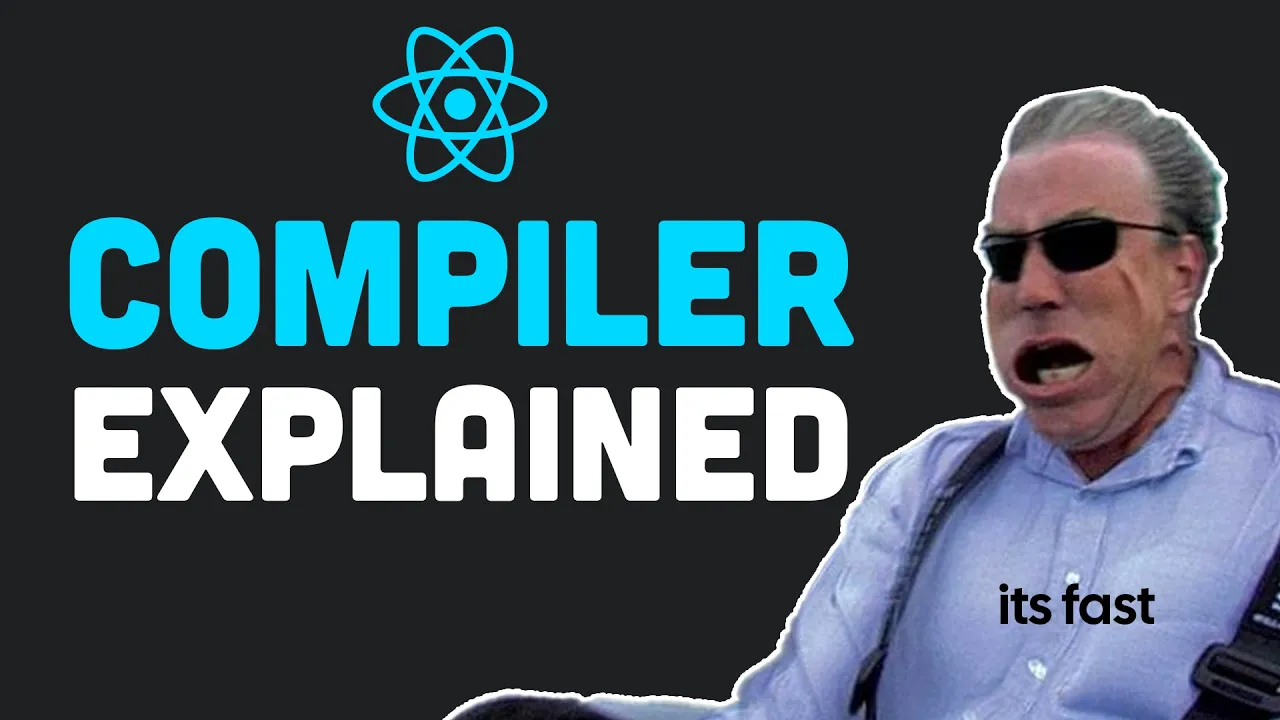
The React team has introduced a game-changing tool called React Compiler that promises to revolutionize how developers handle performance optimizations. This compiler effectively fixes performance issues in your code automatically, potentially making manual optimization techniques like useMemo, useCallback, and React.memo obsolete. Let's explore what React Compiler does, how it works, and why it's such a significant advancement for React developers.
What Does React Compiler Actually Do?
At its core, React Compiler automatically memoizes your code. This means it intelligently tells specific parts of your application not to recalculate or re-render if their inputs haven't changed, significantly reducing unnecessary work during updates. The initial release focuses on two critical use cases:
- Skipping cascading re-renders throughout your component tree
- Preventing expensive calculations outside of React from running repeatedly
These optimizations happen automatically without requiring any code changes from developers, which is what makes React Compiler so revolutionary.
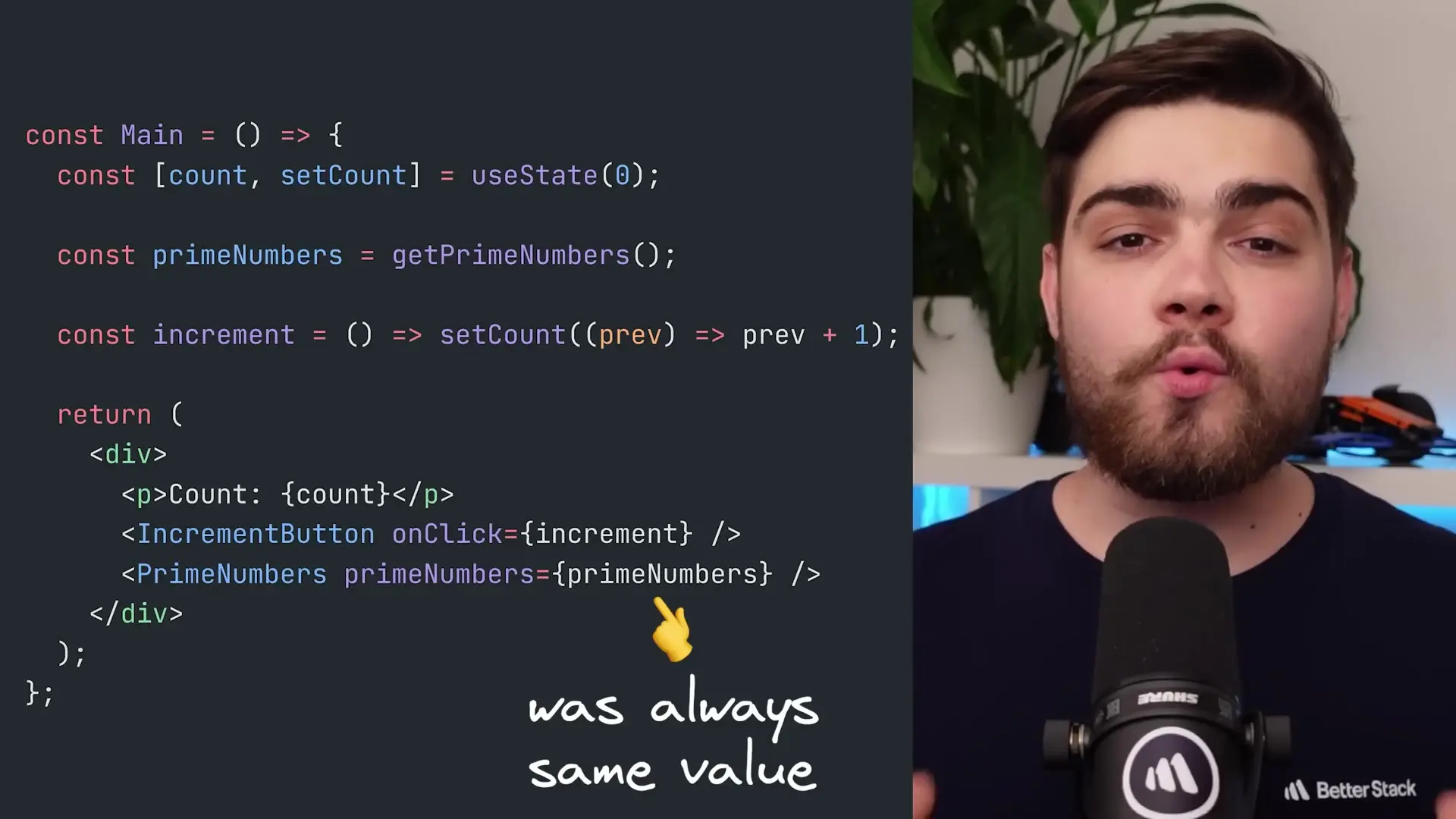
The Problem React Compiler Solves
To understand the value of React Compiler, we need to examine the performance issues it addresses. Consider a typical React application with a parent component containing state and multiple child components. When the parent's state changes, React re-renders not just the parent but all of its children by default—even if those children don't depend on the changed state.
Additionally, expensive calculations (like computing prime numbers) will run on every render, even when their inputs haven't changed. While React is fast enough that this isn't noticeable in simple applications, it becomes problematic as applications grow in complexity or when components perform resource-intensive operations.
Traditional Optimization Methods vs. React Compiler
Before React Compiler, developers had to manually optimize their applications using several techniques:
1. React.memo for Component Memoization
React.memo creates pure components that only re-render when their props change. By wrapping a component in React.memo, you tell React to skip re-rendering that component if its props haven't changed by reference.
const DummyComponent = React.memo(function DummyComponent() {
return <div>Dummy Text</div>;
});
2. useMemo for Value Memoization
useMemo caches the result of expensive calculations between renders. It only recalculates when dependencies in its dependency array change, otherwise returning the cached value.
// Without useMemo - recalculates on every render
const primeNumbers = calculatePrimes(1000);
// With useMemo - only calculates once
const primeNumbers = useMemo(() => calculatePrimes(1000), []);
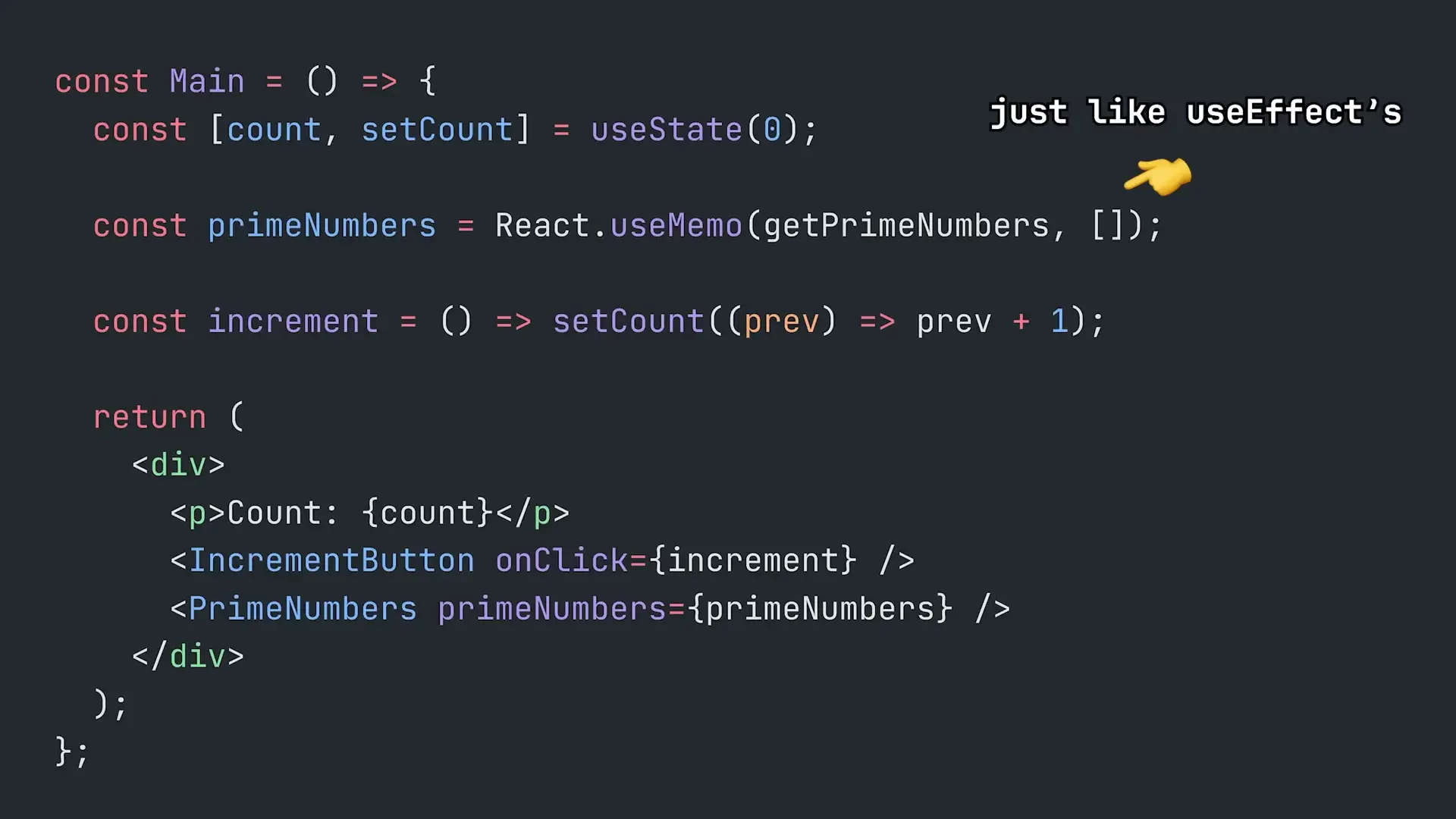
3. useCallback for Function Memoization
useCallback memoizes functions to maintain referential equality between renders. This is particularly important when passing callback functions to memoized child components.
// Without useCallback - creates a new function on every render
const handleIncrement = () => setCounter(counter + 1);
// With useCallback - maintains the same function reference
const handleIncrement = useCallback(() => setCounter(counter + 1), [counter]);
While these techniques work, they have several drawbacks:
- They add complexity and verbosity to your code
- Developers need to know exactly where optimization is needed
- Incorrect implementation can lead to bugs or even worse performance
- They require ongoing maintenance as your application evolves
- The optimization itself has a cost that might not always be worth it
How React Compiler Works Its Magic
React Compiler performs all these optimizations automatically at build time. When you enable the compiler in your project, it analyzes your React components and applies the appropriate optimizations without requiring any code changes. The compiler effectively:
- Identifies components that could benefit from memoization
- Automatically wraps expensive calculations in the equivalent of useMemo
- Prevents unnecessary re-renders by applying React.memo-like behavior
- Maintains function references similar to useCallback
All of this happens behind the scenes, resulting in cleaner, more maintainable code while still achieving optimal performance. The only requirement is that your code follows the standard React rules.
Requirements and Limitations
For React Compiler to work effectively, your code must follow React's rules and best practices. The React team has created an ESLint plugin that helps identify code that violates these rules.
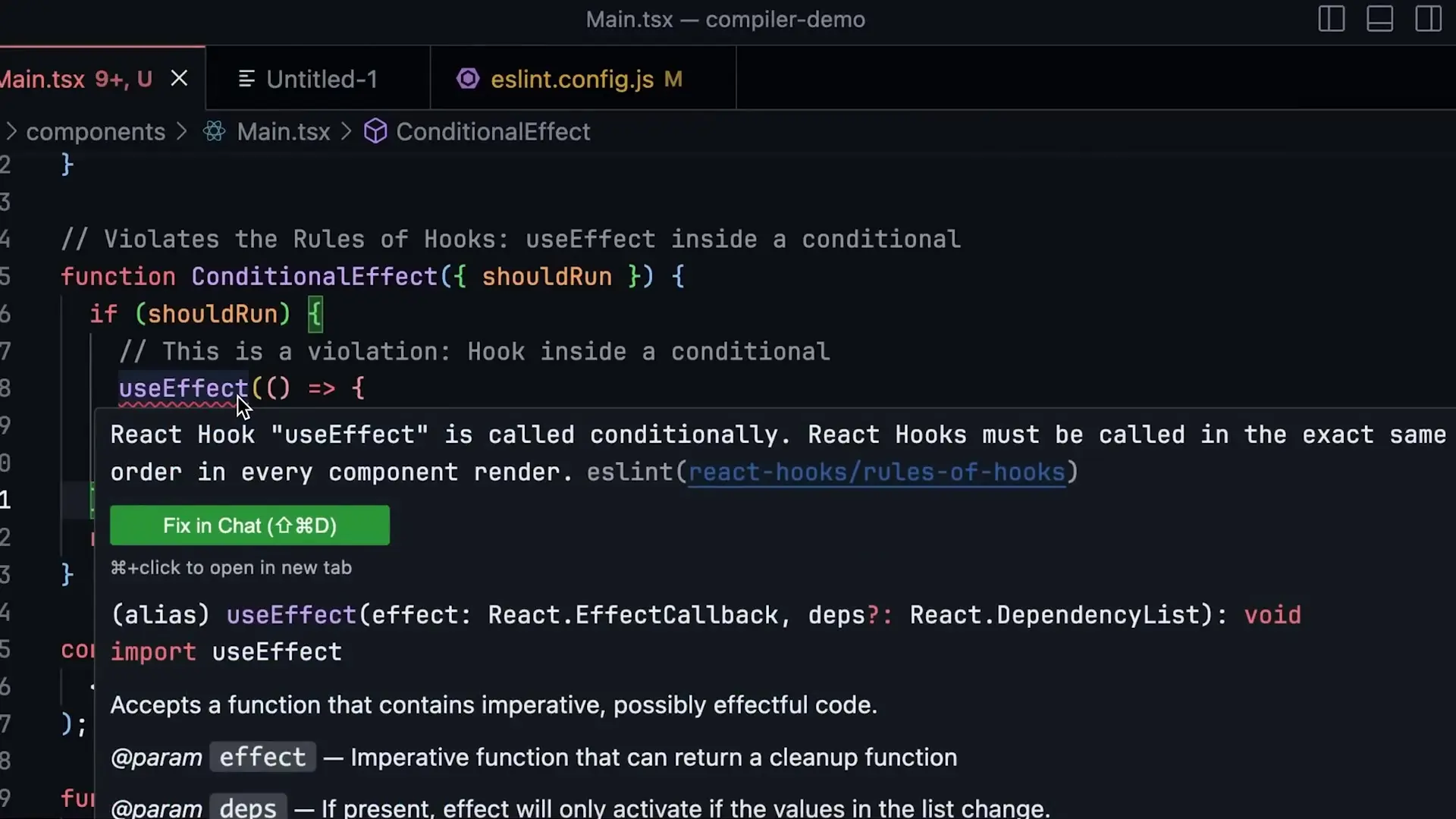
When the compiler encounters code that doesn't follow these rules, it simply skips optimizing that specific component or hook but continues optimizing the rest of your codebase. This graceful degradation ensures that adopting the compiler doesn't break existing code.
React Compiler Integration
React Compiler has reached the release candidate stage and has been successfully used in production at companies like Meta. It's designed to work with various React setups, including:
- Vite
- Next.js
- Expo
- Babel
- Other React-based frameworks and toolchains
The integration process varies depending on your toolchain, but the React team has provided comprehensive documentation to help developers enable the compiler in their projects.
Benefits of Using React Compiler
Adopting React Compiler offers several significant advantages:
- Cleaner, more readable code without manual optimization clutter
- Automatic performance improvements with zero code changes
- Reduced cognitive load—developers can focus on features rather than optimizations
- Consistent optimization across your entire codebase
- Future-proof code as the compiler continues to improve
- Lower maintenance burden as the need for manual optimization decreases
Should You Stop Using useMemo, useCallback, and React.memo?
While React Compiler aims to make these manual optimization hooks less necessary, they won't disappear overnight. There are still valid cases for using them, especially during the transition period as the compiler matures. However, the long-term vision is clear: React is moving toward a future where developers write clean, straightforward code, and the compiler handles optimization concerns.
For new projects, it makes sense to enable the compiler from the start and minimize the use of manual optimization techniques. For existing projects, the compiler can be gradually adopted alongside your current optimization strategies.
Conclusion
React Compiler represents a significant step forward in making React applications faster and easier to maintain. By automatically handling memoization and preventing unnecessary re-renders, it addresses one of the most common performance challenges in React development without adding complexity to your codebase.
As the compiler matures and becomes more widely adopted, we can expect to see a shift in React best practices—moving away from manual optimization techniques toward cleaner, compiler-optimized code. This aligns perfectly with React's philosophy of making it easier to build high-performance user interfaces without sacrificing developer experience.
Whether you're building a new application or maintaining an existing one, React Compiler offers compelling benefits that make it worth considering as part of your development toolchain. The future of React performance optimization is automated, and that future is already here.
Let's Watch!
React Compiler: The Ultimate Solution to Eliminate Re-renders Without Code Changes
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence