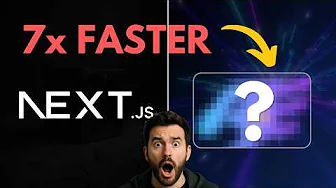
If you're a React developer who's been frustrated with Next.js becoming increasingly complex and slower with each release, there's a compelling alternative worth considering. Fastify with React is emerging as a powerful option that offers significantly better performance while giving developers more control over server code.
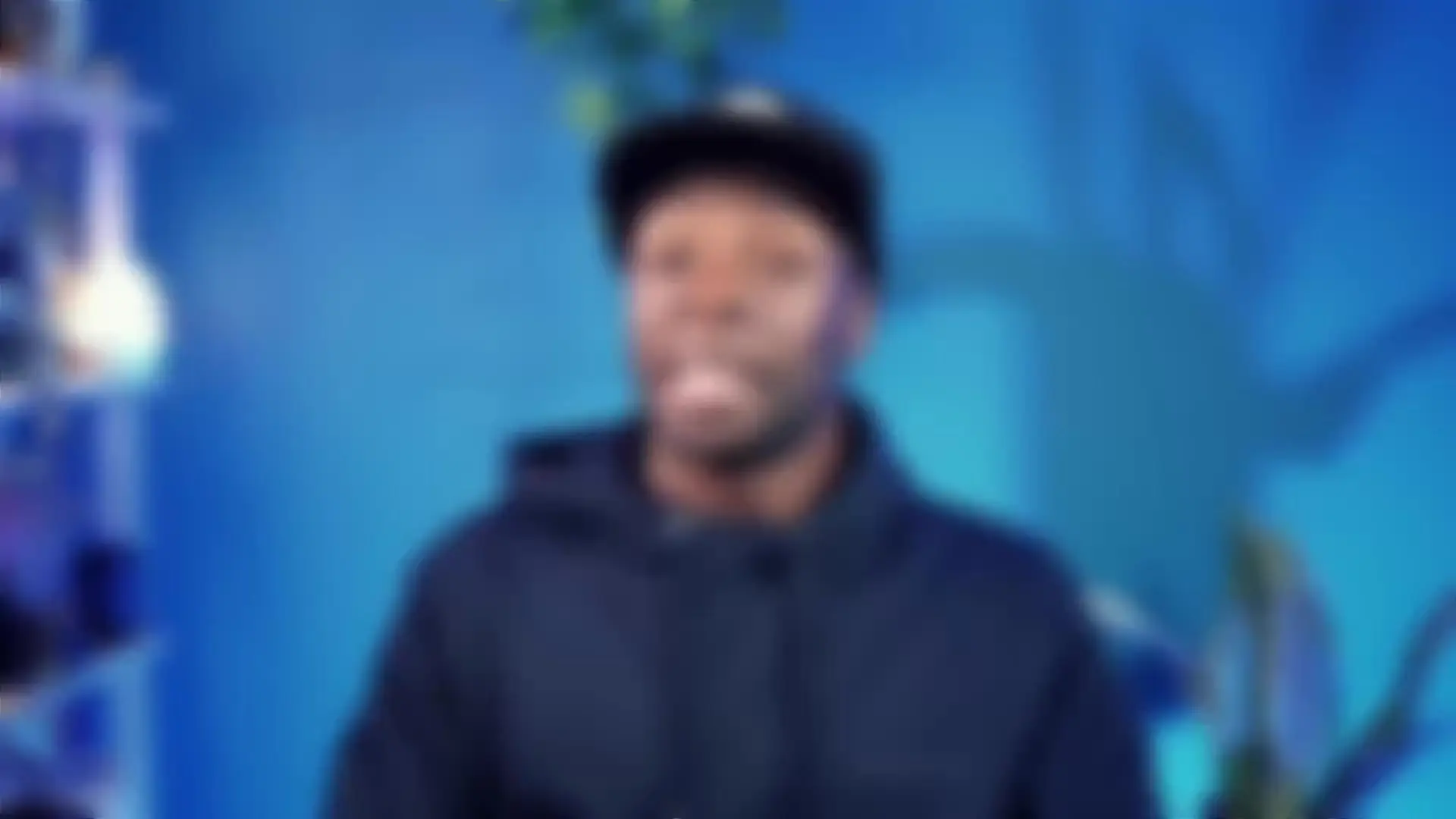
Understanding Fastify with React
Fastify is a Node.js micro framework similar to Express.js but with significantly lower overhead, resulting in much better performance. Fastify React is a React renderer specifically designed for Fastify that works through Vite, allowing developers to write React components and render them on the server using Fastify's powerful engine.
The main focus of Fastify React is server-side rendering (SSR) and client-side hydration. Unlike Next.js, which has grown to include numerous features and complexities, Fastify React takes a more focused approach. This streamlined architecture is actually one of its strengths, as it provides developers with greater control and transparency over their server code.
Performance Comparison: Fastify vs Next.js
Real-world testing has shown remarkable performance differences between Fastify with React and Next.js. In benchmark tests, Fastify React was able to handle approximately 1,000 requests every 10 seconds, while Next.js managed only about 400 requests on the same application.
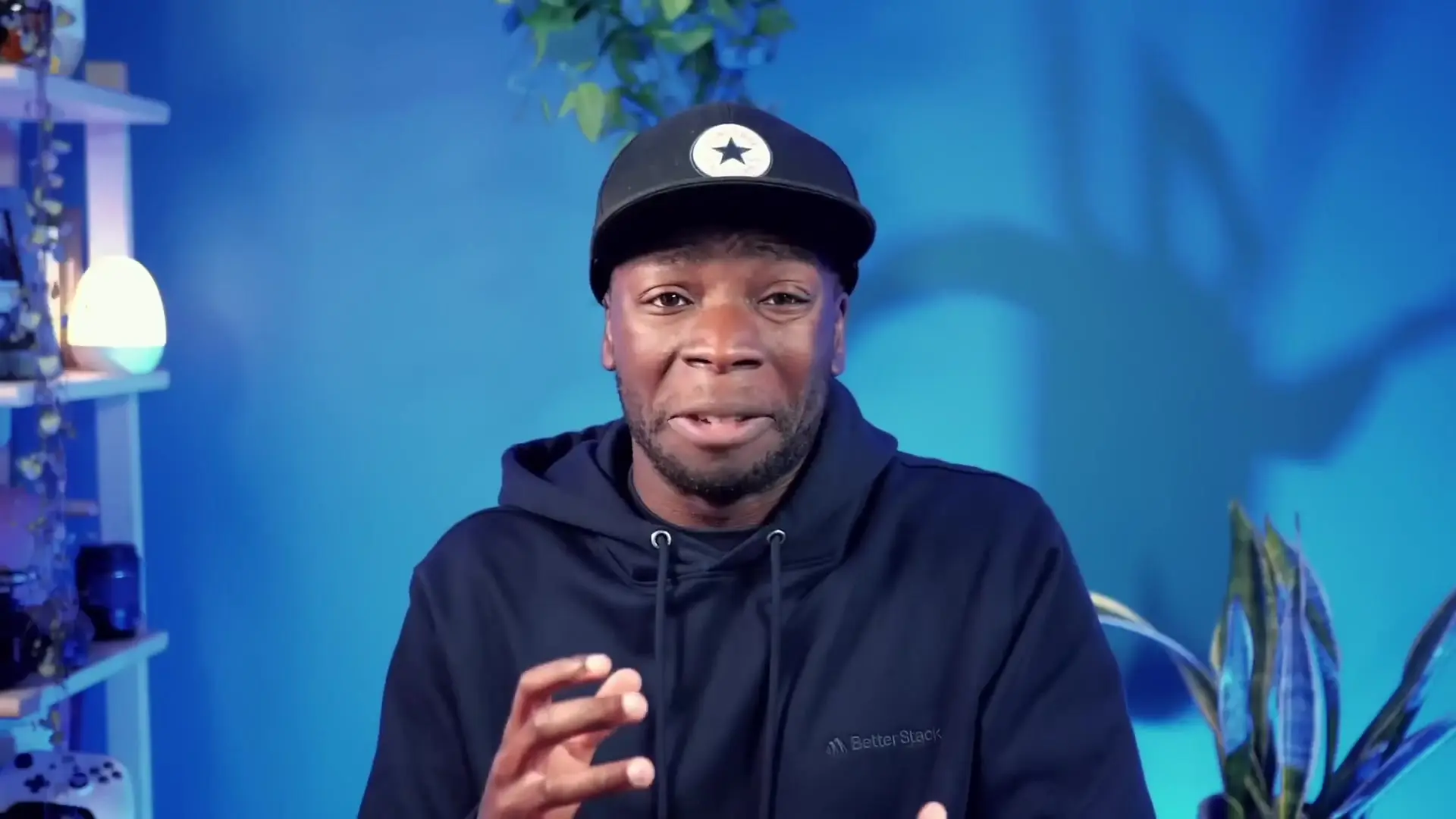
According to benchmark tests run by one of the Fastify maintainers, Next.js achieved just 49 requests per second compared to Fastify with React at 347 requests per second - a difference of over 7x in performance.
Benchmark Testing Methodology
To ensure fair comparison, tests were conducted using identical projects for both frameworks. The test application rendered a spiral pattern on the server side, with both implementations providing the same visual output to users.
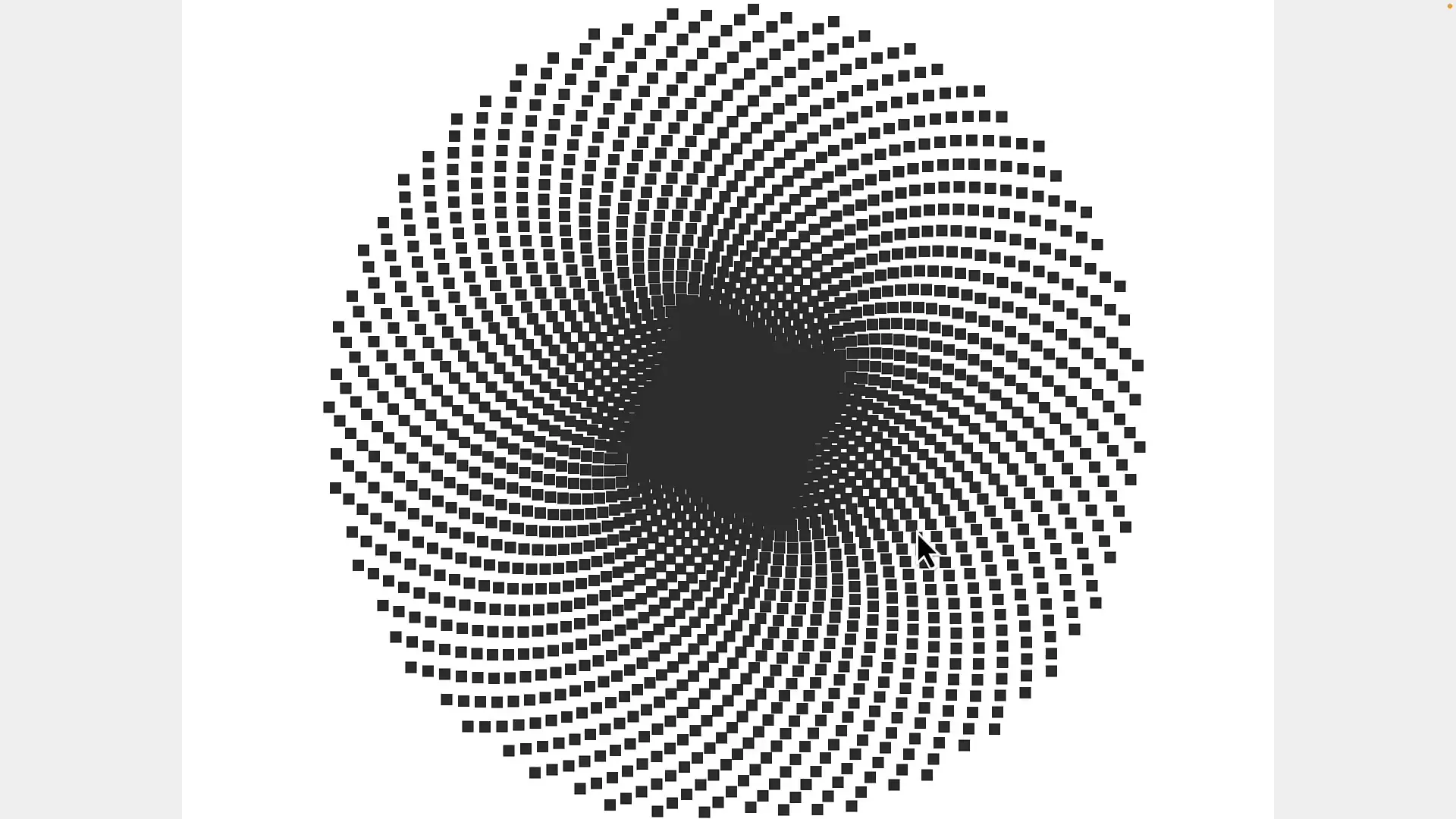
Using AutoCannon for load testing, the Fastify React implementation was able to handle 3,000 requests in 10 seconds with an average of 271.2 requests per second. The Next.js implementation of the same application managed only 520 requests in the same timeframe, averaging just 51 requests per second.
Real-World Application Comparison
To provide a more practical comparison, a to-do list application was built in both Next.js and Fastify with React. This application utilized React hooks like useContext and server actions to represent a more typical production scenario.
- The Next.js application was hosted on Vercel
- The Fastify application was deployed on a Digital Ocean droplet
- Both were tested with AutoCannon under similar conditions
- Tests were conducted on warm starts to ensure fair comparison
In this more complex application scenario, the performance gap narrowed but Fastify still maintained an edge. The Next.js application handled 5,000 requests in 10 seconds with an average of 467 requests per second, while the Fastify React application achieved 487.8 requests per second - still outperforming Next.js, though by a smaller margin.
Technical Implementation of Fastify with React
Setting up Fastify with React involves creating a Fastify server file that configures the Fastify Vite project and runs the server on a specified port. The Vite configuration includes setting up the vite-react and vite-fastify-react plugins, with HTML that includes mounting points for JavaScript hydration.
// Example Fastify server setup
const fastify = require('fastify')({ logger: true })
const fastifyReact = require('@fastify/react')
// Register the React renderer
fastify.register(fastifyReact, {
root: __dirname,
vite: {
plugins: [
require('@vitejs/plugin-react')(),
require('vite-plugin-fastify-react')()
]
}
})
// Define routes
fastify.get('/', async (request, reply) => {
return reply.react('App')
})
// Start server
fastify.listen({ port: 3000 }, (err) => {
if (err) throw err
})
When running in production, all code is rendered on the server, providing fully server-side rendered HTML to the client. This approach offers excellent performance while maintaining the developer experience of working with React.
When to Choose Fastify vs Next.js
The decision between Fastify with React and Next.js depends largely on your specific requirements and hosting environment:
- Choose Fastify with React when: You need maximum performance, want more control over your server code, are hosting on your own infrastructure, or find Next.js too complex for your needs.
- Stick with Next.js when: You're hosting on Vercel (which optimizes Next.js performance), need the extensive feature set and ecosystem, or prefer a more established framework with broader community support.
It's worth noting that hosting Fastify on Vercel isn't as straightforward as using Next.js, which is expected given Vercel's natural optimization for their own framework. However, when running on standard infrastructure, Fastify consistently outperforms Next.js in benchmarks.
Limitations of Fastify with React
While Fastify with React offers impressive performance, it's important to understand its limitations. The team behind Fastify React explored implementing React Server Components but encountered challenges with documentation gaps and compatibility issues between Webpack and Vite's ESM imports.
Additionally, Fastify React doesn't offer the full feature set that Next.js provides. However, this can be viewed as an advantage for developers who prefer a more focused tool that does one thing exceptionally well rather than attempting to solve every possible use case.
Conclusion: Is Fastify the Future for React Developers?
For React developers seeking alternatives to Next.js, Fastify with React represents a compelling option that delivers significantly better performance in many scenarios. The framework's focus on speed and simplicity makes it particularly attractive for applications where performance is critical and developers want more direct control over their server-side code.
While Next.js continues to dominate the React framework landscape, especially for developers deploying to Vercel, Fastify with React demonstrates that there are viable alternatives that can offer substantial performance improvements. As the React ecosystem continues to evolve, having more high-performance options benefits the entire community.
If you're experiencing performance bottlenecks with Next.js or simply want to explore a more lightweight approach to server-side rendering with React, Fastify with React deserves serious consideration for your next project.
Let's Watch!
Fastify vs Next.js: 7x Faster Performance for React Developers
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence