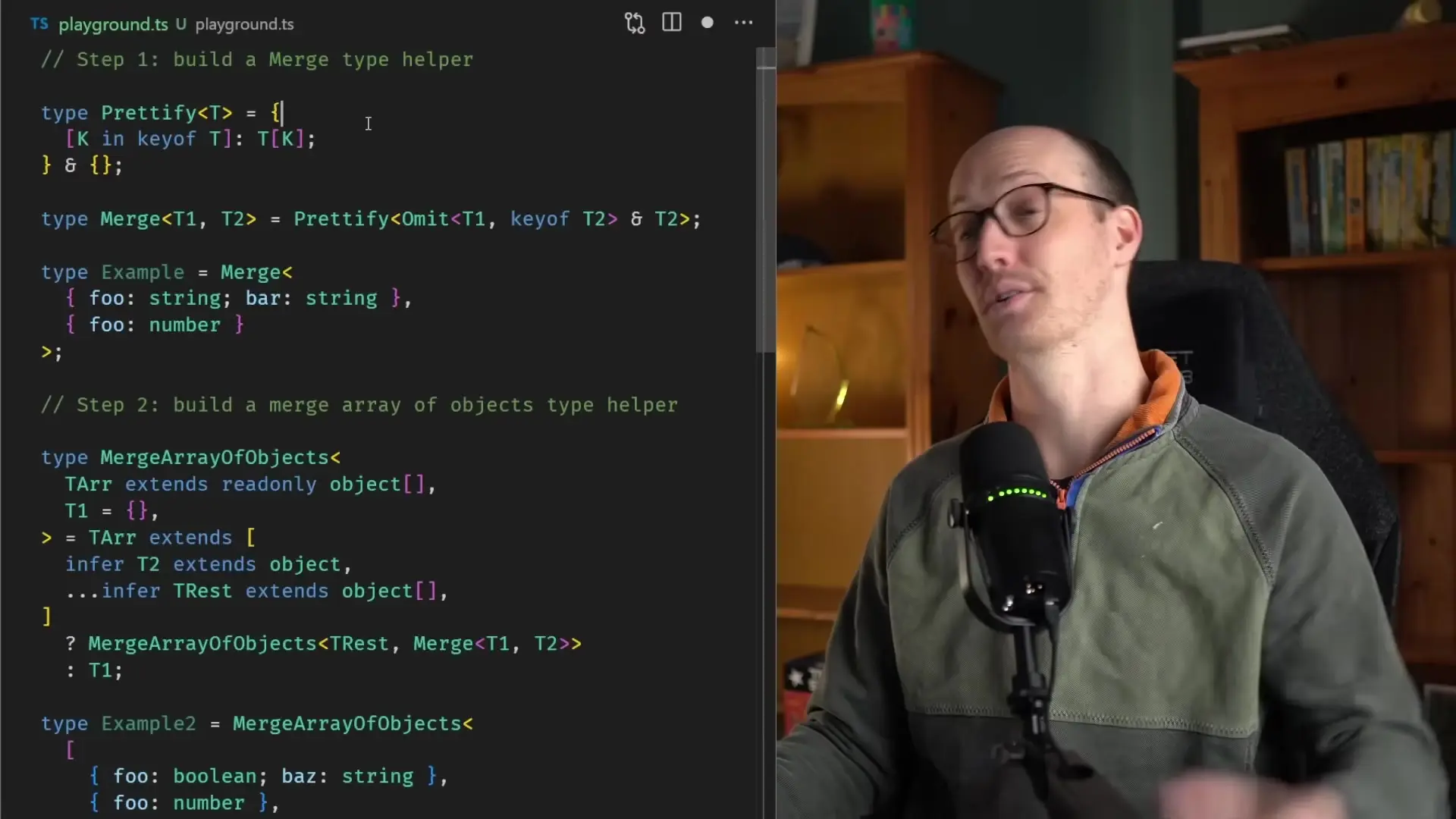
Benefits of Using Type-Safe Object Merging
- Maintains precise type information for all properties after merging
- Correctly models property overrides based on merge order
- Provides better IDE support with accurate autocompletion
- Catches type errors at compile-time rather than runtime
- Makes refactoring safer by ensuring type consistency
Practical Applications
This type-safe object merging approach is particularly useful in several common scenarios:
- Merging configuration objects with proper type checking
- Implementing state updates in React or Redux with precise typing
- Creating utility functions that combine options with defaults
- Building robust APIs that need to merge user input with system defaults
- Enhancing data transformation pipelines with type safety
Conclusion
Creating a type-safe alternative to Object.assign in TypeScript requires advanced generic types and a solid understanding of TypeScript's type system. By using utility types like Omit, conditional types, and recursive type definitions, we've built a robust solution that maintains precise type information throughout the object merging process.
This approach demonstrates the power of TypeScript's type system for creating truly type-safe utilities. The mental model of building generic type helpers and mapping them onto functions provides a template you can follow for creating your own type-safe utilities for other JavaScript functions that lack proper typing.
Next time you need to merge objects in TypeScript, consider using this type-safe approach to ensure your code remains robust and maintainable as your project grows.
Let's Watch!
Creating Type-Safe Object.assign in TypeScript: Advanced Generic Types Tutorial
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence