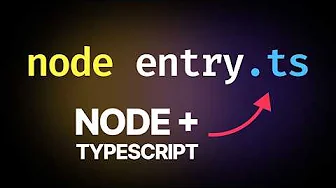
A significant milestone has been reached in the JavaScript ecosystem: Node.js finally supports TypeScript natively without requiring any additional configuration. This breakthrough feature, now available in Node.js 23, eliminates one of the major pain points developers faced when working with TypeScript in Node.js environments.
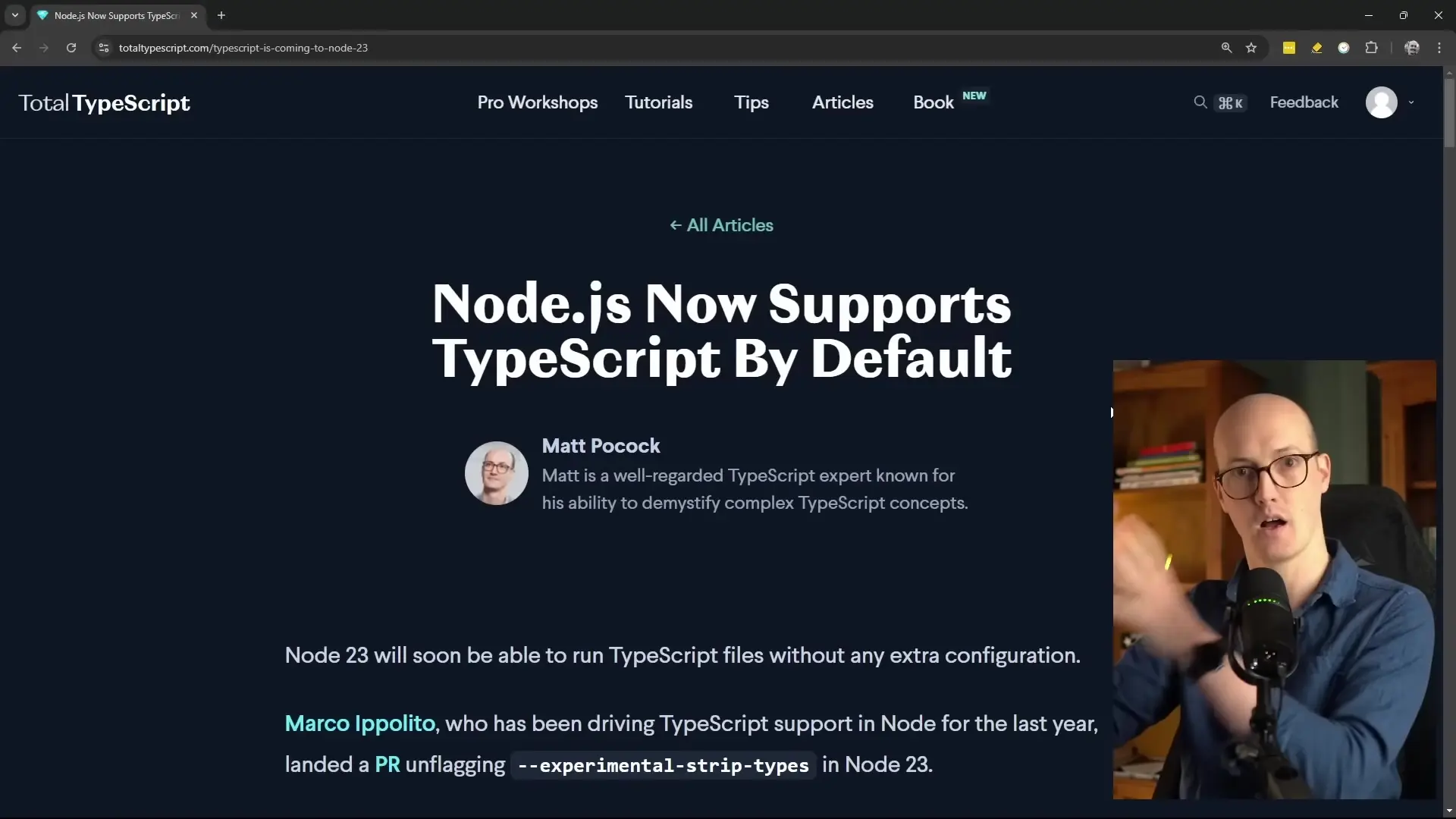
How Native TypeScript Support Works in Node.js
Previously, running TypeScript in Node.js required transpilation steps, additional dependencies, or development tools like ts-node. With Node.js 23, you can now directly execute TypeScript files without any extra steps. This functionality was initially introduced as an experimental feature in Node.js 22 using the '--experimental-strip-types' flag, but has now been made a standard feature in version 23.
// entry.ts
const message: string = "Hello, TypeScript in Node.js!";
console.log(message);
Running this file is now as simple as:
node entry.ts
No transpilation, no configuration, no extra flags—it just works.
Understanding the Implementation
Node.js achieves this native TypeScript support by using a streamlined version of the TypeScript compiler to strip out type annotations at runtime. This approach is focused on execution rather than type checking, meaning your code will run even if it contains type errors.
It's important to note that Node.js isn't performing type checking—it's simply removing the type annotations to make the code executable by the JavaScript engine. For full type checking capabilities, you'll still want to use the TypeScript compiler (tsc) or your IDE's type checking features during development.
Frequently Asked Questions About Node's Native TypeScript Support
- Does it type check your files? No, it only strips types for execution.
- What should my tsconfig.json look like? You can continue using your existing TypeScript configuration for type checking, but it's not required for execution.
- Does it support all TypeScript features? It supports most core TypeScript syntax, but complex features like namespaces may have limitations.
- Will it be backported to previous Node.js versions? Unlikely, as this is a major feature addition to Node.js 23.
What This Means for the JavaScript Ecosystem
This development represents a significant evolution in Node.js's positioning within the JavaScript runtime landscape. While newer runtimes like Deno and Bun have offered native TypeScript support as a key differentiator, Node.js—despite moving at a more measured pace—has now closed this gap.
The addition of native TypeScript support shows that Node.js continues to evolve and adapt to modern JavaScript development practices. As the most widely used JavaScript server runtime, Node.js's embrace of TypeScript without configuration makes the technology even more accessible to developers who prefer the safety and developer experience that TypeScript provides.
How to Get Started with Native TypeScript in Node.js
- Update to Node.js version 23 or later
- Create a TypeScript file with your code
- Run it directly with 'node yourfile.ts'
- Continue using TypeScript compiler or IDE for type checking during development
# Check your Node.js version
node --version
# Should output v23.x.x or higher
# Run a TypeScript file directly
node app.ts
The Collaborative Effort Behind This Feature
This advancement is the result of dedicated work from volunteers contributing to the Node.js project, highlighting the power of open-source collaboration. While commercial runtimes might move faster with full-time development teams, Node.js's community-driven approach ensures changes are thoroughly vetted and stable before becoming standard features.
Conclusion
The addition of native TypeScript support to Node.js represents a significant step forward for the platform. By eliminating configuration barriers, Node.js has made TypeScript more accessible to millions of developers worldwide. While this implementation focuses on execution rather than type checking, it removes a major friction point in the TypeScript development workflow.
As the JavaScript ecosystem continues to evolve, Node.js demonstrates that it remains committed to incorporating modern development practices while maintaining its position as the most widely-used server-side JavaScript runtime. This update shows that competition in the ecosystem ultimately benefits developers, as all platforms continue to improve and innovate.
Let's Watch!
Node.js Now Natively Supports TypeScript: Game-Changing Update for Developers
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence