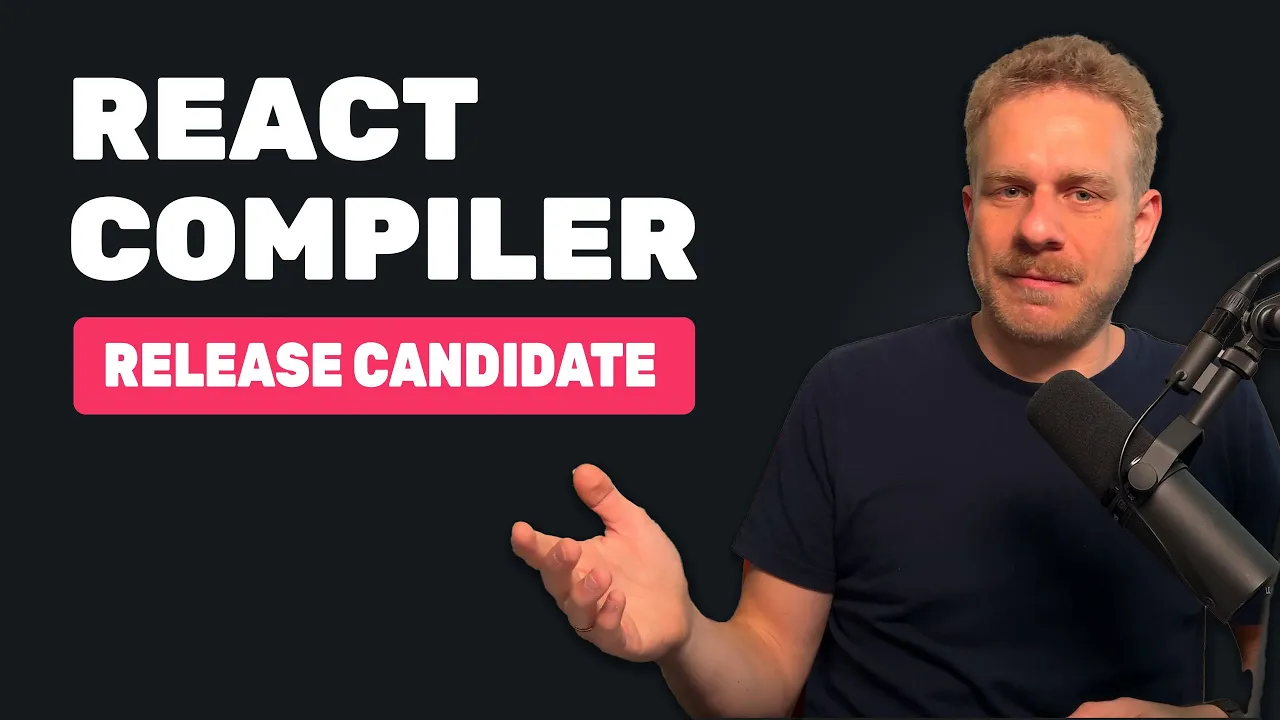
The React compiler has officially entered its release candidate phase, marking a significant milestone in its journey toward stable release. This powerful tool promises to revolutionize how developers optimize their React applications by automatically handling performance optimizations that previously required manual implementation.
While it's not yet officially marked as stable, the React compiler has already proven itself to be remarkably reliable in real-world applications. Based on extensive testing across numerous projects, it performs well in production environments—with one important caveat: your application must adhere to React's established best practices.
What Is the React Compiler?
The React compiler is a tool that analyzes your React code and automatically applies performance optimizations that would otherwise require manual implementation. Its primary function in the current release is to intelligently add `useMemo`, `useCallback`, and `memo` function calls where they would provide performance benefits.
This means developers no longer need to manually identify optimization opportunities and implement these patterns themselves—the compiler handles this analysis and code transformation automatically.
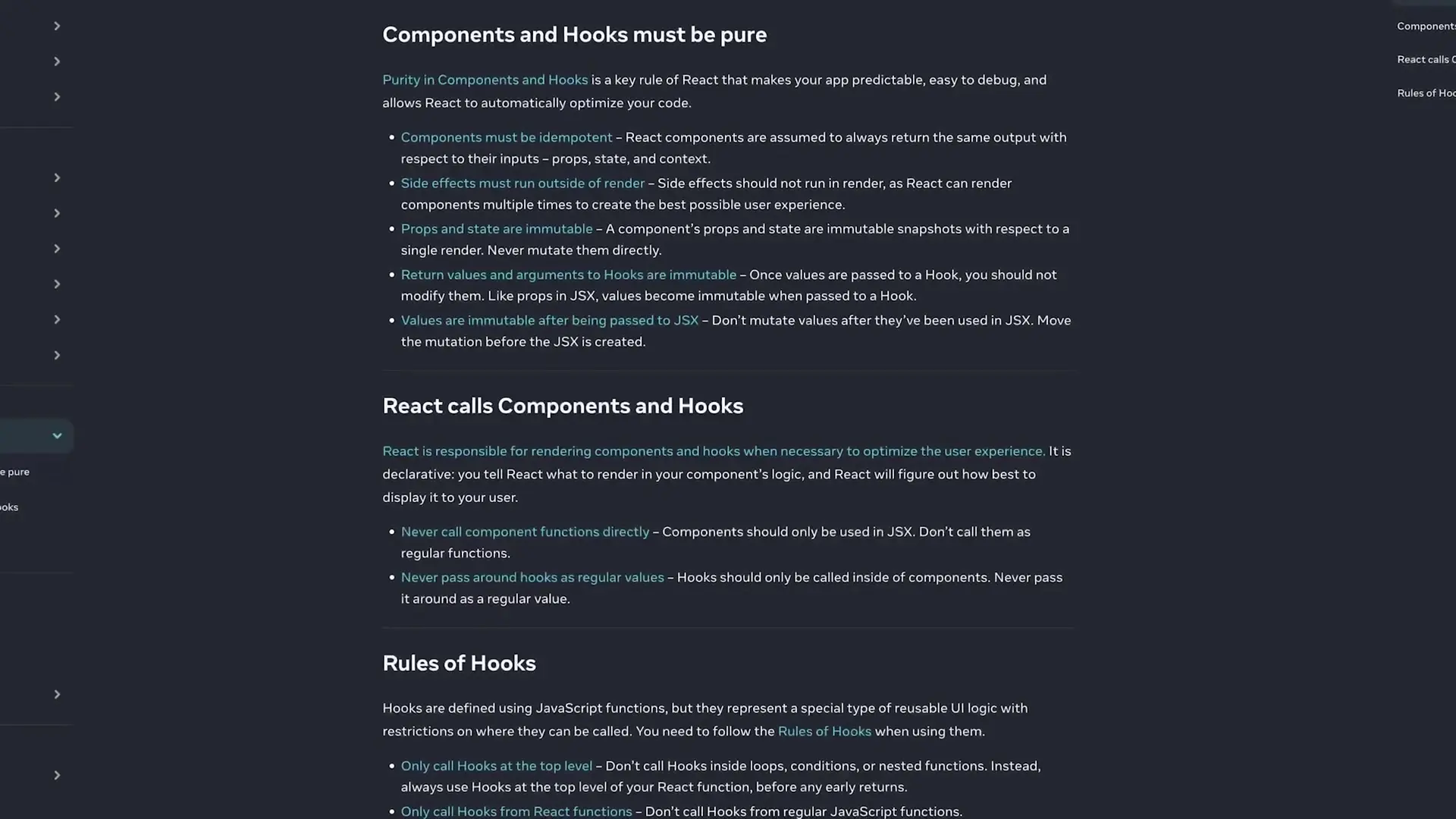
Integrating the React Compiler Into Your Project
Adding the React compiler to your project is straightforward. For Vite projects, you simply need to install an additional dependency and update your Vite configuration to use it. If your project already uses ESLint with the React Hooks plugin, you can simply update to the latest release candidate version of the ESLint React Hooks plugin, which includes the compiler functionality.
# For Vite projects
npm install @vitejs/plugin-react-swc
# Then update your vite.config.js to use the plugin
Understanding the Optimization Techniques
To fully appreciate what the React compiler does, it's important to understand the three main optimization techniques it implements automatically:
1. The memo Function
The `memo` function wraps React components to prevent unnecessary re-renders. When a parent component re-renders, React typically re-renders all its child components regardless of whether their props have changed. By using `memo`, a component will only re-render if its props have actually changed.
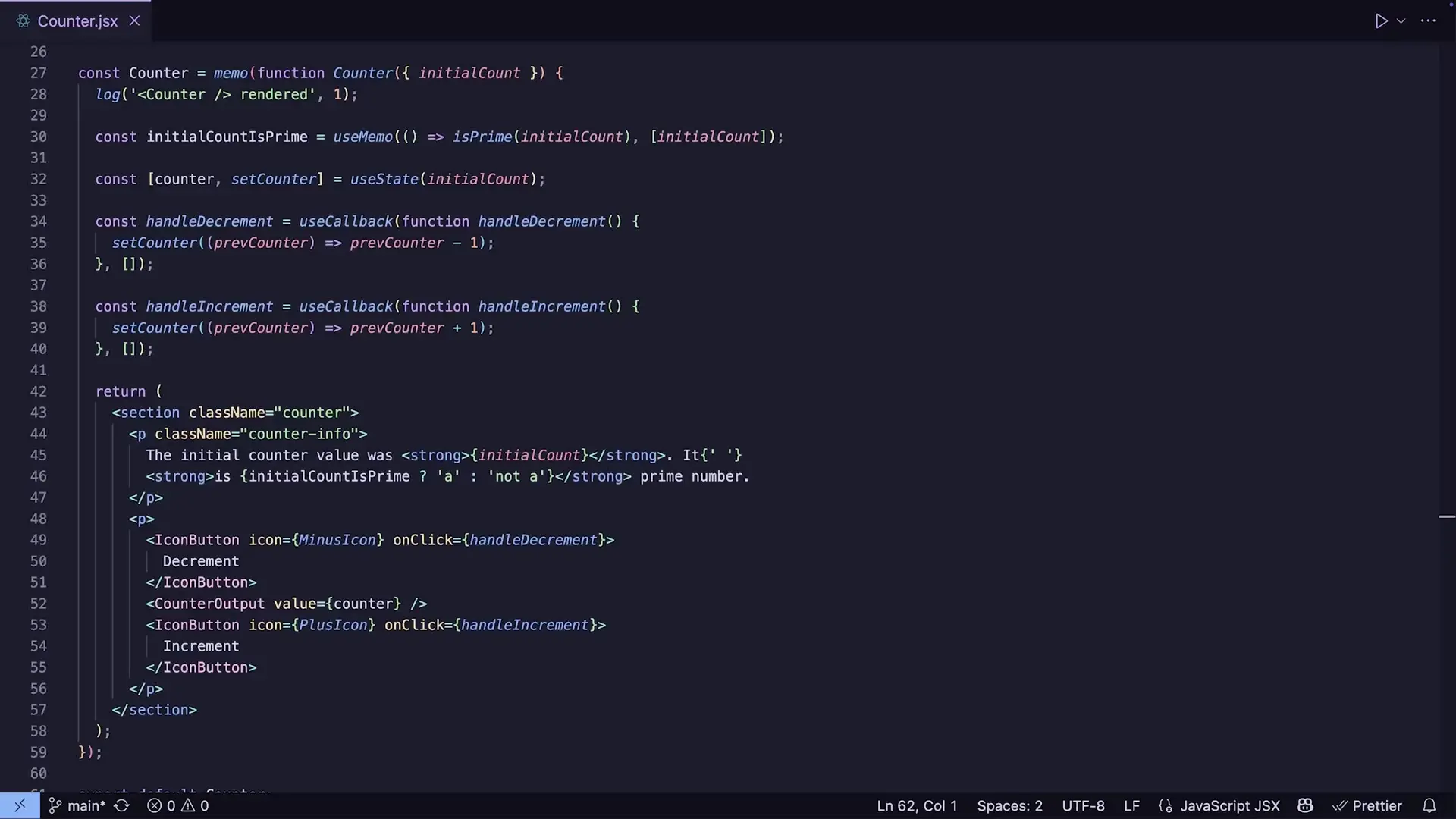
// Without React compiler, you would write:
const OptimizedComponent = React.memo(MyComponent);
// With React compiler, you just write your component normally:
function MyComponent(props) {
// Component code
}
// The compiler adds memo where beneficial
2. The useMemo Hook
The `useMemo` hook prevents expensive calculations from running on every render. It memoizes the result of a computation and only recalculates when specified dependencies change. This is particularly useful for complex calculations that don't need to be repeated if their inputs haven't changed.
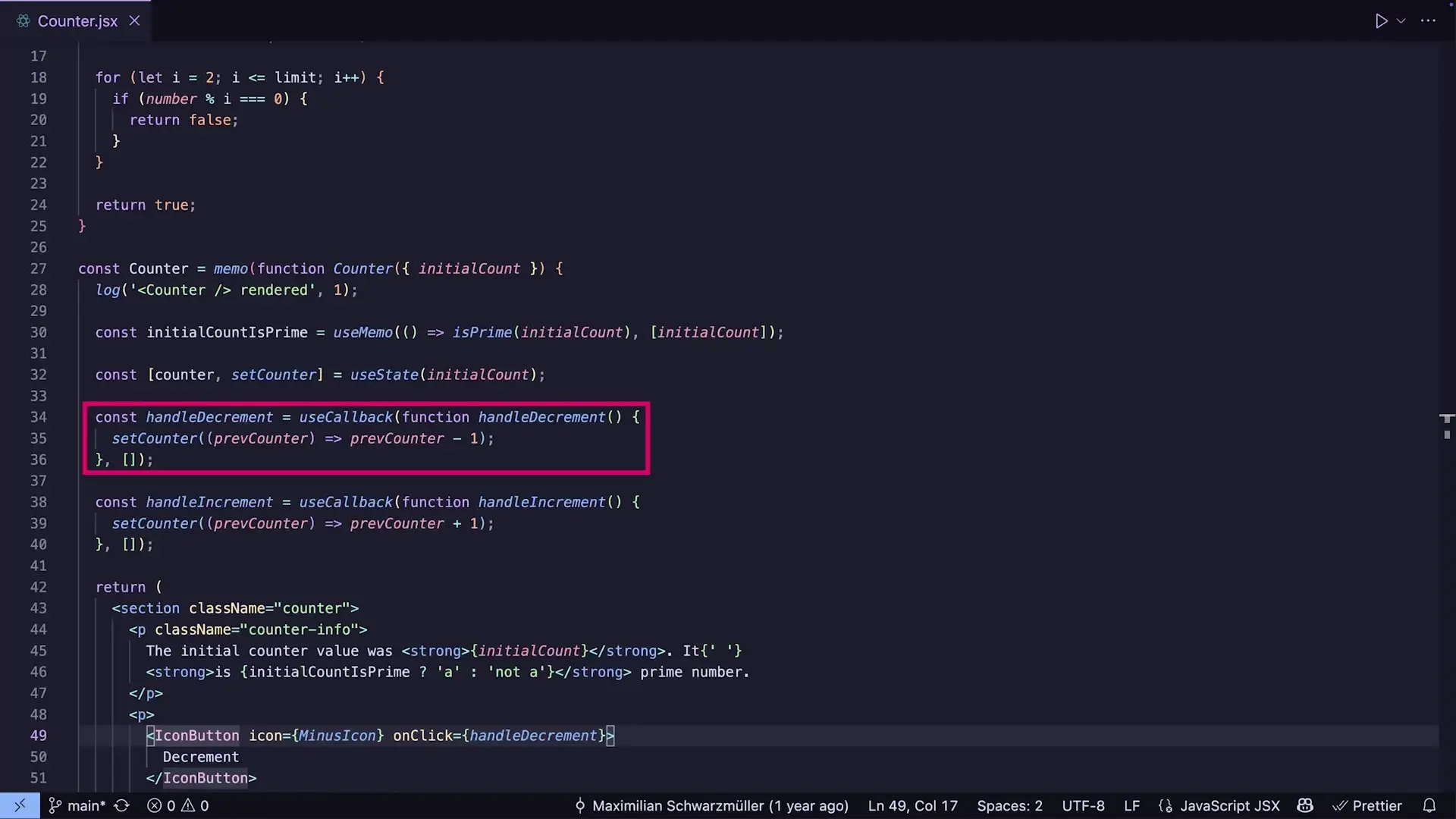
// Without React compiler:
const expensiveResult = React.useMemo(() => {
return computeExpensiveValue(a, b);
}, [a, b]);
// With React compiler:
const expensiveResult = computeExpensiveValue(a, b);
// The compiler adds useMemo with appropriate dependencies
3. The useCallback Hook
The `useCallback` hook memoizes function definitions to prevent unnecessary recreations of functions on each render. This is particularly important when passing functions as props to memoized child components, as a new function reference would cause the child to re-render even if the function's behavior hasn't changed.
// Without React compiler:
const handleClick = React.useCallback(() => {
console.log(count);
}, [count]);
// With React compiler:
const handleClick = () => {
console.log(count);
};
// The compiler adds useCallback with appropriate dependencies
Potential Compatibility Issues
While the React compiler works seamlessly with code that follows React's best practices, it may cause unexpected behavior if your code doesn't adhere to these guidelines. One common issue involves misusing `useEffect` by including dependencies that aren't actually used within the effect function but are relied upon to trigger the effect.
For example, if you have a `useEffect` with dependencies that aren't referenced inside the effect but are used to trigger re-runs, the compiler might optimize this in ways that change your application's behavior. This isn't a flaw in the compiler but rather highlights existing issues in your code that should be addressed.
When to Use the React Compiler
With the React compiler now in release candidate status, there are several scenarios where adopting it makes sense:
- For new React projects, implementing the compiler from the start ensures optimal performance without manual optimization
- For existing projects that follow React best practices, adding the compiler can provide immediate performance benefits
- For teams looking to reduce the cognitive overhead of manual optimizations, allowing developers to focus on feature development
However, for existing projects with non-standard React patterns or heavy reliance on unconventional `useEffect` usage, it's advisable to thoroughly test the application after adding the compiler to ensure no unexpected behavior changes occur.
Benefits of Using the React Compiler
- Automatic performance optimization without manual code changes
- Reduced cognitive load for developers who no longer need to decide when to use memoization
- More consistent application of optimization patterns across your codebase
- Improved performance without the overhead of manually tracking dependencies
- Smaller bundle sizes by avoiding unnecessary manual optimization code
Conclusion
The React compiler represents a significant advancement in React development, automating performance optimizations that previously required manual implementation and careful consideration. By intelligently applying `memo`, `useMemo`, and `useCallback` where they provide the most benefit, the compiler allows developers to write cleaner, more straightforward code while still achieving optimal performance.
With its release candidate status, the React compiler is now ready for serious consideration in production applications. For developers building new React applications or maintaining codebases that follow React best practices, the compiler offers an excellent opportunity to improve performance with minimal effort.
As React continues to evolve, tools like the React compiler demonstrate the framework's commitment to improving developer experience while maintaining high-performance standards. By abstracting away common optimization patterns, React allows developers to focus on what matters most: building great user experiences.
Let's Watch!
React Compiler RC: Automatic Performance Optimization Without Manual Memoization
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence