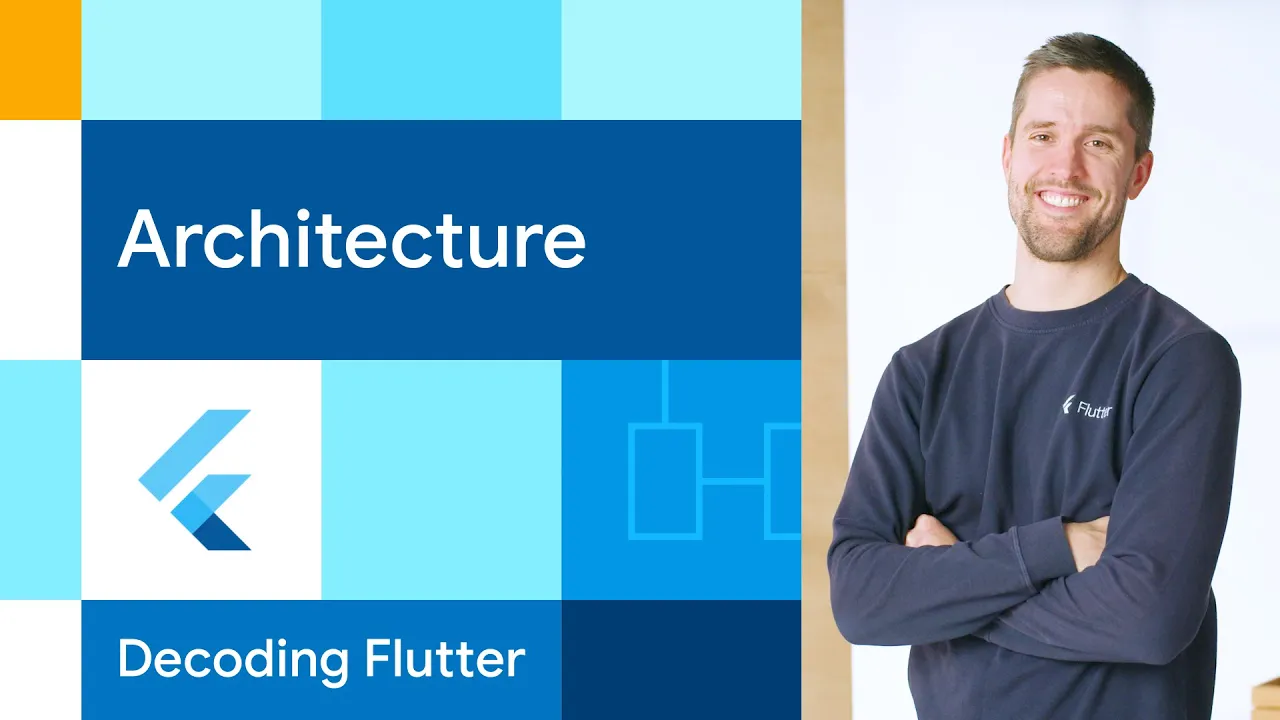
Decoding Flutter Architecture: How The Framework Actually Works
Decoding Flutter Architecture: How The Framework Actually Works Under The Hood
Flutter's elegant UI framework is built upon a sophisticated architecture that might seem mysterious at first glance. At its core, Flutter operates through three interconnected tree structures that work together to create the fluid, reactive UI experience developers love. Understanding these trees is key to mastering Flutter development and optimizing your applications.
Flutter's architecture revolves around three primary trees that handle different aspects of the UI rendering process:
These trees work in concert to provide Flutter's declarative API that makes UI development so intuitive while maintaining excellent performance. Let's explore each tree in detail to understand how Flutter works internally.
Widgets are the building blocks of Flutter applications and form the first tree in Flutter's architecture. They provide the declarative API that developers interact with most of the time. A key characteristic of widgets is that they are immutable and disposable - they're discarded and rebuilt whenever the UI needs to change.
This disposable nature might seem wasteful, but it's actually what makes Flutter's programming model so clean and straightforward. Dart's efficiency makes this approach viable and performant.
Flutter widgets come in several flavors, each serving different purposes:
As a Flutter developer, you'll spend most of your time (over 99%) working with StatelessWidget and StatefulWidget. Let's understand the key differences between these two common widget types.
StatelessWidget is the simpler of the two. It's instantiated by its parent widget's build method and lives only until its part of the UI needs to rebuild. It has a constructor that accepts parameters and a build method that assembles more widgets. StatelessWidgets are perfect for UI components that depend solely on their configuration and don't need to maintain state.
StatefulWidget is more complex and powerful. While the widget itself is still immutable and disposable, it's backed by a longer-lived State object that persists between rebuilds. This State object is where you maintain information that changes over time, like text field contents, animation values, or toggle states.
You should use StatefulWidget when you need:
A key structural difference is that StatefulWidgets relocate their build method to their State object, which is managed by Flutter's second tree - the Element Tree.
While widgets are the stars of the show, elements are the behind-the-scenes directors that make everything work. The Element Tree lives behind the Widget Tree and consists of longer-lived objects that serve as the glue between widgets and the rendering system.
Elements perform several critical functions:
Flutter developers rarely write custom elements, but they interact with them constantly through the BuildContext parameter that appears in build methods. In fact, BuildContext is actually just the Element itself, making itself available for operations like looking up inherited widgets or getting theme data.
When a new widget is added to the Widget Tree, Flutter calls its createElement method, and the resulting Element is attached to the Element Tree. This ensures that the Widget Tree and Element Tree always maintain the same structure.
As Flutter continues traversing the Widget Tree, it's actually the Element that calls its widget's build method, passing itself in as the BuildContext parameter. This produces more widgets, and the process continues recursively down the tree.
When your UI changes (for example, during an animation), the Widget Tree is rebuilt with new widget instances. The Element Tree, however, persists as long as possible. Elements attempt to pair with new widgets at the same position in the tree, maintaining state and minimizing the work needed to update the UI.
For StatefulWidgets, the Element keeps the associated State object alive across widget rebuilds. This is how Flutter maintains state even though widgets themselves are constantly being discarded and recreated.
The third major tree in Flutter's architecture is the RenderObject Tree. RenderObjects are created by RenderObjectWidgets and are responsible for the actual layout and painting of your UI.
Unlike regular widgets, RenderObjectWidgets don't have a build method. Instead, they implement createRenderObject and updateRenderObject methods that produce and update RenderObjects in the RenderObject Tree.
RenderObjects have several key responsibilities in the rendering pipeline:
RenderObjects don't directly produce pixels on the screen. Instead, they generate drawing commands that are later executed by Flutter's rendering engine (either Skia or Impeller, depending on your platform and Flutter version).
While most Flutter developers don't need to write custom RenderObjects often, understanding this layer is valuable for creating complex custom UI components or optimizing performance-critical parts of your application.
Flutter's three-tree architecture creates a highly layered and abstracted rendering system. Each layer transforms the data from the previous layer, moving one step closer to the final pixel buffer that appears on screen.
This layered approach allows Flutter to maintain its developer-friendly API while still achieving excellent performance across platforms. The separation of concerns between these trees enables Flutter to efficiently update only the parts of the UI that actually changed.
While the Widget, Element, and RenderObject trees are the most prominent in Flutter's architecture, there are other specialized tree structures that handle specific aspects of the UI:
These additional trees work alongside the main three to create Flutter's comprehensive UI framework.
Understanding Flutter's three-tree architecture has several practical benefits for developers:
Tools like Flutter DevTools provide visualizations of these trees, allowing you to inspect your application's structure and performance characteristics in real-time.
Flutter's three-tree architecture - Widget, Element, and RenderObject - forms the foundation of its powerful UI framework. This architecture enables Flutter to provide a clean, declarative API for developers while maintaining excellent performance across platforms.
By understanding how these trees interact, you'll gain deeper insight into how Flutter works internally, allowing you to write more efficient code, debug issues more effectively, and create more sophisticated UI components. As you continue your Flutter journey, keep these architectural concepts in mind - they'll serve as a valuable mental model for understanding the framework's behavior.
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence