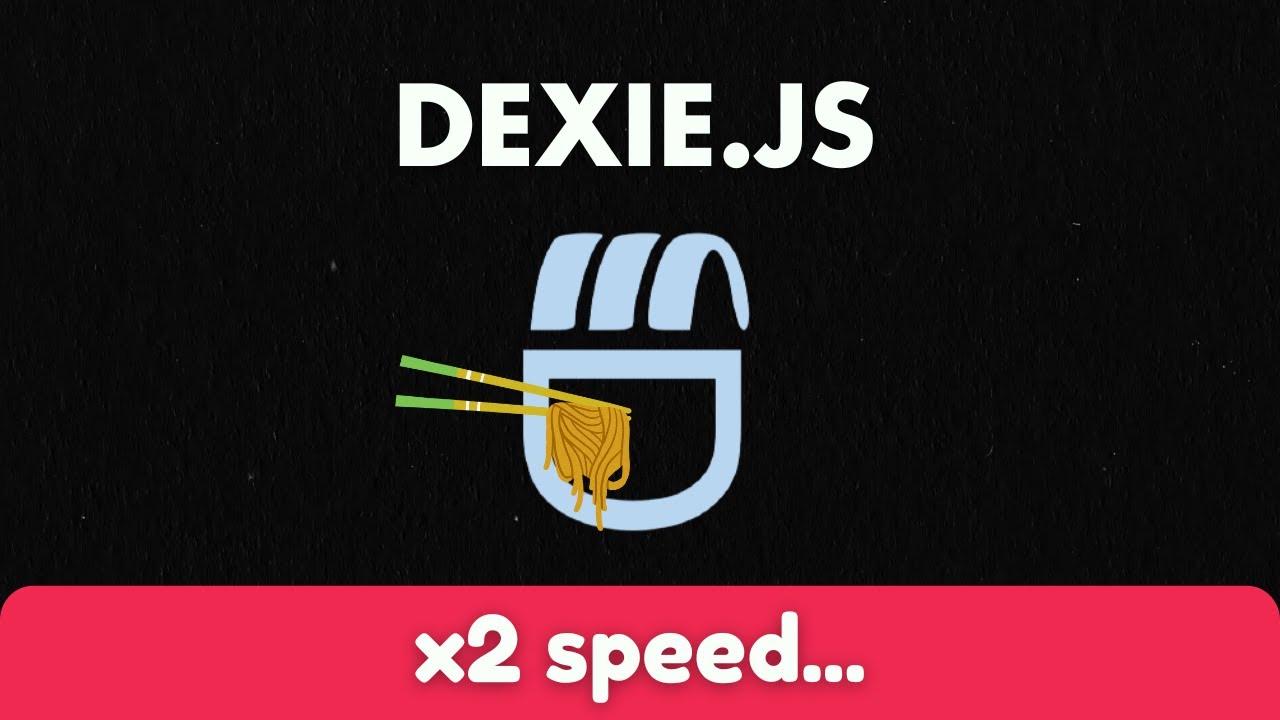
When developing modern web applications, client-side data persistence is often a critical requirement. While localStorage provides a simple solution, it comes with significant limitations. Enter Dexie.js, a powerful wrapper for IndexedDB that transforms browser-based storage capabilities for frontend developers working with complex data structures.
Understanding the Limitations of localStorage
Before diving into Dexie.js, it's important to understand why localStorage falls short for many applications. When attempting to store complex objects in localStorage, you'll quickly encounter issues:
- Complex objects are converted to "[object Object]" when stored directly
- Objects must be manually stringified and parsed using JSON methods
- Storage is limited to approximately 5MB per domain
- Operations are synchronous and block the main thread
- No support for structured queries or indexing
- Limited capability for storing binary data like images
// Attempting to store an object in localStorage
const user = { name: 'John', age: 30, preferences: { theme: 'dark' } };
localStorage.setItem('user', user);
console.log(localStorage.getItem('user')); // "[object Object]"
// The workaround requires manual JSON handling
localStorage.setItem('user', JSON.stringify(user));
const retrievedUser = JSON.parse(localStorage.getItem('user'));
console.log(retrievedUser.name); // "John"
What is Dexie.js and Why Use It?
Dexie.js is a minimalistic wrapper for IndexedDB, the low-level browser database that stores and retrieves data directly on the user's device. While IndexedDB is powerful, its API can be complex and verbose. Dexie.js simplifies this with an elegant, promise-based API that makes working with client-side databases much more developer-friendly.
- Stores real JavaScript objects without manual conversion
- Provides gigabytes of storage (browser-dependent) versus localStorage's 5MB limit
- Operates asynchronously, preventing main thread blocking
- Functions as a complete database with querying capabilities, not just a key-value store
- Enables offline data persistence for Progressive Web Apps (PWAs)
- Supports storage of binary data like images through Blob objects
- Offers TypeScript support for type-safe database operations
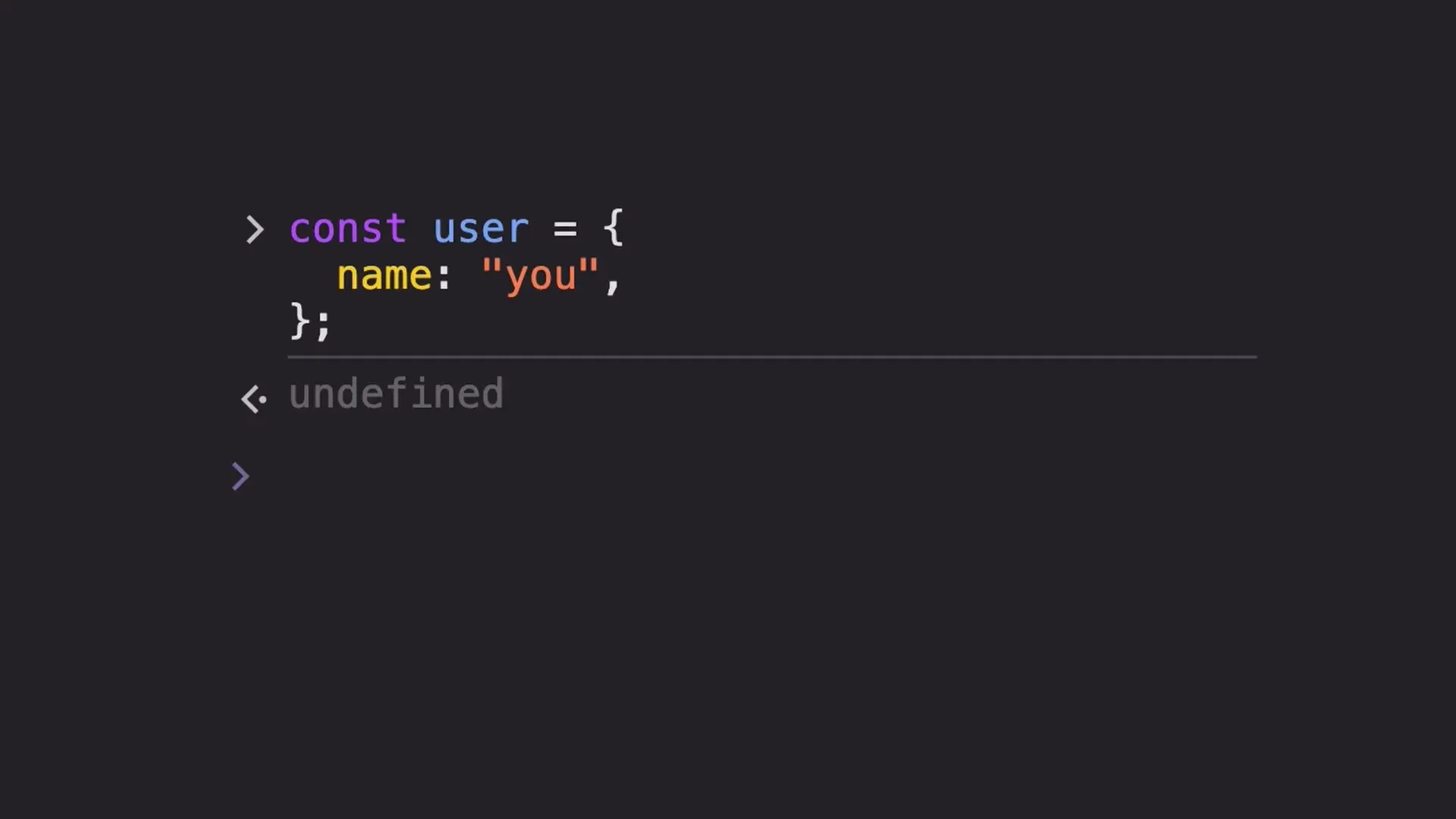
Getting Started with Dexie.js in a React Application
Let's walk through setting up Dexie.js in a React application to demonstrate its capabilities for client-side data persistence. We'll create a simple database schema, implement CRUD operations, and explore how to store and retrieve complex objects.
Step 1: Installation and Setup
First, create a new React project using Vite and install the necessary Dexie.js packages:
# Create a new React project with Vite
npm create vite@latest my-dexie-app --template react-ts
cd my-dexie-app
# Install Dexie.js and React hooks
npm install dexie dexie-react-hooks
Step 2: Creating a Database Configuration
Next, create a database configuration file to define your schema. This is where you'll define the database version, tables, and their structure:
// db.ts
import Dexie, { Table } from 'dexie';
// Define the interface for our data structure
export interface Person {
id?: number; // Optional because it's auto-incremented
name: string;
age: number;
}
// Create a Dexie database class
class AppDatabase extends Dexie {
// Define tables
people!: Table<Person>;
constructor() {
super('AppDatabase');
this.version(1).stores({
people: '++id, name, age' // Primary key with auto-increment, plus indexes
});
}
}
// Create and export a database instance
export const db = new AppDatabase();
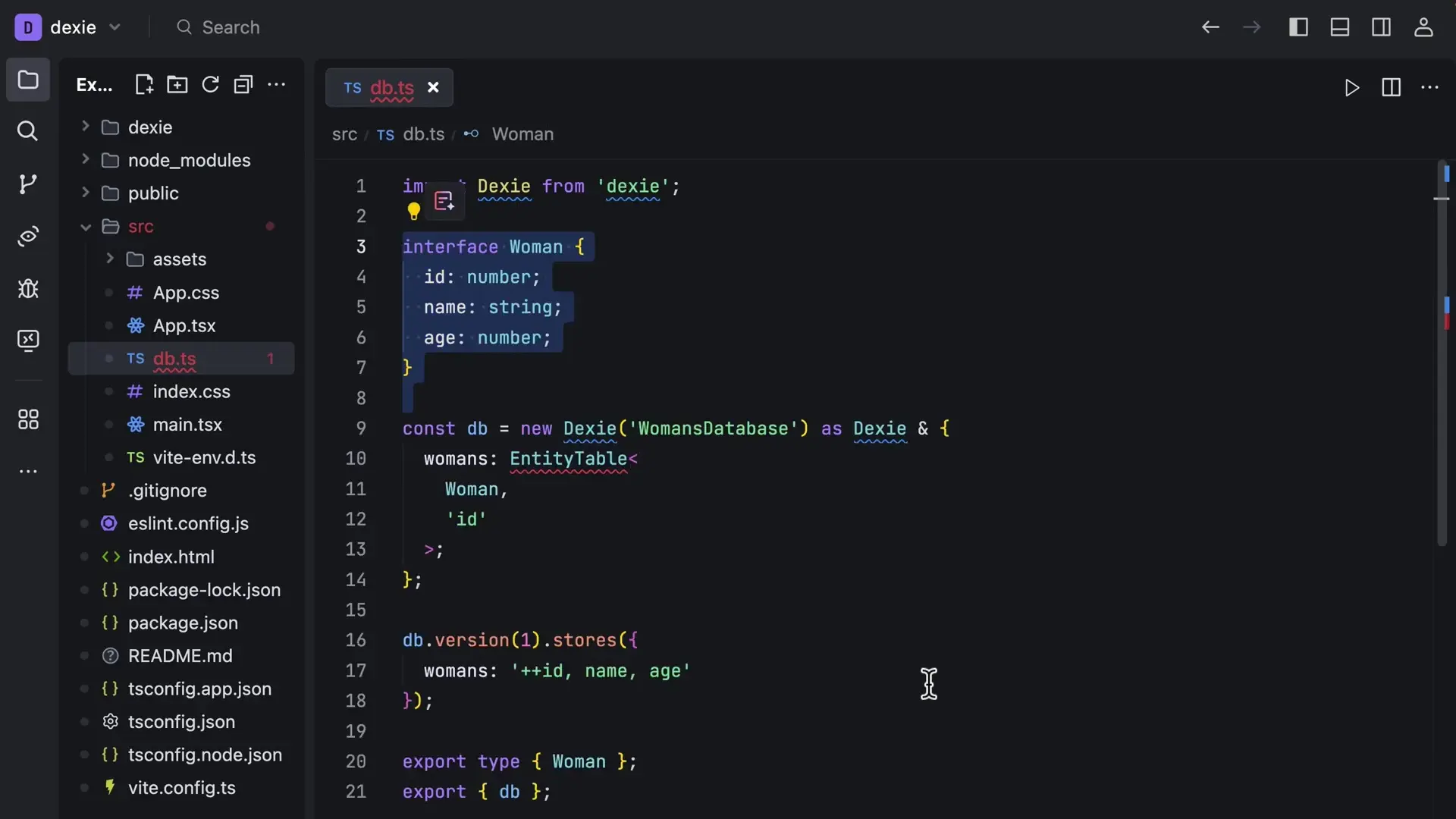
In this configuration, we've defined a database with a single table called 'people'. The schema string '++id, name, age' indicates that 'id' is the primary key with auto-increment (++), and 'name' and 'age' are indexed fields that can be efficiently queried.
Step 3: Creating Components for Database Interaction
Now let's create React components to interact with our database. We'll need a form component to add new records and a list component to display and manage existing records:
// PersonForm.tsx
import React, { useState } from 'react';
import { db } from './db';
export function PersonForm() {
const [name, setName] = useState('');
const [age, setAge] = useState('');
const handleSubmit = async (e: React.FormEvent) => {
e.preventDefault();
if (name && age) {
try {
// Add a new person to the database
await db.people.add({
name,
age: parseInt(age, 10)
});
// Reset form
setName('');
setAge('');
} catch (error) {
console.error('Failed to add person:', error);
}
}
};
return (
<form onSubmit={handleSubmit}>
<div>
<label htmlFor="name">Name:</label>
<input
id="name"
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
required
/>
</div>
<div>
<label htmlFor="age">Age:</label>
<input
id="age"
type="number"
value={age}
onChange={(e) => setAge(e.target.value)}
required
/>
</div>
<button type="submit">Add Person</button>
</form>
);
}
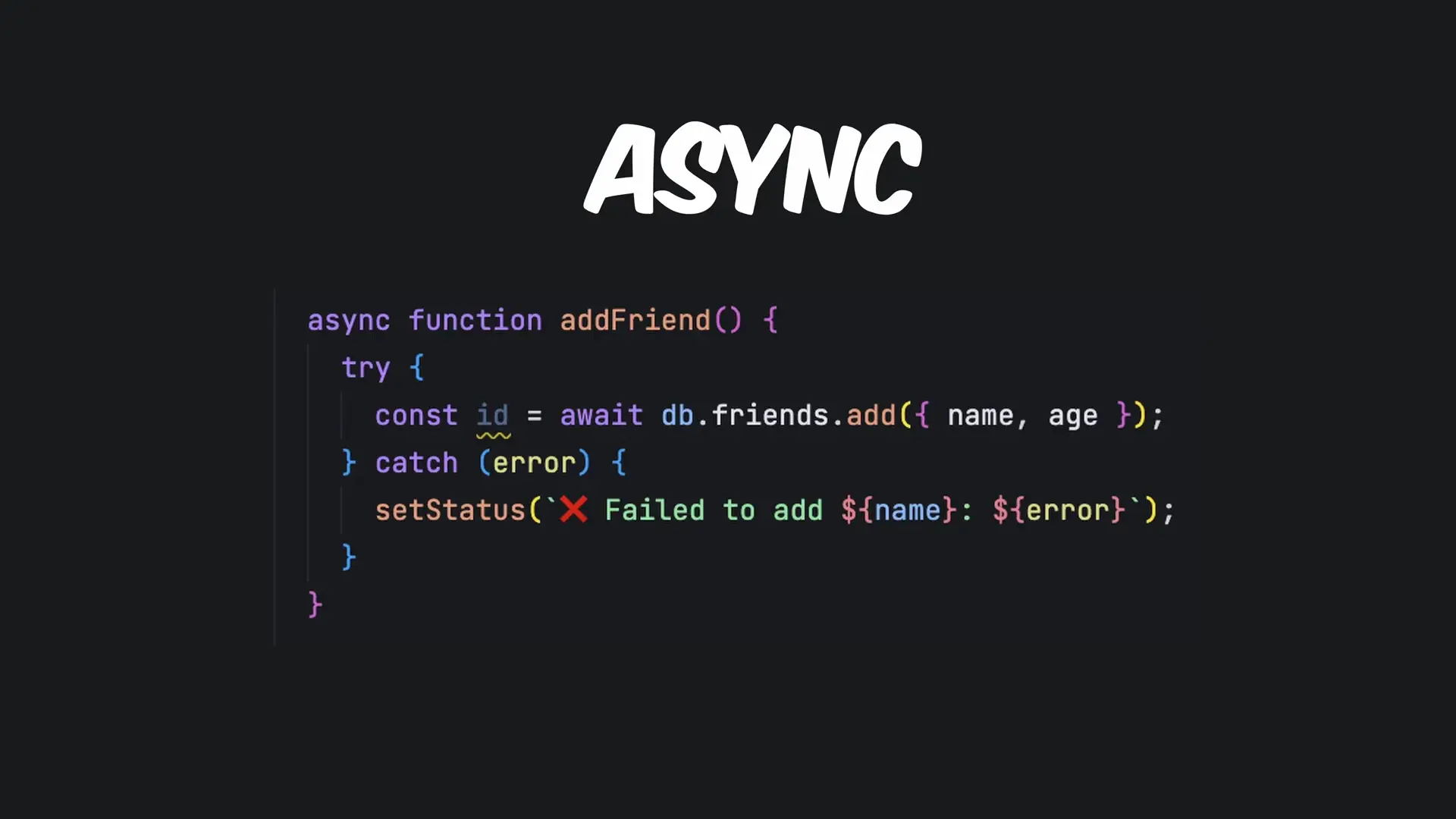
// PeopleList.tsx
import { useLiveQuery } from 'dexie-react-hooks';
import { db, Person } from './db';
export function PeopleList() {
// Use the live query hook to automatically update when data changes
const people = useLiveQuery(
() => db.people.toArray()
);
const deletePerson = async (id: number) => {
try {
await db.people.delete(id);
} catch (error) {
console.error('Failed to delete person:', error);
}
};
if (!people) return <div>Loading...</div>;
return (
<div>
<h2>People</h2>
{people.length === 0 ? (
<p>No people in the database</p>
) : (
<ul>
{people.map((person) => (
<li key={person.id}>
{person.name} (Age: {person.age})
<button onClick={() => person.id && deletePerson(person.id)}>
Delete
</button>
</li>
))}
</ul>
)}
</div>
);
}
Advanced Dexie.js Features
Storing Images with Blob Objects
One of the most powerful features of Dexie.js is its ability to store binary data like images. This is particularly useful for offline-capable applications that need to cache images locally:
// Extending our database schema to include images
export interface Person {
id?: number;
name: string;
age: number;
photo?: Blob; // Store image as a Blob
}
class AppDatabase extends Dexie {
people!: Table<Person>;
constructor() {
super('AppDatabase');
this.version(1).stores({
people: '++id, name, age'
// Note: Blob fields don't need to be indexed
});
}
}
// Example function to save an image from a file input
async function savePersonWithPhoto(name: string, age: number, photoFile: File) {
try {
// Convert File to Blob if needed (though File is already a Blob subclass)
const photo = new Blob([await photoFile.arrayBuffer()], { type: photoFile.type });
// Use the put method which is recommended for larger objects
await db.people.put({
name,
age,
photo
});
console.log('Person with photo saved successfully');
} catch (error) {
console.error('Failed to save person with photo:', error);
}
}
Querying Data with Dexie.js
Dexie.js provides powerful querying capabilities that go far beyond simple key-value retrieval. Here are some examples of how to query your database effectively:
// Filtering by property values
const adultsOnly = await db.people
.where('age')
.aboveOrEqual(18)
.toArray();
// Combining multiple conditions
const youngAdults = await db.people
.where('age')
.between(18, 30)
.and(person => person.name.startsWith('A'))
.toArray();
// Sorting results
const sortedByAge = await db.people
.orderBy('age')
.reverse() // descending order
.toArray();
// Limiting results
const firstFivePeople = await db.people
.limit(5)
.toArray();
// Full-text search (case-insensitive)
const searchResults = await db.people
.filter(person => person.name.toLowerCase().includes('smith'))
.toArray();
Dexie.js vs. localStorage: When to Use Each
While Dexie.js offers significant advantages over localStorage, it's important to choose the right tool for your specific needs:
- Use localStorage when: You need simple key-value storage for small amounts of data (< 5MB), synchronous access is acceptable, and you don't need complex queries or offline persistence.
- Use Dexie.js when: You need to store complex objects or binary data (like images), require large storage capacity, need structured querying capabilities, want asynchronous operations that don't block the main thread, or are building offline-capable applications.
Progressive Web App (PWA) Integration
Dexie.js is particularly valuable for Progressive Web Apps that need to function offline. By combining Dexie.js with service workers, you can create robust offline experiences:
- Cache API requests and responses in Dexie.js when online
- Serve cached data when offline
- Queue write operations performed offline
- Sync with the server when connection is restored
// Example of offline-first data synchronization pattern
export async function fetchAndCacheUsers() {
try {
// Try to fetch from network first
const response = await fetch('https://api.example.com/users');
const users = await response.json();
// Cache the results in Dexie
await db.transaction('rw', db.users, async () => {
// Clear existing cache
await db.users.clear();
// Add all new users
await db.users.bulkAdd(users);
});
return users;
} catch (error) {
console.warn('Network request failed, using cached data');
// Fall back to cached data
return await db.users.toArray();
}
}
Performance Considerations
While Dexie.js offers substantial benefits, it's important to be mindful of performance implications:
- Use indexes wisely - they speed up queries but slow down writes
- Consider using bulkAdd() or bulkPut() for batch operations
- Be cautious with the size of individual objects, especially when storing many large blobs
- Implement database versioning and migrations properly to avoid data loss
- Use the correct methods for your use case (add() for new objects, put() for updates or when storing large objects)
Conclusion: Embracing Client-Side Database Solutions
Dexie.js represents a significant advancement in browser-based data storage capabilities. By providing a friendly wrapper around IndexedDB, it enables developers to create sophisticated web applications with robust offline capabilities, complex data structures, and efficient querying. Whether you're building a PWA, need to store images client-side, or simply want to move beyond the limitations of localStorage, Dexie.js offers a powerful solution for modern web development.
As browsers continue to evolve and users expect increasingly sophisticated web applications, client-side database solutions like Dexie.js will become essential tools in the frontend developer's toolkit. By mastering these technologies now, you'll be well-positioned to create the next generation of web applications that work seamlessly regardless of network conditions.
Let's Watch!
Dexie.js: The Ultimate Browser Database Solution for Complex Data Storage
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence