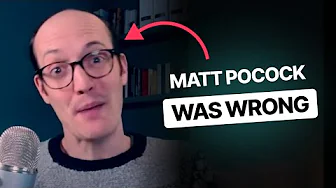
The debate over types vs interfaces in TypeScript has been ongoing since the language's inception. As TypeScript has evolved, so has our understanding of when to use each feature. This article examines the performance implications and practical differences between types and interfaces, helping you make informed decisions in your TypeScript projects.
The Interface vs Type Performance Myth
A common misconception in the TypeScript community is that interfaces are inherently faster than types for defining objects. This misunderstanding often stems from a misinterpretation of the TypeScript performance documentation.
The truth is that when it comes to simple object definitions, there's virtually no performance difference between using a type alias or an interface. The TypeScript compiler processes them with similar efficiency.
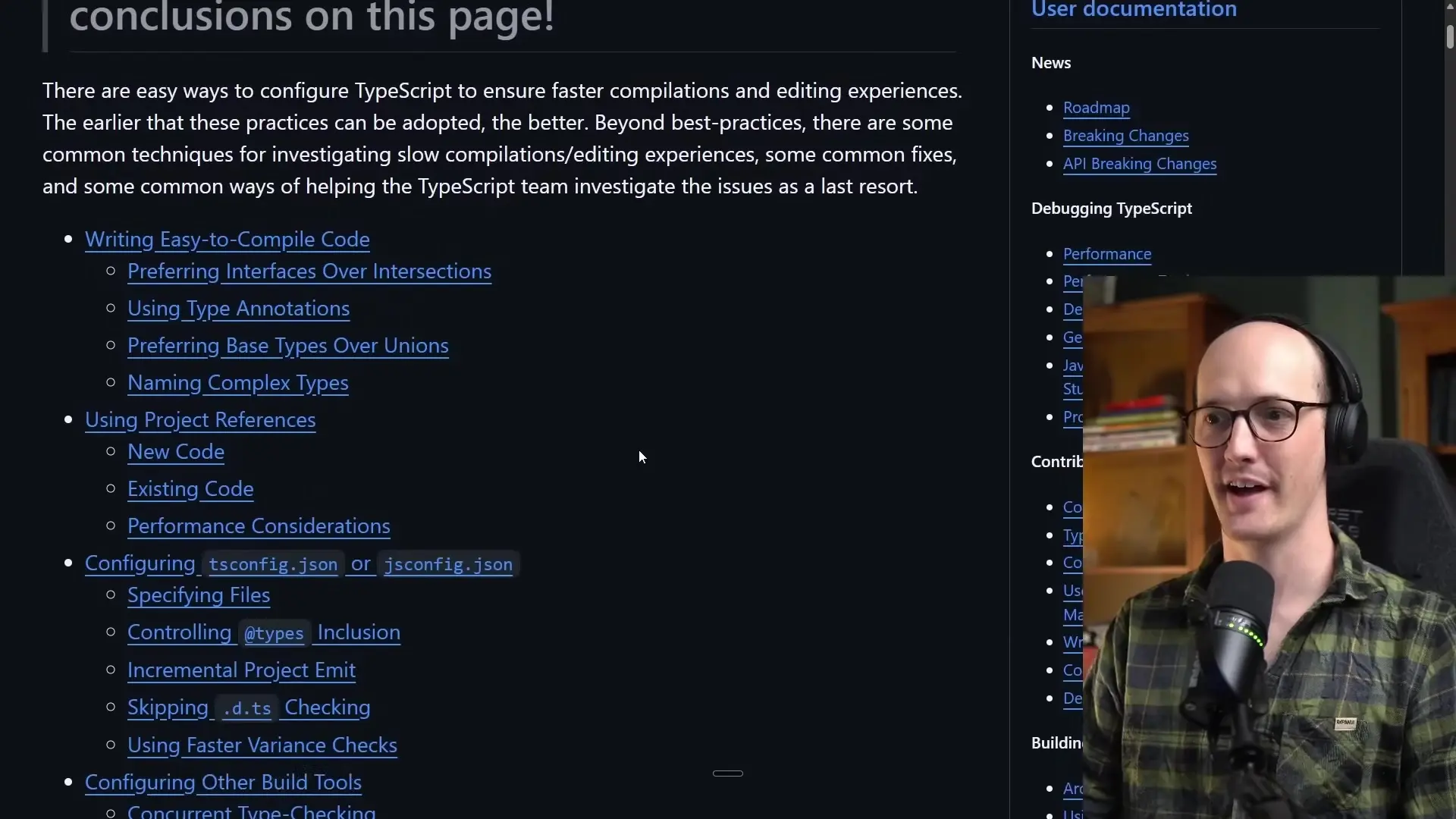
Interface Extensions vs Type Intersections: The Real Performance Story
Where performance differences do emerge is when comparing interface extension with type intersections. The TypeScript documentation specifically recommends using interface extension over type intersections for better compile-time performance.
// Type intersection approach (potentially slower)
type Bar = { id: string; /* other properties */ };
type Baz = { id: number; /* other properties */ };
type Foo = Bar & Baz & { someProp: string };
// Interface extension approach (faster)
interface Bar { id: string; /* other properties */ }
interface Baz { id: number; /* other properties */ }
interface Foo extends Bar, Baz { someProp: string }
The key difference is in how TypeScript handles type checking. When using interface extension, TypeScript can check compatibility at each inheritance level, catching errors early. With type intersections, TypeScript must compute the full combined type before detecting incompatibilities.
Real-World Impact: The Button Component Example
In large React applications, this performance difference can become significant. Consider a common pattern where you extend HTML button properties with custom ones:
// Potentially slower approach using type intersection
type CustomButtonProps = React.ButtonHTMLAttributes<HTMLButtonElement> & {
customProp: string;
};
// Faster approach using interface extension
interface CustomButtonProps extends React.ButtonHTMLAttributes<HTMLButtonElement> {
customProp: string;
}
Companies with large TypeScript codebases have reported significant compile-time improvements after converting type intersections to interface extensions.
Interface Declaration Merging: Feature or Bug?
One unique feature of interfaces is declaration merging - the ability to add properties to an interface by declaring it multiple times. While this enables some advanced patterns, it can also lead to unexpected behaviors and hard-to-track bugs.
// First declaration
interface Animal {
name: string;
}
// Second declaration - these properties get merged with the first
interface Animal {
meow(): void;
}
// The effective type is now both combined
const cat: Animal = {
name: "Whiskers",
meow: () => console.log("Meow!")
};
In large codebases, unintentional declaration merging can create confusing type errors. You can mitigate this risk by enabling the ESLint rule 'no-unsafe-declaration-merging', which will flag potential issues while still allowing intentional merging when needed.
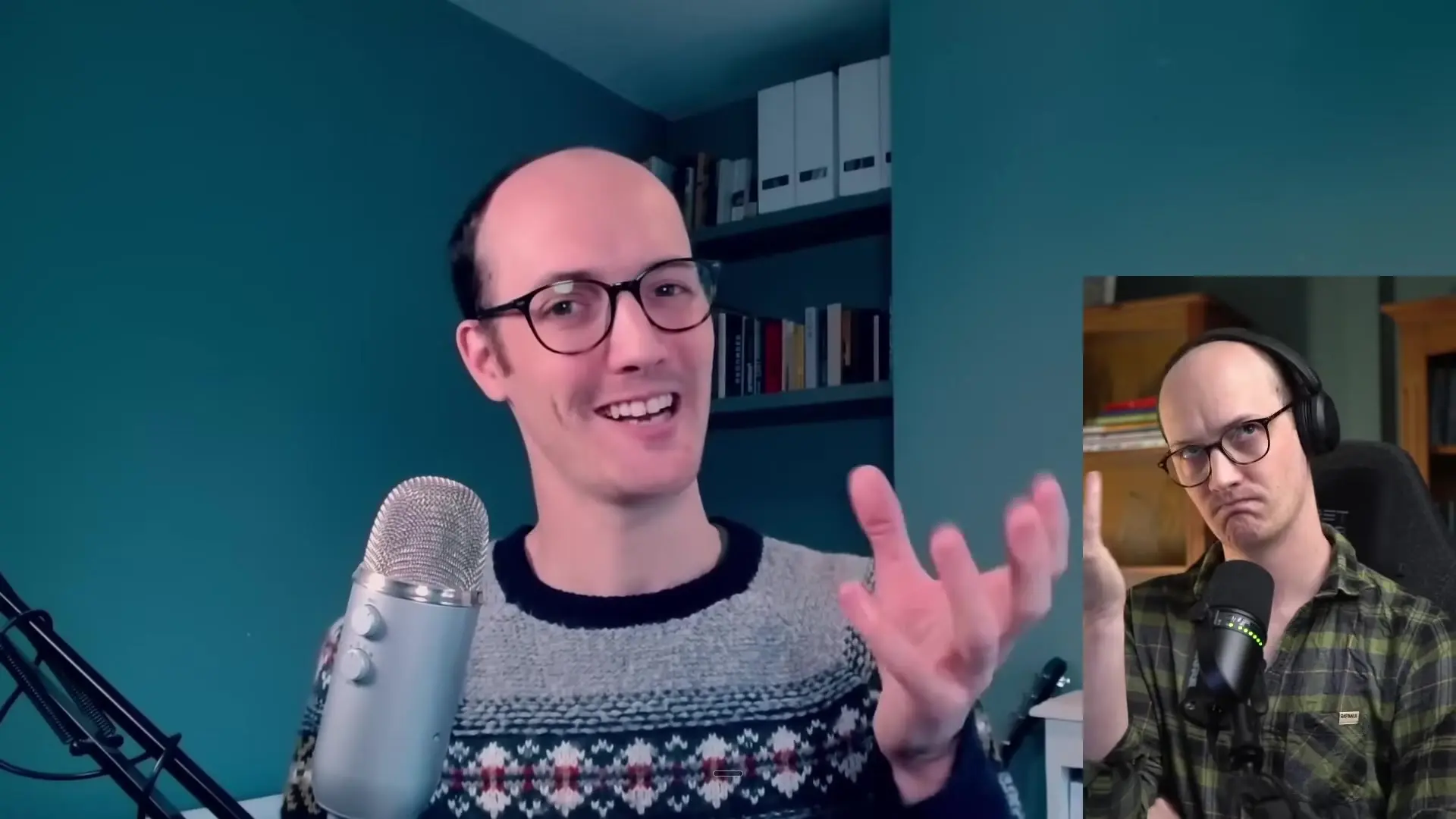
The Surprising Behavior of Index Signatures
Another key difference between types and interfaces appears when working with index signatures and record types. Type aliases have an implicit index signature behavior that interfaces don't share.
// Interface definition
interface KnownAttributes {
x: number;
y: number;
}
// Record type definition
type NumberRecord = Record<string, number>;
const coords: KnownAttributes = { x: 10, y: 20 };
// This fails! Interface doesn't implicitly match Record
const test1: NumberRecord = coords; // Error!
// Using type instead resolves the issue
type KnownAttributesType = {
x: number;
y: number;
};
const coords2: KnownAttributesType = { x: 10, y: 20 };
const test2: NumberRecord = coords2; // Works!
This behavior exists because interfaces could potentially be extended through declaration merging with properties that don't match the index signature. Type aliases, being closed definitions, don't have this concern.
When to Use Types vs Interfaces in TypeScript: Practical Guidelines
Based on these insights, here are practical guidelines for choosing between types and interfaces in your TypeScript projects:
- Use interfaces when extending or implementing complex object types, especially in large codebases where compile performance matters
- Use interfaces for public API definitions that might need to be extended by consumers
- Use types for unions, intersections, mapped types, and other advanced type manipulations
- Use types when you need to ensure your type definition can't be modified through declaration merging
- Use types when working with record types and index signatures for more predictable behavior
TypeScript Class vs Type vs Interface: Understanding the Differences
While this article focuses primarily on types vs interfaces, it's worth noting how classes fit into the picture. Classes in TypeScript are both value-level and type-level constructs, while types and interfaces exist only at the type level.
// Interface (type-level only)
interface User {
name: string;
greet(): void;
}
// Type alias (type-level only)
type UserType = {
name: string;
greet(): void;
};
// Class (both type-level and value-level)
class UserClass {
name: string;
constructor(name: string) {
this.name = name;
}
greet() {
console.log(`Hello, ${this.name}`);
}
}
Classes should be used when you need both the type checking and the runtime implementation. Interfaces and type aliases are erased during compilation and exist purely for development-time type checking.
Conclusion: The Evolving Best Practices
The TypeScript ecosystem continues to evolve, and with it, our understanding of best practices. While the performance differences between types and interfaces for simple object definitions are negligible, the way they behave in complex type systems can have significant implications for both compile-time performance and developer experience.
The most pragmatic approach is to use types as your default choice for most situations, switching to interfaces when you specifically need interface features like declaration merging or when you're extending complex object types in performance-sensitive codebases. This balanced approach gives you the best of both worlds while avoiding the pitfalls of each.
As TypeScript continues to improve its performance through initiatives like the Go-based compiler rewrite, some of these distinctions may become less important. However, understanding the underlying mechanics will always help you write more maintainable and efficient TypeScript code.
Let's Watch!
Types vs Interfaces in TypeScript: Critical Performance Insights for Developers
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence