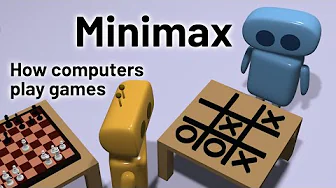
Computer game intelligence has fascinated developers for decades. Whether it's a simple game like tic-tac-toe or a complex one like chess, computers demonstrate remarkable proficiency at analyzing game states and determining optimal moves. At the heart of this capability lies the minimax algorithm—a powerful approach to game-playing artificial intelligence that allows computers to make strategic decisions even against skilled human opponents.
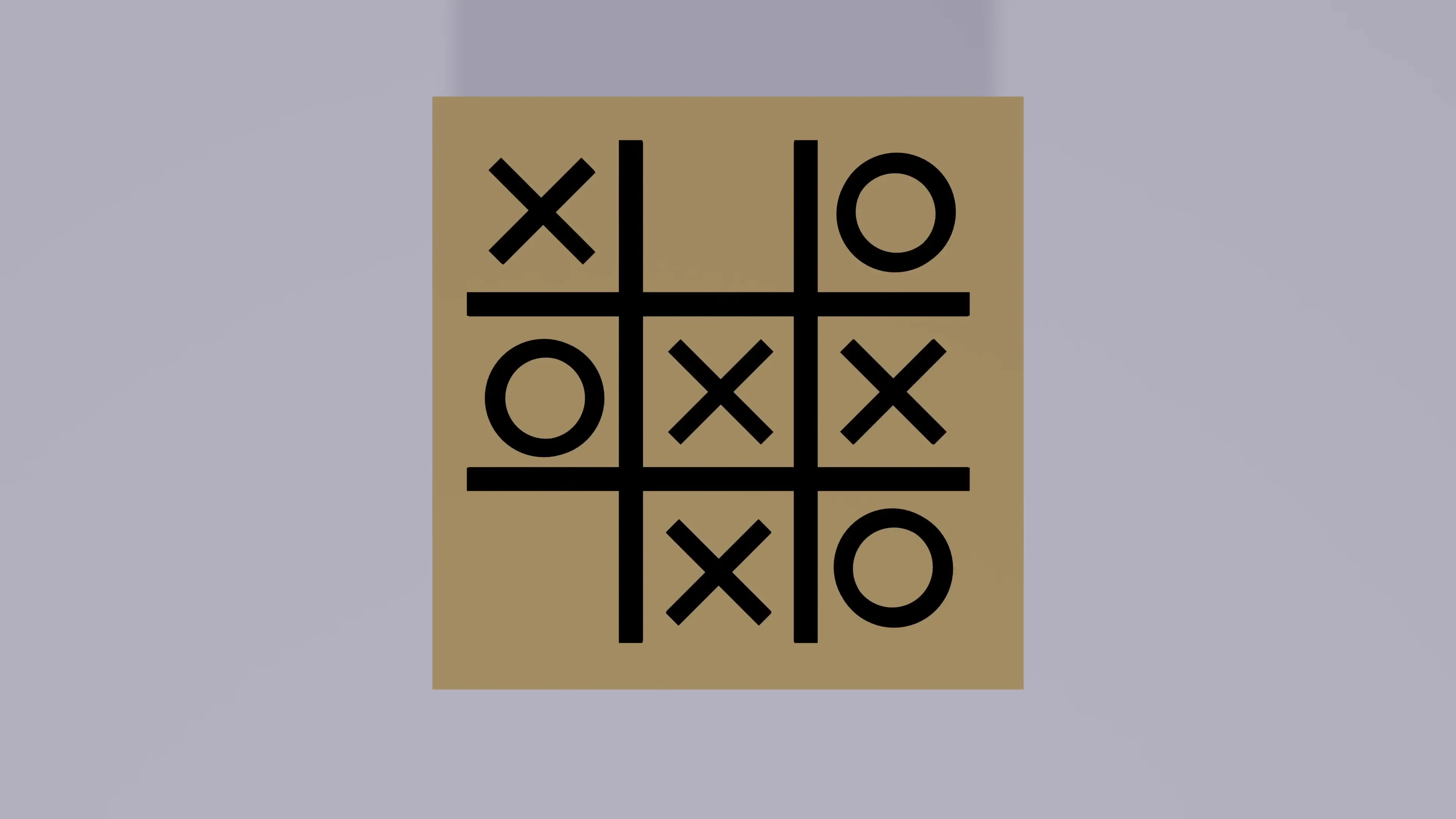
Understanding the Minimax Algorithm in Game Theory
The minimax algorithm is a decision-making algorithm used in artificial intelligence for determining optimal moves in adversarial games—games where players have opposing goals. In its essence, minimax is about ensuring the best possible outcome for yourself regardless of what your opponent does, assuming they also play optimally.
The core concept behind minimax is surprisingly straightforward: it works by recursively evaluating all possible game states that could result from all possible moves, assigning values to these states, and then choosing the move that leads to the most favorable outcome. This approach allows a computer to "think ahead" multiple moves, considering not just immediate advantages but long-term strategic positions.
The Fundamental Components of Minimax
To implement the minimax algorithm effectively, we need to understand several key components that form its foundation:
- Terminal states: Game positions where the game is over (win, loss, or draw)
- State evaluation: Assigning numerical values to game states (e.g., +1 for win, 0 for draw, -1 for loss)
- Player roles: The maximizing player (trying to achieve the highest score) and minimizing player (trying to achieve the lowest score)
- Recursive evaluation: Examining all possible future states to determine the current state's value
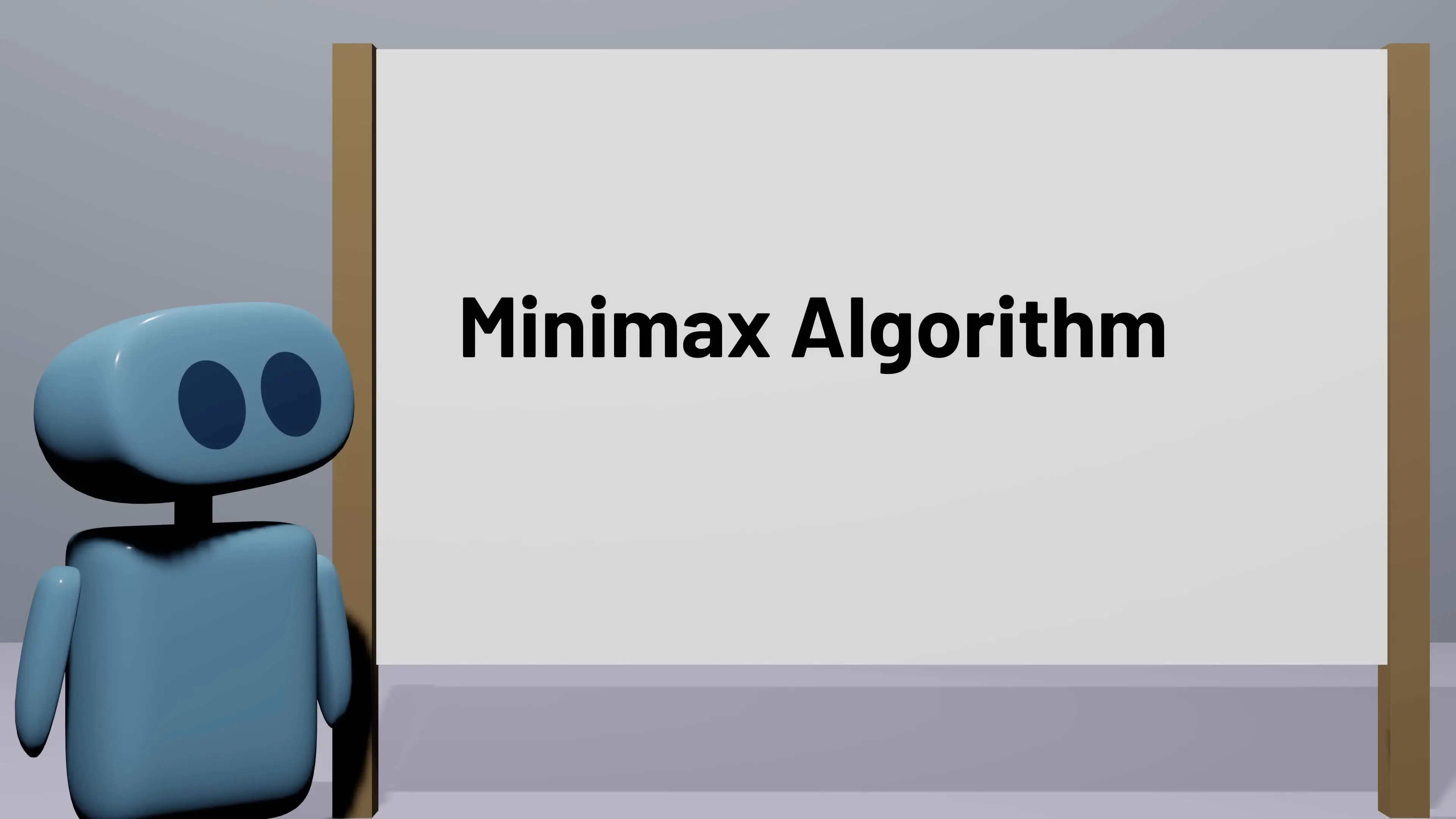
Implementing the Minimax Algorithm
Let's explore how to implement the minimax algorithm using a simple tic-tac-toe example. For our implementation, we'll need several key functions that define the game's rules and structure:
- terminal(state): Determines if a game state is terminal (game over)
- utility(state): Provides the value of a terminal state
- player(state): Identifies whose turn it is in a given state
- actions(state): Returns all possible actions in a state
- result(state, action): Returns the new state after taking an action
With these functions defined, we can implement the core minimax algorithm in JavaScript:
function minimax(state) {
// If the game is over, return the utility value
if (terminal(state)) {
return utility(state);
}
// Determine whose turn it is
const currentPlayer = player(state);
if (currentPlayer === 'max') {
// Max player tries to maximize the score
let value = -Infinity;
// Consider all possible actions
for (const action of actions(state)) {
// Get the resulting state after taking this action
const newState = result(state, action);
// Recursively find the value of this new state
const actionValue = minimax(newState);
// Update the best value if this action leads to a better outcome
value = Math.max(value, actionValue);
}
return value;
} else {
// Min player tries to minimize the score
let value = Infinity;
// Consider all possible actions
for (const action of actions(state)) {
// Get the resulting state after taking this action
const newState = result(state, action);
// Recursively find the value of this new state
const actionValue = minimax(newState);
// Update the best value if this action leads to a better outcome
value = Math.min(value, actionValue);
}
return value;
}
}
To make an actual move in the game, we need a function that uses the minimax algorithm to evaluate all possible moves and select the optimal one:
function findBestMove(state) {
const currentPlayer = player(state);
let bestValue = currentPlayer === 'max' ? -Infinity : Infinity;
let bestMove = null;
// Evaluate each possible move
for (const action of actions(state)) {
const newState = result(state, action);
const moveValue = minimax(newState);
// Update best move if this one is better
if (currentPlayer === 'max' && moveValue > bestValue) {
bestValue = moveValue;
bestMove = action;
} else if (currentPlayer === 'min' && moveValue < bestValue) {
bestValue = moveValue;
bestMove = action;
}
}
return bestMove;
}
Optimality and Limitations of the Minimax Algorithm
The minimax algorithm is theoretically perfect—when implemented correctly, it will always make the optimal move, assuming both players play optimally. For games with small search spaces like tic-tac-toe, this works exceptionally well. A computer using minimax will never lose a game of tic-tac-toe; it will either win or force a draw.
However, the algorithm's practical application faces significant challenges when applied to more complex games:
- Computational complexity: The number of states to evaluate grows exponentially with the depth of the game tree. In chess, for example, there are approximately 10^120 possible game states.
- Time constraints: Evaluating all possible moves in complex games would take an impractical amount of time, even with modern computing power.
- Memory limitations: Storing the entire game tree for complex games would require enormous amounts of memory.
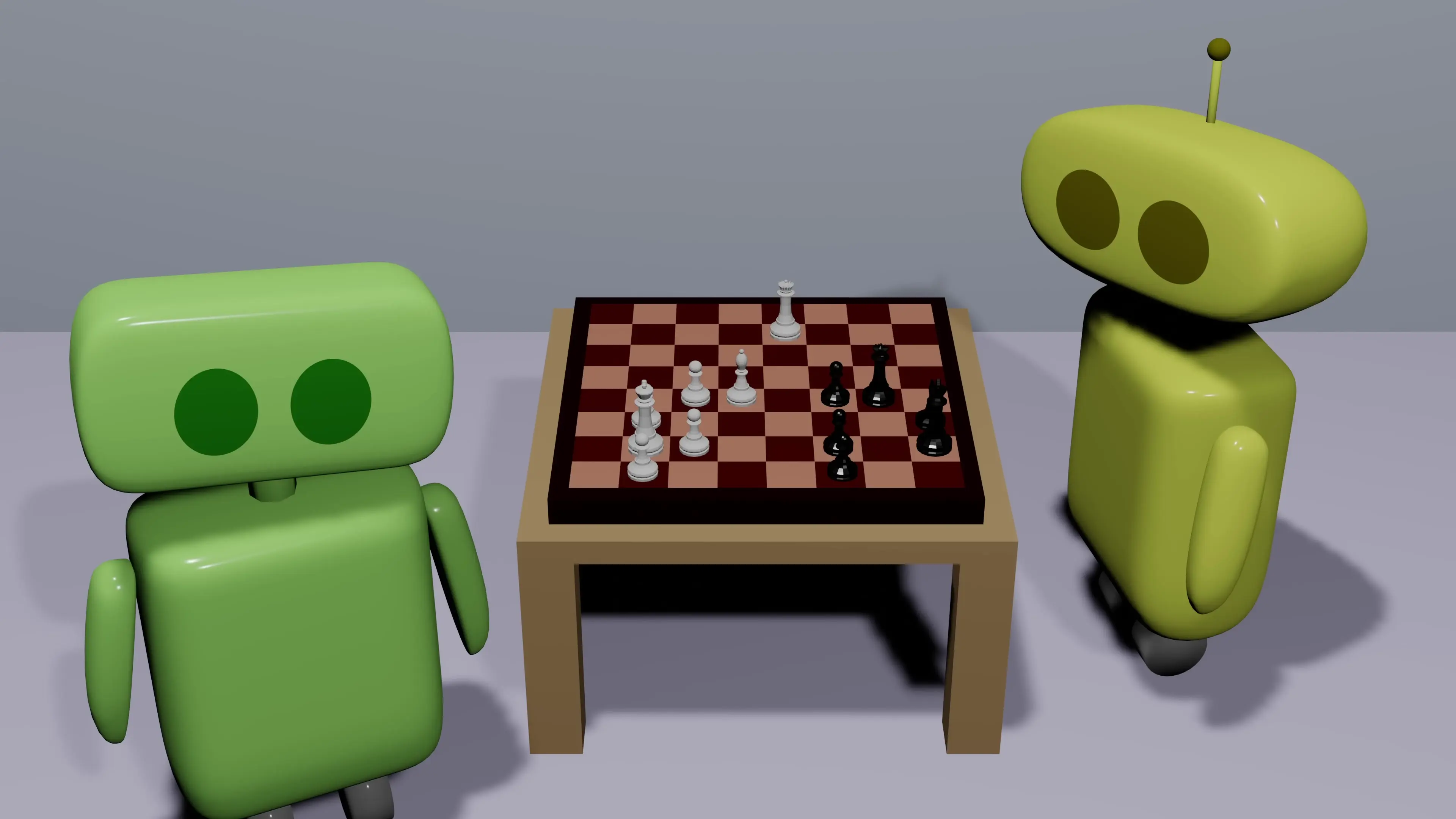
Optimizing Minimax with Alpha-Beta Pruning
To address the computational challenges of minimax, developers commonly implement an optimization technique called alpha-beta pruning. This enhancement significantly reduces the number of nodes that need to be evaluated in the game tree without sacrificing the algorithm's optimality.
Alpha-beta pruning works by maintaining two values, alpha and beta, which represent the minimum score that the maximizing player is assured of and the maximum score that the minimizing player is assured of, respectively. If during the search, a node is found that would cause the current player to make a worse move than previously examined, that entire branch can be "pruned" (skipped) without affecting the final decision.
function minimaxWithAlphaBeta(state, alpha, beta) {
if (terminal(state)) {
return utility(state);
}
const currentPlayer = player(state);
if (currentPlayer === 'max') {
let value = -Infinity;
for (const action of actions(state)) {
const newState = result(state, action);
value = Math.max(value, minimaxWithAlphaBeta(newState, alpha, beta));
// Pruning condition
alpha = Math.max(alpha, value);
if (alpha >= beta) {
break; // Beta cutoff
}
}
return value;
} else {
let value = Infinity;
for (const action of actions(state)) {
const newState = result(state, action);
value = Math.min(value, minimaxWithAlphaBeta(newState, alpha, beta));
// Pruning condition
beta = Math.min(beta, value);
if (beta <= alpha) {
break; // Alpha cutoff
}
}
return value;
}
}
Additional Optimizations for Game AI
Beyond alpha-beta pruning, there are several other techniques that can further enhance the performance of game-playing AI based on the minimax algorithm:
- Depth-limited search: Instead of searching to terminal states, limit the search to a specific depth and use a heuristic evaluation function to estimate the value of non-terminal states.
- Move ordering: Examine moves that are likely to be good first, which can dramatically improve the efficiency of alpha-beta pruning.
- Transposition tables: Store previously evaluated positions to avoid redundant calculations.
- Opening books: Pre-compute optimal moves for the early stages of games.
- Endgame databases: For certain games, pre-compute perfect play for all positions with a limited number of pieces.
Real-World Applications of the Minimax Algorithm
The minimax algorithm and its variants form the foundation of many successful game-playing AI systems:
- Chess engines like Stockfish use enhanced versions of minimax with sophisticated evaluation functions
- Game bots in digital board games and strategy games
- Educational tools that demonstrate optimal play in simple games
- Decision-making systems in competitive scenarios beyond traditional games
While modern game AI often incorporates machine learning techniques like neural networks (as seen in systems like AlphaZero), the principles of minimax remain relevant and continue to inform how we approach adversarial decision-making problems in artificial intelligence.
Conclusion: The Enduring Relevance of Minimax
The minimax algorithm represents one of the most elegant solutions in the field of artificial intelligence for game playing. Its straightforward approach of recursively evaluating game states to determine optimal moves provides a powerful framework for decision-making in adversarial scenarios.
While the algorithm faces practical limitations when applied to complex games, the optimizations we've discussed—particularly alpha-beta pruning—make it feasible to implement effective game AI even for moderately complex games. For developers interested in game AI, understanding minimax provides essential insights into how computers can make strategic decisions in competitive environments.
Whether you're implementing a simple tic-tac-toe AI or exploring more sophisticated game intelligence, the principles of minimax offer a solid foundation for creating challenging and intelligent computer opponents that can think several moves ahead—just like the best human players do.
Let's Watch!
How the Minimax Algorithm Powers AI Game Intelligence: A Developer's Guide
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence