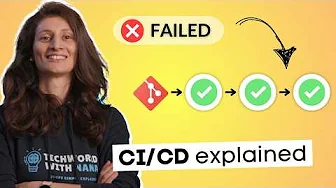
In today's fast-paced software development world, the difference between struggling teams and high-performing ones often comes down to their implementation of CI/CD pipelines. But what exactly is CI/CD, and why has it become such a critical component of modern DevOps practices? This article explores the real-world problems CI/CD solves and provides a practical guide to implementing these practices in your development workflow.
Understanding the Problem: The Manual Deployment Nightmare
Let's start by examining a common scenario that many development teams face without proper CI/CD implementation. Imagine you and your team have completed the first version of an application and it's time to deploy to a server.
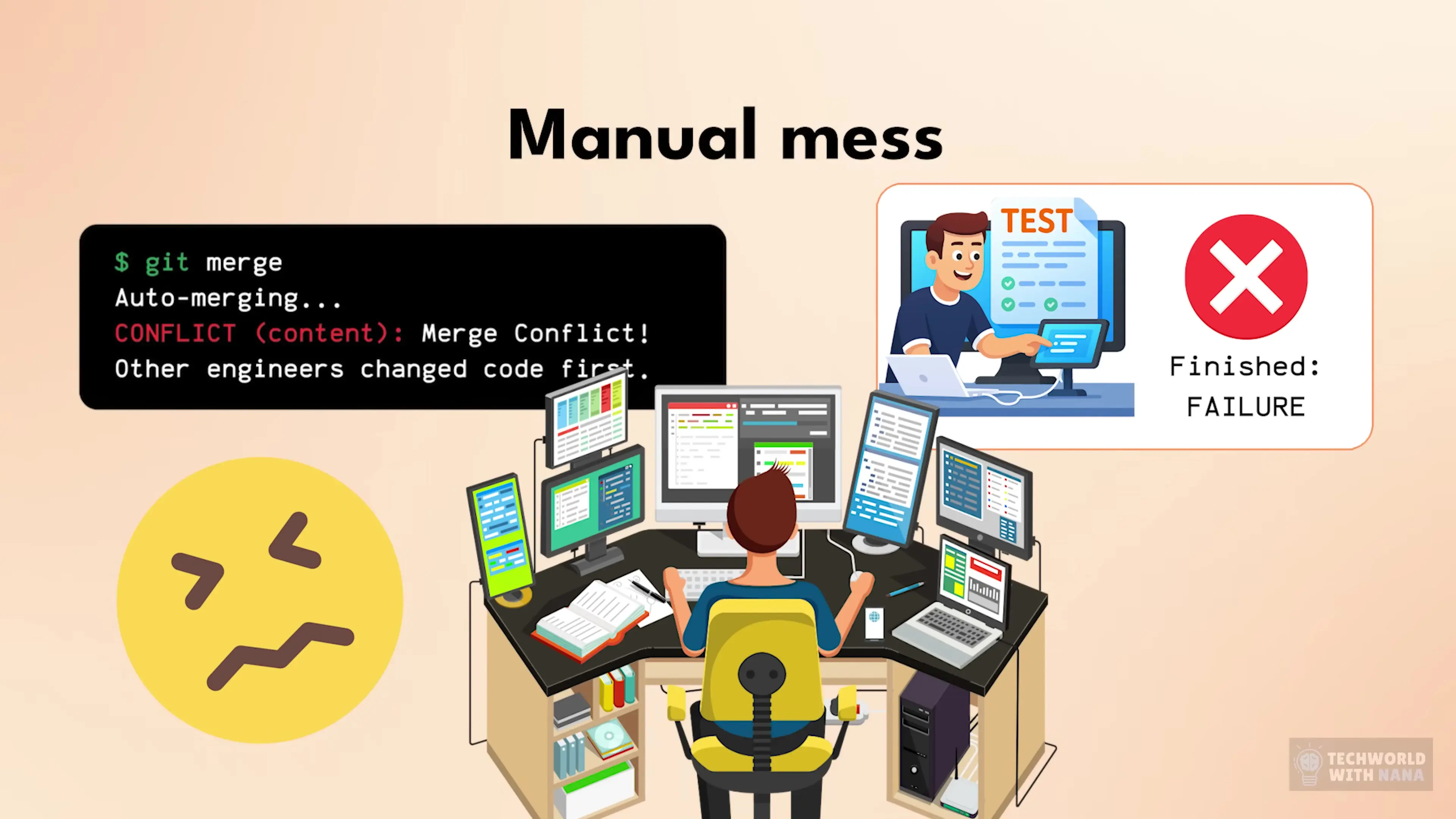
The process typically unfolds like this:
- You check in code and try to merge it to the main branch, only to encounter merge conflicts because other team members made changes
- After manually resolving conflicts, automated tests run and discover multiple issues
- The team rushes to fix these issues because the main branch is now broken
- At the end of the sprint, you implement a code freeze to prevent further changes before deployment
- Manual testing begins to ensure new features work and existing functionality isn't broken
- Someone on the team manually checks out the main branch, builds a Docker image, and pushes it to the repository
- Deployment configuration files are manually modified
- A senior team member manually connects to the server or Kubernetes cluster to deploy the application
- The entire process repeats for production deployment, with even higher stakes and anxiety
This manual approach is problematic for several reasons: it's error-prone, time-consuming, creates bottlenecks, and generates unnecessary stress. The human element introduces constraints at every stage, making the entire process inefficient and unreliable.
What is CI/CD and Why Does It Matter?
CI/CD stands for Continuous Integration and Continuous Delivery/Deployment. These practices represent a fundamental shift in how teams approach software development and deployment.
- Continuous Integration (CI): The practice of frequently integrating code changes into a shared repository, with automated testing to catch issues early
- Continuous Delivery (CD): The ability to release changes to production quickly and sustainably through automated build, test, and deployment processes
- Continuous Deployment: An extension of continuous delivery where every change that passes automated tests is automatically deployed to production
The primary goal of CI/CD is to remove manual processes and replace them with automation, making software delivery more efficient, reliable, and less stressful for development teams.
Implementing Continuous Integration: Catching Issues Early
The first step in transforming our chaotic manual workflow is implementing Continuous Integration. Instead of waiting until merge time to discover issues, we run tests at every stage of development.
- Run tests on feature branches before merging to main
- Test each branch individually to isolate issues
- Run tests on every commit to catch problems immediately
- Encourage developers to commit smaller changes more frequently
This approach produces several immediate benefits:
- Issues are caught faster and earlier in the development workflow
- Problems are simpler and isolated rather than complex and entangled
- Developers can fix issues while working on their current feature
- Stress doesn't pile up before release time
- Merge conflicts are reduced and easier to resolve
Tools like Jenkins, GitHub Actions, GitLab CI, and Azure DevOps provide robust platforms for implementing CI workflows. These tools can be configured to automatically run tests whenever code is pushed to a repository.
# Example GitHub Actions workflow for CI
name: Continuous Integration
on:
push:
branches: [ feature/*, develop ]
pull_request:
branches: [ main ]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up environment
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
Building a Comprehensive CI/CD Pipeline
With CI in place, the next step is automating the deployment process through Continuous Delivery or Deployment. This means setting up a pipeline that automatically builds, tests, and deploys your application whenever changes are merged to the main branch.
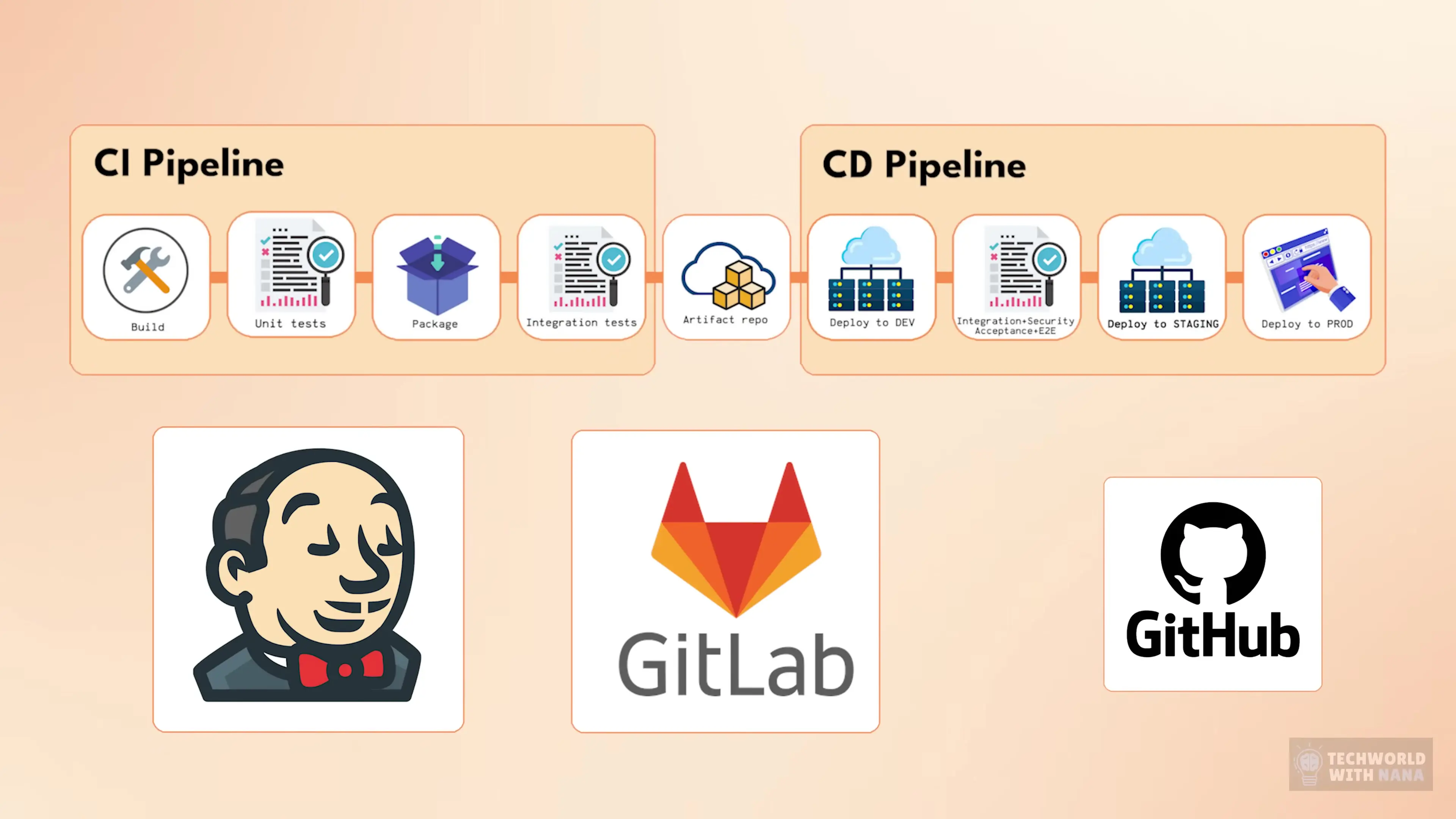
A typical CI/CD pipeline in a tool like Jenkins or GitHub Actions includes these stages:
- Build: Compile code, resolve dependencies, and create artifacts (like Docker images)
- Test: Run unit tests, integration tests, and other automated checks
- Deploy to Development: Automatically deploy to a development environment
- Test in Development: Run additional tests in the development environment
- Deploy to Staging: Deploy to a pre-production environment
- Test in Staging: Run production-like tests and validations
- Deploy to Production: Deploy to the production environment (may require manual approval)
The key is automating all these steps so they happen consistently and reliably without manual intervention. This requires:
- Configuring your CI/CD tool (Jenkins, GitHub Actions, GitLab CI, Azure DevOps)
- Setting up proper access controls and permissions
- Creating scripts for building, testing, and deploying your application
- Implementing environment-specific configurations
- Establishing monitoring and rollback procedures
// Example Jenkins pipeline
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'docker build -t myapp:${BUILD_NUMBER} .'
}
}
stage('Test') {
steps {
sh 'docker run myapp:${BUILD_NUMBER} npm test'
}
}
stage('Deploy to Dev') {
steps {
sh 'kubectl apply -f k8s/dev/deployment.yaml'
sh 'kubectl set image deployment/myapp myapp=myapp:${BUILD_NUMBER} -n dev'
}
}
}
}
Advanced CI/CD Strategies: Canary Deployments
As your CI/CD implementation matures, you can adopt more sophisticated deployment strategies. One popular approach is canary deployments, which reduce risk by gradually rolling out changes to a small subset of users before full deployment.
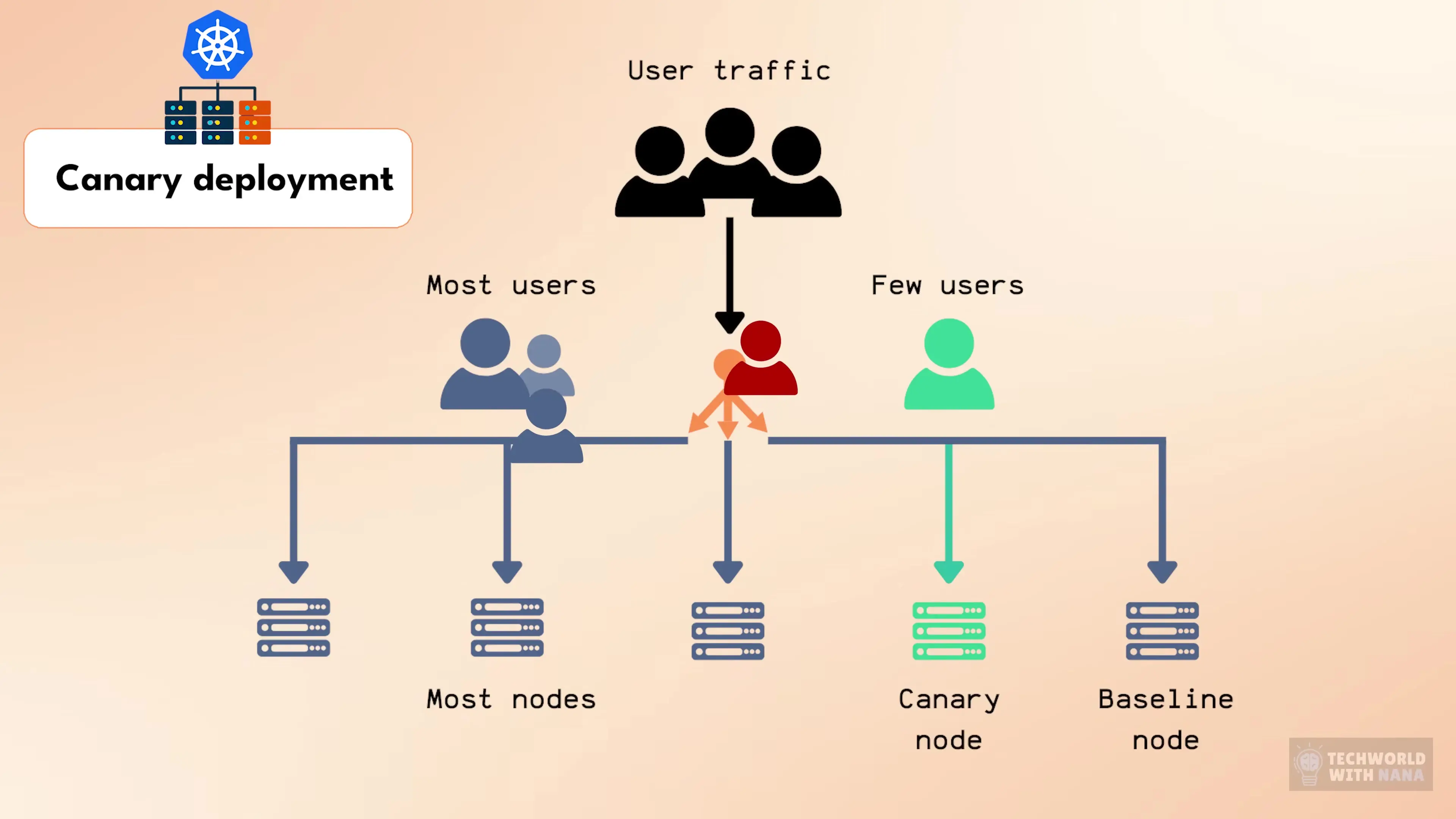
In a canary deployment:
- A new version is deployed to a small subset of your infrastructure
- A small percentage of users (typically 5-10%) are routed to the new version
- Metrics and errors are monitored closely
- If performance is acceptable, the deployment is gradually rolled out to more users
- If issues arise, the deployment can be quickly rolled back with minimal impact
This approach is particularly valuable for high-traffic applications where even small issues can affect thousands of users. Tools like Kubernetes, Istio, and AWS AppMesh make implementing canary deployments more manageable.
Implementing CI/CD in Popular Environments
GitHub-Based CI/CD Pipeline
GitHub Actions provides a powerful way to implement CI/CD directly within your GitHub repository. This approach is particularly popular for open-source projects and teams already using GitHub for source control.
# GitHub Actions workflow for a complete CI/CD pipeline
name: CI/CD Pipeline
on:
push:
branches: [ main ]
jobs:
build-and-deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Build Docker image
run: docker build -t myapp:${{ github.sha }} .
- name: Run tests
run: docker run myapp:${{ github.sha }} npm test
- name: Login to Docker Hub
uses: docker/login-action@v1
with:
username: ${{ secrets.DOCKER_HUB_USERNAME }}
password: ${{ secrets.DOCKER_HUB_ACCESS_TOKEN }}
- name: Push to Docker Hub
run: |
docker tag myapp:${{ github.sha }} username/myapp:latest
docker push username/myapp:latest
- name: Deploy to Kubernetes
uses: steebchen/[email protected]
with:
config: ${{ secrets.KUBE_CONFIG_DATA }}
command: set image deployment/myapp myapp=username/myapp:latest -n production
Jenkins CI/CD Pipeline
Jenkins remains one of the most flexible and widely-used CI/CD tools, particularly in enterprise environments. It offers extensive customization and plugin support.
// Jenkinsfile for a multi-environment pipeline
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'docker build -t myapp:${BUILD_NUMBER} .'
}
}
stage('Test') {
steps {
sh 'docker run myapp:${BUILD_NUMBER} npm test'
}
}
stage('Deploy to Dev') {
when { branch 'develop' }
steps {
sh 'kubectl apply -f k8s/dev/deployment.yaml'
sh 'kubectl set image deployment/myapp myapp=myapp:${BUILD_NUMBER} -n dev'
}
}
stage('Deploy to Staging') {
when { branch 'main' }
steps {
sh 'kubectl apply -f k8s/staging/deployment.yaml'
sh 'kubectl set image deployment/myapp myapp=myapp:${BUILD_NUMBER} -n staging'
}
}
stage('Deploy to Production') {
when { branch 'main' }
input {
message "Deploy to production?"
ok "Yes"
}
steps {
sh 'kubectl apply -f k8s/production/deployment.yaml'
sh 'kubectl set image deployment/myapp myapp=myapp:${BUILD_NUMBER} -n production'
}
}
}
}
Azure DevOps CI/CD Pipeline
Azure DevOps provides a comprehensive set of tools for implementing CI/CD, particularly well-suited for teams working with Microsoft technologies or deploying to Azure.
# azure-pipelines.yml
trigger:
- main
pool:
vmImage: 'ubuntu-latest'
stages:
- stage: Build
jobs:
- job: BuildJob
steps:
- task: Docker@2
inputs:
command: 'build'
Dockerfile: '**/Dockerfile'
tags: '$(Build.BuildId)'
- task: Docker@2
inputs:
command: 'push'
containerRegistry: 'dockerRegistry'
repository: 'myapp'
tags: '$(Build.BuildId)'
- stage: DeployDev
dependsOn: Build
jobs:
- deployment: DeployToDev
environment: 'Development'
strategy:
runOnce:
deploy:
steps:
- task: KubernetesManifest@0
inputs:
action: 'deploy'
kubernetesServiceConnection: 'dev-k8s'
namespace: 'dev'
manifests: '$(Pipeline.Workspace)/k8s/dev/*.yaml'
containers: 'myapp:$(Build.BuildId)'
The Benefits of Implementing CI/CD Pipelines
Implementing a CI/CD pipeline requires an initial investment of time and resources, but the benefits far outweigh the costs:
- Faster delivery: Changes reach users more quickly and frequently
- Improved quality: Automated testing catches issues before they reach production
- Reduced risk: Smaller, more frequent deployments are less likely to cause major issues
- Increased developer productivity: Developers spend less time on manual processes and more time coding
- Better collaboration: Standardized processes improve team coordination
- Reduced stress: Automated, reliable deployments eliminate deployment anxiety
- Improved feedback loop: Faster deployments mean quicker user feedback
CI/CD Best Practices
To get the most from your CI/CD implementation, follow these best practices:
- Commit small changes frequently to reduce integration complexity
- Maintain a comprehensive test suite that runs with every build
- Keep the build process fast to provide quick feedback
- Treat infrastructure as code and version it alongside your application
- Implement proper monitoring and alerting for your pipeline
- Secure your CI/CD pipeline with proper access controls
- Document your pipeline for team knowledge sharing
- Regularly review and optimize your pipeline for efficiency
- Implement feature flags to separate deployment from feature release
- Practice immutable infrastructure to ensure consistency across environments
Conclusion: From Manual Chaos to Automated Efficiency
Implementing CI/CD pipelines transforms software delivery from a manual, error-prone process into a streamlined, automated workflow. While setting up these pipelines requires initial effort, the long-term benefits in terms of reliability, efficiency, and developer satisfaction make it well worth the investment.
By embracing CI/CD practices, development teams can focus on what they do best—writing great code—while leaving the repetitive, error-prone tasks of building, testing, and deploying to automated systems. Whether you're using GitHub Actions, Jenkins, Azure DevOps, or another CI/CD tool, the fundamental principles remain the same: automate everything possible, test early and often, and deploy frequently in small increments.
Start your CI/CD journey by implementing continuous integration first, then gradually add continuous delivery and deployment capabilities as your team becomes more comfortable with the process. Before long, you'll wonder how you ever managed without it.
Let's Watch!
From Manual Chaos to Automated Delivery: CI/CD Pipeline Implementation Guide
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence