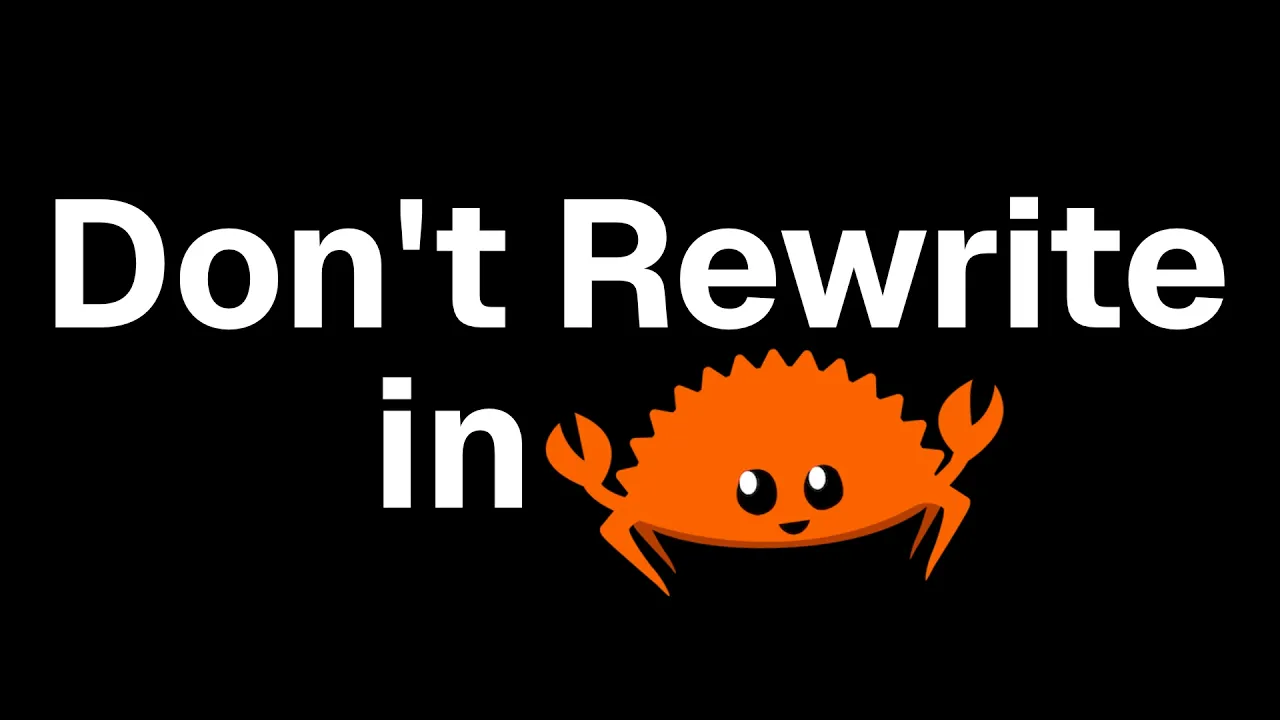
The JavaScript ecosystem is experiencing a significant trend: many popular development tools are being rewritten in 'faster' languages like Rust, Zig, and Go. While the promise of performance gains is enticing, the rush to abandon JavaScript may be premature. This article examines whether these rewrites deliver meaningful benefits or if optimizing existing JavaScript code could achieve similar results with less disruption.
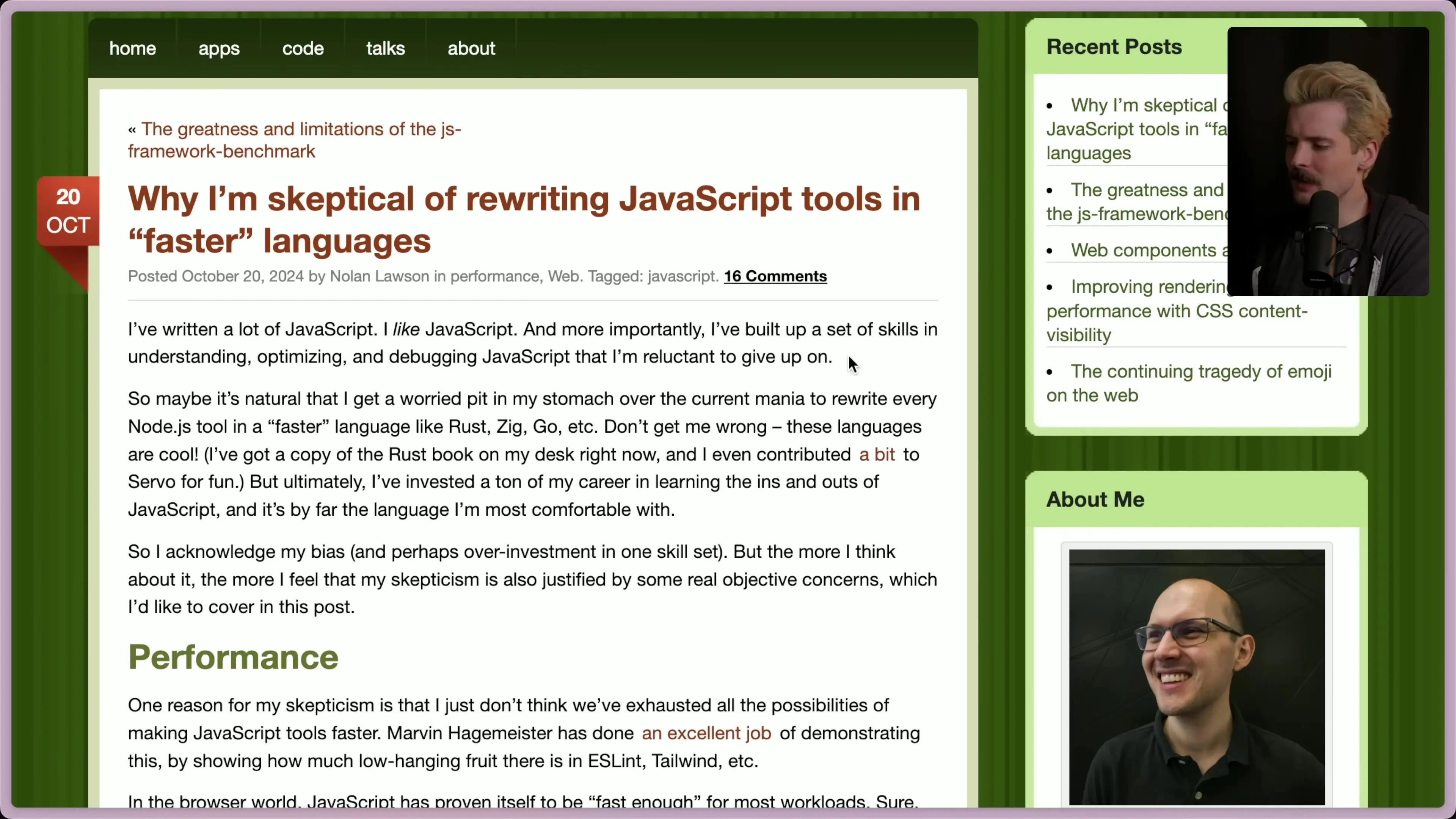
The JavaScript Performance Paradox
JavaScript has become ubiquitous across desktop applications (via Electron), backend services (via Node.js), and of course, frontend web development. Critics often question this proliferation, citing JavaScript's performance limitations compared to compiled languages like Rust. But is JavaScript truly too slow for these applications, or have we simply failed to optimize it properly?
In the browser world, JavaScript has proven itself capable of handling complex applications and intensive workloads. WebAssembly (WASM) exists for edge cases requiring extreme performance, but it remains a niche solution rather than a wholesale replacement for JavaScript. This suggests that for most applications, properly optimized JavaScript is fast enough.
Low-Hanging Fruit: JavaScript Optimization Techniques
Before rushing to rewrite tools in another language, consider the substantial performance gains possible through JavaScript optimization. Developers like Marvin Hagemeister have demonstrated impressive speed improvements in popular tools like ESLint and Tailwind by applying straightforward optimization techniques:
- Avoiding unnecessary type conversions
- Preventing function creation inside loops
- Optimizing parsing algorithms
- Reducing memory allocations
- Eliminating redundant operations
In one example, Hagemeister reduced Tailwind class name parsing time from 400ms to just 92ms through JavaScript optimizations alone—a 77% improvement without changing languages. This demonstrates that significant performance gains are often available through better JavaScript coding practices.
The Rewrite Fallacy: New Language vs. New Implementation
When a tool is rewritten from JavaScript to Rust and shows dramatic performance improvements, is the language change responsible, or is it simply the benefit of a fresh implementation? Consider these factors that contribute to faster rewrites regardless of language:
- Performance-focused design from the start (unlike many first-generation tools)
- Pre-established API surfaces that don't require experimental iterations
- Lessons learned from the original implementation's shortcomings
- Modern architectural approaches not available when the original was created
- Freedom from backward compatibility constraints
As developer Ryan Carniato points out, rewrites are often faster simply because they're rewrites—the second implementation benefits from all the knowledge gained from the first attempt. This means a JavaScript rewrite of a JavaScript tool could potentially achieve similar performance gains to a Rust rewrite.
The "Make it Work, Make it Right, Make it Fast" Principle
The software development adage "make it work, make it right, make it fast" applies perfectly to this situation. Many JavaScript tools were created with the primary goal of solving a problem (make it work), then refined for correctness and usability (make it right), but may have never reached the optimization stage (make it fast). The current wave of rewrites is essentially addressing this final step.
Fast JavaScript will always outperform slow JavaScript, just as fast Rust will outperform slow Rust. The language choice is only one factor in the performance equation.
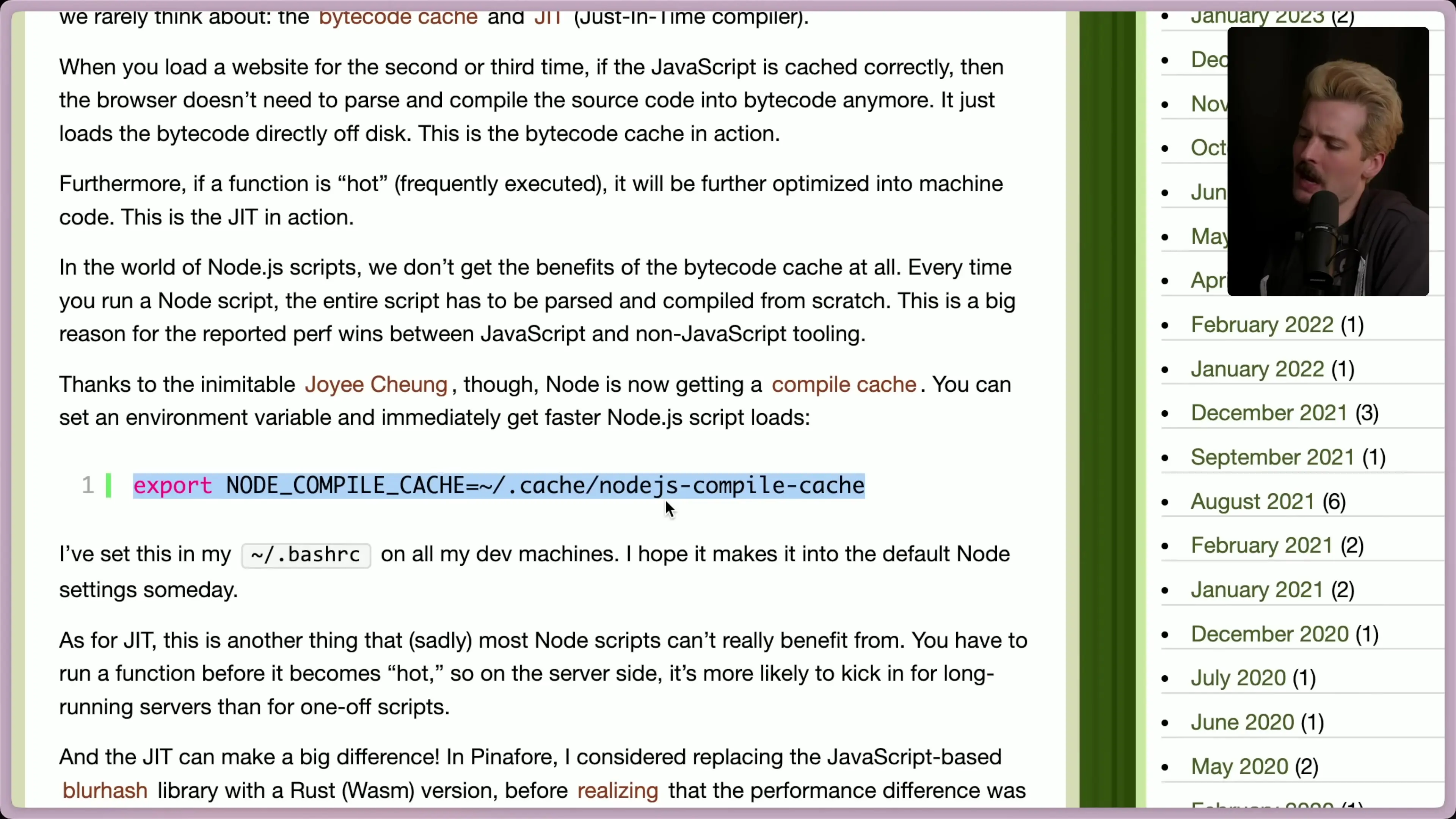
The Hidden Costs of Language Switching
While performance is a crucial consideration, it's not the only factor in evaluating whether to rewrite tools in a different language. Switching languages introduces several significant costs and risks:
- Developer velocity: JavaScript's ubiquity means most web developers can contribute to JS tools immediately
- Ecosystem compatibility: JavaScript tools naturally integrate with other JavaScript-based systems
- Maintenance burden: Maintaining tools in multiple languages requires broader expertise
- Community fragmentation: Splitting development across language communities can slow innovation
- Learning curve: New contributors face barriers when tools use less familiar languages
These factors help explain why, despite performance limitations, JavaScript has remained dominant in web development tooling. The productivity benefits often outweigh raw performance advantages, especially for tools that aren't run continuously or in performance-critical contexts.
JavaScript Performance Optimization Best Practices
Before deciding to rewrite a JavaScript tool in another language, consider implementing these optimization strategies:
// Instead of creating functions in loops
const handler = (item) => { /* process item */ };
items.forEach(handler);
// Instead of repeated type conversions
const count = Number(inputValue); // Convert once
if (count > 0) { /* use count multiple times */ }
// Use efficient data structures
const uniqueItems = new Set(items); // Instead of array with .includes() checks
Additionally, leverage these JavaScript performance tools and techniques:
- Profile code with Chrome DevTools or Node.js profiler to identify bottlenecks
- Use benchmarking libraries like Benchmark.js to compare implementation approaches
- Consider JIT-friendly coding patterns that help V8 optimize execution
- Implement caching strategies for expensive computations
- Utilize Node.js worker threads for CPU-intensive operations
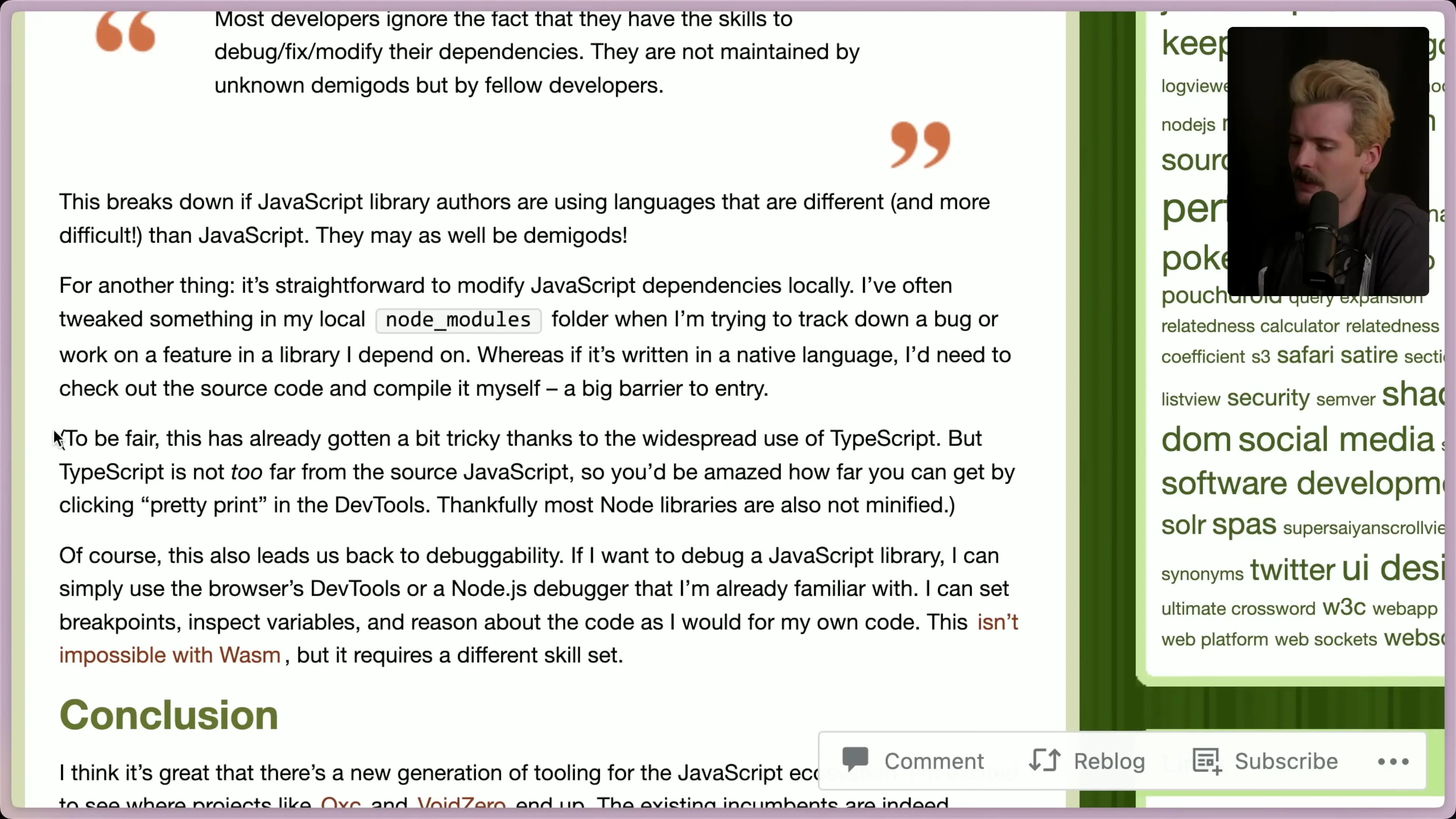
When Language Rewrites Make Sense
Despite the arguments for optimizing JavaScript, there are legitimate cases where rewriting in languages like Rust or Go is justified:
- For CPU-bound operations where JavaScript's single-threaded nature is a fundamental limitation
- When memory usage is a critical concern (JavaScript's garbage collection can be unpredictable)
- For tools that need to run in environments where JavaScript runtimes aren't available
- When the performance requirements are extreme and every millisecond counts
- When the tool is part of a larger ecosystem primarily built in another language
The key is making this decision based on measured performance requirements rather than following trends or assumptions about language performance.
Conclusion: Thoughtful Optimization Over Reflexive Rewrites
The current trend of rewriting JavaScript tools in languages like Rust offers genuine performance benefits in some cases. However, it's essential to recognize that many performance issues can be solved through better JavaScript optimization without sacrificing the ecosystem advantages and developer velocity that JavaScript provides.
Before embarking on a language rewrite, consider whether performance problems stem from fundamental language limitations or simply from unoptimized code. In many cases, a focused JavaScript optimization effort may deliver comparable performance improvements with far less disruption to your development workflow and ecosystem.
The next time you encounter a slow JavaScript tool, ask whether it needs a new language or just better code. The answer might surprise you—and save you considerable development time in the process.
Let's Watch!
Why JavaScript Is Fast Enough: Stop Rewriting Tools in Other Languages
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence