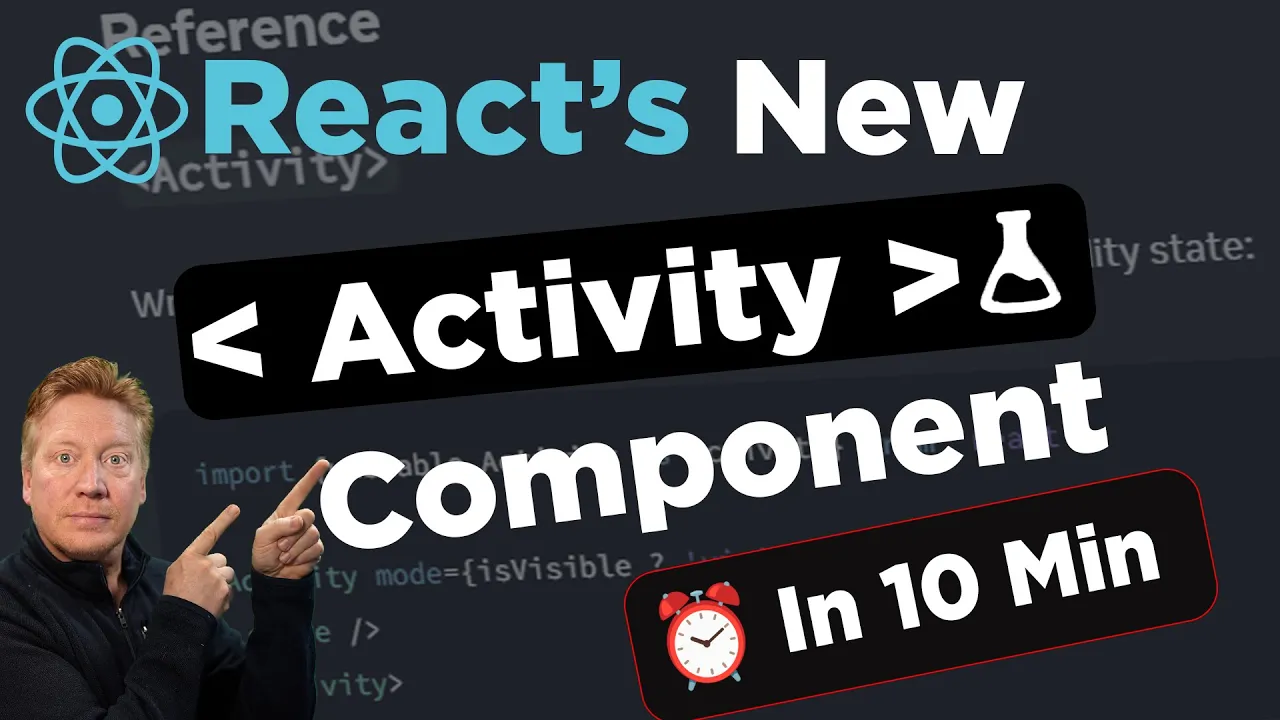
React's experimental release introduces a powerful new tool for performance-minded engineers: the Activity component. If you're building large systems like dashboards or data-intensive applications, this feature could be the performance breakthrough you've been waiting for. Let's explore how this component works and why it represents a significant advancement in React's rendering capabilities.
The Challenge: Component Visibility in Complex UIs
When building dashboard applications with numerous data visualizations, developers typically face a dilemma when toggling component visibility. There are traditionally two approaches, each with significant drawbacks:
- Mounting/unmounting components from the DOM: Provides good performance when components are hidden, but incurs a heavy performance cost when remounting
- Using CSS to hide/show components: Keeps components ready for instant display, but renders everything constantly, causing major performance issues
Neither approach is ideal for performance-critical applications like dashboards with multiple charts, graphs, or complex data visualizations. This is where React's experimental Activity component enters the picture, offering a novel third option.
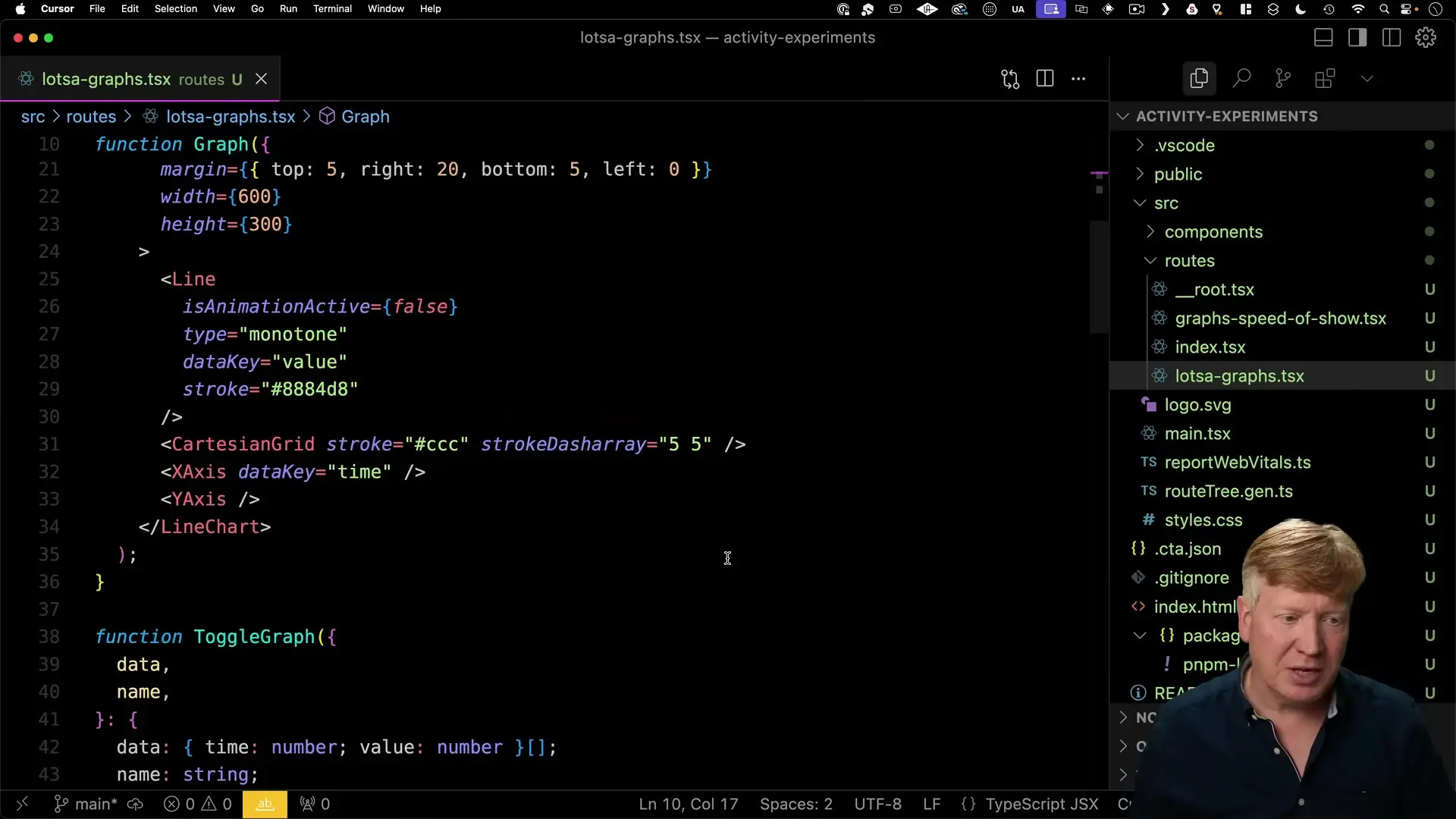
Introducing the Activity Component
The Activity component is available in React's experimental builds and provides a middle ground between the two traditional approaches. It allows components to remain in the virtual DOM even when they're not visible, updating them in low-priority background tasks while keeping them ready for immediate display when needed.
import { unstable_Activity as Activity } from 'react';
function ToggleGraph({ data, name }) {
const [isVisible, setIsVisible] = useState(false);
return (
<div>
<button onClick={() => setIsVisible(!isVisible)}>
{isVisible ? 'Hide' : 'Show'} {name}
</button>
<Activity mode={isVisible ? 'visible' : 'hidden'}>
<Graph data={data} name={name} />
</Activity>
</div>
);
}
As shown in the code above, using the Activity component is straightforward. You import it with the unstable_ prefix (indicating its experimental status) and wrap your component with it, providing a mode prop that can be either 'visible' or 'hidden'.
How Activity Component Works Behind the Scenes
The magic of the Activity component lies in how React handles the rendering process:
- When in 'hidden' mode, the component still renders to the virtual DOM, but in a low-priority task
- React intelligently determines how many hidden components to process based on your device's performance capabilities
- The virtual DOM representations stay updated, making them instantly available when switched to 'visible' mode
- User interactions remain responsive because high-priority events take precedence over background rendering tasks
- When switching to 'visible' mode, React can quickly apply the pre-computed virtual DOM to the actual DOM
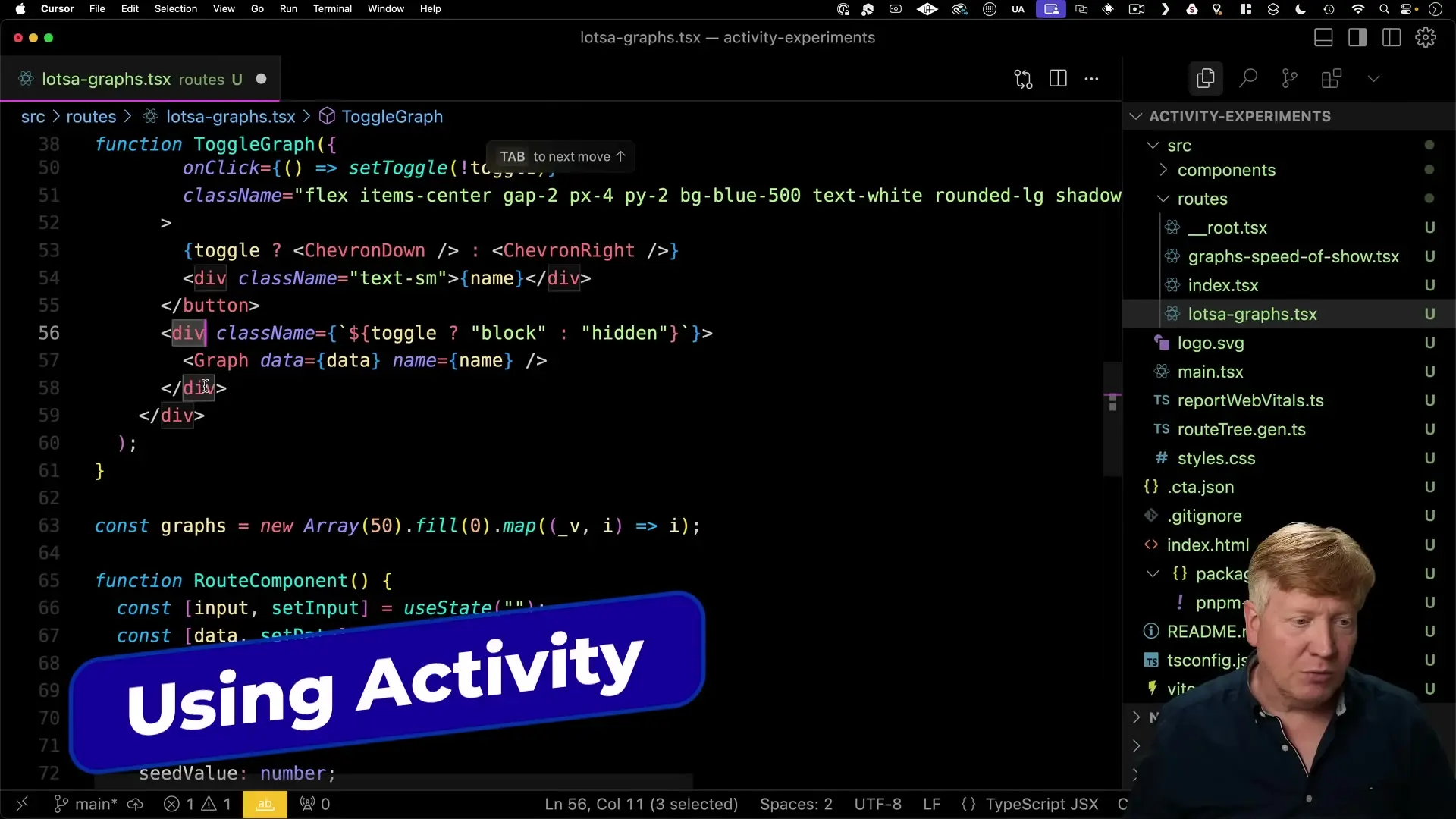
Performance Benefits: Measurable Improvements
The performance advantages of the Activity component become evident when measuring render times in a dashboard application with multiple graphs. In a test scenario with 50 data visualizations, we can observe significant improvements:
- Traditional mounting approach: ~480ms to show a hidden graph (full rerender and DOM insertion)
- Activity component approach: ~180ms to show a hidden graph (applying pre-computed VDOM to DOM)
- Maintained frame rates close to 60fps even with multiple visible components
- Responsive user input even during background rendering processes
These improvements are particularly valuable for data-intensive applications where rendering performance directly impacts user experience. The Activity component essentially gives you the best of both worlds: the performance benefits of conditional rendering with the immediate availability of CSS-based toggling.
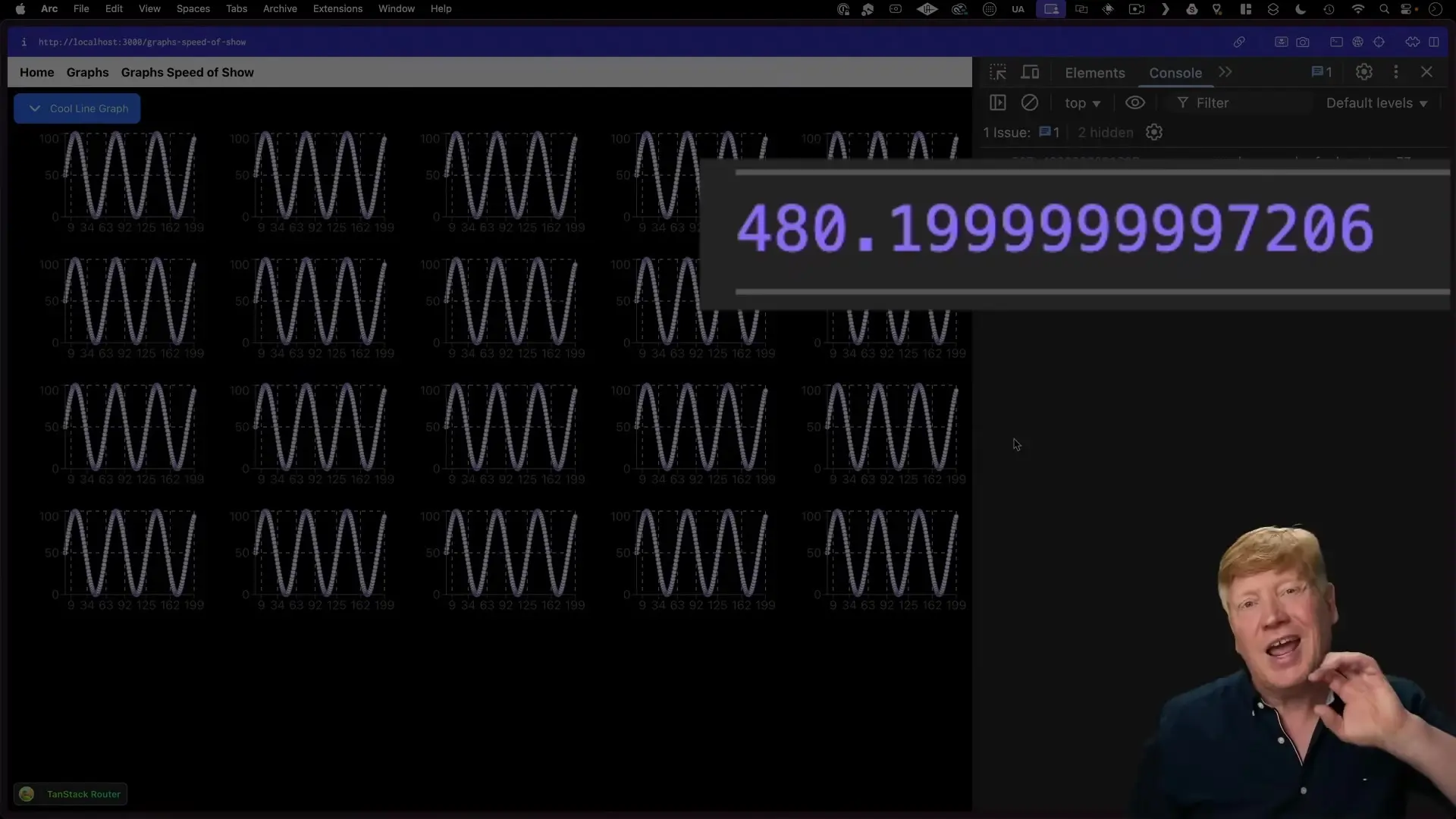
When to Use the Activity Component
The Activity component is particularly beneficial in these scenarios:
- Dashboard applications with multiple data visualizations
- UIs with complex components that are expensive to render
- Applications where users frequently toggle between different views
- Systems where maintaining state between visibility changes is important
- Performance-critical applications where every millisecond of rendering time matters
Limitations and Considerations
While the Activity component offers significant performance benefits, it's important to understand its limitations:
- It won't solve performance issues caused by rendering too many components simultaneously
- As an experimental feature, the API may change before official release
- The number of background-rendered components varies based on device performance
- There's still some performance cost to keeping components updated in the virtual DOM
Implementation Guide: Using Activity in Your Project
To start using the Activity component in your project, follow these steps:
- Install React's experimental build in your project
- Import the Activity component with the unstable_ prefix
- Wrap your toggleable components with Activity, using mode="visible" or mode="hidden"
- Test performance in your specific use case
- Monitor React's release notes for updates as this feature evolves
// In your package.json
{
"dependencies": {
"react": "experimental",
"react-dom": "experimental"
}
}
// In your component file
import { unstable_Activity as Activity } from 'react';
function MyToggleableComponent({ isVisible, children }) {
return (
<Activity mode={isVisible ? 'visible' : 'hidden'}>
{children}
</Activity>
);
}
Conclusion: A Significant Step Forward for React Performance
React's experimental Activity component represents a significant advancement in how we handle component visibility in complex applications. By providing a middle ground between full DOM mounting/unmounting and CSS-based visibility, it offers performance-minded developers a powerful new tool for building responsive, efficient user interfaces.
For dashboard applications and data visualization systems in particular, this feature could be a game-changer, dramatically improving both rendering performance and user experience. As React continues to evolve, innovations like the Activity component demonstrate the framework's ongoing commitment to solving real-world performance challenges.
While still experimental, the Activity component is certainly worth exploring for performance-critical applications. Its intelligent approach to background rendering and prioritization of user interactions makes it a valuable addition to React's performance optimization toolkit.
Let's Watch!
React's New Activity Component: A Game-Changer for Dashboard Performance
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence