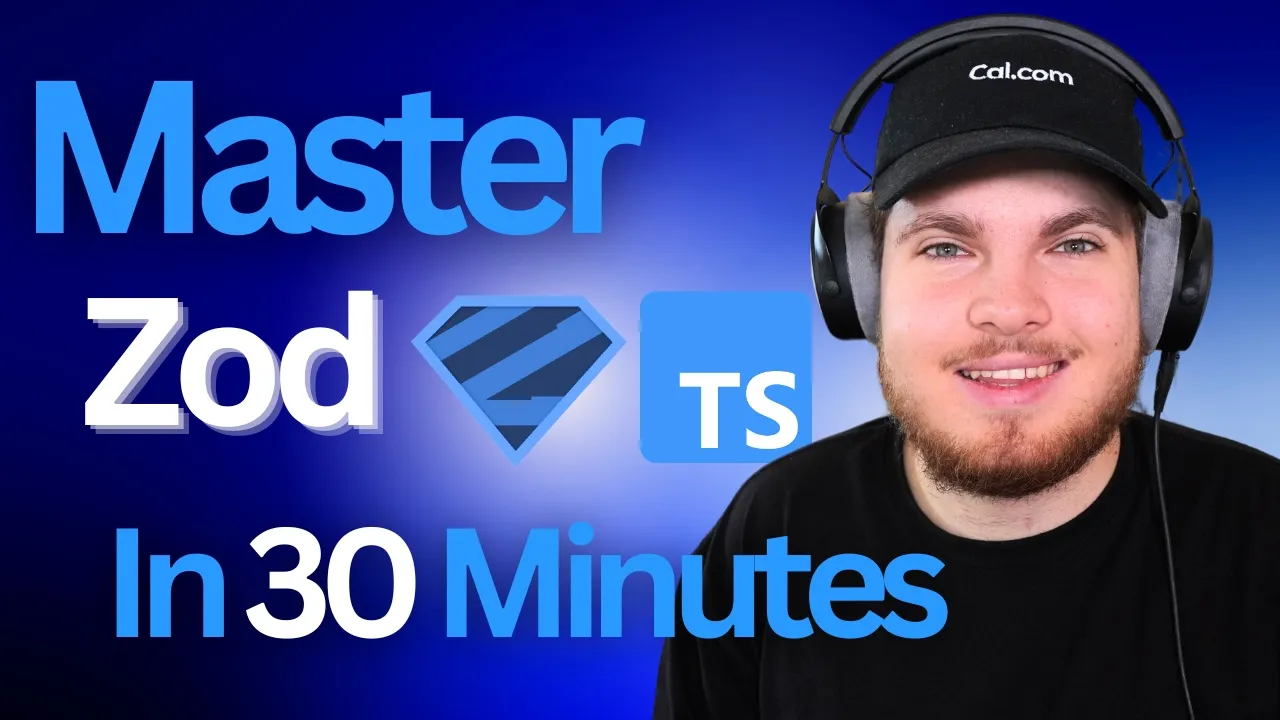
If you're using TypeScript, you might think your application is fully type-safe. But there's a critical gap in TypeScript's protection that many developers overlook—runtime type safety. Let's explore why Zod has become an essential tool for serious TypeScript developers who want complete type protection.
The Problem: TypeScript's Compile-Time Limitation
Consider this simple scenario: you have a function that processes user data with specific type requirements defined in TypeScript. The function expects a properly formatted email, numeric IDs, and specific data structures. Your IDE shows errors during development, but what happens when the application actually runs?
interface UserData {
id: number;
name: string;
email: string;
age: number;
preferences?: {
theme: 'light' | 'dark';
notifications: boolean;
};
}
function processUserData(userData: UserData): string {
const userSummary = {
...userData
};
return JSON.stringify(userSummary);
}
At first glance, this looks perfectly safe. TypeScript enforces the correct types during development. But here's what many developers miss: once TypeScript compiles to JavaScript, all those type checks disappear. Your runtime code has no type safety whatsoever.
The Dangerous Gap Between Compile-Time and Runtime
Let's see what happens when we pass invalid data to our function:
// Data from an API or user input
const userDataFromAPI = {
id: "123", // Should be a number!
name: "John Doe",
email: "invalid-email", // Not a valid email format
age: 10
};
// This will show TypeScript errors in your IDE
// But the code will still run!
processUserData(userDataFromAPI);
Despite TypeScript showing errors in your development environment, this code will still execute in production. TypeScript only validates at compile time, but all those safeguards are gone when your application is running. This means bad data can still flow through your system, potentially causing bugs or security issues.
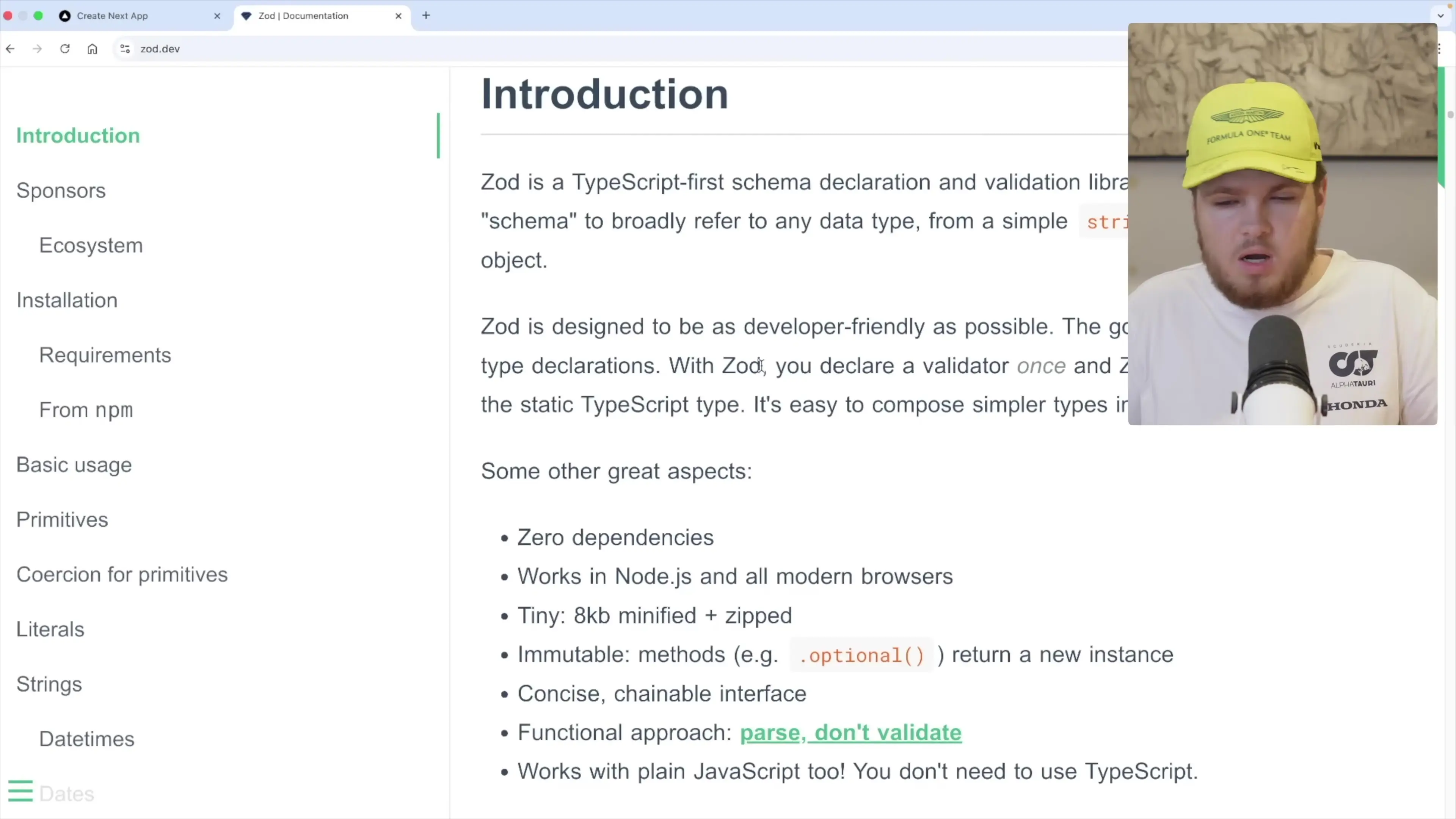
Enter Zod: Runtime Type Validation
Zod bridges this critical gap by providing runtime validation that matches your TypeScript types. It's a TypeScript-first schema validation library that ensures your data conforms to expected types and formats even after compilation.
Key benefits of Zod include:
- Runtime type checking that persists after compilation
- Type inference that eliminates duplicate type declarations
- Detailed validation errors that pinpoint exactly what's wrong
- Support for complex validation rules beyond simple types
- Chainable API for building sophisticated schemas
Implementing Zod in Your Project
Let's see how to implement Zod in our example:
import { z } from 'zod';
// Define your schema once
const userDataSchema = z.object({
id: z.number(),
name: z.string(),
email: z.string().email(), // Validates email format
age: z.number().positive(), // Ensures age is positive
preferences: z.object({
theme: z.enum(['light', 'dark']),
notifications: z.boolean()
}).optional()
});
// TypeScript type is automatically inferred from the schema
type UserData = z.infer<typeof userDataSchema>;
function processUserData(userData: UserData): string {
// Validate at runtime
const validatedData = userDataSchema.parse(userData);
// Now we know the data is safe to use
return JSON.stringify(validatedData);
}
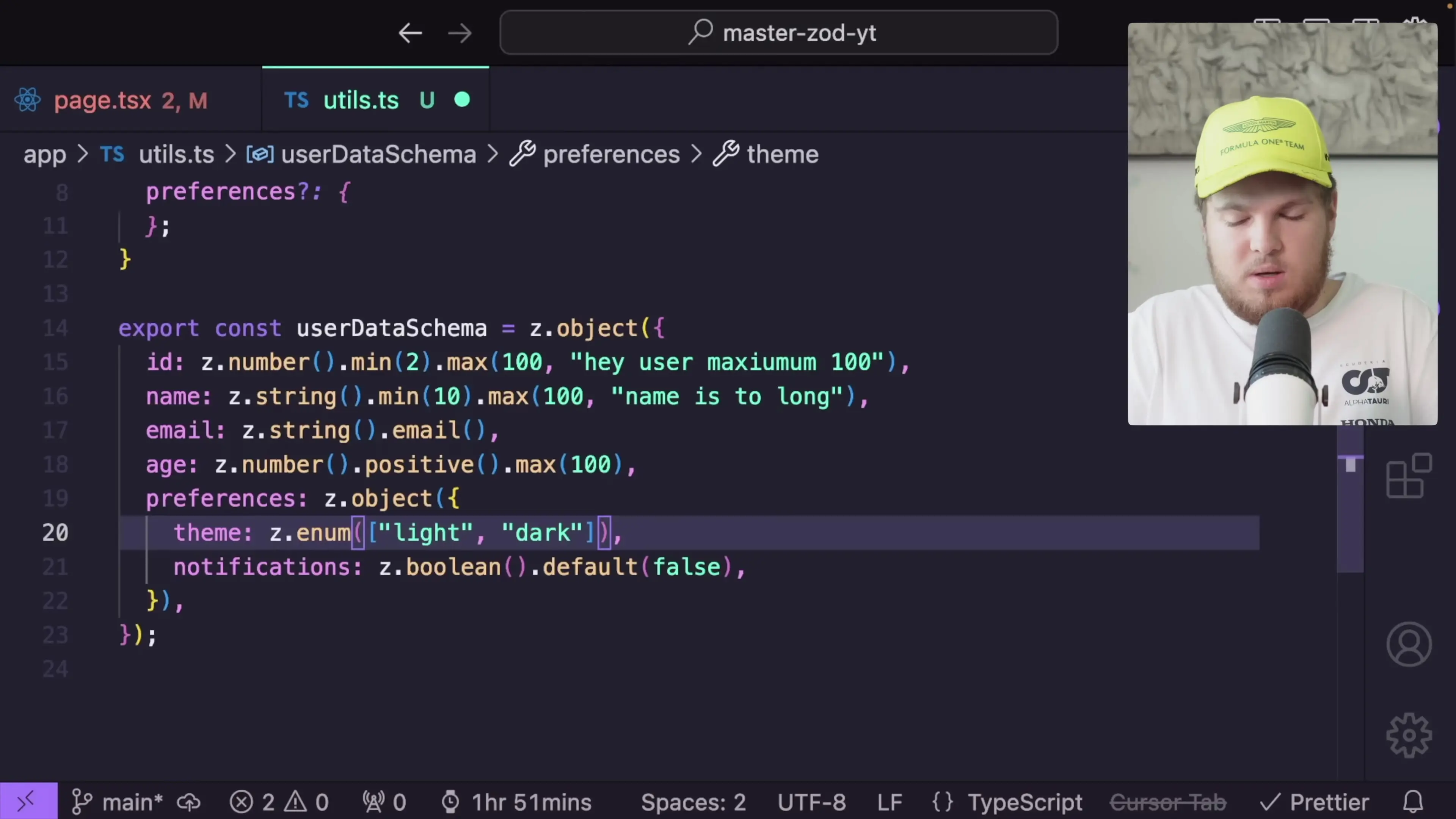
With this implementation, if someone tries to pass invalid data to your function, Zod will throw a detailed error at runtime, preventing the function from processing bad data.
Advanced Validation with Zod
Zod goes beyond simple type checking. You can create sophisticated validation rules to ensure data quality:
const advancedUserSchema = z.object({
id: z.number().int().positive(),
name: z.string().min(2).max(100),
email: z.string().email(),
age: z.number().min(13).max(120), // Age restrictions
password: z.string()
.min(8)
.regex(/[A-Z]/, { message: "Password must contain at least one uppercase letter" })
.regex(/[0-9]/, { message: "Password must contain at least one number" }),
confirmPassword: z.string()
}).refine(data => data.password === data.confirmPassword, {
message: "Passwords don't match",
path: ["confirmPassword"]
});
Integrating Zod with React Forms
Zod pairs perfectly with form libraries like React Hook Form for complete form validation:
import { useForm } from 'react-hook-form';
import { zodResolver } from '@hookform/resolvers/zod';
function UserForm() {
const {
register,
handleSubmit,
formState: { errors }
} = useForm({
resolver: zodResolver(userDataSchema)
});
const onSubmit = (data) => {
// Data is already validated by Zod
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register("name")} />
{errors.name && <p>{errors.name.message}</p>}
<input {...register("email")} />
{errors.email && <p>{errors.email.message}</p>}
<button type="submit">Submit</button>
</form>
);
}
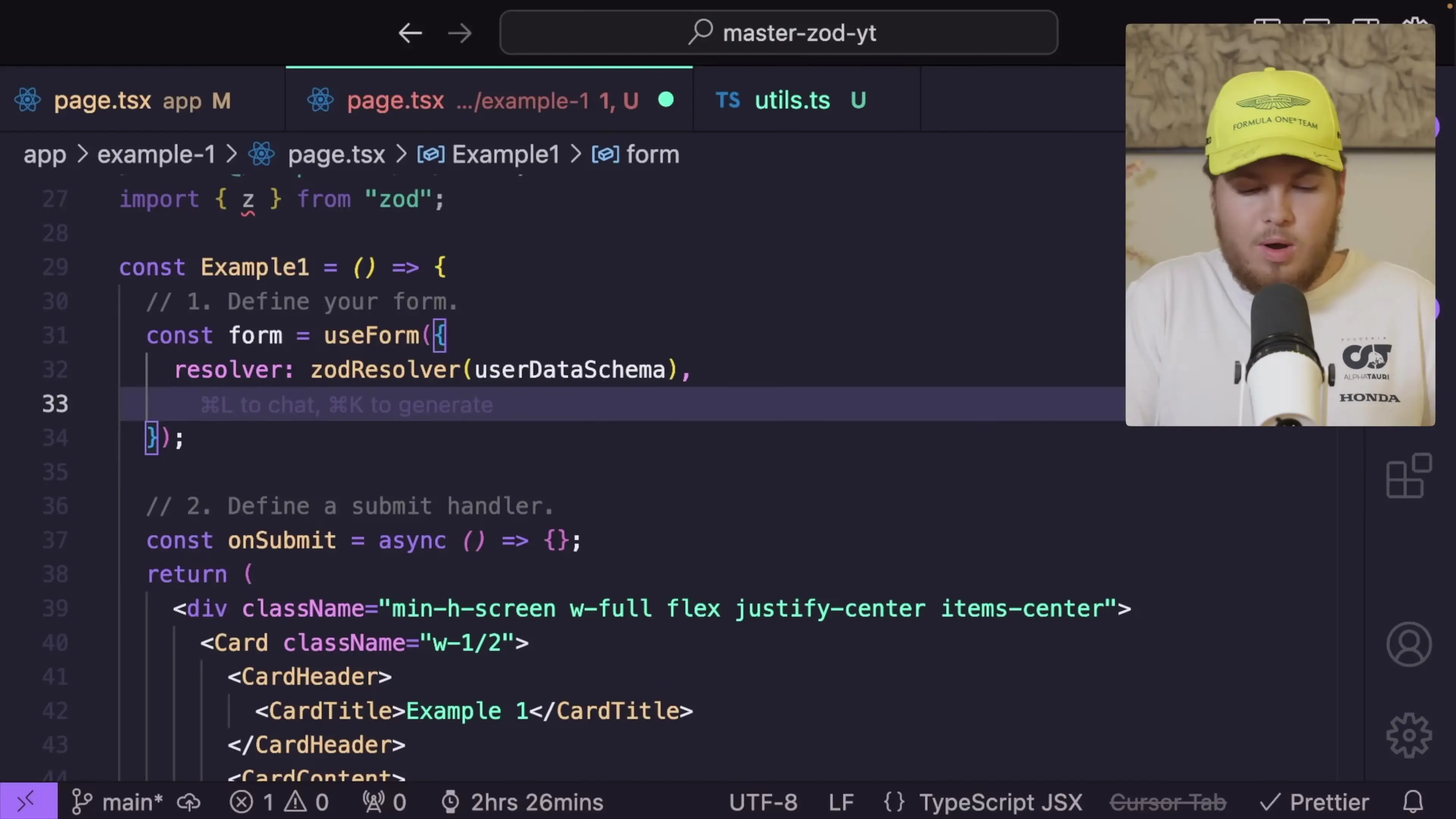
Best Practices for Using Zod
- Define schemas in a central location for reuse across your application
- Use z.infer
to generate TypeScript types from schemas - Add specific error messages for better user feedback
- Validate all external data at application boundaries (API endpoints, form submissions)
- Use safeParse() instead of parse() when you want to handle validation errors gracefully
When to Use Zod
Zod is particularly valuable in these scenarios:
- Working with external APIs where you don't control the data format
- Processing user input from forms
- Validating configuration files or environment variables
- Building public APIs that need to validate client requests
- When you need to ensure data integrity in critical applications
Conclusion
TypeScript alone can't protect your application from invalid data at runtime. Zod fills this critical gap by providing runtime validation that matches your TypeScript types. By implementing Zod in your projects, you get the best of both worlds: TypeScript's excellent developer experience during development and Zod's robust runtime validation when your application is running in production.
Don't leave your application vulnerable to bad data. Add Zod to your TypeScript projects and enjoy true end-to-end type safety.
Let's Watch!
Why Zod is Essential for Type-Safe JavaScript: Runtime Validation Guide
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence