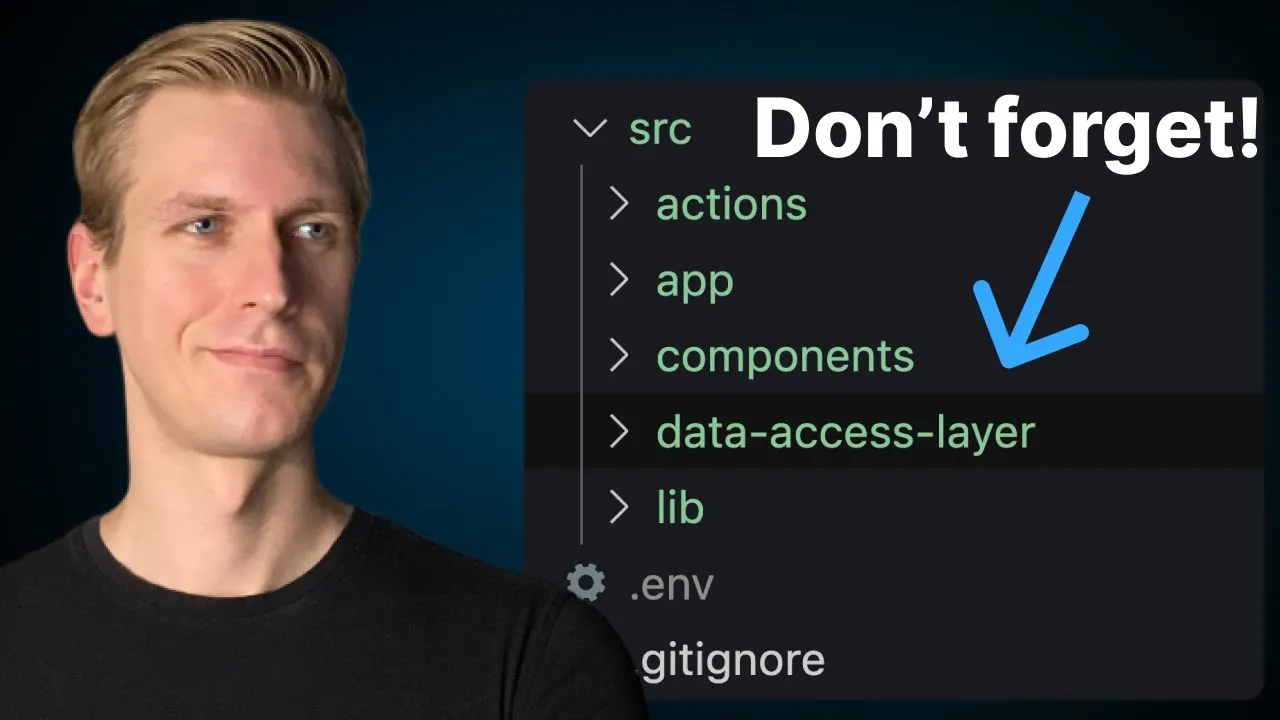
Authentication is a critical aspect of building secure Next.js applications. Recent security vulnerabilities in Next.js middleware have highlighted the importance of implementing robust authentication strategies that go beyond the conventional approaches. This article explores why using a data access layer for authentication provides better security than relying solely on middleware or page components.
The Authentication Challenge in Next.js
Next.js has become a popular framework for building React applications, offering powerful features for server-side rendering, static site generation, and API routes. However, securing these applications properly requires careful consideration, especially when handling sensitive data.
Recently, there have been security concerns with Next.js middleware where attackers could potentially bypass authentication checks. This vulnerability affects applications that rely exclusively on middleware for authentication, exposing sensitive data to unauthorized users.
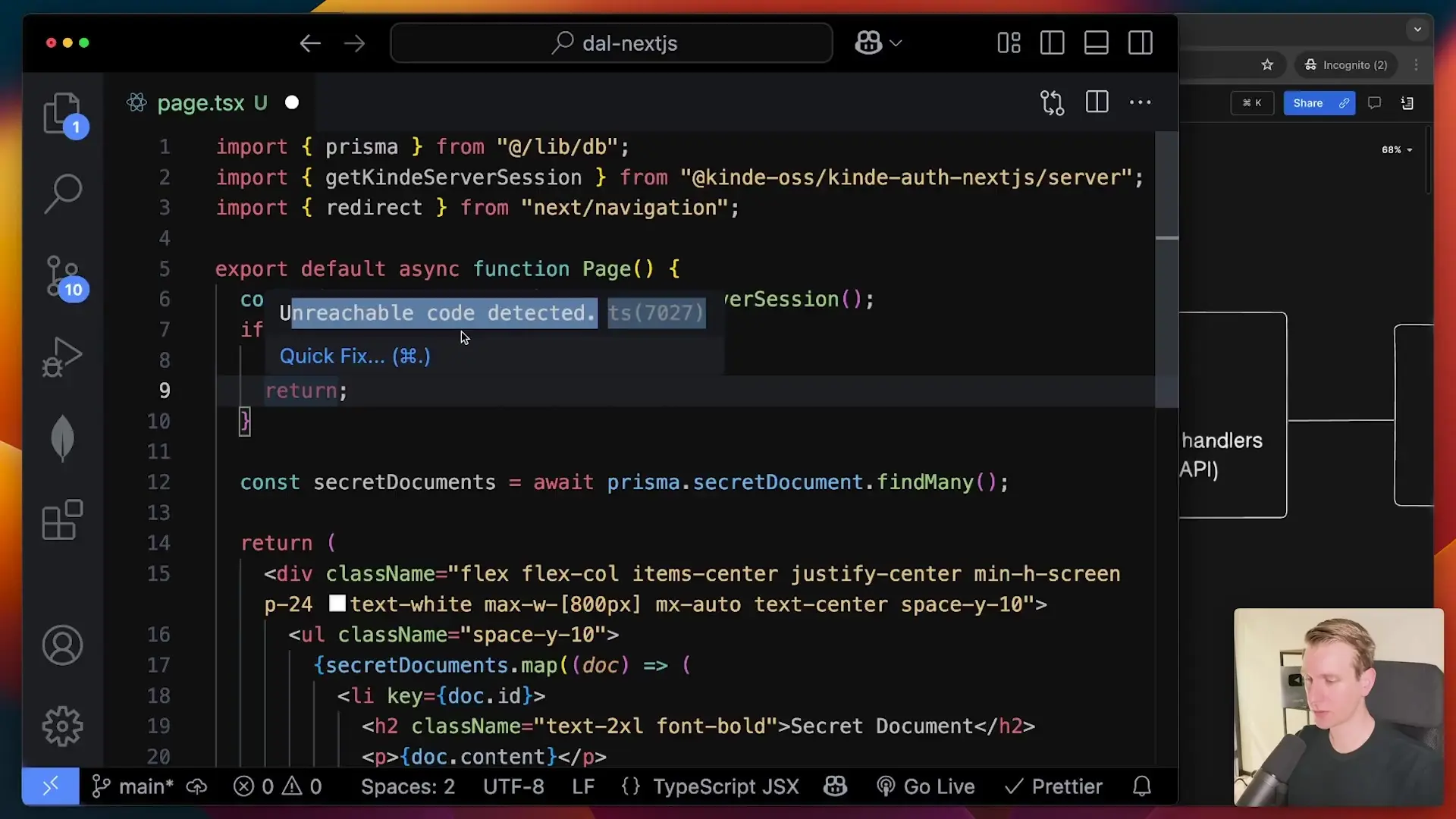
Traditional Authentication Approaches in Next.js
Traditionally, developers have implemented authentication in Next.js applications using one of two approaches:
- Using middleware to check authentication before requests reach page components
- Implementing authentication checks directly within page components
While these approaches work in many scenarios, they have limitations when it comes to building robust, secure applications. Let's look at a practical example to understand why.
Example: Protecting Sensitive Documents
Consider a simple application that stores and displays confidential documents. The most critical aspect of this application is protecting the document data from unauthorized access. If we implement authentication only at the page component level, we might do something like this:
// app/documents/page.js
import { auth } from '@/lib/auth';
import prisma from '@/lib/prisma';
export default async function DocumentsPage() {
// Check if user is authenticated
const session = await auth();
if (!session) {
redirect('/login');
// Code below is unreachable after redirect
}
// Fetch documents from database
const documents = await prisma.document.findMany();
return (
<div>
<h1>Secret Documents</h1>
<ul>
{documents.map((doc) => (
<li key={doc.id}>
<h2>{doc.title}</h2>
<p>{doc.content}</p> {/* Sensitive data */}
</li>
))}
</ul>
</div>
);
}
This approach works initially, but as your application grows and you start refactoring components, you might move data fetching logic into separate components, potentially bypassing the authentication check.
The Problem with Component-Level Authentication
As applications evolve, React developers typically create reusable components to improve code organization. Consider what happens when we refactor our document list into a separate component:
// components/DocumentsList.js
import prisma from '@/lib/prisma';
export default async function DocumentsList() {
// Data fetching moved to this component
const documents = await prisma.document.findMany();
return (
<ul>
{documents.map((doc) => (
<li key={doc.id}>
<h2>{doc.title}</h2>
<p>{doc.content}</p> {/* Sensitive data */}
</li>
))}
</ul>
);
}
Now, if this component is used somewhere else in the application where authentication isn't checked, you've inadvertently created a security vulnerability. The same issue can occur with middleware-only authentication if the middleware can be bypassed.
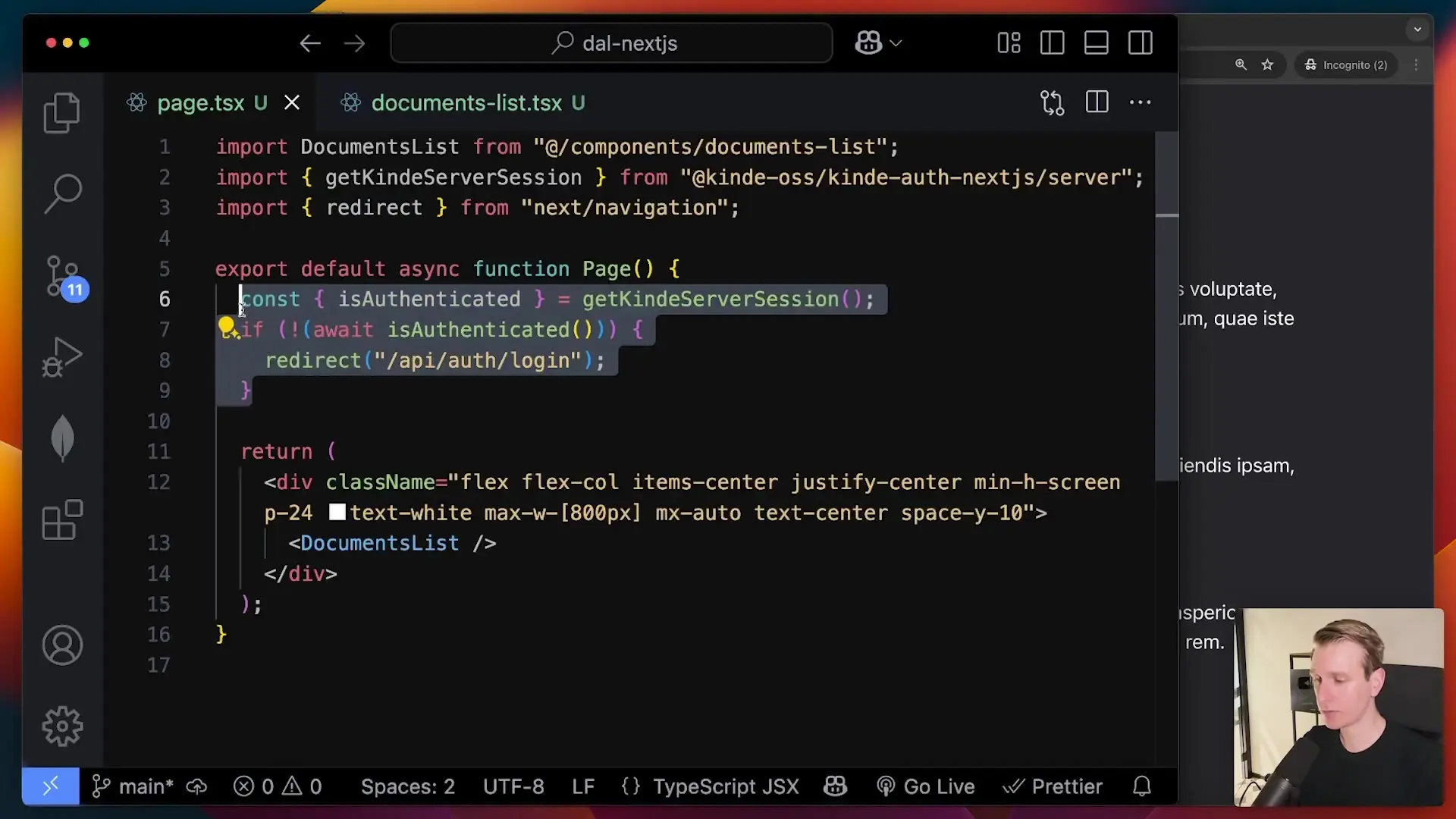
The Data Access Layer Solution
A more robust approach is to implement a data access layer (DAL) that centralizes all data access and enforces authentication at this level. The Next.js team recommends this approach for building secure applications.
A data access layer is essentially a set of functions or classes that handle all interactions with your data sources (databases, APIs, etc.) and enforce security rules consistently. By implementing authentication checks at this level, you ensure that sensitive data can only be accessed by authorized users, regardless of where in your application the data is requested.
Implementing a Data Access Layer
Here's how you might implement a data access layer for our document example:
// lib/data-access.js
import { auth } from '@/lib/auth';
import prisma from '@/lib/prisma';
export async function getDocuments() {
// Authentication check happens here, in the data access layer
const session = await auth();
if (!session) {
// Either throw an error or return null/empty array
throw new Error('Unauthorized access');
}
// Only proceed to fetch data if user is authenticated
return prisma.document.findMany();
}
// Add other data access functions with similar authentication checks
Now, update your components to use this data access layer instead of accessing the database directly:
// components/DocumentsList.js
import { getDocuments } from '@/lib/data-access';
export default async function DocumentsList() {
// Using the data access layer function
// This will throw an error if user is not authenticated
const documents = await getDocuments();
return (
<ul>
{documents.map((doc) => (
<li key={doc.id}>
<h2>{doc.title}</h2>
<p>{doc.content}</p>
</li>
))}
</ul>
);
}
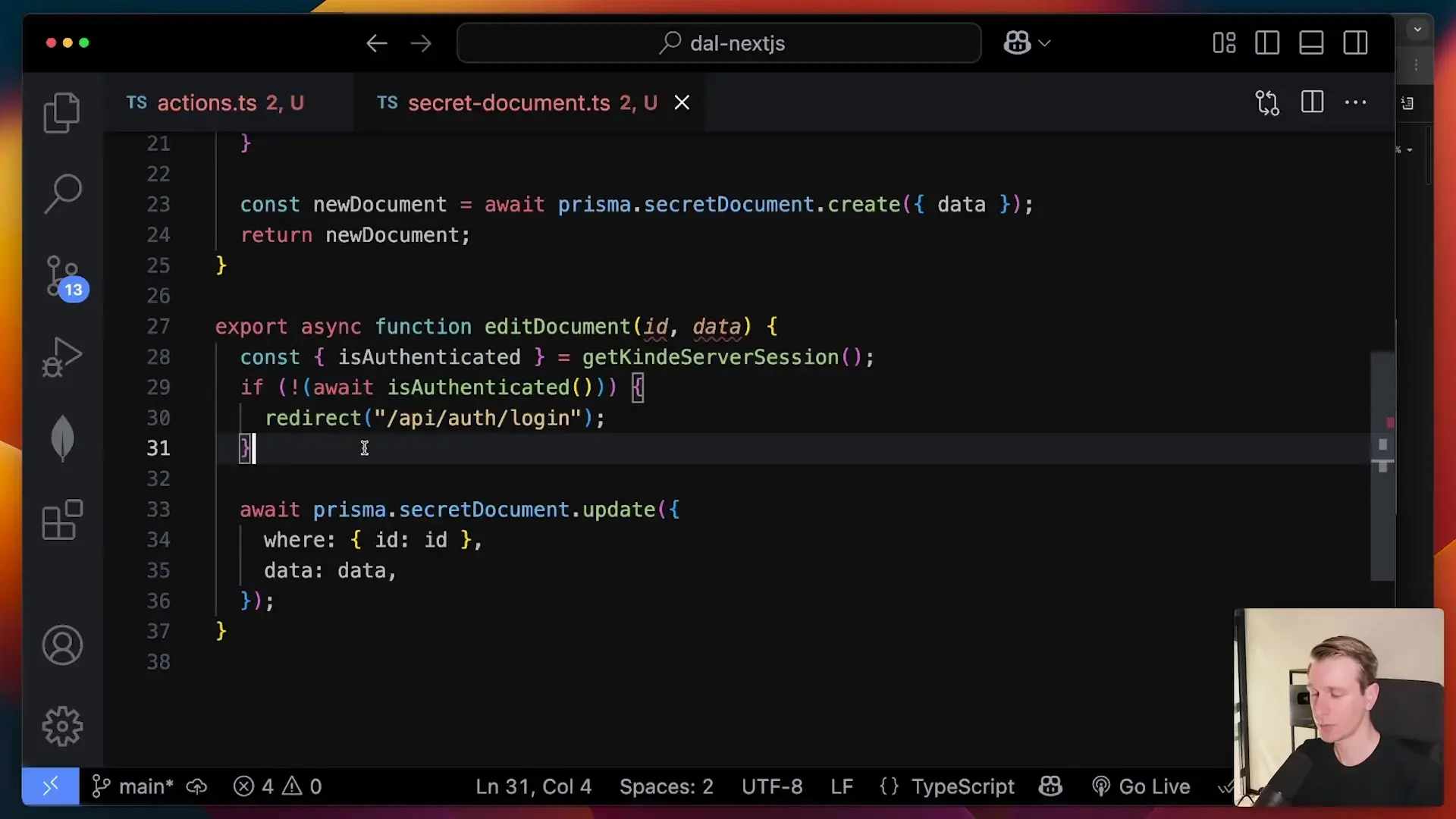
Best Practices for Authentication in Next.js
To build secure Next.js applications, consider implementing these best practices:
- Use a multi-layered approach to authentication (defense in depth)
- Implement authentication checks in middleware as a first line of defense
- Add authentication checks in page components for UI-level protection
- Create a data access layer that enforces authentication for all data operations
- Use established authentication libraries like NextAuth.js, Clerk, or Auth0
- Store authentication tokens securely in HTTP-only cookies
- Implement proper CSRF protection
- Regularly update your Next.js version to get security patches
Working with Cookies in Next.js Authentication
Many authentication solutions for Next.js use cookies to store authentication tokens. The Next.js cookies API provides a convenient way to work with cookies in both client and server components.
// Setting a cookie in a server action
import { cookies } from 'next/headers';
async function login(formData) {
// Authenticate user and get token
const token = await authenticateUser(formData);
// Set the token in a secure, HTTP-only cookie
cookies().set({
name: 'auth-token',
value: token,
httpOnly: true,
secure: process.env.NODE_ENV === 'production',
sameSite: 'strict',
maxAge: 60 * 60 * 24 * 7 // 1 week
});
redirect('/dashboard');
}
Using HTTP-only cookies helps protect against XSS attacks since client-side JavaScript cannot access the cookie content. This is particularly important for storing sensitive authentication tokens.
Progressive Web App (PWA) Considerations
If you're building a Next.js PWA, you need to consider how authentication works in offline scenarios. The Next.js PWA capabilities allow your application to function offline, but this introduces additional authentication challenges.
For PWAs, consider implementing token refresh mechanisms and clear session timeout policies. You might also need to handle authentication state synchronization when the application comes back online after being offline.
Documentation and Resources
The Next.js docs provide excellent guidance on implementing authentication. Additionally, many authentication providers offer specific documentation for integrating with Next.js:
- Next.js Authentication Documentation
- NextAuth.js Documentation
- Clerk Documentation for Next.js
- Auth0 Next.js SDK Documentation
The Next.js GitHub repository is also a valuable resource for examining how authentication is implemented in example applications and for staying updated on security-related issues and fixes.
Conclusion
Building secure authentication in Next.js applications requires more than just middleware or component-level checks. By implementing a data access layer that enforces authentication for all data operations, you create a robust security system that protects your sensitive data regardless of how your application evolves.
Remember that security is not a one-time implementation but an ongoing process. Stay updated with the latest security best practices, regularly check the Next.js docs and GitHub repository for security updates, and continuously review and improve your authentication implementation.
By following these best practices and implementing a proper data access layer, you can build Next.js applications that are not only feature-rich and performant but also secure and trustworthy.
Let's Watch!
Building Secure Next.js Apps: Why Data Access Layers Beat Middleware for Authentication
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence