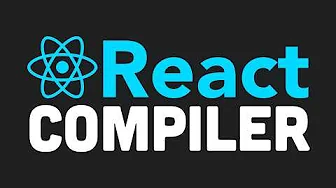
React has introduced a game-changing compiler that promises to revolutionize how developers optimize their applications. This new React memo compiler automatically handles memoization in your codebase, effectively making manual implementations of React.memo, useMemo, and useCallback obsolete. But what exactly is this compiler solving, and how does it work behind the scenes?
Understanding the Problem: Unnecessary Re-renders
At its core, the React memoization compiler addresses one of the most common performance issues in React applications: unnecessary re-renders. When state changes in a parent component, React typically re-renders all child components by default, regardless of whether their props have changed. For simple applications, this isn't problematic, but as your application grows in complexity, these cascading re-renders can significantly impact performance.
Consider a scenario where updating a counter in a parent component causes all child components to re-render, even when those child components display static content or perform expensive calculations that don't depend on the counter value. This is precisely the type of inefficiency the React compiler aims to eliminate.
The React Compiler's Approach to Memoization
The React memo compiler focuses on two key optimization scenarios:
- Skipping cascading re-renders of child components when their props haven't changed
- Preventing expensive calculations from running repeatedly when their inputs remain the same
What makes this compiler remarkable is that it achieves these optimizations automatically, without requiring any changes to your existing code. It analyzes your components and determines where memoization would be beneficial, then applies those optimizations during the build process.
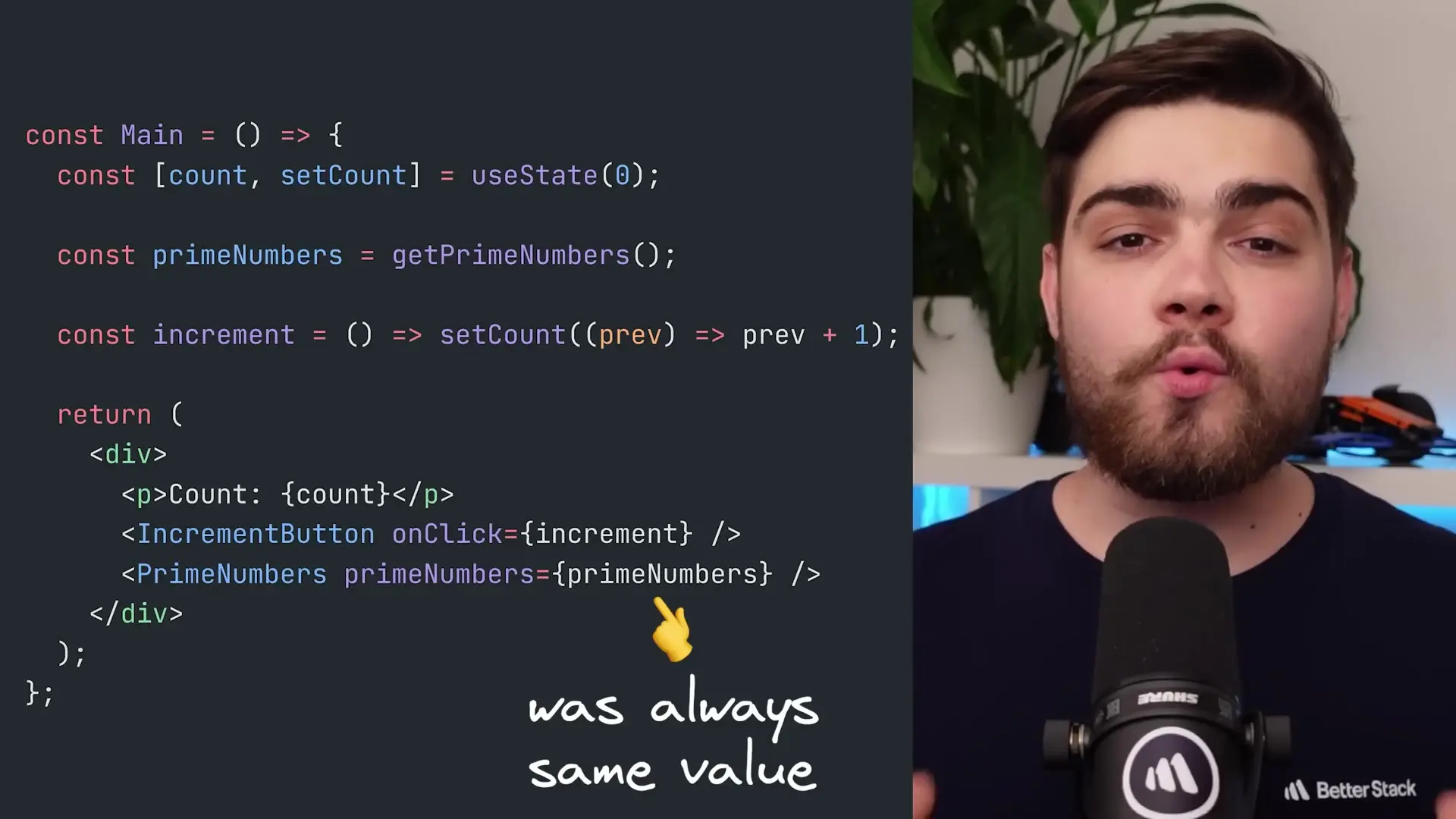
The Traditional Approach: Manual Memoization
Before the React memoization compiler, developers had to manually optimize their components using three primary tools:
- React.memo: A higher-order component that memoizes a component, preventing re-renders unless its props change
- useMemo: A hook that memoizes calculated values, only recalculating when dependencies change
- useCallback: A hook that memoizes function definitions to maintain referential equality between renders
Let's examine how these would be implemented manually in a typical React application:
// Manually memoizing a component
const MemoizedChildComponent = React.memo(ChildComponent);
// Manually memoizing an expensive calculation
const memoizedValue = useMemo(() => {
return calculateExpensivePrimes(1000);
}, []); // Empty dependency array means calculate once
// Manually memoizing a function
const memoizedCallback = useCallback(() => {
setCounter(counter + 1);
}, [counter]); // Only recreate when counter changes
While effective, this approach requires developers to identify optimization opportunities manually, understand the intricacies of reference equality in JavaScript, and make deliberate decisions about when to apply memoization techniques. It also clutters the code with optimization-focused logic that distracts from the core business logic.
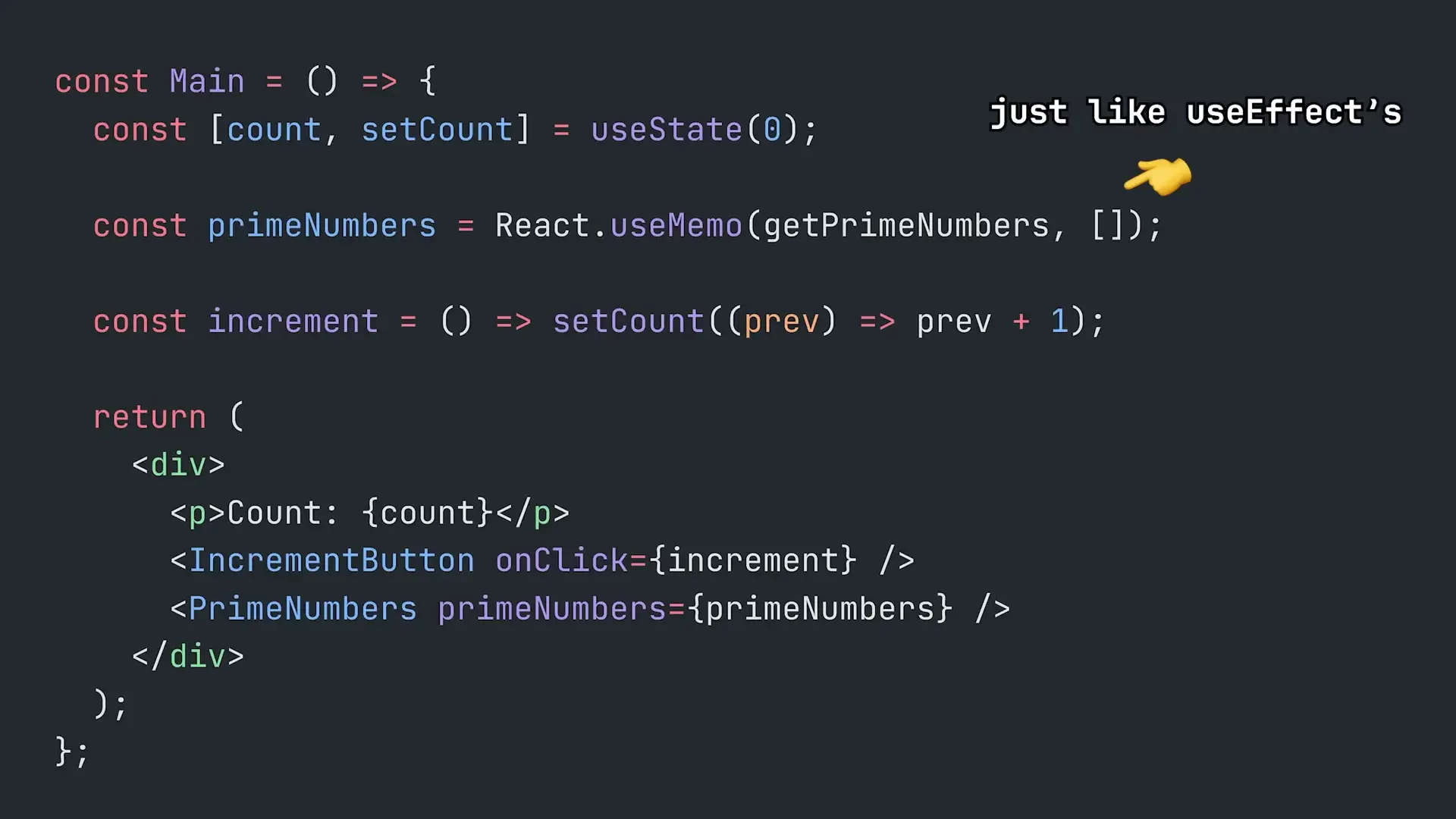
The Pitfalls of Manual Memoization in React
Manual memoization in React comes with several challenges that the React forget compiler aims to address:
- Reference equality issues: JavaScript compares objects and arrays by reference, not value. This means that even if two arrays contain identical values, they're considered different if created separately.
- Development overhead: Identifying which components or calculations to memoize requires careful analysis and testing.
- Maintenance burden: As your application evolves, you need to continually reassess your memoization strategy.
- Optimization trade-offs: Developers must weigh the performance benefits against the added complexity in the codebase.
How to Enable the React Memoization Compiler in Your Project
The React memo compiler has reached release candidate status and is ready for implementation in production environments. It has already been successfully deployed at companies like Meta, demonstrating its reliability for real-world applications.
To enable the React memoization compiler in your project, you'll need to follow the integration steps based on your build system. The compiler supports various frameworks and build tools, including:
- Vite
- Next.js
- Expo
- Babel
- And other common React build environments
The React team has also provided an ESLint plugin that helps identify code patterns that might prevent the compiler from optimizing certain components or hooks. When these patterns are detected, the compiler will simply skip over them while still optimizing the rest of your codebase.
Requirements for Effective Compiler Optimization
For the React memoization compiler to work effectively with your code, there's only one fundamental requirement: your components must follow the standard rules of React. These include:
- Pure rendering functions without side effects
- Proper handling of dependencies in hooks
- Adherence to React's component lifecycle patterns
- Following immutability principles when updating state
If your codebase already follows React best practices, the compiler should work seamlessly without requiring any modifications to your existing code.
Benefits of Using the React Memoization Compiler
Adopting the React memo compiler offers several significant advantages:
- Automatic optimization: The compiler identifies and applies memoization opportunities without manual intervention.
- Cleaner code: Eliminates the need for explicit React.memo, useMemo, and useCallback wrappers.
- Improved performance: Prevents unnecessary re-renders and recalculations throughout your application.
- Reduced cognitive load: Developers can focus on business logic rather than performance optimizations.
- Consistent optimization: The compiler applies optimizations uniformly across your entire codebase.
Real-World Impact: Memoizing Child Components in React
To understand the practical impact of the React memoization compiler, consider a common scenario where a parent component contains multiple child components and performs expensive calculations:
function ParentComponent() {
const [counter, setCounter] = useState(0);
// Expensive calculation that doesn't depend on counter
const primeNumbers = calculatePrimes(10000);
return (
<div>
<h1>Counter: {counter}</h1>
<button onClick={() => setCounter(counter + 1)}>Increment</button>
<ChildComponent data={primeNumbers} />
<AnotherChildComponent text="Static text" />
<YetAnotherChildComponent />
</div>
);
}
Without optimization, each click of the increment button would cause all three child components to re-render and recalculate the prime numbers, even though nothing relevant to those components has changed. With the React memo compiler, only the parent component re-renders to update the counter display, while the child components and expensive calculation remain untouched.
Conclusion: The Future of Memoization in React
The React memoization compiler represents a significant step forward in React's evolution, automating what was previously a manual, error-prone optimization process. By intelligently applying memoization at build time, it delivers the performance benefits of memoized React components without the development overhead.
As this technology matures and becomes the standard approach to React optimization, developers can focus more on creating feature-rich applications and less on performance tuning. The React memo compiler effectively bridges the gap between development efficiency and application performance, making it an essential tool for modern React applications.
While manual memoization techniques will remain in the React API for backward compatibility and edge cases, the compiler's automatic approach will likely become the recommended standard for most React applications going forward.
Let's Watch!
React Compiler: The End of Manual Memoization in Modern React Apps
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence