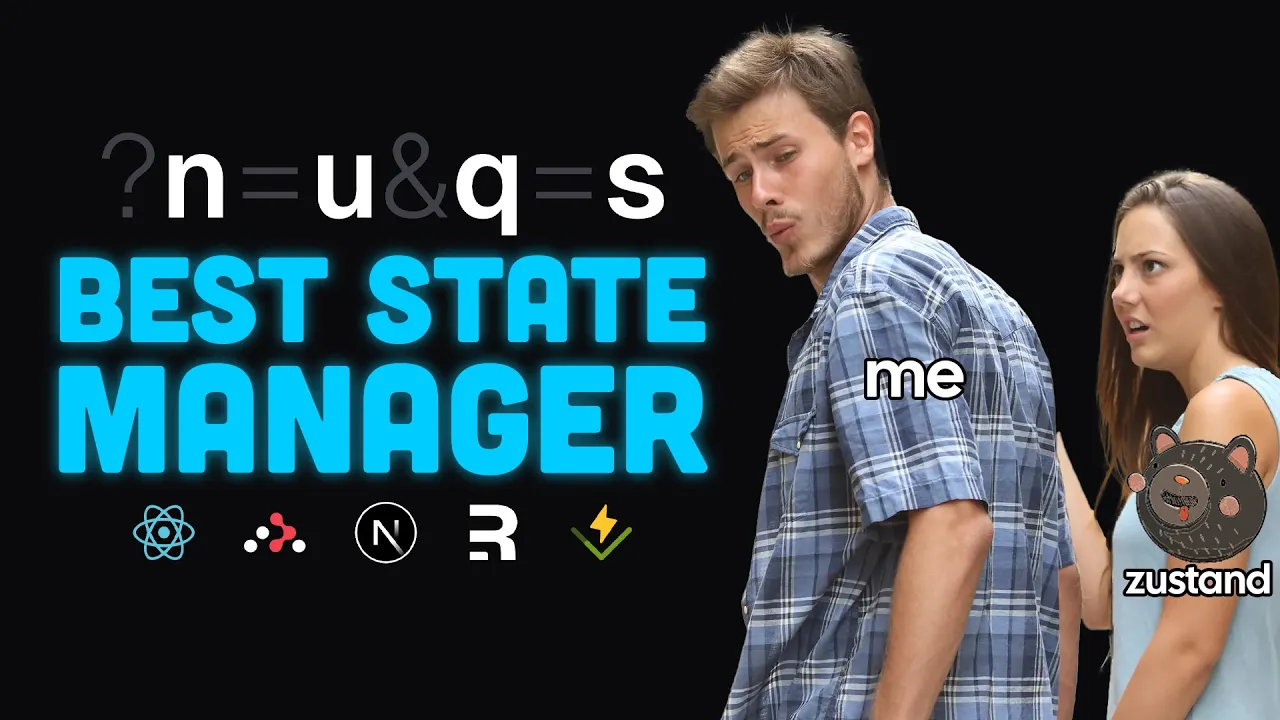
When it comes to React state management, developers often reach for complex libraries like Redux, Zustand, or Recoil. But what if the best solution has been hiding in plain sight since the dawn of the web? URL query parameters might just be the most underrated state management solution in the React ecosystem, offering built-in persistence, shareability, and navigation support without additional complexity.
The Problem with Traditional React State
Consider this common scenario: you're building a product page with selectable options like size, color, and quantity. Using React's useState hook, everything works perfectly until the user refreshes the page. Suddenly, all their selections disappear, reverting to default values.
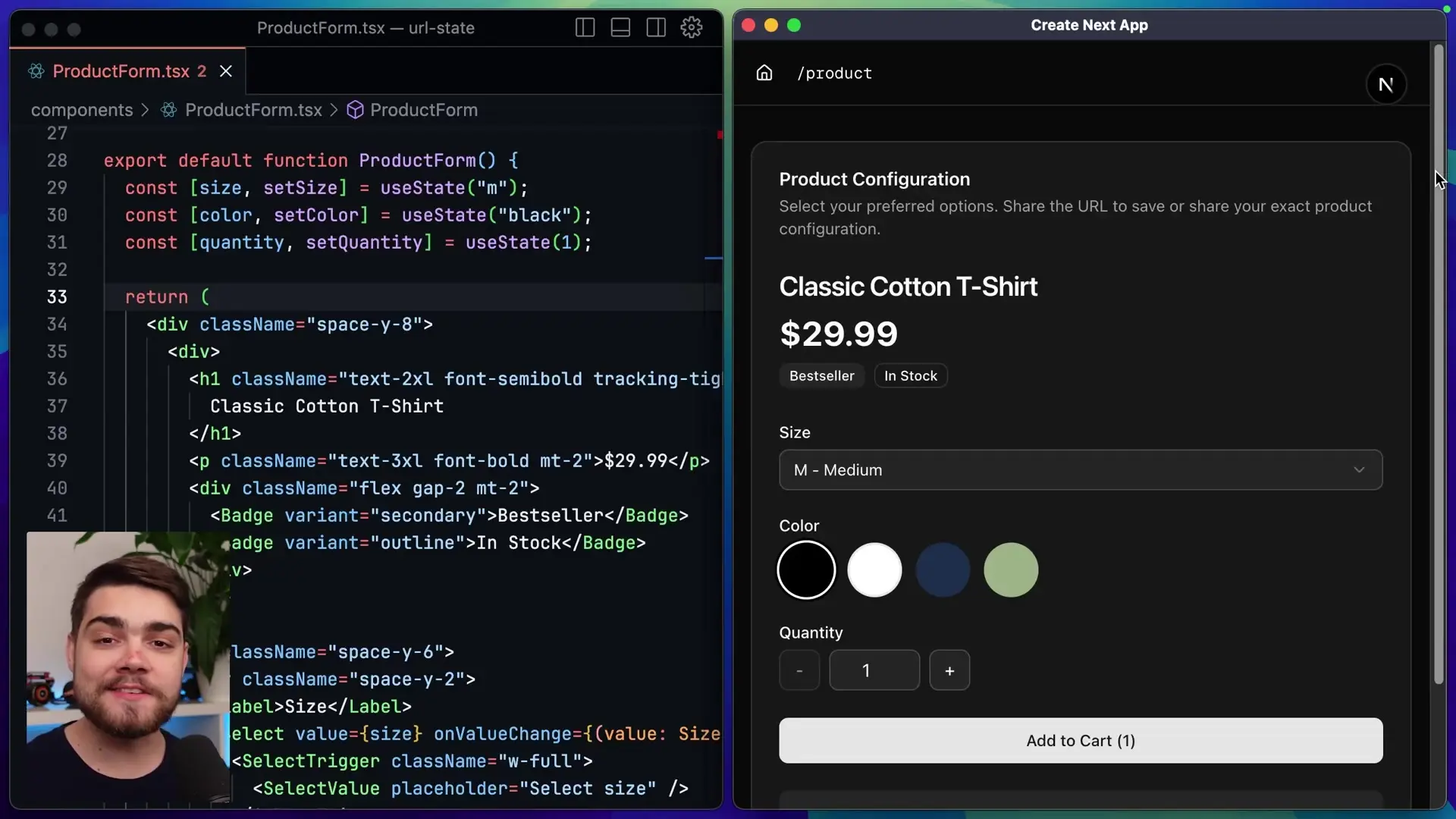
This creates a frustrating user experience. If a user carefully selects options, then refreshes the page, bookmarks the URL, or shares the link with a friend, all their selections are lost. The fundamental issue is that useState stores information in memory, which is wiped clean on page reload.
Enter URL Query Parameters as State
URL query parameters solve this problem elegantly. By storing state information directly in the URL (like example.com/product?size=large&color=blue&quantity=2), you get several benefits automatically:
- Persistence across page refreshes
- Shareable URLs that preserve state
- Browser history navigation support
- Bookmarkable states
- SEO benefits from descriptive URLs
Think about it - popular websites like YouTube, Amazon, and Google Search all use query parameters to maintain state. When you search for something on Google, that search term becomes part of the URL, making it easy to share or revisit later.
Introducing Nux: Type-Safe URL State Management
While the concept of URL-based state is powerful, implementing it correctly can be challenging. Parsing string values, handling type safety, and managing browser history require careful consideration. This is where Nux comes in - a specialized library that makes URL state management in React seamless and type-safe.
Nux provides a useQueryState hook that works nearly identically to React's useState, making adoption straightforward. The library supports most React frameworks including Next.js, React Router, Remix, and vanilla React.
// Traditional React state
const [size, setSize] = useState('medium');
// Nux query parameter state
const [size, setSize] = useQueryState('size', 'medium');
Setting Up Nux in Your React Project
Getting started with Nux is straightforward. First, install the package via npm, yarn, or pnpm:
npm install nux
Then, set up the appropriate adapter for your framework. For example, with Next.js App Router, you would:
// app/layout.tsx
import { NuxNextAppRouterAdapter } from 'nux/adapters/next-app-router';
export default function RootLayout({ children }) {
return (
<html>
<body>
<NuxNextAppRouterAdapter>
{children}
</NuxNextAppRouterAdapter>
</body>
</html>
);
}
Once set up, you can start using the useQueryState hook throughout your application.
Type-Safe Parsing with Nux
One of the challenges with URL parameters is that they're always strings, but your application often needs other data types like numbers, booleans, or arrays. Nux solves this with built-in parsers.
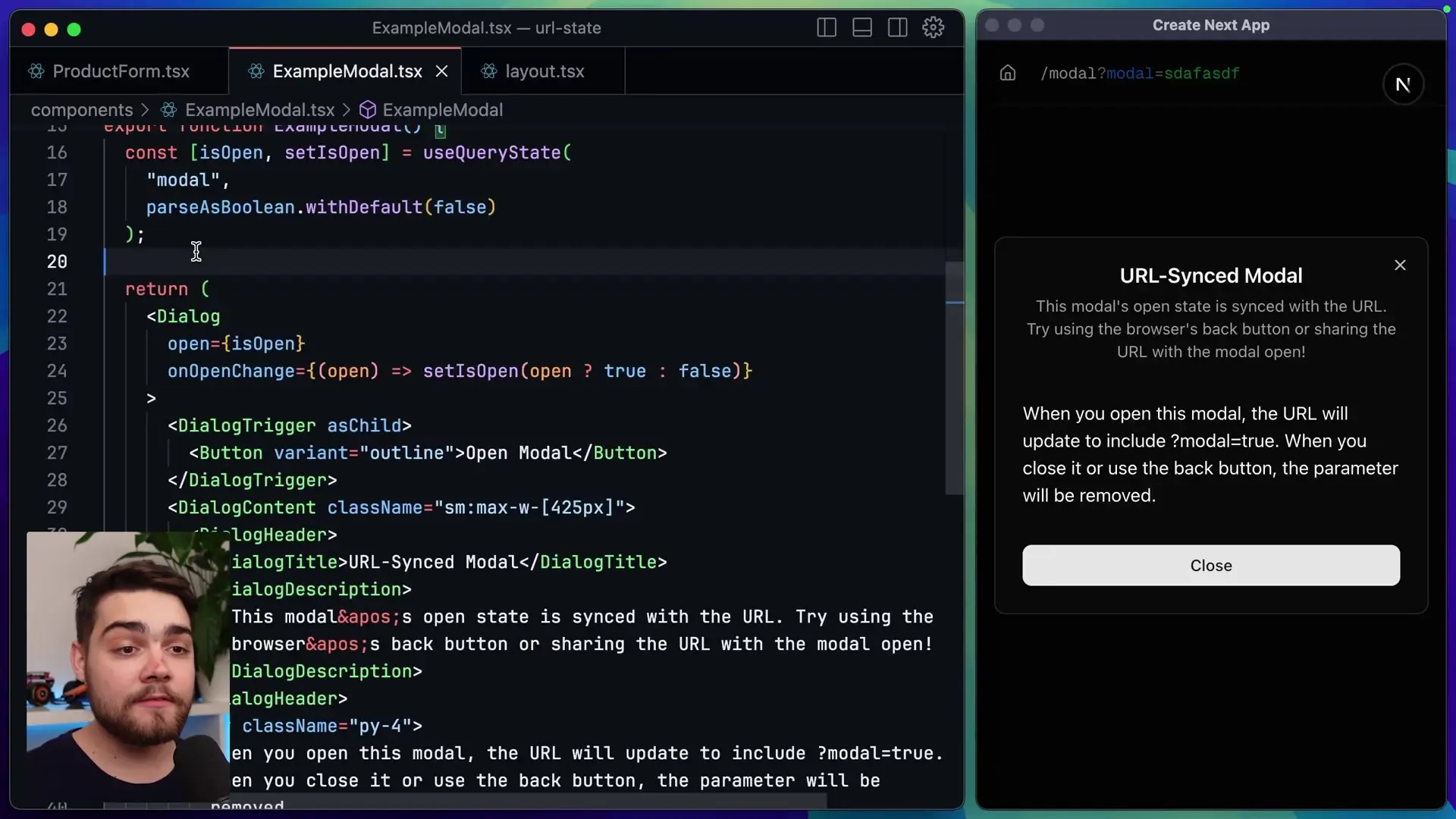
For example, to handle a boolean parameter for a modal's open state:
import { useQueryState } from 'nux';
import { parseAsBoolean } from 'nux/parsers';
function Modal() {
// Parse the 'modal' parameter as a boolean with a default of false
const [isOpen, setIsOpen] = useQueryState(
'modal',
parseAsBoolean.withDefault(false)
);
return (
<>
<button onClick={() => setIsOpen(true)}>Open Modal</button>
{isOpen && (
<div className="modal">
<button onClick={() => setIsOpen(false)}>Close</button>
<h2>Modal Content</h2>
</div>
)}
</>
);
}
Nux supports a variety of parsers out of the box:
- parseAsString - For text values
- parseAsInteger - For whole numbers
- parseAsFloat - For decimal numbers
- parseAsBoolean - For true/false values
- parseAsIsoDateTime - For date/time values
- parseAsJson - For complex objects
- parseAsArrayOf - For arrays of values
Advanced Features: Throttling and History Management
Nux goes beyond basic parameter handling to solve edge cases that would be difficult to implement manually.
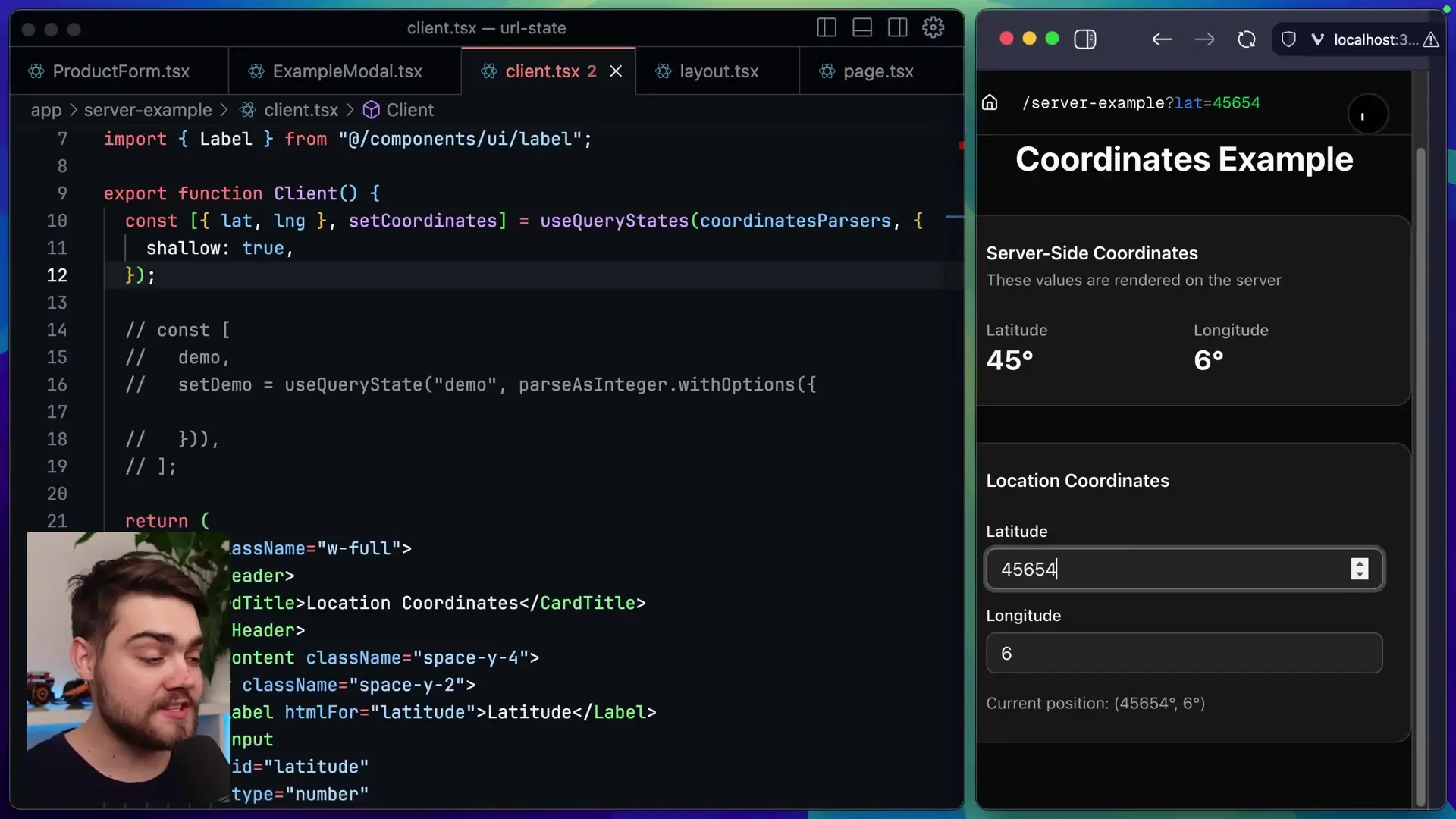
Throttling Updates
Browser APIs have rate limits for history updates, which can cause issues with fast-changing values like input fields. Nux automatically handles this with throttling, ensuring the UI remains responsive while managing URL updates efficiently.
For search inputs or other cases where you want to reduce server requests, you can customize the throttle time:
const [searchTerm, setSearchTerm] = useQueryState(
'q',
parseAsString.withOptions({
throttleMs: 500 // Only update URL after 500ms of inactivity
})
);
History Mode Control
Nux lets you control how URL changes affect browser history with the history option:
- replace (default): Updates the current history entry, ideal for filters and form inputs
- push: Creates a new history entry, better for navigation steps like pagination
const [page, setPage] = useQueryState(
'page',
parseAsInteger.withOptions({
defaultValue: 1,
history: 'push' // Creates new history entries for back/forward navigation
})
);
Real-World Use Cases for URL State Management
URL-based state management shines in numerous scenarios:
- Product filters and sorting options on e-commerce sites
- Pagination controls for data tables or search results
- Form wizard steps that users might need to bookmark
- Modal or dialog states that should persist on refresh
- Search parameters and filter selections
- Map view coordinates and zoom levels
- Dashboard configuration settings
- Multi-step processes where users might need to return later
Using URL State with Server Components
For frameworks like Next.js that support server components, URL parameters offer a seamless way to pass state to the server without additional API calls. The server can read the same parameters directly from the request URL, enabling efficient server-side rendering based on the current state.
// In a Next.js server component
export default function ProductGrid({ searchParams }) {
const size = searchParams.size || 'medium';
const color = searchParams.color || 'black';
// Use these values for server-side data fetching
const products = fetchProductsByFilters({ size, color });
return (
<div className="product-grid">
{products.map(product => (
<ProductCard key={product.id} product={product} />
))}
</div>
);
}
SEO Benefits of URL State
Beyond the user experience advantages, URL-based state management offers significant SEO benefits. Search engines can index different states of your application, making filtered or specific views discoverable through search. This is particularly valuable for e-commerce sites, where category pages with specific filters can rank for long-tail keywords.
Conclusion: The Old Solution to a Modern Problem
URL query parameters represent a time-tested approach to state management that predates React by decades. With libraries like Nux, we can leverage this proven pattern with modern type safety and developer experience. For many React applications, especially those with shareable states, this approach offers the perfect balance of simplicity, functionality, and user experience.
Next time you reach for a complex state management library, consider whether URL parameters might be the simpler, more user-friendly solution. After all, they've been successfully managing state on the web for over 30 years - sometimes the best solutions are hiding in plain sight.
Let's Watch!
Why URL Query Parameters Are the Ultimate React State Manager You're Overlooking
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence