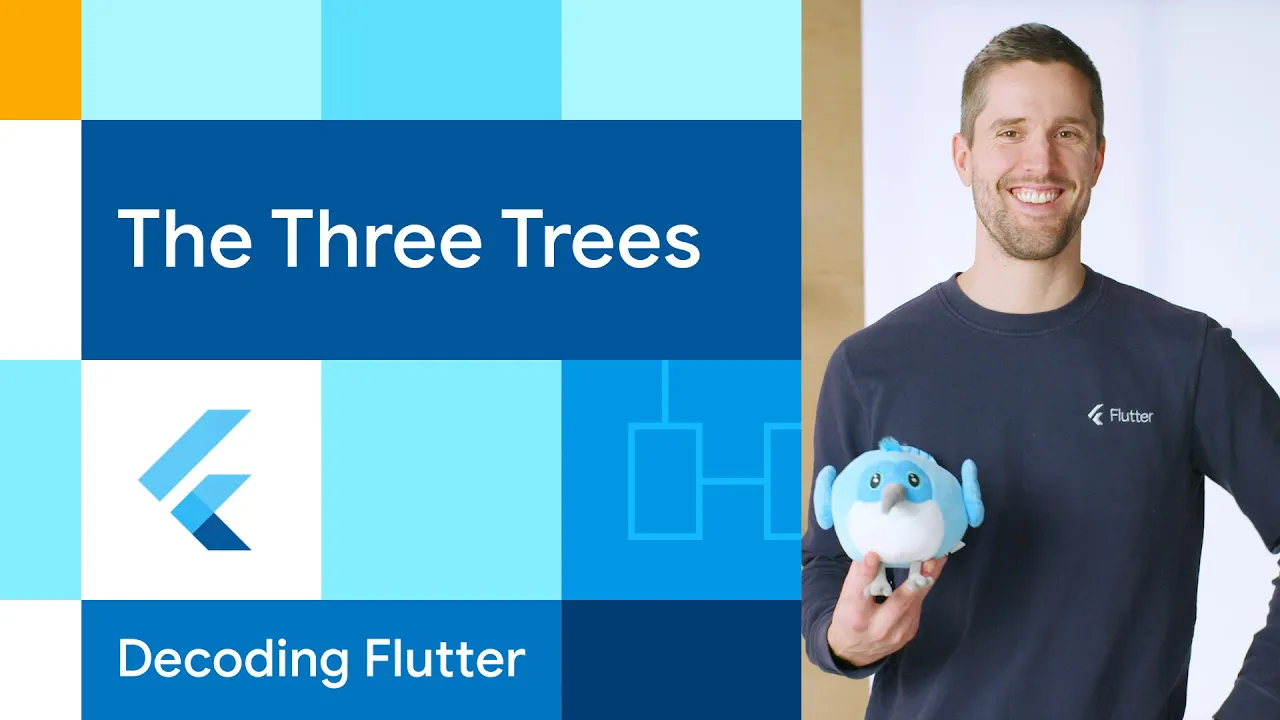
Decoding Flutter's Three-Tree Architecture: A Visual Guide for Developers
Decoding Flutter's Three-Tree Architecture: A Visual Guide to How Flutter Works Internally
Flutter has emerged as one of the most powerful cross-platform development frameworks, but understanding how it actually transforms your Dart code into beautiful, performant applications across multiple platforms requires diving deeper into its architecture. This article deconstructs Flutter's internal structure, exploring the systems that make it uniquely powerful for developers.
At its core, Flutter is a declarative, multi-platform UI framework written in Dart and heavily supported by Google. This foundation provides Flutter with several key characteristics that make it stand out in the development ecosystem:
Flutter's declarative UI system represents a fundamental shift from imperative UI frameworks. In Flutter, you don't directly manipulate the interface (like "change this button's color" or "hide this view"). Instead, you describe what the UI should look like based on the current application state. When the state changes, Flutter reruns your build methods to generate a new UI description, then efficiently updates only what needs to change.
This approach simplifies state management and creates a predictable relationship between application state and UI. It's the foundation of Flutter's declarative ui system, making your interfaces reactive to data changes without complex update logic.
Dart is intrinsically tied to Flutter's success and capabilities. Released by Google in 2013, Dart brings several advantages to Flutter development:
Dart's ability to compile directly to native code without runtime interpretation enables Flutter to achieve impressive performance even on lower-end devices. This compilation strategy is central to how flutter compiles code and represents a significant advantage over interpreted solutions.
The Flutter development workflow begins with installing the Flutter SDK, which adds the Flutter CLI tool to your environment. This tool provides essential commands for managing your Flutter projects:
The flutter app build process transforms your Dart code into platform-specific binaries through a sophisticated pipeline that handles everything from dependency resolution to asset bundling and compilation.
Widgets are the fundamental building blocks in Flutter. Every UI element in Flutter is a widget, from simple text and buttons to complex layouts and animations. A typical Flutter widget follows this structure:
class MyCustomWidget extends StatelessWidget {
final String title;
const MyCustomWidget({Key? key, required this.title}) : super(key: key);
@override
Widget build(BuildContext context) {
// Access runtime information via context
final screenSize = MediaQuery.of(context).size;
return Container(
width: screenSize.width * 0.8,
child: Text(title),
);
}
}
The build method is the heart of every widget, where you define what should be rendered. It receives a BuildContext parameter that provides access to crucial runtime information like screen dimensions, accessibility settings, and theme data. The flutter build context serves as a gateway to the widget's position in the widget tree and the services available at that position.
One of Flutter's most powerful architectural features is its separation of concerns through three distinct tree structures that work together to render your UI:
This flutter component hierarchy enables the framework to efficiently update only what needs to change when your application state changes. It's also what powers Flutter's famous hot reload feature, allowing developers to see code changes almost instantly without losing application state.
Flutter's stateful hot reload is a game-changing feature for developer productivity. It allows you to inject updated source code into a running Dart VM, after which the framework automatically rebuilds the widget tree, preserving the application state. This means you can make UI changes and see them reflected in under a second, all without restarting your app or losing your current navigation state.
The flutter hot reload architecture is possible because of the clear separation between the persistent element tree (which maintains state) and the disposable widget tree (which defines the UI structure). This separation is a cornerstone of the flutter framework flow.
Unlike most cross-platform frameworks that wrap native UI components, Flutter draws every pixel of your UI directly to a canvas provided by the platform. This approach gives Flutter complete control over the rendering process and ensures consistent behavior across platforms.
Historically, Flutter has used Skia, the same open-source 2D graphics engine that powers Google Chrome and Android, as its rendering backend. More recently, the Flutter team has been developing Impeller, a new rendering engine designed specifically for Flutter's needs to achieve more consistent performance across devices.
The flutter rendering process involves transforming the render tree into a series of drawing commands that are executed by the rendering engine. This process includes layout calculation, painting, and compositing, all optimized for performance.
Flutter's architecture is often visualized as a layer cake with distinct components working together:
This flutter architecture diagram illustrates how your Dart code travels through these layers to become a native application. The framework layer processes your widget tree, the engine handles rendering and platform communication, and the embedder integrates with the host platform.
Understanding the flutter vs native architecture differences helps explain Flutter's unique advantages:
The flutter vs native rendering approach gives Flutter the ability to deliver pixel-perfect UIs across platforms without compromise, though it does mean the framework must implement all UI components from scratch.
Flutter's architecture represents a bold reimagining of cross-platform development. By controlling the entire rendering pipeline, leveraging Dart's compilation capabilities, and organizing code through a three-tree structure, Flutter delivers on its promise of beautiful, fast, and consistent applications across platforms.
For developers looking to understand flutter deeply, appreciating these architectural decisions provides valuable context for why Flutter works the way it does. Whether you're building simple apps or complex enterprise solutions, the flutter framework structure gives you powerful tools to realize your vision with less compromise than traditional cross-platform approaches.
As Flutter continues to evolve with improvements like the Impeller rendering engine, its architecture remains focused on the core principles that have made it successful: developer productivity, rendering fidelity, and cross-platform consistency.
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence