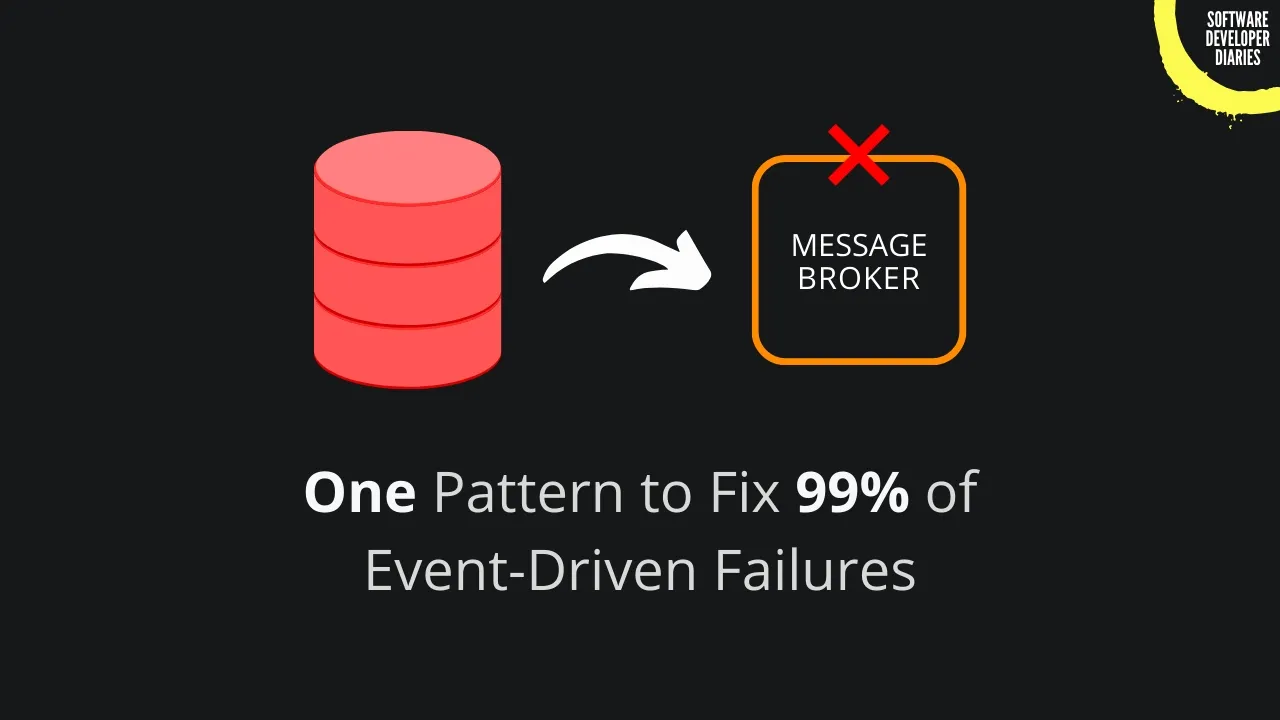
In modern distributed systems, particularly those built with microservices architecture, ensuring data consistency across services is a significant challenge. When one service needs to both update its database and notify other services about the change, we face a critical problem: how do we guarantee that both operations succeed, even if components fail? This is where the Transactional Outbox Pattern comes in—a powerful solution used in virtually any asynchronous system that requires reliable message delivery.
The Problem: Synchronous Communication and Temporal Coupling
Let's consider a common e-commerce scenario to understand the problem. When a customer places an order, the order service needs to save the order in its database and then trigger post-processing tasks like sending confirmation emails or notifying suppliers about shipping requirements.
The traditional approach involves a synchronous workflow:
- The order service saves the order in its database
- It makes a synchronous HTTP request to a post-processing service
- The post-processing service handles the order (sending emails, etc.)
- The post-processing service sends an acknowledgment back to the order service
This approach creates what's known as temporal coupling—a dependency where both services must be available simultaneously for the operation to succeed. If the post-processing service is down or experiencing issues, the entire workflow breaks. Even worse, we end up with an inconsistent state: the order exists in the database, but the necessary post-processing never occurred.
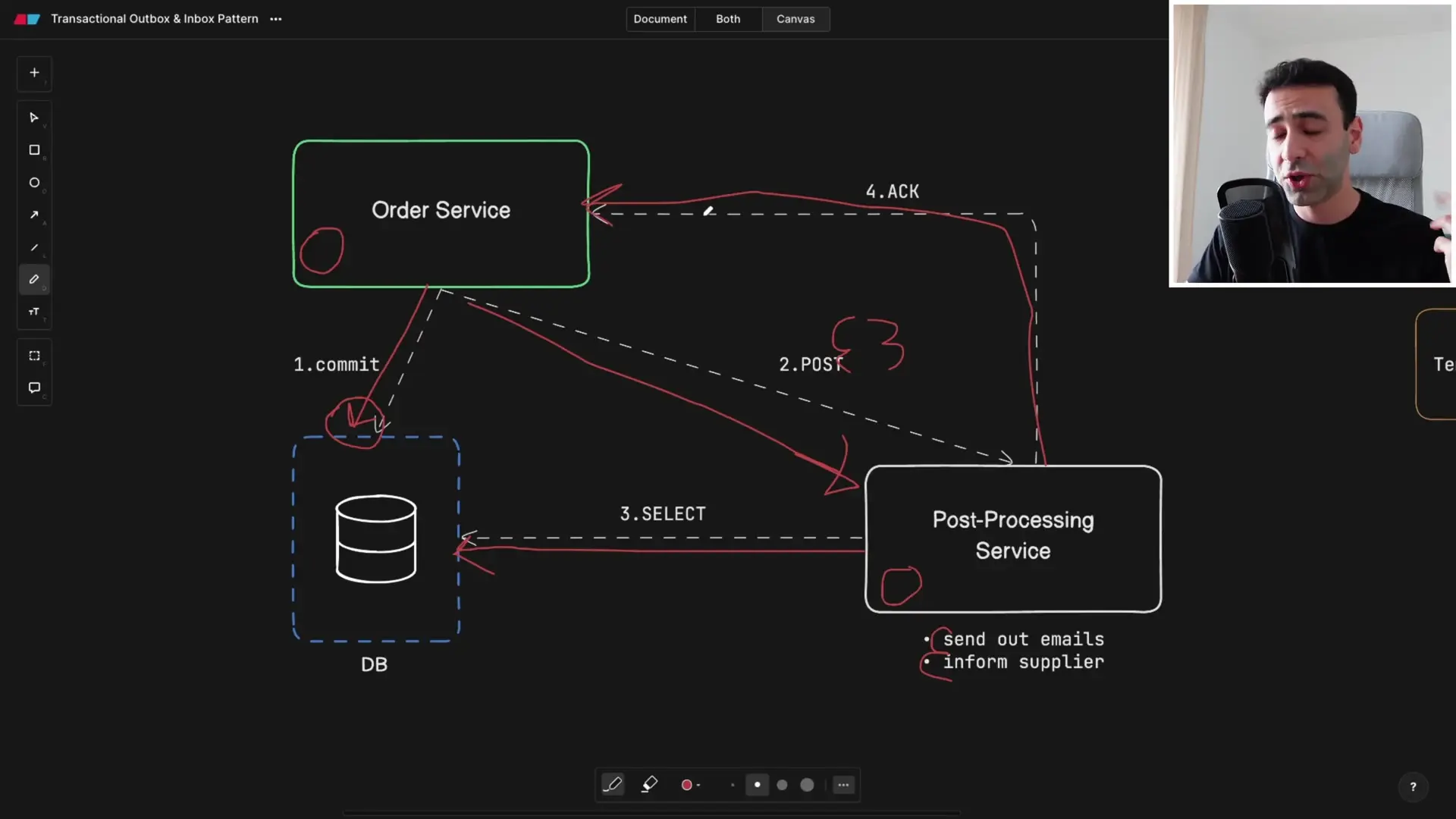
First Attempt: Adding a Message Broker
A common solution is to introduce asynchronous communication using a message broker like RabbitMQ:
- The order service saves the order in its database
- It sends a message to a message broker
- The post-processing service subscribes to these messages
- When it receives a message, it processes the order and sends an acknowledgment
This approach reduces temporal coupling, but introduces new challenges:
- Message duplication: If the broker doesn't acknowledge receipt, the order service might send the same message multiple times, requiring deduplication logic
- Partial failures: If the message broker is down when the order service tries to send the message, we still end up with an inconsistent state—the order exists in the database but no downstream processing occurs
The Solution: Transactional Outbox Pattern
The Transactional Outbox Pattern elegantly solves these issues by ensuring atomic operations and reliable message delivery. Here's how it works:
- Add an "outbox" table to your service's database
- When processing an order, use a single database transaction to both save the order and insert a message into the outbox table
- A separate relay service (or worker) periodically polls the outbox table for unsent messages
- The relay service sends these messages to the message broker and marks them as sent
- Downstream services consume these messages from the broker
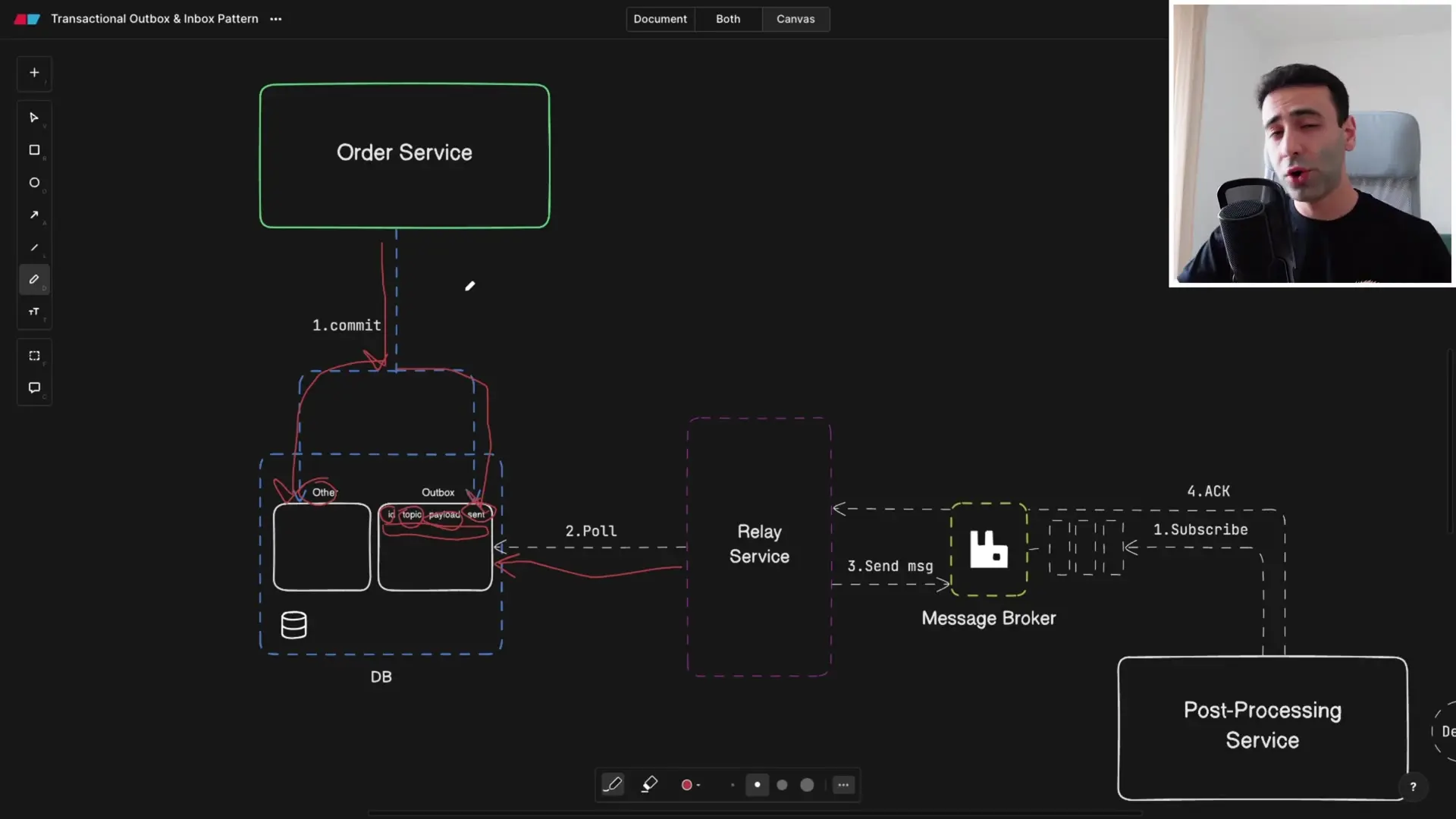
The Outbox Table Structure
A typical outbox table includes these columns:
- ID: A unique identifier for the message
- Topic: The message topic or type (e.g., "OrderCreated")
- Payload: The message content (typically JSON)
- Sent: A flag indicating whether the message has been processed (0=unsent, 1=sent)
CREATE TABLE outbox (
id VARCHAR(36) PRIMARY KEY,
topic VARCHAR(255) NOT NULL,
payload JSON NOT NULL,
sent TINYINT(1) DEFAULT 0,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
Why This Pattern Works: Database as the Source of Truth
The key insight of the Transactional Outbox Pattern is using the database as the single source of truth. By storing both business data and messages in the same database, we leverage the ACID properties of database transactions to ensure consistency.
Even if the message broker, relay service, or post-processing service fails, the messages remain safely stored in the outbox table. When the failed components recover, message processing resumes automatically from where it left off.
Implementation Example with Node.js and MySQL
Here's a simplified implementation using Node.js, MySQL, and RabbitMQ:
1. Order Service - Creating an Order with Outbox
// Using MySQL with transactions
async function createOrder(orderData) {
const connection = await mysql.createConnection(dbConfig);
try {
await connection.beginTransaction();
// Insert order into orders table
const [orderResult] = await connection.execute(
'INSERT INTO orders (customer_id, total, status) VALUES (?, ?, ?)',
[orderData.customerId, orderData.total, 'created']
);
const orderId = orderResult.insertId;
// Insert message into outbox table
await connection.execute(
'INSERT INTO outbox (id, topic, payload, sent) VALUES (?, ?, ?, ?)',
[
uuidv4(),
'order.created',
JSON.stringify({ orderId, ...orderData }),
0
]
);
await connection.commit();
return { success: true, orderId };
} catch (error) {
await connection.rollback();
throw error;
} finally {
await connection.end();
}
}
2. Relay Service - Publishing Messages from Outbox
// Relay service that polls the outbox table
async function relayMessages() {
const connection = await mysql.createConnection(dbConfig);
try {
// Get unsent messages
const [messages] = await connection.execute(
'SELECT * FROM outbox WHERE sent = 0 LIMIT 100'
);
if (messages.length === 0) return;
// Connect to RabbitMQ
const mqConnection = await amqp.connect('amqp://localhost');
const channel = await mqConnection.createChannel();
// Process each message
for (const message of messages) {
// Publish to appropriate exchange/queue
await channel.assertExchange(message.topic, 'topic', { durable: true });
channel.publish(
message.topic,
'',
Buffer.from(message.payload),
{ persistent: true }
);
// Mark as sent
await connection.execute(
'UPDATE outbox SET sent = 1 WHERE id = ?',
[message.id]
);
}
await channel.close();
await mqConnection.close();
} catch (error) {
console.error('Error relaying messages:', error);
} finally {
await connection.end();
}
}
// Run the relay service periodically
setInterval(relayMessages, 1000);
3. Post-Processing Service - Consuming Messages
// Consumer service
async function startConsumer() {
try {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const exchange = 'order.created';
const queue = 'order-processing-service';
await channel.assertExchange(exchange, 'topic', { durable: true });
await channel.assertQueue(queue, { durable: true });
await channel.bindQueue(queue, exchange, '');
channel.consume(queue, async (msg) => {
if (!msg) return;
try {
const order = JSON.parse(msg.content.toString());
// Process order (send emails, notify suppliers, etc.)
await processOrder(order);
// Acknowledge message
channel.ack(msg);
} catch (error) {
console.error('Error processing message:', error);
// Nack and requeue on error
channel.nack(msg, false, true);
}
});
} catch (error) {
console.error('Consumer error:', error);
}
}
startConsumer();
Optimizing the Pattern for Scalability
For high-volume systems, the basic implementation may need optimization:
- Adjust polling frequency: Balance between timely message delivery and database load
- Implement parallel processing: Use multiple relay service workers to process messages concurrently
- Batch processing: Process multiple outbox messages in a single operation
- Message cleanup: Implement a strategy to archive processed messages
- Idempotent consumers: Ensure downstream services can handle duplicate messages safely
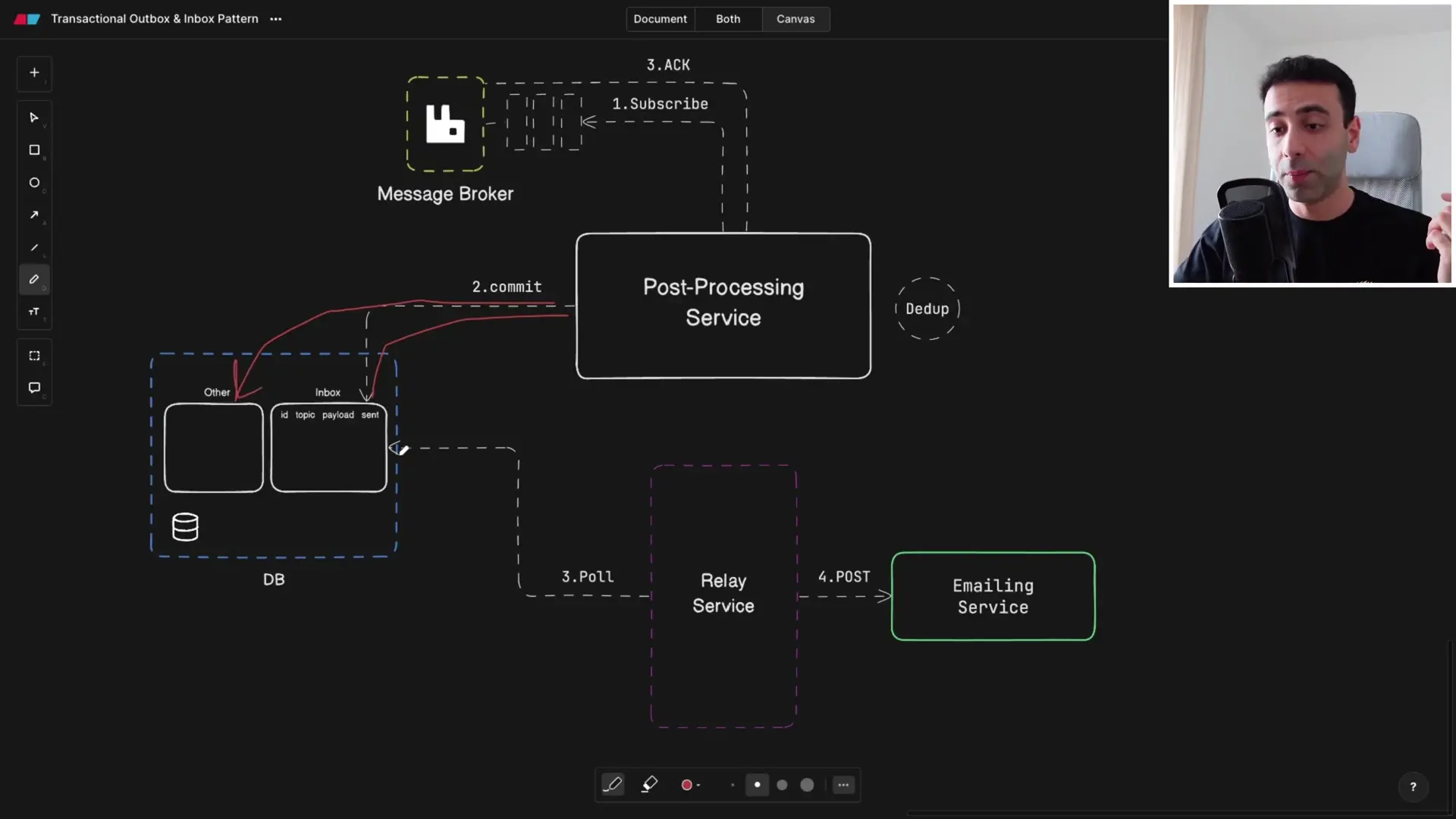
Complementary Pattern: Inbox Pattern for Consumers
The Transactional Outbox Pattern can be complemented with an Inbox Pattern on the consumer side to ensure exactly-once processing semantics. The Inbox Pattern works similarly:
- The consumer service maintains an "inbox" table in its database
- When receiving a message, it first checks if the message ID exists in the inbox
- If not, it processes the message and records the ID in the inbox table
- If the message ID already exists, it's a duplicate and can be safely ignored
async function processMessage(message) {
const connection = await mysql.createConnection(consumerDbConfig);
try {
await connection.beginTransaction();
// Check if message was already processed
const [rows] = await connection.execute(
'SELECT id FROM inbox WHERE message_id = ?',
[message.id]
);
if (rows.length > 0) {
// Message already processed, skip
await connection.commit();
return { success: true, status: 'already_processed' };
}
// Process the message (business logic)
await performBusinessLogic(message.payload);
// Record message as processed
await connection.execute(
'INSERT INTO inbox (message_id, processed_at) VALUES (?, NOW())',
[message.id]
);
await connection.commit();
return { success: true, status: 'processed' };
} catch (error) {
await connection.rollback();
throw error;
} finally {
await connection.end();
}
}
Benefits of the Transactional Outbox Pattern
- Atomic operations: Database updates and message publishing are guaranteed to succeed or fail together
- Resilience to failures: Messages are preserved even if the message broker or other services fail
- Eventual consistency: The system will eventually reach a consistent state, even after failures
- Reduced temporal coupling: Services can operate independently
- Scalability: The pattern works well in high-volume distributed systems
- Auditability: The outbox table provides a record of all integration events
When to Use This Pattern
The Transactional Outbox Pattern is particularly valuable in these scenarios:
- Microservices architectures with data consistency requirements across service boundaries
- Event-driven systems where reliable event publishing is critical
- E-commerce platforms where order processing must be reliable
- Financial applications where transaction integrity is essential
- Systems with strict audit requirements for data changes
- High-availability systems that must handle component failures gracefully
Conclusion
The Transactional Outbox Pattern is a powerful solution for ensuring data consistency and reliable message delivery in distributed systems. By leveraging database transactions to atomically update data and record outgoing messages, it creates resilient systems that can recover from component failures without losing data or creating inconsistencies.
While implementing this pattern requires additional infrastructure and code complexity, the benefits of improved reliability, consistency, and system resilience make it worthwhile for many real-world applications. As microservices and event-driven architectures continue to grow in popularity, the Transactional Outbox Pattern remains an essential tool in the distributed systems architect's toolkit.
Let's Watch!
The Transactional Outbox Pattern: Essential for Reliable Microservices Communication
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence