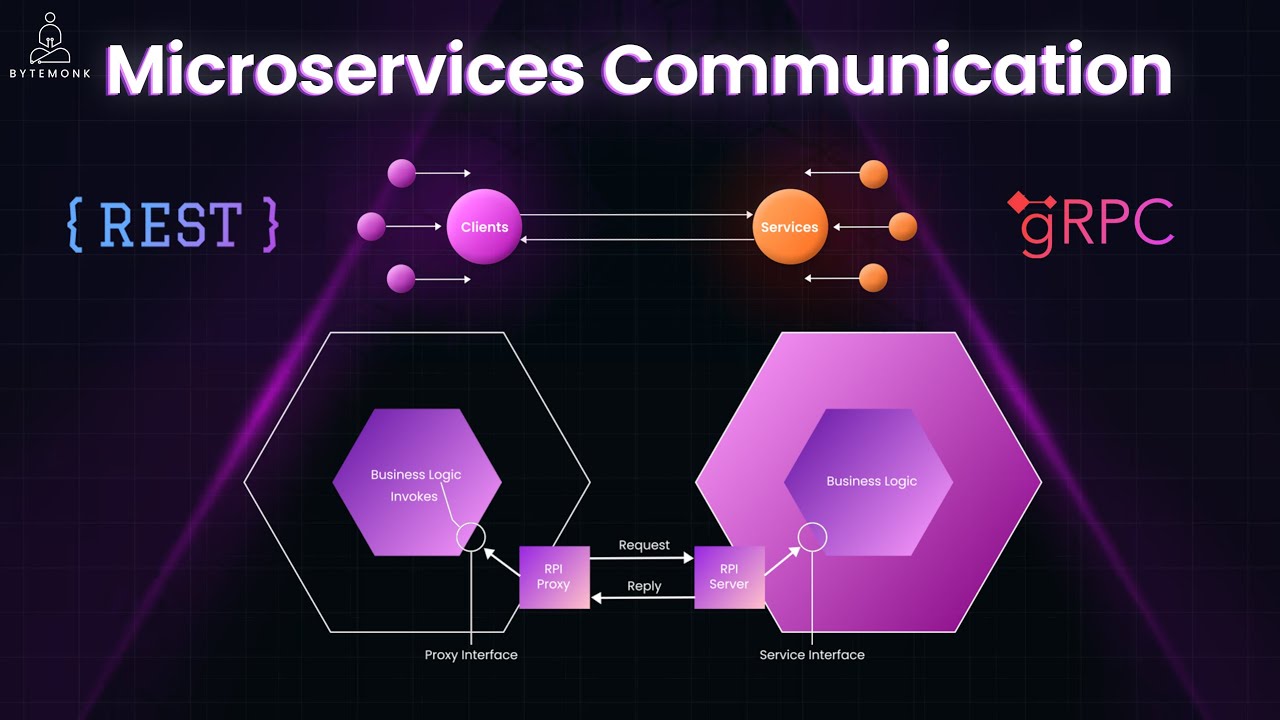
When building applications with microservices, one of the most critical decisions you'll face is determining how your services will communicate with each other. This interprocess communication (IPC) strategy can significantly impact your application's performance, scalability, and maintainability. In this comprehensive guide, we'll explore the two primary communication patterns for microservices: synchronous approaches like REST and gRPC versus asynchronous messaging using protocols like AMQP and Kafka.
Understanding Microservices Interaction Styles
Before diving into specific technologies, it's essential to understand the fundamental interaction styles between microservices. These interaction patterns form the foundation of your architecture and directly impact your application's resilience and scalability.
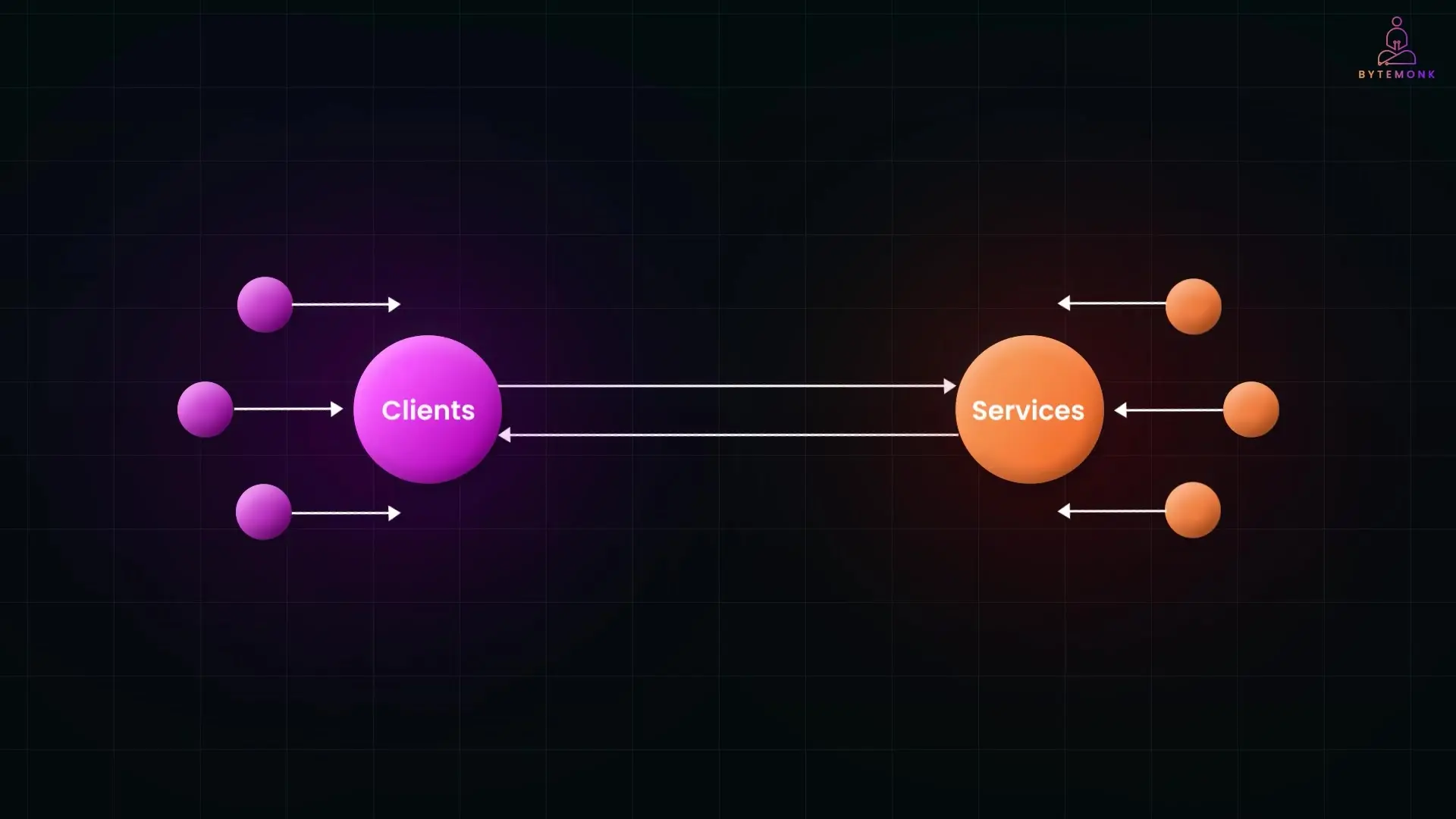
Microservice interactions can be categorized along two primary dimensions:
Dimension 1: Cardinality of Communication
- One-to-One: A single client communicates with a specific service
- One-to-Many: A client's request is processed by multiple services
Dimension 2: Timing of Communication
- Synchronous: The client sends a request and waits for an immediate response (like a phone call)
- Asynchronous: The client sends a request and continues without waiting for an immediate response (like sending an email)
These dimensions create several distinct interaction patterns that you'll encounter in microservices architectures:
One-to-One Interaction Patterns
- Request-Response (Synchronous): Client sends a request and waits for a response. This is the most common pattern but tends to create tight coupling between services.
- Asynchronous Request-Response: Client sends a request but continues processing without waiting. The service responds when ready, and the client handles the response later.
- One-way Notifications: Client sends a message to a service without expecting any reply. This is purely informational.
One-to-Many Interaction Patterns
- Publish-Subscribe (Pub/Sub): Client broadcasts a notification, and any number of interested services can listen and react.
- Publish-Async Responses: Client broadcasts a request and waits for a specified time to collect multiple responses from different services.
Most real-world microservices applications use a combination of these interaction styles depending on specific use cases and requirements.
API-First Design: The Foundation of Effective Microservices Communication
Regardless of which IPC mechanism you choose, clearly defining your APIs is crucial for successful microservices communication. This is where API-first design becomes essential.
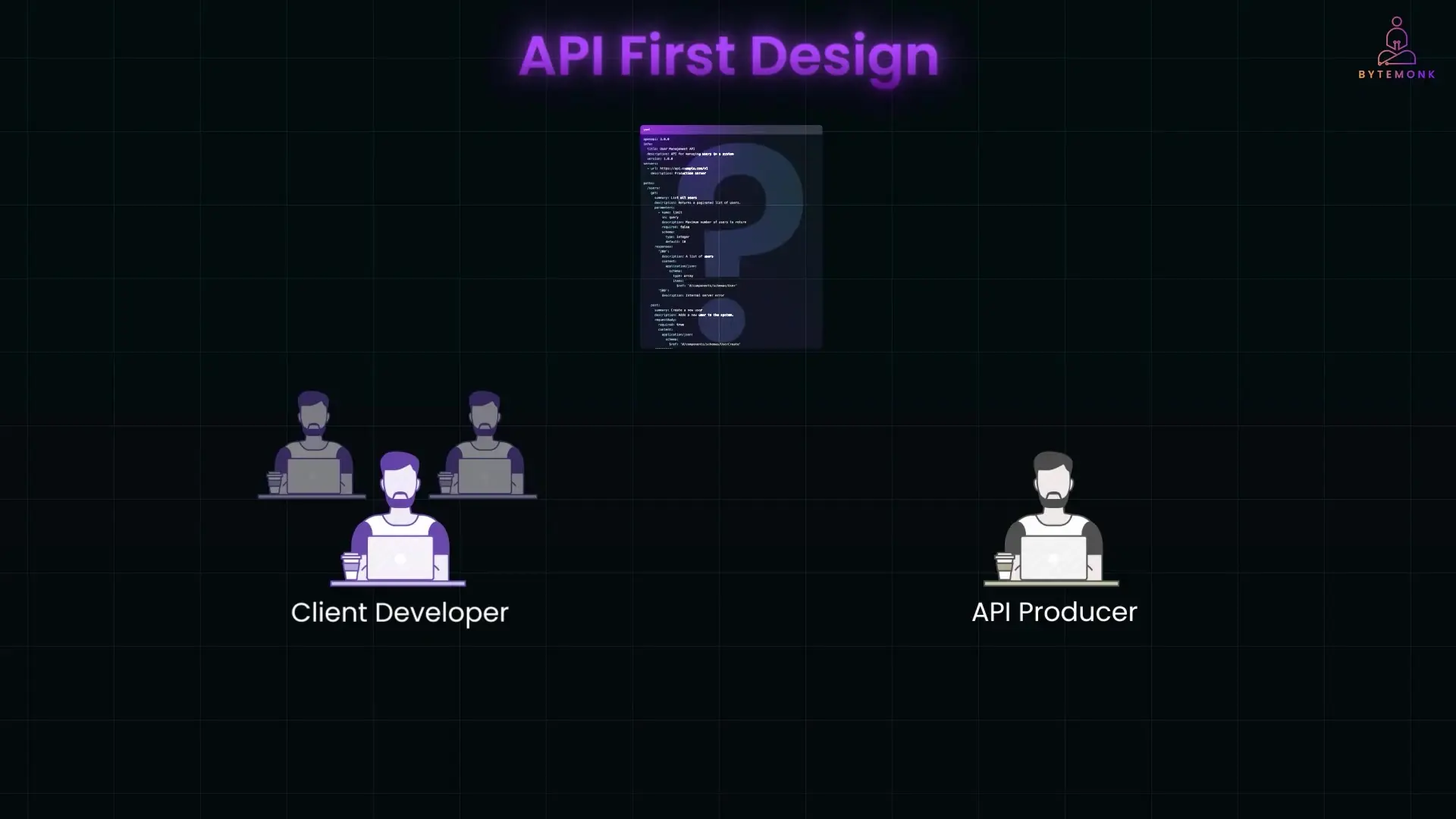
API-first design follows this workflow:
- Create clear definitions of your APIs before implementation
- Share these definitions with client developers who will consume the APIs
- Gather feedback and make adjustments to the API design
- Only after consensus is reached, begin implementing the services
This approach ensures that what you build actually matches what your clients need. Even in smaller projects, skipping this step can lead to significant integration problems. For example, backend developers working in Java and frontend engineers using Angular might confidently complete their work, only to discover that their components can't communicate because the REST APIs weren't clearly defined beforehand.
The specific API definition approach will depend on your chosen IPC mechanism:
- For messaging-based systems: Define message channels, types, and formats
- For HTTP-based APIs: Define URLs, HTTP methods (GET, POST, etc.), and request/response formats
Message Formats: The Foundation of Microservices Communication
At the core of any IPC strategy are the messages that carry data between services. Choosing the right message format is critical as it affects performance, usability, and the ability to evolve your APIs over time.
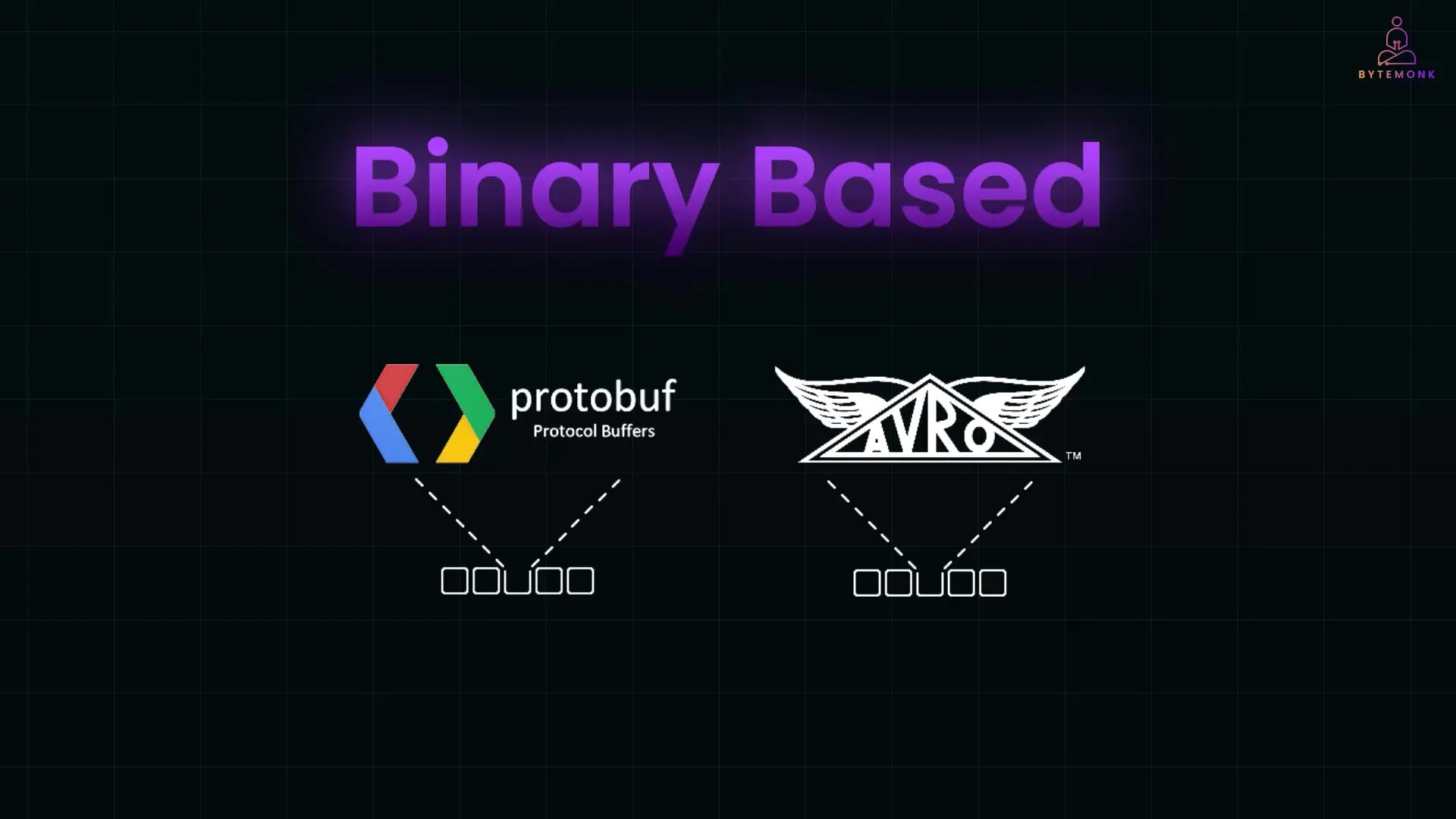
A language-neutral message format is essential in microservices architecture. While you might implement services in Java today, tomorrow you could be using Go, Python, or JavaScript. Language-specific serialization mechanisms (like Java serialization) should be avoided.
Text-Based Message Formats
Text-based formats like JSON and XML are popular choices for microservices communication because:
- Human-readable and easy to debug
- Self-describing with clearly named properties
- Flexible - services can extract only needed fields and ignore the rest
- Schema evolution friendly - adding new fields rarely breaks existing services
To define the structure of these messages, developers use schema definitions like XML Schema for XML or JSON Schema for JSON. These schemas serve dual purposes:
- Documentation of message structure
- Validation of incoming messages to ensure conformity
Binary Message Formats
Binary formats like Protocol Buffers (protobuf) and Avro offer different advantages:
- Significantly more efficient in terms of message size and processing speed
- Use Interface Definition Language (IDL) to define message structures
- Generate code for serialization/deserialization automatically
- Enforce API-first design by requiring clear definitions upfront
- Provide compile-time type checking in strongly-typed languages
There are important differences between Protocol Buffers and Avro worth noting:
- Protocol Buffers tag each field, allowing consumers to skip unknown fields easily
- Avro requires consumers to know the schema ahead of time
- Protocol Buffers generally handle API evolution more gracefully
REST vs gRPC: Comparing Synchronous Communication Options
Let's examine the two most popular synchronous communication patterns for microservices: REST and gRPC.
REST: The Ubiquitous API Style
REST (Representational State Transfer) has become the de facto standard for building APIs due to its simplicity and widespread adoption. REST APIs are built on these principles:
- Everything is treated as a resource (customers, orders, products)
- Resources are manipulated using standard HTTP methods (GET, POST, PUT, DELETE)
- Uses familiar HTTP protocol that works across all platforms
- Typically uses JSON for data exchange (though XML is also supported)
- Stateless communication - each request contains all information needed
# Example REST API endpoints for an Order service
POST /orders # Create a new order
GET /orders/{orderId} # Retrieve details about a specific order
PUT /orders/{orderId} # Update an existing order
DELETE /orders/{orderId} # Delete an order
Advantages of REST for Microservices
- Simplicity and familiarity - most developers understand REST concepts
- Excellent tooling and library support across all languages
- Works natively in browsers - ideal for web applications
- Human-readable - easier debugging and development
- Mature ecosystem with established patterns for authentication, caching, etc.
Limitations of REST for Microservices
- Less efficient than binary protocols - larger payload sizes
- No built-in code generation - requires manual client implementation
- Limited to request/response pattern - no streaming support
- Lack of strict contract enforcement compared to IDL-based approaches
- Can be chatty - may require multiple requests to accomplish complex operations
gRPC: The High-Performance Alternative
gRPC is a modern, high-performance RPC (Remote Procedure Call) framework developed by Google. It's gaining significant traction in microservices architectures for these reasons:
- Built on HTTP/2, providing multiplexing, bidirectional streaming, and header compression
- Uses Protocol Buffers by default for efficient binary serialization
- Strongly typed with code generation for multiple languages
- Supports multiple communication patterns: unary, server streaming, client streaming, and bidirectional streaming
- Built-in support for authentication, load balancing, and health checking
// Example gRPC service definition for an Order service
syntax = "proto3";
service OrderService {
// Create a new order
rpc CreateOrder(CreateOrderRequest) returns (Order);
// Get order details
rpc GetOrder(GetOrderRequest) returns (Order);
// Stream order updates in real-time
rpc WatchOrderUpdates(GetOrderRequest) returns (stream OrderUpdate);
}
message CreateOrderRequest {
string customer_id = 1;
repeated OrderItem items = 2;
}
message GetOrderRequest {
string order_id = 1;
}
message Order {
string order_id = 1;
string customer_id = 2;
string status = 3;
repeated OrderItem items = 4;
}
message OrderItem {
string product_id = 1;
int32 quantity = 2;
float price = 3;
}
Advantages of gRPC for Microservices
- Significantly better performance - smaller payload size and faster processing
- Strong typing and contract enforcement - fewer runtime errors
- Code generation - automatic client/server stubs in multiple languages
- Support for streaming - enables real-time updates and more efficient data transfer
- Built on HTTP/2 - benefits from multiplexing and header compression
- Bi-directional communication - ideal for complex interactions
Limitations of gRPC for Microservices
- Limited browser support - requires a proxy for web applications
- Steeper learning curve compared to REST
- Binary format not human-readable - requires special tools for debugging
- Less mature ecosystem than REST
- Schema changes require more careful management
Choosing Between REST and gRPC for Your Microservices
The choice between REST and gRPC isn't black and white - it depends on your specific requirements and constraints. Here's a decision framework to help you choose:
When to Choose REST
- Public APIs that need to be consumed by a wide variety of clients
- Browser-based applications without an API gateway
- When simplicity and developer familiarity are priorities
- Projects where human-readable payloads are beneficial for debugging
- When you need to leverage the extensive REST ecosystem (tools, libraries)
- For rapid prototyping and development where performance isn't critical
When to Choose gRPC
- Internal microservices communication where performance is critical
- Polyglot environments with services in multiple programming languages
- Real-time applications requiring streaming capabilities
- When strong typing and contract enforcement are important
- Low-latency, high-throughput communication channels
- IoT applications with limited bandwidth or processing power
Hybrid Approach
Many organizations adopt a hybrid approach, using both REST and gRPC depending on the specific use case:
- gRPC for internal service-to-service communication
- REST for public-facing APIs and browser integration
- API gateway to translate between protocols when needed
Performance Comparison: REST vs gRPC
Performance is often a key consideration when choosing between REST and gRPC. While specific metrics will vary based on implementation details, here are some general performance characteristics:
- Message Size: gRPC with Protocol Buffers typically produces payloads 30-40% smaller than JSON in REST
- Latency: gRPC generally offers lower latency due to HTTP/2 and binary serialization
- Throughput: gRPC can handle more requests per second under the same hardware constraints
- Connection Efficiency: gRPC's multiplexing allows multiple requests over a single connection
- CPU Usage: Protocol Buffers serialization/deserialization is less CPU-intensive than JSON parsing
However, it's important to note that for many applications, the performance difference may not be significant enough to be the deciding factor. Other considerations like developer experience, ecosystem support, and specific requirements often play a larger role in the decision.
Conclusion: Making the Right Choice for Your Microservices Architecture
Selecting the right communication pattern for your microservices is a critical architectural decision that impacts performance, developer productivity, and long-term maintainability. REST and gRPC each have their strengths and ideal use cases.
Remember that the interaction style (synchronous vs. asynchronous, one-to-one vs. one-to-many) is often more important than the specific technology. Start by understanding your communication requirements, then select the appropriate pattern and technology.
Regardless of which approach you choose, adopting an API-first design methodology will ensure that your services are well-defined, properly documented, and meet the needs of their consumers. This foundation is essential for building a robust, scalable microservices architecture that can evolve over time.
As microservices architectures continue to evolve, we're seeing increasing adoption of hybrid approaches that leverage the strengths of multiple communication patterns. The key is to understand the trade-offs and make informed decisions based on your specific requirements rather than following trends blindly.
Let's Watch!
REST vs gRPC: How to Choose the Best Communication Pattern for Microservices
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence