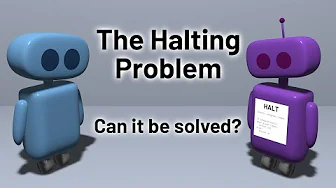
Have you ever experienced a program freezing on your computer and wondered if it would eventually respond or if it was stuck in an infinite loop? This common frustration touches on one of the most profound concepts in computer science: the halting problem. This article explores what the halting problem is, why it's fundamentally unsolvable, and what this means for software development.
What is the Halting Problem?
The halting problem asks a seemingly simple question: Can we create a program that can determine, for any arbitrary program and input, whether that program will eventually terminate (halt) or run forever? In other words, can we build a universal program analyzer that can predict if any given program will finish running or get stuck in an infinite loop?
This question has profound implications for computing. If such a program existed, we could automatically detect infinite loops, deadlocks, and other non-terminating behaviors before they occur. Unfortunately, as we'll see, this is provably impossible.
Understanding the Halting Problem with Examples
Let's consider a simple program called "walk" to understand the concept better:
function walk(input):
steps = 0
while steps != input:
moveForward()
turnRight()
steps = steps + 1
return
If we run this program with the input 4, it will perform four iterations of the loop (moving, turning, and incrementing the step counter) and then terminate. However, if we run it with the input -1, the program will never halt because the step counter (which starts at 0 and only increases) can never equal -1.
This is a simple halting problem example where we, as humans, can determine whether the program will halt for specific inputs. But can we create a general algorithm that works for any program?
The Hypothetical Halt Program
Let's imagine we could create a function called "halt" that solves the halting problem:
function halt(program, input):
// Analyzes the program and its input
// Returns TRUE if the program will eventually halt
// Returns FALSE if the program will run forever
If such a function existed, it would be incredibly useful. We could use it to detect infinite loops, ensure programs terminate, and solve many other computational problems.
Why is the Halting Problem Unsolvable? The Proof
To understand why the halting problem is unsolvable, we'll use a technique called proof by contradiction. We'll assume that a solution exists and then show that this assumption leads to a logical contradiction.
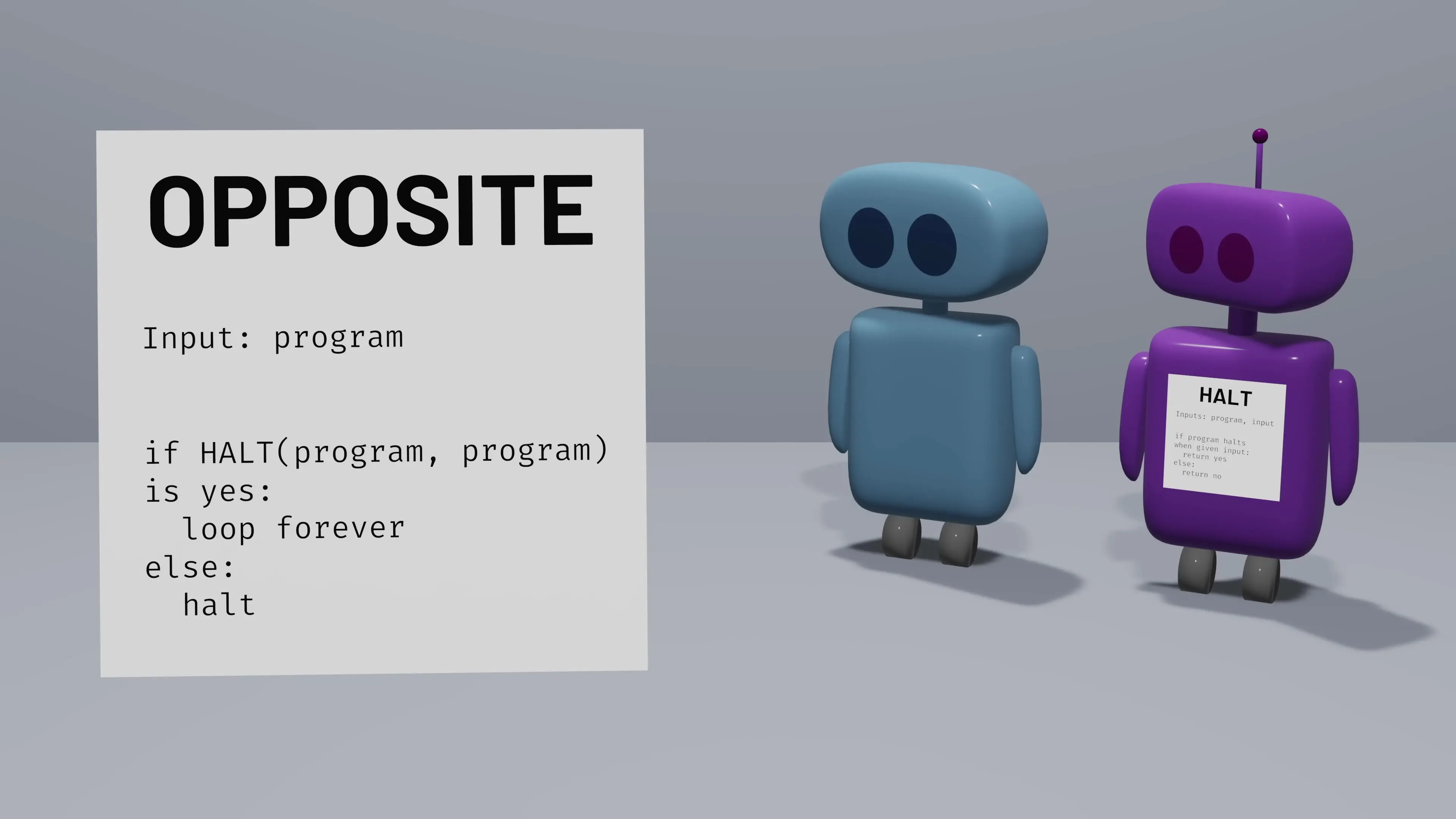
If we assume our "halt" function exists, we could create another program called "opposite" that does the following:
function opposite(program):
if halt(program, program) == TRUE:
// If halt says the program would terminate when given itself as input
while TRUE:
// Loop forever (never halt)
else:
// If halt says the program would run forever when given itself as input
return // Halt immediately
The "opposite" program takes a program as input and does the opposite of what the halt function predicts. If halt says the program would terminate when run with itself as input, opposite enters an infinite loop. If halt says the program would run forever, opposite terminates immediately.
Now comes the crucial step: What happens if we run opposite with itself as input? Let's analyze both possibilities:
- If halt(opposite, opposite) returns TRUE: This means opposite should terminate when given itself as input. But by the definition of opposite, it would then enter an infinite loop and never terminate. This is a contradiction.
- If halt(opposite, opposite) returns FALSE: This means opposite should run forever when given itself as input. But by the definition of opposite, it would then terminate immediately. Another contradiction.
No matter what halt predicts, the opposite program will do the exact opposite, creating a logical paradox. Since our assumption (that the halt function exists) leads to a contradiction, the assumption must be false. Therefore, the halting problem cannot be solved by any computer program.
Implications of the Halting Problem
The unsolvability of the halting problem has profound implications for computer science and software development:
- There cannot be a general algorithm that detects all infinite loops or determines if any arbitrary program will terminate.
- Certain bugs in software are theoretically impossible to detect automatically in all cases.
- There are fundamental limits to what automated program analysis tools can achieve.
- Complete automation of certain debugging and verification tasks is impossible.
Real-World Applications and Limitations
Despite the halting problem being unsolvable in the general case, we can still create useful tools that address specific instances:
- Static code analyzers can detect some classes of infinite loops and non-terminating behavior.
- Programming languages and runtime environments implement timeouts to handle potentially non-terminating processes.
- Restricted programming models can guarantee termination by limiting expressiveness.
- Heuristic approaches can provide good-enough solutions for many practical cases.
Conclusion: Living with Unsolvable Problems
The halting problem is a perfect example of how theoretical computer science informs practical programming. It demonstrates that there are fundamental limits to computation—some problems simply cannot be solved algorithmically.
So the next time one of your programs appears to be frozen, remember that it's not just an annoyance—it's a manifestation of a deep mathematical truth. Your computer literally cannot know for certain whether that program will eventually finish or run forever. This is not a limitation of current technology but a fundamental constraint on what computation can achieve.
Understanding these limitations helps us build better software by recognizing when we're approaching problems that might be fundamentally unsolvable in their general form, and finding practical workarounds for specific cases. The halting problem reminds us that even in the digital realm, some questions will always remain unanswerable.
Let's Watch!
Why the Halting Problem Remains Unsolvable: A Computer Science Paradox
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence