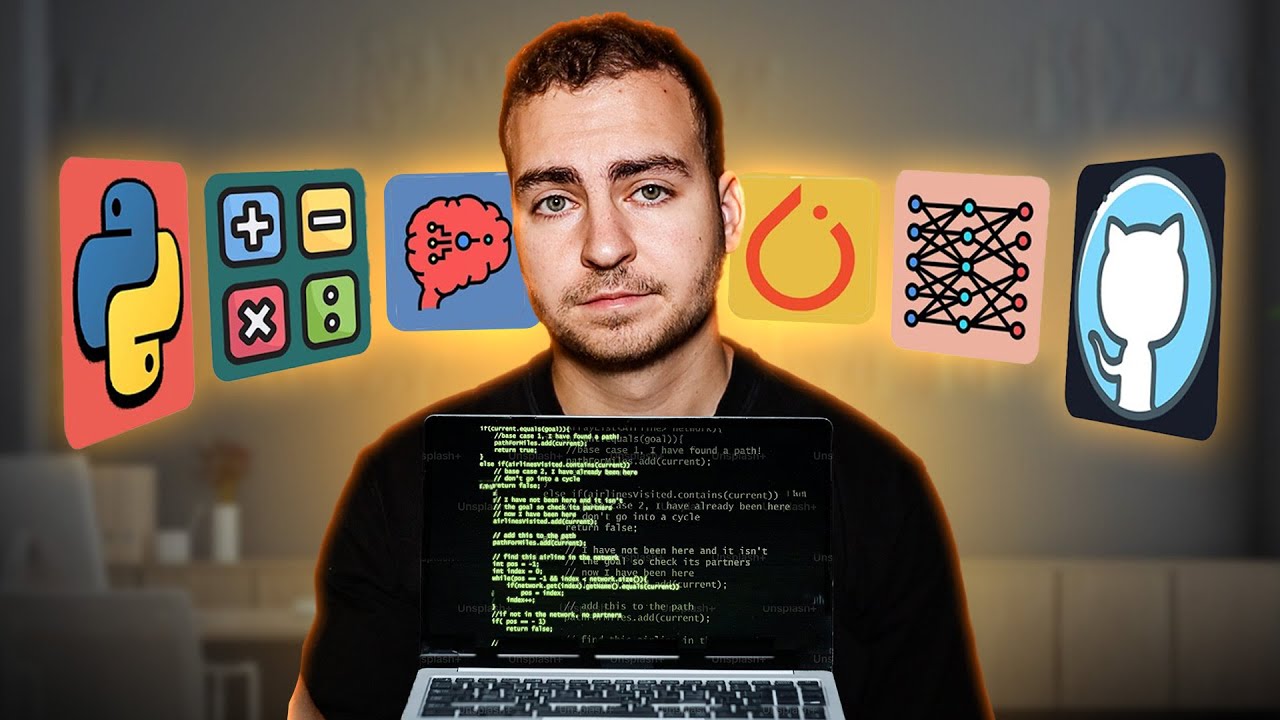
Artificial intelligence and machine learning are evolving at breakneck speed, making it challenging to know where to begin. If you're looking to fast-track your AI and ML learning journey in 2025, you need a structured approach that balances theory with practical application. This guide outlines exactly how to efficiently master these technologies with a focus on building real skills rather than just theoretical knowledge.
Step 0: Develop an Engineer's Mindset
Before diving into specific technologies, it's crucial to cultivate an engineer's mindset. What separates good software engineers from great ones isn't memorization of concepts but the ability to break down complex problems and think critically. Throughout your learning journey, focus on understanding the underlying principles rather than just following tutorials. This critical thinking skill is precisely where humans add value that AI currently cannot replicate.
Approach each concept with curiosity and ask questions like: Why does this work? What problem does this solve? How could this be improved? This foundation will serve you well as you navigate the technical landscape of AI and ML.
Step 1: Master Python Fundamentals with Practical Projects
Despite the proliferation of no-code tools, Python remains the lingua franca of AI and machine learning. To become an effective AI/ML engineer, start by building a solid Python foundation. Focus on learning the fundamentals while skipping advanced theoretical concepts initially.
The fastest way to learn Python for AI is to build automation projects as quickly as possible. This practical approach helps you gain confidence while developing useful skills.
- Start with web scraping projects to collect data
- Learn essential libraries like NumPy, Matplotlib, and Pandas
- Practice data manipulation and visualization
- Build simple scripts that automate repetitive tasks
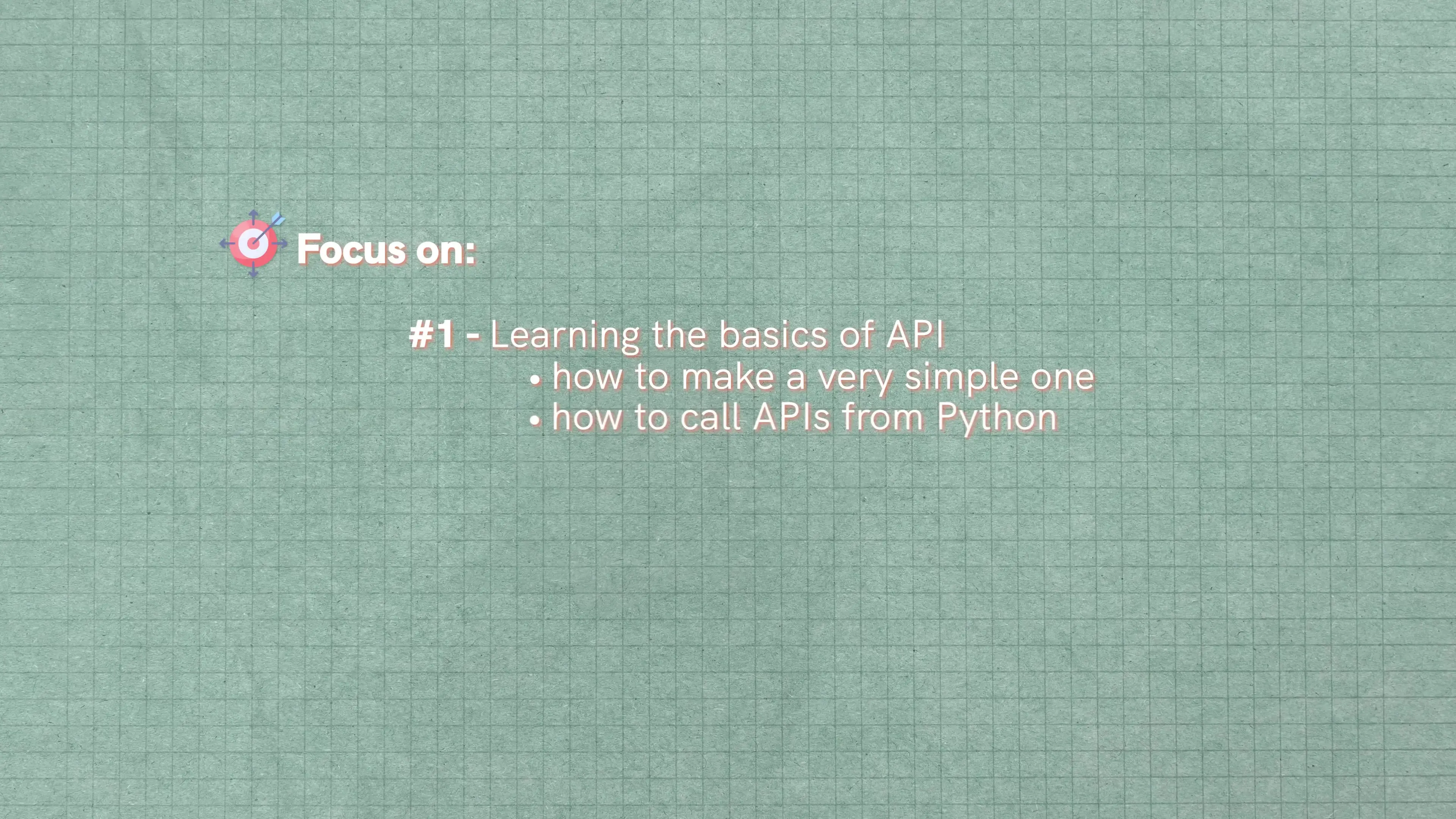
A crucial skill to develop early is working with APIs - both creating simple ones and calling external APIs from your Python code. This will prepare you for the interconnected nature of modern AI systems, which frequently communicate via APIs.
# Simple example of calling an API in Python
import requests
response = requests.get('https://api.openai.com/v1/models',
headers={'Authorization': f'Bearer {API_KEY}'})
if response.status_code == 200:
models = response.json()
for model in models['data']:
print(model['id'])
Step 2: Develop Data Literacy
The foundation of all effective AI and ML solutions is data. Without good data or the ability to work with it properly, even the most sophisticated algorithms will fail. That's why your next focus should be becoming data literate.
This step involves learning how to query, manage, and visualize data effectively. Start by mastering these essential skills:
- Basic SQL - learn joins, select statements, and fundamental query operations
- Advanced Pandas operations for data manipulation and analysis
- Data visualization techniques using libraries like Matplotlib and Seaborn
- Data cleaning and preprocessing methods
Working with large datasets will give you practical experience that's invaluable when you move to more complex AI applications. Aim to become comfortable handling various data formats, identifying patterns, and preparing data for machine learning models.
# Example of data manipulation with Pandas
import pandas as pd
import matplotlib.pyplot as plt
# Load and explore dataset
df = pd.read_csv('customer_data.csv')
# Basic data cleaning
df = df.dropna()
df['purchase_date'] = pd.to_datetime(df['purchase_date'])
# Analysis and visualization
monthly_sales = df.groupby(df['purchase_date'].dt.month)['amount'].sum()
monthly_sales.plot(kind='bar')
plt.title('Monthly Sales')
plt.show()
Step 3: Start Working with AI Models Immediately
Unlike traditional learning approaches that recommend mastering theory before practice, the current AI landscape allows beginners to build impressive applications with minimal knowledge. Leverage this to maintain motivation and see what's possible early in your journey.
Begin experimenting with these technologies immediately:
- OpenAI API for accessing models like GPT-4
- Claude API for alternative language model capabilities
- Ollama for running models locally on your computer
- LangChain and LangGraph for building basic AI agents
- Vector databases and retrieval-augmented generation (RAG)
For creating simple user interfaces and dashboards, tools like Streamlit make it easy to visualize your AI applications without deep frontend knowledge. This hands-on experience will reinforce your coding skills while teaching you the practical aspects of AI application development.
# Simple AI application using OpenAI and Streamlit
import streamlit as st
import openai
openai.api_key = st.secrets["OPENAI_API_KEY"]
st.title("Simple AI Text Generator")
user_input = st.text_input("Enter a prompt:")
if st.button("Generate") and user_input:
response = openai.Completion.create(
model="gpt-3.5-turbo-instruct",
prompt=user_input,
max_tokens=150
)
st.write(response.choices[0].text)
Step 4: Learn Core Machine Learning Fundamentals
After exploring what's possible with modern AI tools, take a step back to learn the core machine learning fundamentals. While large language models are powerful, they're often overkill for many AI tasks. Understanding traditional ML techniques will help you build more efficient, targeted solutions.
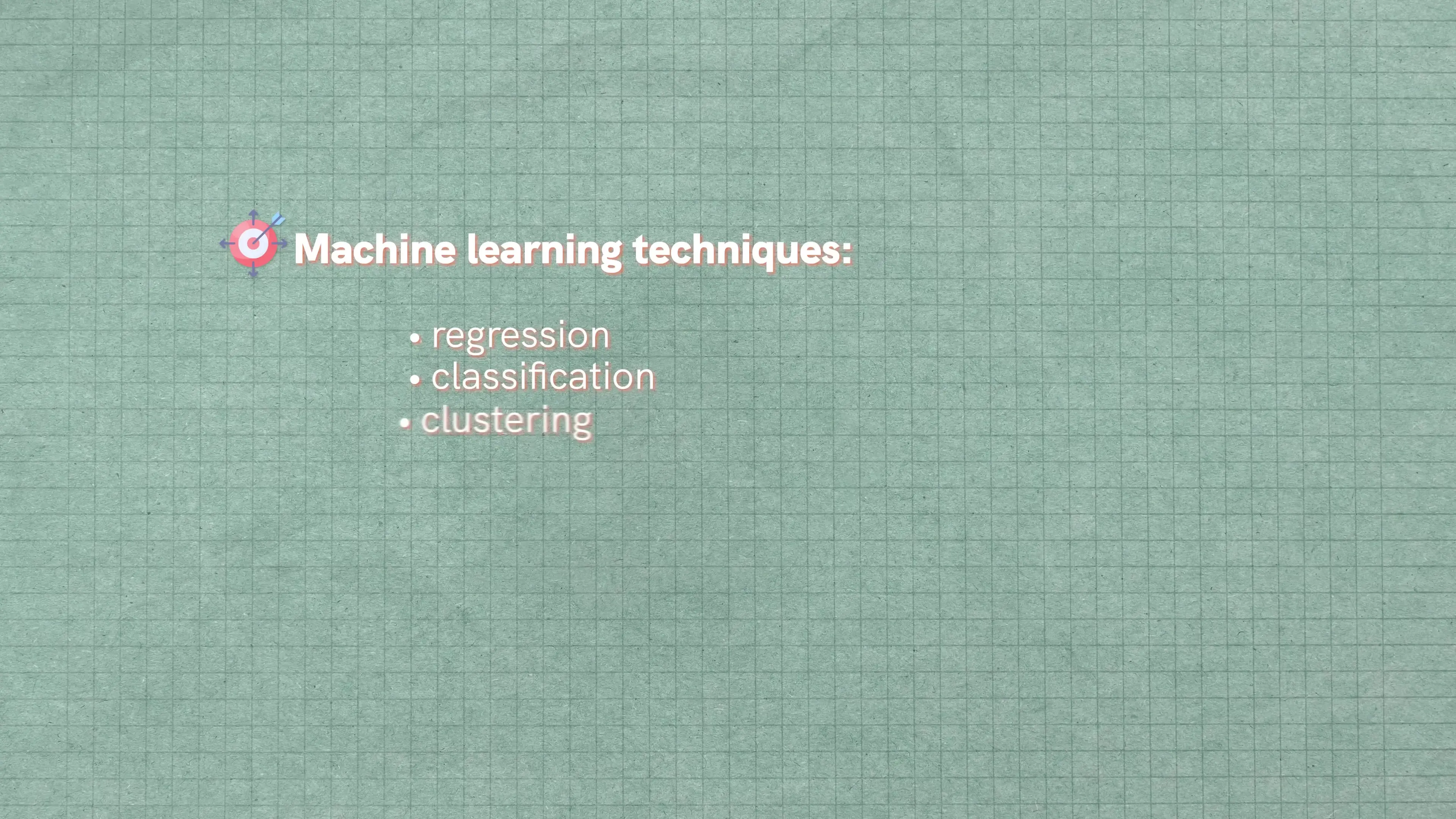
Focus on these time-tested machine learning techniques that remain valuable today:
- Regression algorithms for predicting continuous values
- Classification methods for categorizing data
- Clustering techniques for identifying patterns and groupings
- Decision trees and random forests
- Support vector machines
Libraries like scikit-learn make implementing these algorithms straightforward in Python. After mastering these basics, progress to neural networks - another fundamental technique that remains incredibly useful. Explore libraries like PyTorch and TensorFlow to build more complex machine learning applications that don't rely on LLMs.
# Simple classification example with scikit-learn
from sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3)
# Train model
model = RandomForestClassifier(n_estimators=100)
model.fit(X_train, y_train)
# Evaluate
predictions = model.predict(X_test)
accuracy = accuracy_score(y_test, predictions)
print(f"Model accuracy: {accuracy:.2f}")
Step 5: Go All-In on LLMs and AI Agents
With a solid foundation in traditional machine learning, you're now ready to dive deeper into large language models and AI agents. This is where the cutting edge of AI development is happening in 2025, and mastering these technologies can set you apart.
Start by understanding how LLMs actually work. Learn about the architecture of generative pre-trained transformers (GPT), what they can and cannot do, and their limitations. This knowledge will help you use these tools more effectively and avoid common pitfalls.
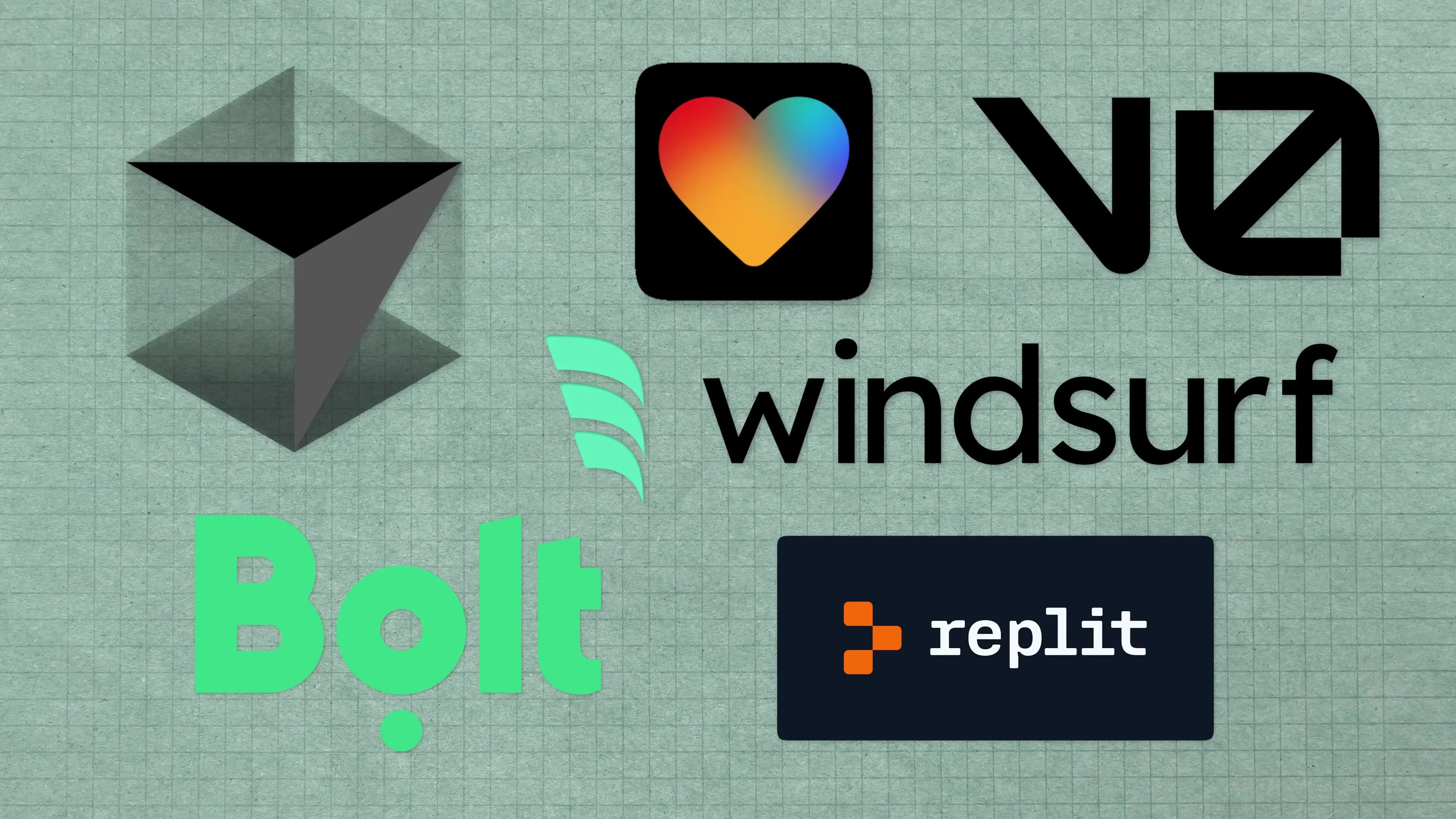
After understanding the fundamentals, explore the growing ecosystem of tools that make working with LLMs more efficient:
- Crew AI for orchestrating complex AI agent workflows
- Langflow for visual development of LLM applications
- N8N for automation and integration
- LiveKit for real-time communication capabilities
- Vector databases like Pinecone, Weaviate, or Chroma for efficient knowledge retrieval
As a developer, you'll be able to leverage these no-code and low-code tools more effectively than non-technical users, combining them with your programming skills to build sophisticated AI systems.
Step 6: Build a Portfolio of AI Projects
As you progress through your learning journey, focus on building a portfolio of diverse AI projects that demonstrate your skills. Each project should showcase different aspects of your AI and ML knowledge, from data preprocessing to model deployment.
Consider creating projects like:
- A sentiment analysis tool for social media data
- A recommendation system for products or content
- An image classification application
- A custom AI agent that can perform specific tasks
- A RAG system that answers questions from your personal knowledge base
Document your process, challenges, and solutions for each project. This portfolio will not only reinforce your learning but also serve as valuable evidence of your skills for potential employers or clients if you're pursuing a career in AI.
Step 7: Stay Current and Join the AI Community
The field of AI is evolving rapidly, with new techniques, models, and tools emerging constantly. Dedicate time to staying current with the latest developments and joining communities where you can learn from others.
- Follow AI researchers and practitioners on platforms like Twitter/X and LinkedIn
- Join Discord servers and forums focused on AI and ML
- Participate in Kaggle competitions to practice your skills
- Contribute to open-source AI projects
- Attend virtual or in-person AI meetups and conferences
Engaging with the community will expose you to different perspectives, help you discover new techniques, and provide opportunities for collaboration and feedback on your work.
Conclusion: The Engineer's Path to AI Mastery
Learning AI and machine learning in 2025 requires a balanced approach that combines foundational knowledge with hands-on experience using cutting-edge tools. By following this roadmap - from developing an engineer's mindset to mastering Python, becoming data literate, exploring AI models, learning core ML fundamentals, diving into LLMs, building a project portfolio, and engaging with the community - you'll be well-equipped to thrive in the rapidly evolving AI landscape.
Remember that the most effective learning comes from building real projects that solve actual problems. Don't get caught in tutorial hell or endless theoretical study. Instead, apply what you learn immediately, embrace the challenges, and continuously refine your skills through practical application. This approach will help you learn ml fast and develop the problem-solving abilities that truly set apart successful AI engineers.
Let's Watch!
7 Step Fast-Track Guide to Learning AI & ML in 2025
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence